What We Are Learn:
ToggleTop 70 Java Program Interview Questions: Here, we will list down all the Java Programs.
Java Programs
Input String arr[] = {“Rama”, “Test”, “Type”, “Tata”}
Output should be – “RTTTaeyamsptatea”
Java Program Interview Concatenate Characters From Each String: This program aims to concatenate characters from each string in an input array based on their positions. It utilizes a LinkedHashMap to store the characters at each position and generates the expected output string. The output for the given input array {“Rama”, “Test”, “Type”, “Tata”} would be “RTTTaeypmsptatea”.
Input: {“Rama”, “Test”, “Type”, “Tata”}
Output: RTTTaeyamsptatea
For More Java Interview Programs, you Can check Here.
Concatenate Characters From Each String
package com.softwaretestingo.interviewprograms; import java.util.ArrayList; import java.util.LinkedHashMap; import java.util.Map; public class InterviewPrograms1 { // For More Programs Visit: https://www.softwaretestingo.com/ /*Input String arr[] = {"Rama", "Test", "Type", "Tata"} Output should be - "RTTTaeyamsptatea" */ public static void main(String[] args) { String arr[] = {"Rama", "Test", "Type", "Tata"}; System.out.println("Inpu Array Is: "+ "{\"Rama\", \"Test\", \"Type\", \"Tata\"}"); LinkedHashMap<Integer, ArrayList<String>> map= new LinkedHashMap<>(); String ans=""; for (int i = 0; i<=arr.length-1; i++) { String word= arr[i]; for (int j = 0; j <=word.length()-1; j++) { ArrayList<String> list= new ArrayList<>(); if(map.containsKey(j)) { ArrayList<String> list1= map.get(j); list1.add(String.valueOf(word.charAt(j))); map.put(j, list1); } else { list.add(String.valueOf(word.charAt(j))); map.put(j, list); } } } for(Map.Entry<Integer, ArrayList<String>> e:map.entrySet()) { // System.out.print(e.getKey()+"" +e.getValue()); ArrayList<String> l1= e.getValue(); for (int i = 0; i <=l1.size()-1; i++) { ans=ans+l1.get(i); } } System.out.println("Expected Output Is: "+ans); } }
Output:
Inpu Array Is: {"Rama", "Test", "Type", "Tata"} Expected Output Is: RTTTaeyamsptatea
Alternative Way:
package com.softwaretestingo.interviewprograms; public class InterviewPrograms1_1 { public static void main(String[] args) { String[] strArray = { "Rama", "Test", "Type", "Tata" }; int maxLength = 0; for (String str : strArray) { maxLength = str.length(); } String result = ""; for (int i = 0; i < maxLength; i++) { for (String str : strArray) { if (i < str.length()) { result = result + str.charAt(i); } } } System.out.println(result);//RTTTaeyamsptatea } }
Output:
RTTTaeyamsptatea
Input: today110is210my310interview
Output: yadot110si210ym310weivretni
Reverse Characters Only From String: This Java program aims to reverse the order of non-numeric substrings within a given input string while keeping the numeric substrings unchanged.
Reverse Characters Only With Out Changing The Digits
Input – today10is20my30interview.
Output- yadot10si20ym30weivretni.
Solution 1:
package com.softwaretestingo.interviewprograms; public class InterviewPrograms2 { // For More Programs Visit: https://www.softwaretestingo.com/ /* Input - today10is20my30interview. * Output- yadot10si20ym30weivretni. */ public static void main(String[] args) { String s = "today110is210my310interview"; String[] arr = s.split("(?<=\\D)(?=\\d)|(?<=\\d)(?=\\D)"); for (int i = 0; i< arr.length; i++) { if(!Character.isDigit(arr[i].charAt(0))) { arr[i] = String.valueOf(new StringBuilder(arr[i]).reverse()); } } System.out.println(String.join("", arr)); } }
Output:
Input: today110is210my310interview Output: yadot110si210ym310weivretni
Solution 2:
package com.softwaretestingo.interviewprograms; public class InterviewPrograms2_1 { /* Input - today10is20my30interview. * Output- yadot10si20ym30weivretni. */ public static void main(String[] args) { String input = "today10is20my30interview"; System.out.println("Input: "+input); StringBuilder res = new StringBuilder ( ); StringBuilder temp = new StringBuilder ( ); for ( int i = 0 ; i < input.length ( ) ; i ++ ) { char ch = input.charAt (i); if ( Character.isDigit (ch) ) { while ( i < input.length ( ) && Character.isDigit ( input.charAt ( i ) ) ) { temp.append ( input.charAt ( i ) ) ; i ++ ; } res.append ( temp ) ; temp.setLength ( 0 ) ; i-- ; } else { temp.insert ( 0 , ch ) ; } } res.append(temp); System.out.println("Output: "+res.toString()); } }
Output:
Input: today10is20my30interview Output: yadot10si20ym30weivretni
String s =“lekhale” How many times “le” is Getting repeated
Input: lekhale
Output: 2
Find the Number Of Times Substring Appear In a String: This Java program aims to count the number of times a specific substring (“le” in this case) is repeated within a given input string.
Find the Number Of Times substrings appear in a String
Solution 1:
package com.softwaretestingo.interviewprograms; public class InterviewPrograms3 { /* * String s =“lekhale” How many times “le” is Getting repeated, write a code to * find out using java. */ public static void main(String[] args) { String s="lekhale"; System.out.println("Input: "+s); //This will print the length of the string (7) //System.out.println(s.length()); //this will give you the total length of the string after replace le with space(3) //System.out.println((s.replaceAll("le","").length())); //Need to divide the number of character int result = (s.length()-s.replaceAll("le","").length())/2; System.out.println("Output: "+result); } }
Output:
Input: lekhale Output: 2
Solution 2:
package com.softwaretestingo.interviewprograms; public class InterviewPrograms3_1 { /* * String s =“lekhale” How many times “le” is Getting repeated, write a code to * find out using java. */ public static void main(String[] args) { String s = "lekhale"; int count = 0; int index = s.indexOf("le"); while (index != -1) { count++; index = s.indexOf("le", index + 1); } System.out.println("The substring 'le' is repeated " + count + " times in the given string."); } }
Output:
The substring 'le' is repeated 2 times in the given string.
Solution 3:
package com.softwaretestingo.interviewprograms; public class InterviewPrograms3_2 { /* * String s =“lekhale” How many times “le” is Getting repeated, write a code to * find out using java. */ public static void main(String[] args) { String s = "lekhale"; int occurence = s.split("le").length; System.out.println("The substring 'le' is repeated " + occurence + " times in the given string."); } }
Output:
The substring 'le' is repeated 2 times in the given string.
Input string: “dadeadrs”
Output: “dddaaers”
Order Character Based On Number Of Occurrences: This Java program aims to rearrange the characters in a given input string by grouping them based on their appearances. The program achieves this by creating a new list and iterating over the input string.
It checks if a character is already present in the list, and if not, it adds the character to the list and looks for additional occurrences in the remaining characters. Finally, the program prints the characters in the modified order.
If you are preparing for your next job, then we recommend that you check this blog post on java programs for interview questions.
Order Character Based On Number Of Occurrences
package com.softwaretestingo.interviewprograms; import java.util.LinkedList; import java.util.List; public class InterviewPrograms4 { /* * Input string: "dadeadrs" * Output: "dddaaers" */ public static void main(String[] args) { String s="dadeadrs"; List <Character> ls = new LinkedList<>( ); for(int i=0;i<s.length();i++) { if(!ls.contains(s.charAt(i))) { ls.add(s.charAt(i)); for (int j=i+1; j<s.length(); j++ ) { if ( s.charAt ( i ) == s.charAt ( j ) ) { ls.add (s.charAt (j)) ; } } } } for ( Character character : ls) { System.out.print (character) ; } } }
Output:
dddaaers
Input: Welcome to Mis2is2ip2i Bla4k Adam
Output:Welcome to Mississippi Blaaaak Adam
This Java program aims to expand the characters in a given input string based on the preceding digit. If a digit is encountered in the input string, the program repeats the preceding character by the value of the digit minus one. The expanded string is then printed as the output.
Follow this link for the Java programs for interview questions.
