Variable in Java: This is another extended post of the Core Java Tutorial for Beginners Java posts. In our earlier Java post, we understood how to write the first Java program, the Hello World Program in Java. In that program, we did not use any Variables, but when we started learning the other concepts of Java, we had to understand what variables are in Java.
In this blog post on Variable in Java, we are going to discuss the concepts like:
Variable In Java
In Java, a variable is a container that holds a value of a specific data type. A variable can be considered a named memory location that stores data for later use in a program. Variables play a crucial role in programming, allowing us to store and manipulate data flexibly and dynamically. In Java, variables must be declared before they can be used, and they can be assigned a value.
Before assigning any value to a variable, let’s understand how to declare a variable first.
How to Declare a Variable in Java?
We can declare a variable in Java by following the below syntax:
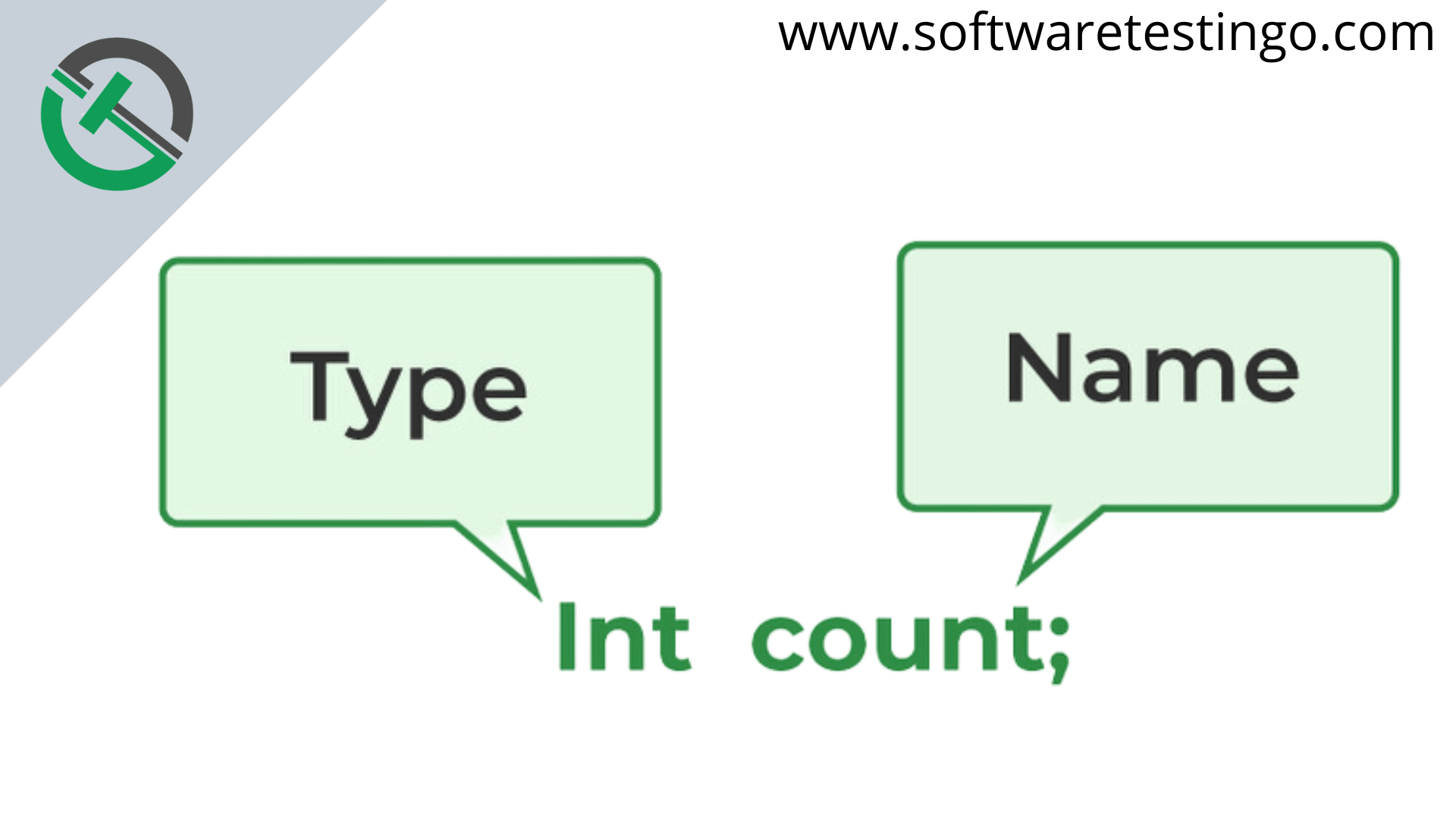
Data_type Name_of_Variable = Value;
Note: The Data_type and Name_of_Variable are compulsory while declaring a variable, but the Value is optional because, in Java, we can first declare the variable, and later on, as per the requirement, we can assign the value to that variable.