Print the Preceding Character By the Value
package com.softwaretestingo.interviewprograms; public class InterviewPrograms6 { public static void main(String[] args) { String input = "Welcome to Mis2is2ip2i Bla4k Adam"; System.out.println("Input: "+input); StringBuilder res = new StringBuilder(); for ( int i = 0 ; i <input.length(); i ++ ) { char currentCharacter = input.charAt(i); if ( Character.isDigit (currentCharacter)) { int count = Character.getNumericValue (currentCharacter) ; char charToAppend = input.charAt (i-1); while (count -- >1) { res.append (charToAppend); } } else { res.append (currentCharacter); } } System.out.println("Output:"+res); } }
Output:
Input: Welcome to Mis2is2ip2i Bla4k Adam Output:Welcome to Mississippi Blaaaak Adam
Input= abbcccdeee
Output= a1b2c3d1e3
Print Character And Count Of a Word: This Java program aims to count the occurrences of each character in a given input string and generate an output string that represents each character followed by its count.
The specific transformation is applied to the input string “abbcccdeee” to obtain the output string “a1b2c3d1e3”.
Check frequently asked Java programs for interviews.
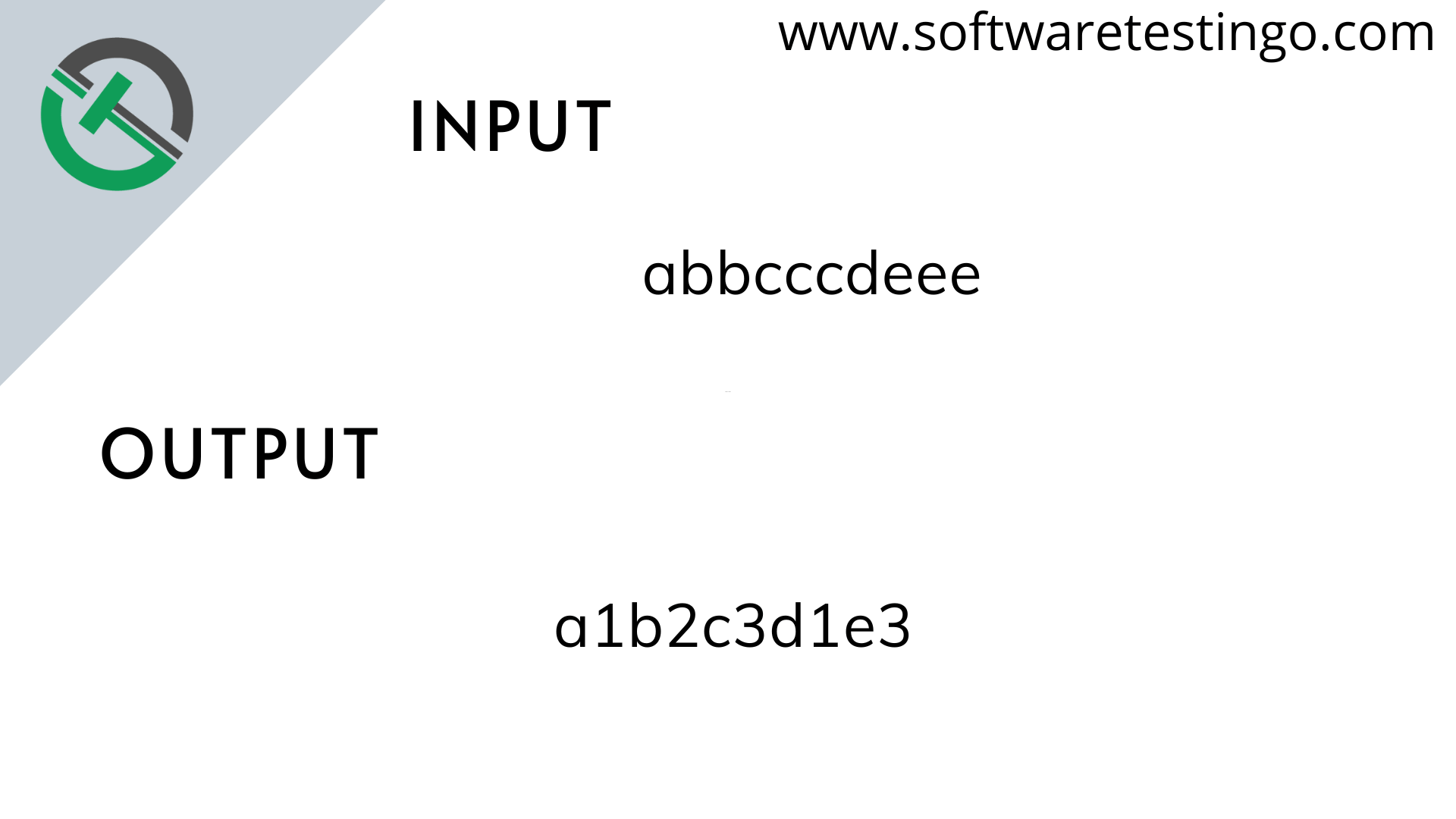
Print Character And Count Of a Word
package com.softwaretestingo.interviewprograms; import java.util.LinkedHashMap; import java.util.Map; import java.util.Map.Entry; public class InterviewPrograms7 { /* * Input= abbcccdeee * Output= a1b2c3d1e3 */ public static void main(String[] args) { String s="abbcccdeee"; char [ ] ch= s.toCharArray(); Map<Character, Integer> maps= new LinkedHashMap<Character,Integer>(); for (Character c:ch) { Integer count = maps.get(c); if (count != null) { maps.put (c, ++count); } else { maps.put(c,1); } } StringBuffer sb = new StringBuffer(); for (Entry< Character , Integer > entry : maps.entrySet()) { sb.append(entry.getKey()).append(entry.getValue()); } System.out.println (sb); } }
Output:
a1b2c3d1e3
Here’s a step-by-step explanation of what we are achieving through this program:
- The program starts by defining a class named “InterviewPrograms7” in the package “com.softwaretestingo.interviewprograms”.
- The main method is declared as public and static, allowing it to be accessed without creating an instance of the class.
- A string variable named “s” is declared and assigned the value “abbcccdeee”.
- The input string “s” is converted into a character array using the toCharArray() method.
- A LinkedHashMap named “maps” is created to store characters as keys and their respective counts as values. LinkedHashMap is used to preserve the insertion order.
- The program iterates over each character in the character array using an enhanced for loop.
- Within the loop, it checks if the character is already present as a key in the “maps” LinkedHashMap.
- If the character is present, it retrieves the current count from the “maps” LinkedHashMap and increments it by 1.
- If the character is not present, it adds the character as a key to the “maps” LinkedHashMap and sets its count to 1.
- After iterating through all the characters in the input string, a StringBuffer named “sb” is created.
- The program iterates over each entry (key-value pair) in the “maps” LinkedHashMap using a for-each loop.
- Within the loop, it appends the character and its count to the StringBuffer “sb”.
- Finally, the program prints the StringBuffer “sb” as the compressed output string.
Through this program, we achieve the goal of transforming the input string “abbcccdeee” into its compressed representation “a1b2c3d1e3” by counting the occurrences of each character and appending their counts to the respective characters.
Explanation:
package com.softwaretestingo.interviewprograms; public class InterviewPrograms11_1 { /* * Input string =abbcccdeee * Output= a1b2c3d1e3 */ public static void main(String[] args) { StringBuilder sb = new StringBuilder(); String str = "abbcccdeee"; String[] strArray = str.split(""); int i = 0 ; while ( i < strArray.length ) { int counter=0 ; int j = i ; while ( j < strArray . length ) { if ( ! strArray [ i ] .equals ( strArray [ j ] ) ) break; counter++ ; j++; } sb.append ( strArray [ i ] ) . append ( counter ) ; i = j ; } System.out.println (sb.toString()); } }
Create a StringBuilder: We create a StringBuilder object named sb to construct the final output string.
StringBuilder sb = new StringBuilder();
Initialize the input string: We assign the string value “abbcccdeee” to the variable str. This serves as the input for our program.
Split the input string into an array: We split the input string into an array of individual characters using the split(“”) method. Each character is stored as a separate element in the strArray.
String[] strArray = str.split("");
Process the input array and generate the output string: We use nested loops to iterate through the input array strArray. The outer loop iterates over each character, and the inner loop counts the consecutive occurrences of the current character. Once a different character is encountered, we break out of the inner loop. The character and its count are appended to the StringBuilder sb. The outer loop then continues from the next distinct character.
int i = 0; while (i < strArray.length) { int counter = 0; int j = i; while (j < strArray.length) { if (!strArray[i].equals(strArray[j])) break; counter++; j++; } sb.append(strArray[i]).append(counter); i = j; }
Print the output string: We print the generated output string by converting the StringBuilder sb to a string using the toString() method.