For example, to declare an integer variable called “myNumber”, you would use the following code:
int myNumber;
This code tells the Java compiler that you want to create a variable of type “int” (short for integer) and call it “myNumber”. However, the variable is not assigned any value, so it contains the default value for its data type, 0, for integers.
You can also declare and initialize a variable in a single statement using the following syntax:
data_type variable_name = initial_value;
For example, to declare and initialize an integer variable called “myNumber” to a value of 42, you would use the following code:
int myNumber = 42;
This code tells the Java compiler that you want to create a variable of type “int” and call it “myNumber”, and you want to assign it an initial value of 42.
When declaring variables in Java, variable names must follow certain Naming Conventions. Variable names can only contain letters, numbers, and underscores, and they must begin with a letter or underscore. Variable names are case-sensitive, so “myNumber” and “mynumber” are two different variable names. Additionally, variable names cannot be the same as Java keywords like “int”, “double”, “boolean”, etc.
Here are some examples of different types of variable declarations in Java:
int myNumber; // declaring an integer variable double myDouble; // declaring a double variable boolean isTrue; // declaring a boolean variable String myString; // declaring a string variable
Once you declare a variable, you can assign a value using the assignment operator, the equals sign “=”.
myNumber = 42; // assigning a value to the integer variable myDouble = 3.14; // assigning a value to the double variable isTrue = true; // assigning a value to the boolean variable myString = "SoftwareTestingo"; // assigning a value to the string variable
You can also declare and initialize a variable in a single statement like this:
int myNumber = 42; double myDouble = 3.14; boolean isTrue = true; String myString = "SoftwareTestingo";
Example: How To Declare Variable In Java
Check the program below to understand how to declare variables and assign values to those variables later <.
package softwaretestingo.java.basics; public class VariableProgram1 { public static void main(String[] args) { /*This example we are see how to declare a variable first and later * we can assign value to those variable */ //Declaring Variables int num; char ch; String str; //Assign the Value to the above variables num=2023; ch='A'; str="SoftwareTestingo"; //Let's Print The Values System.out.println("The Value Of num:-"+num); System.out.println("The Value Of ch:-"+ch); System.out.println("The Value Of str:-"+str); } }
Output:
The Value Of num:-2023 The Value Of ch:-A The Value Of str:-SoftwareTestingo
Java Variable Types
In Java, there are three types of variables, and each type of variable has a different scope and lifetime, and they are used in different ways in Java programs. Here are the 3 types of Variables in Java:
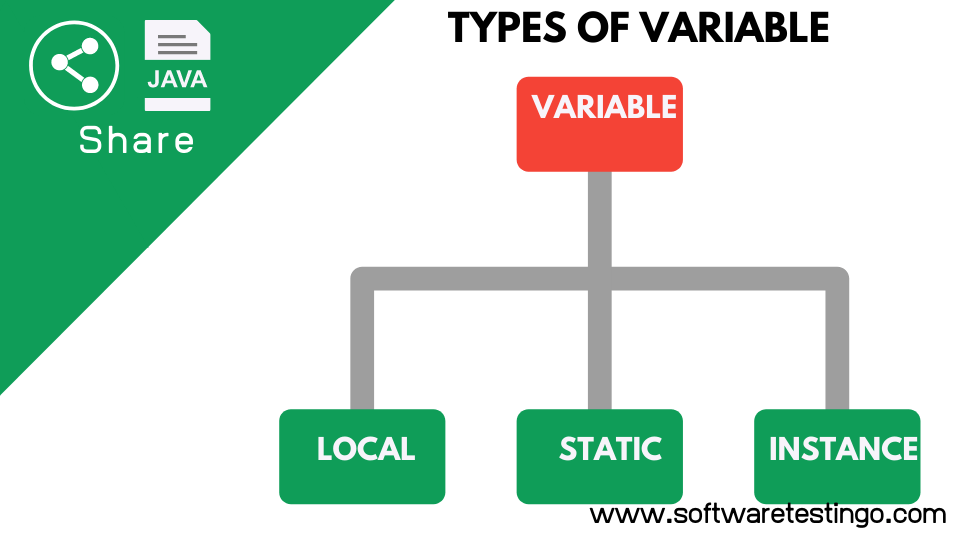
Local Variables in Java
We are declaring those variables inside the method body as local variables. The scope of the local variables is limited within the method area only because the local variable is created when the method, constructor, or block starts execution, and the variable is destroyed when you exit from the method or block. So, you can’t change their values and access them outside of that method.