System.out.println(sb.toString());
In summary, this program takes an input string, counts the consecutive occurrences of each character, and generates an output string by concatenating each character with its respective count. The program demonstrates the usage of loops, string manipulation, and the StringBuilder class in Java.
package com.softwaretestingo.interviewprograms; public class InterviewPrograms38 { /* * Input = AAABBBBCC * Output = A3B4C2 */ public static void main(String[] args) { String str = "AAABBBBCC"; int length = str.length ( ) ; // Created an object of a StringBuffer class . StringBuffer sb= new StringBuffer(); int count = 1; // counter for counting number of occurrences // input String // length of a String . for ( int i=0; i<length; i++) { // if i reaches at the end then append all and break the loop if (i == length-1) { sb.append(str.charAt(i)+""+count); break ; } // if two successive chars are equal then increase the if (str.charAt(i) == str.charAt(i+1)) { count ++ ; } else { // else append character with its count sb.append(str.charAt(i)+""+count); count = 1 ; // resetting the counter } } // String representation of a StringBuffer object System.out.println(sb.toString()); } }
Output
A3B4C2
Solution: Answer
Input { “Hi” , “maven” , “selenium ” , “java” }
Output { “Hi”, “java”, “maven” , “selenium” }
WAP to Sort Array Of String According To String Length: The program InterviewPrograms52 aims to sort an array of strings based on the lengths of the strings in ascending order.
In this program, we have used the concepts of For Loop and If Statement. For better understanding, you can follow the tutorials which we have already shared by clicking on the below link:
Sort Array Of String According To Strig Length
package com.softwaretestingo.interviewprograms; import java.util.Arrays; public class InterviewPrograms52 { /* * Input { "Hi" , "maven" , "selenium " , "java" } * Output{ "Hi", "java", "maven" , "selenium" } */ public static void main(String[] args) { String ip[]={"Hi","maven","selenium","java"}; for(int i=0;i<ip.length;i++) { for(int j=i+1;j<ip.length;j++) { if(ip[i].length()>ip[j].length()) { String temp=ip[j]; ip[j]=ip[i]; ip[i]=temp; } } } System.out.println(Arrays.toString(ip)); } }
Output
[Hi, java, maven, selenium]
Input is a phone number Ex : 1234567890
Output: (123)45-67-890
The program InterviewPrograms50 aims to format a given phone number by inserting parentheses and hyphens at specific positions to make it more readable.
Format a Phone Number in Human-Readable View
package com.softwaretestingo.interviewprograms; public class InterviewPrograms50 { /* * Input is a phone number Ex : 1234567890 * Output: (123)45-67-890 */ public static void main(String[] args) { String input="1234567890"; String output=input.replaceFirst("(\\d{3})(\\d{2})(\\d{2})(\\d+)", "($1)$2-$3-$4"); System.out.println(output); String output1="("+input.substring(0,3)+")"+input.substring(3,5)+"-"+input.substring(5,7)+"-"+input.substring(7); System.out.println(output1); } }
Output
(123)45-67-890 (123)45-67-890
Alternative Way 1:
This Java program takes an input phone number and formats it into a specific pattern. The input is a 10-digit long phone number (represented as phoneFmt), and the desired output is in the format (XXX)-XX-XX-XXX, where X represents a digit from the original number.
package com.softwaretestingo.interviewprograms; import java.text.DecimalFormat; public class InterviewPrograms50_1 { /* * Input is a phone number Ex : 1234567890 * Output: (123)45-67-890 */ public static void main(String[] args) { long phoneFmt = 123456789L; //get a 12 digits String, filling with left '0' (on the prefix) DecimalFormat phoneDecimalFmt = new DecimalFormat("0000000000"); String phoneRawString= phoneDecimalFmt.format(phoneFmt); java.text.MessageFormat phoneMsgFmt=new java.text.MessageFormat("({0})-{1}-{2}"); //suposing a grouping of 3-3-4 String[] phoneNumArr={phoneRawString.substring(0, 3), phoneRawString.substring(3,6), phoneRawString.substring(6)}; System.out.println(phoneMsgFmt.format(phoneNumArr)); } }
Output:
(012)-345-6789
Input: “minimum”
Output: n=1
The program InterviewPrograms49 aims to find the first non-repeated character in a given string (s). The program uses two nested loops to compare each character of the string with every other character to count the number of occurrences (distinct) of each character.
Find the first non-repeated character in a word in Java
package com.softwaretestingo.interviewprograms; public class InterviewPrograms49 { /* wap to find the first non repeating character. */ public static void main(String[] args) { String s="minimum"; int distinct=0; for(int i=0;i<s.length();i++) { for(int j=0;j<s.length();j++) { if(s.charAt(i)==s.charAt(j)) { distinct++; } } if(distinct==1) { System.out.println(s.charAt(i)+"--"+distinct+"\n"); break; } String d=String.valueOf(s.charAt(i)).trim(); s.replaceAll(d,""); distinct=0; } } }
Output
n--1
Input=”1222bbbbcccaaaammmmm”
Output=”1222cccbbbbaaaammmmm”
Wap To Print Characters As Per Number Of Occurrences: The program InterviewPrograms47 aims to sort a given string based on the frequency of characters in ascending order.
Print Characters As Per the Number Of Occurrences
package com.softwaretestingo.interviewprograms; import java.util.HashMap; import java.util.Map; public class InterviewPrograms47 { /* * String input="1222bbbbcccaaaammmmm" * String output="1222cccbbbbaaaammmmm" * * We need to sort the string base on character size */ public static void main(String[] args) { System.out.println(sortFreq("1222bbbbcccaaaammmmm")); } public static String sortFreq(String str) { StringBuilder sb = new StringBuilder(); Map<Character, Integer> hm = new HashMap<>(); for (Character c : str.toCharArray()) { hm.put(c, hm.getOrDefault(c, 0) + 1); } System.out.println("frequency map of the String is " + hm); hm.entrySet().stream() .sorted(Map.Entry. <Character, Integer>comparingByValue()) .forEach(a -> {Character key = a.getKey(); int value = a.getValue(); for (int i = 0; i < value; i++) { sb.append(key); } }); System.out.println(sb); return sb.toString(); } }
Output
frequency map of the String is {1=1, a=4, 2=3, b=4, c=3, m=5} 1222cccaaaabbbbmmmmm 1222cccaaaabbbbmmmmm
Alternative 1
In this program InterviewPrograms47_1, we are sorting a given string based on the frequency of characters in ascending order.
package com.softwaretestingo.interviewprograms; import java.util.HashMap; import java.util.List; import java.util.Map; import java.util.TreeMap; import java.util.stream.Collectors; public class InterviewPrograms47_1 { /* * String input="1222bbbbcccaaaammmmm" * String output="1222cccbbbbaaaammmmm" * * We need to sort the string base on character size */ public static void main(String[] args) { String input = "1222cccbbbbaaaammmmmmm"; Map<String, Integer> outputMap = new HashMap<>(); for (String letter : input.split("")) { outputMap.compute(letter, (k,v)->v==null?1:v+1); } System.out.println(outputMap); Map<Integer, List<String>> inversed = outputMap .entrySet() .stream().collect(Collectors.groupingBy(Map.Entry::getValue, Collectors.mapping(Map.Entry::getKey, Collectors.toList()))); inversed.values().forEach(list->list.sort(null)); new TreeMap<>(inversed).forEach((k,v)->v.forEach(letter-> System.out.print(letter.repeat(k)))); } }
Output
{a=4, 1=1, b=4, 2=3, c=3, m=7} 1222cccaaaabbbbmmmmmmm
Find Sum of numbers in given strings.
Input : “Welcome[21], Java1How are you78”
OutPut : 100 [21+1+78]
Find Sum of digits in given strings.
Input : “Welcome[21], Java1How are you78”
OutPut : 19 [2+1+1+7+8]
Sum Of Digits Of a String: This Java program achieves two tasks:
- Finding the sum of numbers in the given string:
- The program takes an input string containing alphanumeric characters and special characters.
- It extracts all the numeric values from the string and calculates their sum.
- For the input string “Welcome[21], Java1How are you78”, the output will be 100 (21 + 1 + 78).
- Finding the sum of digits in the given numbers:
- The program takes the same input string as above and extracts the numeric values.
- For each extracted number, it calculates the sum of its digits.
- The digits of the numbers are summed, and the final output is the sum of these digits.
- For the input string “Welcome[21], Java1How are you78”, the output will be 19 (2 + 1 + 1 + 7 + 8).
Sum Of Digits Of a String
package com.softwaretestingo.interviewprograms; import java.util.Arrays; import java.util.List; import java.util.stream.Collectors; public class InterviewPrograms46 { /* * 1. Find Sum of numbers in given strings. * Input : "Welcome[21], Java1How are you78" * OutPut : 100 [21+1+78] * * 2. Find Sum of digits in given strings. * Input : "Welcome[21], Java1How are you78" * OutPut : 19 [2+1+1+7+8] */ public static void main(String[] args) { InterviewPrograms46 main = new InterviewPrograms46(); String testData = "Welcome[21], Java1How are you78"; List<Integer> integers = main.getIntegers(testData); System.out.println("Sum of numbers : " + integers.stream().reduce(0, (n1, n2) -> n1 + n2)); System.out.println("Sum of digits of numbers : " + integers.stream().filter(num->num>0).map(num -> main.sumOfDigits(num)).reduce(0, (n1, n2) -> n1 + n2)); } private Integer sumOfDigits(Integer number) { int sum = 0; while (number > 0) { sum += number % 10; number = number / 10; } return sum; } private List<Integer> getIntegers(String testData) { return Arrays.asList(testData.split(" ")).stream().map(word -> getNumber(word)).collect(Collectors.toList()); } private Integer getNumber(String data) { String num = ""; try { for (Character ch : data.toCharArray()) { if (Character.isDigit(ch)) num += String.valueOf(ch); } } catch (Exception ex) { return 0; } return num.length() > 0 ? Integer.parseInt(num) : 0; } }
Output
Sum of numbers : 100 Sum of digits of numbers : 19
Input INDIA
Output INDIIA
Print Duplicate Character Based on its Occurrence Times: The given Java program takes an input string “INDIA” and finds and duplicates each character in the output string based on its occurrence. The output will be “INDIIA,” where each character that appears more than once in the input string is duplicated in the output string.
Print Duplicate Character Based on its Occurrence Times
package com.softwaretestingo.interviewprograms; import java.util.HashMap; public class InterviewPrograms45 { /* * Input INDIA * Output INDIIA */ public static void main(String[] args) { findDuplicate("INDIA"); } public static void findDuplicate(String str) { String newStr = ""; HashMap<Character,Integer> hm = new HashMap<Character,Integer>(); for(int i=0;i<str.length();i++) { if(hm.containsKey(str.charAt(i))) { newStr = newStr+str.charAt(i)+str.charAt(i); } else { newStr = newStr+str.charAt(i); hm.put(str.charAt(i),1); } } System.out.println(newStr); } }
Output
INDIIA
Input: “HELLO WORLD”
Output: “HExxxO WORxxxD”
WAP to Replace a Character Sequence With Increase Order: The given Java program manipulates a given input string “HELLO WORLD” to replace each occurrence of the letter ‘L’ with a sequence of ‘x’ characters. The output will be “HExxxO WORxxxD,” where the number of ‘x’ characters increases for each occurrence of ‘L.’
Replace a Character Sequence With Increase Order
package com.softwaretestingo.interviewprograms; public class InterviewPrograms44 { /* * input: "HELLO WORLD" * Output: "HExxxO WORxxxD" */ public static void main(String[] args) { String s="HELLO WORLD"; String z=""; int count=1; for(int i=0;i<s.length();i++) { if(s.charAt(i)=='L') { for(int j=0;j<count;j++) { z=z+'x'; } count++; } else { z=z+s.charAt(i); } } System.out.println(z); } }
Output
HExxxO WORxxxD
Input= AB2C99423A
Output =A2B9C9A423
Separate Two Characters With a Digit: The given Java program takes an input string AB2C99423A and rearranges its characters to separate alphabetic and non-alphabetic characters. The resulting output is A2B9C9A423.
Separate Two Characters With a Digit
package com.softwaretestingo.interviewprograms; public class InterviewPrograms41 { /* * String input= AB2C99423A * String output =A2B9C9A423 */ public static void main(String[] args) { String s = "AB2C99423A"; char[] cArr = s.toCharArray(); char resultArr[] = new char [cArr.length*2]; int evenCount = 0 ; int oddcount = 1 ; for ( int i=0 ; i<s.length(); i++ ) { if ( Character.isAlphabetic(cArr[i])) { resultArr [evenCount] = cArr[i]; evenCount = evenCount+2; } else if(!Character.isAlphabetic(cArr[i])) { resultArr [oddcount]= cArr[i]; oddcount = oddcount+2 ; } } System.out.println(resultArr); } }
Output
A2B9C9A4
Alternative Way 1:
The given Java program takes an input string AB2C99423A and rearranges its characters to separate alphabetic and numeric characters. The resulting output is A2B9C9A423.
package com.softwaretestingo.interviewprograms; public class InterviewPrograms41_1 { /* * String input= AB2C99423A * String output =A2B9C9A423 */ public static void main(String[] args) { String inpu = "AB2C99423A"; String charac = ""; String intege = ""; String result=""; for(int i=0;i<inpu.length();i++) { if(Character.isLetter(inpu.charAt(i))) { charac = charac + inpu.charAt(i); } else if(Character.isDigit(inpu.charAt(i))) { intege = intege + inpu.charAt(i); } } int index1=0, index2=0; for(int i=0;i<inpu.length();i++) { if(i%2==0 && charac.length()>index1) { result = result + charac.charAt(index1); index1++; } else { if(intege.length()>index2) { result = result + intege.charAt(index2); index2++; } } } System.out.println(result); } }
Output
A2B9C9A423
Input : Aut@oma#tion@#
Output: Aut@oma#tion..
Replace Last Two Special Character With Dots: This Java program aims to remove the last alphanumeric character from a given input string and replace it with dots.
Replace Last Two Special Character With Dots
package com.softwaretestingo.interviewprograms; public class InterviewPrograms40 { /* * Input string : Aut@oma#tion@# * Output: Aut@oma#tion.. * Input maybe any string, but only the last alphanumeric character should be removed */ public static void main(String[] args) { String s = "Aut@oma#tion@#"; String [ ] newstring = s.split(""); int count = 0 ; String last = ""; for ( int i = s.length()-1; i>=0;i--) { if (!newstring[i].matches("[a-zA-Z]")) { count++; last=last+"."; } else { break; } } System.out.print(s.substring(0, s.length()-count)+last); } }
Output
Aut@oma#tion..
Input : { ‘a’, ‘b’, ‘c’, ‘d’, ‘e’ }
Output: true
Check Consecutive Character Sequence in an Array: This Java program aims to check whether the elements of an integer array form a consecutive sequence starting from a specific value. It does so by converting the characters from a given char array into integers and then checks for consecutive values in the resulting integer array.
Check Consecutive Character Sequence in an Array
package com.softwaretestingo.interviewprograms; public class InterviewPrograms37 { public static void main(String[] args) { char[] JavaCharArray = { 'a', 'b', 'c', 'd', 'e' }; int val[]=new int[5]; for(int i=0;i<5;i++) { val[i]=(int)JavaCharArray[i]; } System.out.println(checker(val)); } public static boolean checker(int[] array) { int array_element_count=array.length; int f_val=array[0]; int count=0; for(int i=0;i<5;i++) { if(array[i]==(f_val+i)) { count++; } } if(count==array_element_count) { return true; } return false; } }
Output
true
Input – Hi I am Naveen I need and answer
Output – answer and deen I Naveen ma I Hi
Reverse Every Third Word In a String: This Java program rearranges the words in the input string in a specific pattern. It reverses every third word in the input string and then prints the modified string.