Program: Local Variables Program
package softwaretestingo.java.basics; public class LocalVariable { String myVar="Outside Local Test Method"; //Local Method public void localTestMethod() { String localvar="Inside Local Test Method"; System.out.println("Local Variable str Value:-"+localvar); } public static void main(String[] args) { LocalVariable obj=new LocalVariable(); obj.localTestMethod(); //System.out.println(obj.localvar); System.out.println("Variable Value Outside Method:-"+obj.myVar); } }
Output:
Local Variable str Value:-Inside Local Test Method Variable Value Outside Method:-Outside Local Test Method
If you look at the above Java program, we can see that the local variable (localvar) can be accessed inside the local method. But if we try to access that variable outside the localTestMethod, we will get the below error. This demonstrates the scope of a variable.
When we are creating a local variable, we need to initialize it; otherwise, we will get the below error:
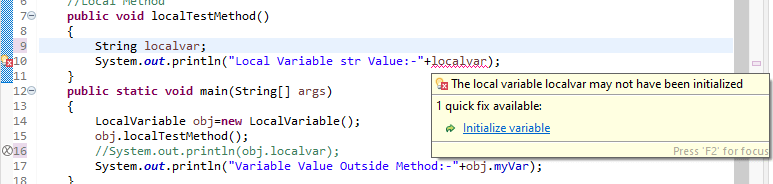
Static or Class Variables In Java
A static variable is also called a class variable because these variables are associated with the class and can be accessed by all the instances of that class. When we create a class or static variable, we declare with the static keyword at the time of declaration. One copy of the class or static variable is created for each class, and all the objects use the same copy.
Note: Static variables or Methods are executed when you create the objects.
Program: Static or Class Variables Program
package com.softwaretestingo.Basic; public class ClassVariable { public static int intVar=10; public static void main(String[] args) { ClassVariable obj1=new ClassVariable(); ClassVariable obj2=new ClassVariable(); System.out.println("Object1: "+obj1.intVar); System.out.println("Object2: "+obj2.intVar); //Initialize Class Variable Inside Main Method obj1.intVar=20; System.out.println("Updated Value: "+obj1.intVar); System.out.println("After Update the Value: "+intVar); System.out.println("By Calling From a Newly Created Object: "+obj2.intVar); } }
Output:
Object1: 10 Object2: 10 Updated Value: 20 After Update the String Value: 20 By Calling From a Newly Created Object: 20
Explanation:
From the above output, you can find that when you use a class variable, the value is the same for all instances. If you change the value by a single instance, it will reflect in all other instances.
System.out.println("Class Variable: "+intVar);
You can also access a class variable like the above, but you can not access instance and local variables like this.
Instance Variables In Java
When you create an instance (Object), that instance will create its own copy of instance variables. If you change something on a specific instance variable, then that does not affect other instance variables. This shows that each instance has its copy of instance variables.
Program: Instance Variables Program
package softwaretestingo.java.variable; public class InstanceVariable { public String instVariable="Instance Variable"; public static void main(String[] args) { InstanceVariable obj1=new InstanceVariable(); InstanceVariable obj2=new InstanceVariable(); System.out.println("Obj1 Value: "+obj1.instVariable); System.out.println("Obj2 Value: "+obj2.instVariable); //Instance Variable Value Changed obj1.instVariable="Variable Value Changed"; System.out.println("Obj1 Value: "+obj1.instVariable); System.out.println("Obj2 Value: "+obj2.instVariable); } }
Output:
Obj1 Value: Instance Variable Obj2 Value: Instance Variable Obj1 Value: Variable Value Changed Obj2 Value: Instance Variable
Explanation:
If you see the above output, we find that the instance variable value is not the same for all instances, like a class variable.
Scope of Variables
Variable Type | Declared In | Scope |
---|---|---|
Local Variables | Method, Constructor, or Block | Limited to the block in which it is declared. It can’t be accessed outside the block. It must be initialized before use. |
Instance Variables | Class, but outside of any method or block | Limited to the class in which it is declared. It can be accessed from any method within the class. Initialized to a default value. |
Class Variables (Static Variables) | Class, but outside of any method or block, and with the static keyword | Limited to the class in which it is declared. It can be accessed from any method within the class. Initialized to a default value. |
Final Words:
After going through the article about the Variables in Java, you can now understand that instance variables belong to an instance of a class, static variables belong to the class itself, and local variables are only visible within a method, constructor, or block of code. Understanding the different types of variables and their scopes is important for writing effective and efficient Java programs.
We hope this article gives a clear idea about the variables in Java, different types of Java variable types, instance variables, java class variables, and local variables java, and also discusses How to define variables in Java. We hope you enjoyed reading our post! If you have any additional thoughts or comments, please share them in the comment section below. We’d love to hear from you.