Reverse Every Third Word In a String
package com.softwaretestingo.interviewprograms; public class InterviewPrograms29 { /* * Input - Hi I am Naveen I need and answer * Output - answer and deen I Naveen ma I Hi */ public static void main(String[] args) { String str = "Hi I am Naveen I need and answer"; String[] sp=getPattern(str); // print Array for(int j=sp.length-1; j>=0; j--) { System.out.print(sp[j] + " "); } } private static String[] getPattern(String revStr) { String[] splitStr = revStr.split(" "); for ( int j = splitStr.length-3; j>=0 ; j=j-3) { String temp=splitStr[j]; StringBuilder sb = new StringBuilder(temp); splitStr[j] = sb.reverse().toString(); } return splitStr ; } }
Output
answer and deen I Naveen ma I Hi
Alternative 1
This Java program rearranges the words in the input string in a specific pattern. It reverses every third word in the input string and then prints the modified string.
package com.softwaretestingo.interviewprograms; public class InterviewPrograms29_1 { /* * Input - Hi I am Naveen I need and answer * Output - answer and deen I Naveen ma I Hi */ public static void main(String[] args) { String input = "Hi I am Naveen i need and answer"; String[] inputArr=input.split(" "); String outputStr = "" ; int j = 0 ; for (int i=inputArr.length-1; i>=0 ; i-- ) { ++ j ; String indexedStr = ""; if (j%3==0) { for (int k=inputArr[i].length()-1 ; k>=0 ; k--) { indexedStr = indexedStr + inputArr[i].charAt(k); } } else { indexedStr=inputArr[i]; } outputStr = outputStr + " " + indexedStr; } System.out.println(outputStr.trim()); } }
Output
answer and deen i Naveen ma I Hi
Here’s a breakdown of the program:
- Define the main method:
- The entry point of the program is the main method.
- Initialize variables and process the input string:
- The input string input is initialized with the value “Hi I am Naveen I need and answer”.
- The program splits the input string into words using the space character as a delimiter, and the result is stored in the inputArr array.
- A string outputStr is initialized to store the final modified string.
- Loop through the words in reverse order:
- The program iterates through the inputArr array from the last element to the first using a for loop.
- Determine whether to reverse the word:
- For each word, a counter j is incremented.
- If the counter j is divisible by 3 (i.e., every third word), the characters of the word are reversed and stored in indexedStr.
- If the counter j is not divisible by 3, the word remains unchanged, and the original word is stored in indexedStr.
- Build the modified string:
- The indexedStr (either reversed or original) is appended to the outputStr with a space.
- Print the modified string:
- After processing all words in the input string, the program prints the outputStr after trimming any leading or trailing spaces.
Overall, this program reverses every third word in the input string and then prints the modified string. The output will be “answer and deen I Naveen ma I Hi” for the given input string. The program uses basic looping and string manipulation to achieve the desired result.
Input – Ramakant
Output – R1a1m1a2k1a3n1t1
Print Character and Occurrences Count: This Java program takes an input string and generates a new string where each character is followed by the count of its occurrences in the original string. For instance, for the input string “Ramakant,” the output will be “R1a1m1a2k1a3n1t1.”
Print Character and Occurrences Count
package com.softwaretestingo.interviewprograms; public class InterviewPrograms35 { /* * Input - Ramakant * Output - R1a1m1a2k1a3n1t1 * I have to take input string and in output will print no of occurance after each character */ public static void main(String[] args) { String name = "Ramakant"; String text = ""; for (int i = 0; i < name.length(); i++) { char[] ch = name.toCharArray(); text = text + ch[i] + ((name.substring(0, i).length())-(name.substring(0, i).replace(String.valueOf(ch[i]),"").length())+1); } System.out.println(text); } }
Output
R1a1m1a2k1a3n1t1
Input :Hello world,welcome to my world,my world
Output:world Hello ,world my to welcome ,world my
Reverse String Based on Commas: This Java program takes an input string containing comma-separated phrases, and for each phrase, it reverses the order of words within that phrase while keeping the phrases themselves in the same order. It achieves this without using any predefined functions for string manipulation.
Reverse String Based on Commas
package com.softwaretestingo.interviewprograms; public class InterviewPrograms30 { /* * Input :Hello world,welcome to my world,my world * Output:world Hello ,world my to welcome ,world my * my Condition: without using predefined function */ public static void main(String[] args) { String str1 = "Hello world,welcome to my world,my world"; String arr[] = str1.split(","); String res = ""; for(int i=0;i<arr.length;i++) { String[] de = arr[i].split(" "); for(int j=de.length-1;j>=0;j--) { res = res + de[j] + " "; } res = res + ","; } res = res.substring(0,res.length()-1); System.out.println(res); } }
Output
world Hello ,world my to welcome ,world my
Alternative 1
This Java program takes an input string containing comma-separated phrases. For each phrase, it reverses the order of words within that phrase while keeping the phrases themselves in the same order. The program achieves this without using any predefined functions for string manipulation, relying on basic looping and string concatenation.
package com.softwaretestingo.interviewprograms; public class InterviewPrograms30_1 { /* * Input :Hello world,welcome to my world,my world * Output:world hello ,world my to welcome ,world * my Condition: without using predefined function */ public static void main(String[] args) { //inbuilt function split(); String input = "Hello world,welcome to my world,my world" ; String output = ""; //inbuilt function1 String[] str_array= input.split(","); int len1= str_array.length; //System.out.println("Level 1 array length "+len1); System.out.println("Input String == "+input); for (String s : str_array) { //System.out.println("Level words "+s); String[] sub_str_array = s.split(" ") ; int len2= sub_str_array.length; //System.out.println("Level 2 array length "+len2); if(len2>1) { String gap=" "; int c=0; for (int i=len2; i>0; i-- ) { if (i==len2) { //System.out.println("Level 2 Reverse words "+sub_str_array[i-1]); output=output+sub_str_array[i-1]; } else { output=output+gap+sub_str_array[i-1]; } c++; } } //if1 end //add , after each string except for the last one in each loop if(s==str_array[len1-1]) {} else output= output+","; } //End of 1st for loop System.out.println("Output String == "+output); } //main function //class end }
Output
Input String == Hello world,welcome to my world,my world Output String == world Hello,world my to welcome,world my
List a[ ] = [2, 1,1,4,5,5,6,7]
Expected output : [2,4,6,7] (Delete Duplicate Ones)
Print Unique Digits Of an Array: This Java program takes an input array of integers, removes any repeated numbers, and creates a new list containing only the unique numbers. It achieves this using a HashMap to track the occurrence of each number.
Print Unique Digits Of an Array
package com.softwaretestingo.interviewprograms; import java.util.ArrayList; import java.util.Arrays; import java.util.HashMap; import java.util.List; public class InterviewPrograms34 { /* * List a[ ] = [2, 1,1,4,5,5,6,7] * Expected output : [2,4, 6,7] * Numbers which gets repeated we should remove and make output as above I know using HashSet * we get as [2, 1,4,5 , 6,7] But interviewee asked about deleting repeated ones */ public static void main(String[] args) { Integer arr[]= {2,1,1,4,5,5,6,7}; List <Integer> ar = Arrays.asList(arr); HashMap<Integer,Integer> hm = new HashMap<>(); ar.forEach(e ->{if(hm.containsKey(e)) { hm.remove(e); } else { hm.put(e,1); }}); ar = new ArrayList<>(hm.keySet()); System.out.println(ar); } }
Output
[2, 4, 6, 7]
Alternative 1:
This Java program takes an input array of integers, removes any repeated numbers, and creates a new set containing only the unique numbers. It achieves this using a HashSet to efficiently track and eliminate duplicates.
package com.softwaretestingo.interviewprograms; import java.util.HashSet; public class InterviewPrograms34_1 { /* * List a[ ] = [2, 1,1,4,5,5,6,7] * Expected output : [2,4, 6,7] * Numbers which gets repeated we should remove and make output as above I know using HashSet * we get as [2, 1,4,5 , 6,7] But interviewee asked about deleting repeated ones */ public static void main(String[] args) { Integer ar[]= {2,1,1,4,5,5,6,7}; HashSet<Integer> hs= new HashSet<Integer>(); for(int s1:ar) { if(hs.add(s1)==false) { hs.remove(s1); } } System.out.println(hs); } }
Output
[2, 4, 6, 7]
Alternative Way 2:
This Java program takes an input list of integers, removes any numbers that occur more than once, and creates a new list containing only the unique numbers. It achieves this by using a HashMap to count the occurrences of each number in the input list.
package com.softwaretestingo.interviewprograms; import java.util.ArrayList; import java.util.Arrays; import java.util.HashMap; import java.util.List; public class InterviewPrograms34_2 { /* * List a[ ] = [2, 1,1,4,5,5,6,7] * Expected output : [2,4, 6,7] * Numbers which gets repeated we should remove and make output as above I know using HashSet * we get as [2, 1,4,5 , 6,7] But interviewee asked about deleting repeated ones */ public static void main(String[] args) { ArrayList<Integer> intList= new ArrayList<>(Arrays.asList(2,1,1,4,5,5,6,7)); HashMap<Integer,Integer> getCount=new HashMap<Integer,Integer>(); for ( int c : intList ) { if (getCount.containsKey(c)) { getCount.put(c, getCount.get(c)+1); } else { getCount.put(c,1); } } List<Integer> newIntList= new ArrayList<Integer>(); for (int c:intList ) { if ( getCount.get(c)<2) { newIntList.add (c); } } System.out.println(newIntList); } }
Output
[2, 4, 6, 7]
Input = “GDP in 2016 has fallen from 6.8% to 4.5%”
Output = “GDP in 6102 has fallen from 8.6% to 5.4%”
Reverse Digits Only From a String: This Java program takes an input string and reverses the order of digits within the numbers present in the string. It achieves this by iterating through the input string character by character and using two StringBuffer objects: rev to store the reversed string and number to store the digits of a number temporarily.
Reverse Digits Only From a String
package com.softwaretestingo.interviewprograms; public class InterviewPrograms32 { /* * Input = “GDP in 2016 has fallen from 6.8% to 4.5%” * Output = “GDP in 6102 has fallen from 8.6% to 5.4%” * Only reverse the numbers of a string. */ public static void main(String[] args) { String s="GDP in 2016 has fallen from 6.8% to 4.5%"; StringBuffer rev=new StringBuffer(); StringBuffer number=new StringBuffer(); int length=s.length(); for(int i=0;i<length;i++) { Character c=s.charAt(i); if(c.isDigit(c) || c.equals('.')) { number.append(c); } else if(!number.isEmpty()) { String rev1=""; for(int j=number.length()-1;j>=0;j--) { rev1=rev1+number.charAt(j); } rev.append(rev1+c); number.delete(0, number.length()); } else { rev.append(c); } } System.out.print(rev); } }
Output
GDP in 6102 has fallen from 8.6% to 5.4%
input :list1–[3,R,M,4,89,f]
OutPut:list2–[3,4,89,M,R,f]
WAP to Move Digits to Left and Characters to Right: This Java program aims to reorder the elements in the input array list1 such that all numeric values are moved to the left side, and all non-numeric values (characters) are moved to the right side, while maintaining their relative order within each group.
Move Digits to Left and Characters to Right
package com.softwaretestingo.interviewprograms; import java.util.Arrays; public class InterviewPrograms23 { /* * input :list1--[3,R,M,4,89,f] * OutPut:list2--[3,4,89,M,R,f] */ public static void main(String[] args) { String[] arr = pushAllCharToRight(new String[] {"3" ,"R", "M" , "4" , "89" , "f"}); System.out.println(Arrays.toString(arr)); } public static String[] pushAllCharToRight(String[] array) { int i= 0 ; int j = array.length-1; while (i < j) { if (Character.isLetter(array[j].charAt(0))) { j--; } try { Integer.parseInt(array[i]); i++; } catch (NumberFormatException e) { String temp = array[j]; array[j--] = array[i]; array[i] = temp; } } return array; } }
Output
[3, 89, 4, M, R, f]
Alternative 1:
This Java program aims to reorder the elements in the lst ArrayList such that all numeric values are moved to the left side, and all non-numeric values (characters) are moved to the right side, while maintaining their relative order within each group. The program then sorts the ArrayList containing the elements.
package com.softwaretestingo.interviewprograms; import java.util.ArrayList; import java.util.Arrays; public class InterviewPrograms23_1 { /* * input :list1--[3,R,M,4,89,f] * OutPut:list2--[3,4,89,M,R,f] */ public static void main(String[] args) { ArrayList lst=new ArrayList(); ArrayList lstSorted=new ArrayList(); lst.add(3); lst.add("R"); lst.add("M"); lst.add(4); lst.add(89); lst.add("f"); String [] stringLst=new String[lst.size()]; for(int i=0;i<lst.size();i++) { if(lst.get(i).getClass().getSimpleName().equalsIgnoreCase("Integer")) { stringLst[i]=Integer.toString((int)lst.get(i)); } else stringLst[i]=(String)lst.get(i); } Arrays.sort(stringLst); for(int b=0;b<stringLst.length;b++) { lstSorted.add(stringLst[b]); } System.out.println("Given List: "+lst); System.out.println("Sorted List: "+lstSorted); } }
Output
Given List: [3, R, M, 4, 89, f] Sorted List: [3, 4, 89, M, R, f]
Input:{01IND02AUS03ENG}
Output:
{
“01”: IND,
“02”:AUS,
“03”:ENG
}
O/P should be printed in json array with key value pair
WAP to Convert String into JSON Array with key-Value Pairs: This Java program achieves the goal of converting a given input string {01IND02AUS03ENG} into a JSON array with key-value pairs. The program takes the input string, processes it, and produces the output JSON representation.
Convert String into JSON Array with key-Value Pairs
package com.softwaretestingo.interviewprograms; import java.util.HashMap; import java.util.Map; import org.json.simple.JSONObject; public class InterviewPrograms22 { /* * Input:{01IND02AUS03ENG} * Output: * { * "01": IND, * "02":AUS, * "03":ENG * } * O/P should be printed in json array with key value pair. * * Download Jar File From Here: https://code.google.com/archive/p/json-simple/downloads * After that Add the Jar File In the Java Project build Path: (right-click on the project, Properties->Libraries and add new JAR.) */ public static void main(String[] args) { String s = "01IND02AUS03ENG"; InterviewPrograms22 solution = new InterviewPrograms22(); solution.getSolution(s); } public void getSolution(String test) { String word[] = test.split("([0-9])+"); String num[] = test.split("([a-zA-z])+"); Map<String, String> hasmMap = new HashMap<String, String>(); for(int i=0; i<num.length; i++) { for(int j=i+1; j<=i+1; j++) { hasmMap.put(num[i], word[j]); } } JSONObject data = new JSONObject(hasmMap); System.out.println(data); } }
Output
{"01":"IND","02":"AUS","03":"ENG"}
Alternative 1:
This Java program achieves the goal of converting a given input string {01IND02AUS03ENG} into a HashMap with key-value pairs. The program takes the input string, processes it, and produces the output HashMap representation.
package com.softwaretestingo.interviewprograms; import java.util.HashMap; public class InterviewPrograms22_1 { /* * Input:{01IND02AUS03ENG} * Output: * { * "01": IND, * "02":AUS, * "03":ENG * } * O/P should be printed in json array with key value pair. * */ public static void main(String[] args) { String input = "01IND02AUS03ENG"; HashMap <String ,String> map= new HashMap <String,String>( ); String [ ] intArr = input.split("([0-9])+"); String [ ] StrArr = input.split("([a-z]|[A-Z])+"); int iCount = 1 ; for (String b:StrArr) { map.put(b,intArr[iCount]); iCount++; } System.out.println(map); } }
Output
{01=IND, 02=AUS, 03=ENG}
Input int a[]={5,0,4,6,0,7,0}
Output = {0,0,0,5,4,6,7}
Move Zeros Left By Maintaining Order Of Digits: This Java program achieves the goal of moving all zeros in the input array to the beginning while maintaining the order of other numbers. The program takes the input array int a[]={5,0,4,6,0,7,0}, and the output array should be {0,0,0,5,4,6,7}.
Move Zeros Left By Maintaining the Order Of Digits
package com.softwaretestingo.interviewprograms; public class InterviewPrograms21 { /* * Input int a[]={5,0,4,6,0,7,0} * Output = {0,0,0,5,4,6,7} * Goal is to print all zero first and then the rest of other numbers as it is. */ public static void main(String[] args) { int[] array = {5,0,4,6,0,7,0}; int current = array.length - 1; for (int i = array.length - 1; i >= 0; i--) { if (array[i] != 0) { array[current] = array[i]; current--; } } while (current >= 0) { array[current] = 0; current--; } for (int i = 0; i < array.length; i++) { System.out.print(array[i] + " "); } } }
Output
0 0 0 5 4 6 7
Alternative 1:
This Java program achieves the goal of moving all zeros in the input array to the beginning while maintaining the order of other numbers. The program takes the input array int a[]={5,0,4,6,0,7,0}, and the output should be {0,0,0,5,4,6,7}.
package com.softwaretestingo.interviewprograms; import java.util.LinkedList; public class InterviewPrograms21_1 { /* * Input int a[]={5,0,4,6,0,7,0} * Output = {0,0,0,5,4,6,7} * Goal is to print all zero first and then the rest of other numbers as it is. */ public static void main(String[] args) { int z[]= {5,0,4,6,0,7,0}; LinkedList<Integer> zeroFirst = new LinkedList<>(); for(int i:z) { if(i==0) zeroFirst.addFirst(i); else zeroFirst.add(i); } System.out.println(zeroFirst); } }
Output
[0, 0, 0, 5, 4, 6, 7]
Alternative 2:
This Java program achieves the goal of moving all zeros in the input array to the beginning while maintaining the order of other numbers. The program takes the input array int a[]={5,0,4,6,0,7,0}, and the output should be {0,0,0,5,4,6,7}.
package com.softwaretestingo.interviewprograms; public class InterviewPrograms21_2 { /* * Input int a[]={5,0,4,6,0,7,0} * Output = {0,0,0,5,4,6,7} * Goal is to print all zero first and then the rest of other numbers as it is. */ public static void main(String[] args) { int [] a = {5, 0, 4, 6, 0, 7, 0}; String s = ""; for (int i : a) { if (i == 0) s = i + "," + s; else s = s + i + ","; } for (String i : s.split(",")) System.out.print(i+" "); } }
Output
0 0 0 5 4 6 7
Alternative 3:
This Java program achieves the goal of moving all zeros in the input array to the beginning while maintaining the order of other numbers. The program takes the input array int a[]={5,0,4,6,0,7,0}, and the output should be {0,0,0,5,4,6,7}.
package com.softwaretestingo.interviewprograms; public class InterviewPrograms21_3 { /* * Input int a[]={5,0,4,6,0,7,0} * Output = {0,0,0,5,4,6,7} * Goal is to print all zero first and then the rest of other numbers as it is. */ public static void main(String[] args) { int [] givenArray = { 5 , 0 , 4 , 6 , 0 , 7 , 0 }; //reqArray [] = { 0,0,0,5,4,6,7 }; int reqArray [] = new int[givenArray.length]; int reqArrayIndex = 0 ; // to add zeroes in the beginning for (int j=0; j<givenArray.length ; j++ ) { if (givenArray[j]==0) { reqArray[reqArrayIndex]=0; reqArrayIndex++ ; } } // to add digits without changing order for ( int l=0 ; l<givenArray.length; l++ ) { if (givenArray[l]!=0) { reqArray[reqArrayIndex]=givenArray[l]; reqArrayIndex++; } } for ( int arr=0; arr<reqArray.length; arr++ ) { System.out.print(reqArray[arr]+" "); } } }
Output
0 0 0 5 4 6 7
Alternative 4:
This Java program achieves the goal of moving all zeros in the input array to the beginning while maintaining the order of other numbers. The program takes the input array int a[]={5,0,4,6,0,7,0}, and the output should be {0,0,0,5,4,6,7}.
package com.softwaretestingo.interviewprograms; public class InterviewPrograms21_4 { /* * Input int a[]={5,0,4,6,0,7,0} * Output = {0,0,0,5,4,6,7} * Goal is to print all zero first and then the rest of other numbers as it is. * WITH OUT BUILT IN FUNCTION */ public static void main(String[] args) { int zeroCount = 0 ; int [ ] arr = { 5 , 0 , 4 , 6 , 0 , 7 , 0 }; for (int i =0 ; i<arr.length ; i++ ) { if (arr[i] == 0) { zeroCount ++ ; } } System.out.println ("Zero count is : " +zeroCount); int arr1 [] = new int[arr.length]; for ( int i=0 ; i<zeroCount; i++) { arr1[i]=0; } int newIndex=0; for (int i=0 ; i<arr.length; i++ ) { if ( arr[i]>0 ) { arr1[zeroCount+newIndex]= arr[i]; newIndex ++ ; } } for ( int i=0 ; i<arr1.length; i++ ) { System.out.print(arr1[i]+","); } } }
Output
Zero count is : 3 0,0,0,5,4,6,7,
Input 1B3A2D4C
Output BAAADDCCCC
Print Character by its Corresponding Count: This Java program takes an input string “1B3A2D4C” and produces an output string “BAAADDCCCC”. The program interprets the input as a combination of letters and digits, where each letter is followed by its corresponding count of occurrences. It then reconstructs the output string based on this information.
Print the Character by its Corresponding Count
package com.softwaretestingo.interviewprograms; import java.util.ArrayList; import java.util.Iterator; import java.util.LinkedHashMap; import java.util.List; import java.util.Map; public class InterviewPrograms20 { /* * Input 1B3A2D4C * Output BAAADDCCCC * WALLMART */ public static void main(String[] args) { String s = "1B3A2D4C"; String[] sArray = s.split(""); List<String> intList = new ArrayList<>(); List<String> sList = new ArrayList<>(); for(String temp : sArray) { if(temp.matches("[a-zA-Z]")) { sList.add(temp); } else { intList.add(temp); } } Map<String, Integer> map = new LinkedHashMap<>(); Iterator<String> itr1 = sList.iterator(); Iterator<String> itr2 = intList.iterator(); while(itr1.hasNext() && itr2.hasNext()) { map.put(itr1.next(), Integer.parseInt(itr2.next())); } for(Map.Entry<String, Integer> m : map.entrySet()) { for(int i=0;i<m.getValue();i++) { System.out.print(m.getKey()); } } } }
Output
Alternative:
This Java program aims to transform a given input string according to a specific pattern and output the modified string. Let’s break down the program step by step for beginners:
package com.softwaretestingo.interviewprograms; public class InterviewPrograms20_1 { // Interview Questions Asked In Unify technologies // Suppose input string is "c3d4a2" then output should be "cccddddaa" public static void main(String[] args) { String s="c3d4a2"; String ans=""; for (int i = 0; i<=s.length()-1; i++) { String r=String.valueOf(s.charAt(i)); int rep= Integer.valueOf(String.valueOf(s.charAt(i+1))); for (int j =1 ; j<=rep; j++) { ans=ans+r; } i=i+1; } System.out.println("The Inputted Parameter Is: "+s); System.out.print("Expected output Is: "+ans); } }
Output:
The Inputted Parameter Is: c3d4a2 Expected output Is: cccddddaa
Input string “AAAABBCCCDDDDEEEG”
Output string “A4B2C3D4E3G1”
Count Occurrence of Characters in a String: This Java program takes an input string “AAAABBCCCDDDDEEEG” and generates an output string “A4B2C3D4E3G1”. The program compresses the input string by representing each character and the number of occurrences of that character consecutively.
Count Occurrence of Characters in a String
package com.softwaretestingo.interviewprograms; import java.util.ArrayList; public class InterviewPrograms19 { /* * Input string "AAAABBCCCDDDDEEEG" * Output string "A4B2C3D4E3G1" */ public static void main(String[] args) { String s ="AAAABBCCCDDDDEEEG"; ArrayList<Character> list = new ArrayList<>(); for(int i=0;i<s.length();i++) { int count=1; if(!list.contains(s.charAt(i))) { list.add(s.charAt(i)); for(int j=i+1;j<s.length();j++) { if(s.charAt(i)==s.charAt(j)) { count++; } } System.out.print(s.charAt(i)+""+count); } } } }
Output
A4B2C3D4E3G1
Alternative 1:
This Java program achieves the same goal as the previous one, which is to compress an input string “AAAABBCCCDDDDEEEG” into an output string “A4B2C3D4E3G1”. However, it uses a different approach to achieve this compression.
package com.softwaretestingo.interviewprograms; import java.util.HashMap; import java.util.Map; public class InterviewPrograms19_1 { /* * Input string "AAAABBCCCDDDDEEEG" * Output string "A4B2C3D4E3G1" */ public static void main(String[] args) { String s = "AAAABBCCCDDDDEEEG"; String[] sArray = s.split(""); Map<String, Integer> map = new HashMap<>(); for(String temp : sArray) { if(map.containsKey(temp)) { map.put(temp, map.get(temp)+1); } else { map.put(temp, 1); } } System.out.println(map.toString().replaceAll("\\W","")); } }
Output
A4B2C3D4E3G1
Alternative 2:
This Java program also achieves the goal of compressing the input string “AAAABBCCCDDDDEEEG” into the output string “A4B2C3D4E3G1”. It uses a HashMap to count the occurrences of each character in the input string and then prints the compressed output.
package com.softwaretestingo.interviewprograms; import java.util.HashMap; import java.util.Map; import java.util.Map.Entry; import java.util.Set; public class InterviewPrograms19_2 { /* * Input string "AAAABBCCCDDDDEEEG" * Output string "A4B2C3D4E3G1" */ public static void main (String [] args) { String str = "AAAABBCCCDDDDEEEG"; occurences (str); } public static void occurences(String str) { int count = 0 ; Map <Character, Integer> hs=new HashMap <Character ,Integer>(); for ( int i = 0 ; i<str.length(); i++) { if ( hs.containsKey(str.charAt(i))) { count =hs.get(str.charAt(i)); count = count+1; hs.put(str.charAt(i),count); } else { hs.put(str.charAt(i), 1); } } Set<Map.Entry <Character, Integer>> s = hs.entrySet(); for (Entry<Character,Integer> s1 : s ) { System.out.print (s1.getKey()+ ""+s1.getValue()); } } }
Output
A4B2C3D4E3G1
Input string: “AcBCbDEdea”
Output string:”AaBbCcDdEe”
Sorting Characters Alphabetically Ignoring Case Sensitive: This Java program transforms an input string into a new format following specific rules. The goal of the program is to create a new string in which each character from the input string is repeated exactly twice, once in uppercase and once in lowercase, and they appear in sorted order based on their lowercase representation.
Sorting Characters Alphabetically Ignoring Case Sensitive
package com.softwaretestingo.interviewprograms; import java.util.Set; import java.util.TreeMap; public class InterviewPrograms17 { /* * Input string: "AcBCbDEdea" * Output string:"AaBbCcDdEe" */ public static void main(String[] args) { TreeMap<Character,Integer> map1 = new TreeMap<Character,Integer>(); String S1="AcBCbDEdea"; System.out.println("Given Initial String: "+S1); char[] array = S1.toLowerCase().toCharArray(); for(char c:array) { if(map1.containsKey(c)) { map1.put(c,map1.get(c)+1); } else { map1.put(c,1); } } Set<Character> set=map1.keySet(); System.out.print("The String Gets Transformed to the Below Format: "); for(Character character:set) { if(map1.get(character)==2) { System.out.print(Character.toUpperCase(character)+""+Character.toLowerCase(character)); } } } }
Output:
Given Initial String: AcBCbDEdea The String Gets Transformed to the Below Format: AaBbCcDdEe
Input=”Selenium”
Output:
eleniumS
leniumeS
eniumleS
niumeleS
iumneleS
umineleS
muineleS
Cyclically Shifting the Input String: This Java program generates a set of substrings by cyclically shifting the input string “Selenium” characters to the left. It showcases nested loops and string manipulation to achieve this result.
Cyclically Shifting the Input String
package com.softwaretestingo.interviewprograms; public class InterviewPrograms14 { /* * Input="Selenium" * Output: * eleniumS * leniumeS * eniumleS * niumeleS * iumneleS * umineleS * muineleS */ public static void main(String[] args) { String str= "Selenium"; // char [ ] chs str.toCharArray ( ) ; int len = str.length() , temp = 0 ; String out = ""; for (int k = 0 ; k<len-1; k++) { for ( int i = k + 1 ; i < len ; i ++ ) { out =out + str.charAt(i); } temp= k ; while ( k >= 0 ) { out= out + str.charAt ( k ) ; k -- ; } k=temp; System.out.println ( out ) ; out=""; } } }
Output:
eleniumS leniumeS eniumleS niumeleS iumneleS umineleS muineleS
Alternative 1:
This Java program generates a set of substrings by cyclically shifting the characters of the input string “Selenium” to the left. The program achieves this by manipulating a character array representing the input string and repeatedly shifting the characters to generate the desired substrings.
package com.softwaretestingo.interviewprograms; public class InterviewPrograms14_1 { /* * Input="Selenium" * Output: * eleniumS * leniumeS * eniumleS * niumeleS * iumneleS * umineleS * muineleS */ public static void main(String[] args) { String str = "Selenium"; char [ ] input = str.toCharArray(); int len = input.length ; printArr(input, len); } public static void printArr (char[] input, int len) { for (int i = 0 ; i<input.length ; i++ ) { char temp = input[0]; for ( int j = 1 ; j < len ; j ++ ) { input[j-1]=input[j]; } input[len-1]=temp; len--; System.out.println(input); } } }
Output:
eleniumS leniumeS eniumleS niumeleS iumneleS umineleS muineleS muineleS
Input: tomorrow
Output: t#m##rr###w
Replace a Specific Character with # Increasing Number: This Java program takes an input string “tomorrow” and converts it into a modified version where each occurrence of the letter ‘o’ is replaced with an increasing number of ‘#’ symbols. The number of ‘#’ symbols for each ‘o’ is determined by its position in the original string.
Replace a Specific Character with # Increasing Number
package com.softwaretestingo.interviewprograms; public class InterviewPrograms12 { /* * Input: tomorrow * Output: t#m##rr###w */ public static void main(String[] args) { StringBuilder charactersToAppend = new StringBuilder("#"); String input = "tomorrow"; while (input.contains("o")) { input = input.replaceFirst("o", charactersToAppend.toString()); charactersToAppend.append("#"); } System.out.println(input); } }
Output:
t#m##rr###w
Alternative 1:
package com.softwaretestingo.interviewprograms; public class InterviewPrograms12_1 { /* * Input: tomorrow * Output: t#m##rr###w */ public void getSolution(String input) { int count = 0; String output = ""; char ch[] = input.toCharArray(); for(int i=0; i<ch.length; i++) { if(ch[i] == 'o') { count++; for(int j=0; j<count; j++) { output = output+Character.toString(ch[i]); output = output.replace(ch[i], '#'); } } else { output = output+Character.toString(ch[i]); } } System.out.println(output); } public static void main(String[] args) { String s = "tomorrow"; InterviewPrograms12_1 soluSoultionTest = new InterviewPrograms12_1(); soluSoultionTest.getSolution(s); } }
Output:
t#m##rr###w
Alternative 2:
This Java program aims to take an input string and modify it to replace each occurrence of the letter ‘o’ with an increasing number of ‘#’ symbols. It demonstrates an alternative approach to achieving the same result as the previous program using a different logic.
package com.softwaretestingo.interviewprograms; public class InterviewPrograms12_2 { /* * Input: tomorrow * Output: t#m##rr###w */ public static String replaceWithString(String input,char present,String replace ) { int count =0; String s =""; while(input.contains(Character.toString(present))) { int n = input.indexOf(present)>0?(count++):(count=0); if(count == n) break; for(int i= count; i>1; i--) { s+=replace; } input= input.replaceFirst(Character.toString(present),s); } return input; } public static void main(String[] args) { String result=replaceWithString("tomorrow",'o',"#"); System.out.println(result); } }
Output:
t#m##rr###w
Input:{A, B, C, D}
Output:{AA, BB, CC, DD}
Print Each Character 2 Times: This Java program aims to generate an output array based on a given input array. The input array is defined as {‘A’, ‘B’, ‘C’, ‘D’}. The desired output is an array where each element is a concatenation of the corresponding elements from the input array.
Print Each Character 2 Times Of Character Array
For example, given the input array {A, B, C, D}, the expected output is {AA, BB, CC, DD}.
package com.softwaretestingo.interviewprograms; public class InterviewPrograms10 { /* * Input:{A, B, C, D} * Output:{AA, BB, CC, DD} */ public static void main(String[] args) { char[] ch = {'A', 'B', 'C', 'D'}; append(ch); } static void append(char ch[]) { char[] ch1=ch.clone(); System.out.println(ch); System.out.println(ch1); char[] result= new char[0]; StringBuilder sb = new StringBuilder(); for(int i=0;i<ch.length;i++) { sb.append(ch[i]); sb.append(ch1[i]); sb.append(' '); result = sb.toString().toCharArray(); } System.out.println(result); } }
Output:
AA BB CC DD
Alternative Way:
package com.softwaretestingo.interviewprograms; import java.util.ArrayList; public class InterviewPrograms10_1 { /* * Input:{A, B, C, D} * Output:{AA, BB, CC, DD} */ public static void main(String[] args) { String[]arr= {"A","B","C","D"}; ArrayList<String> AL=new ArrayList<>(); for(int i=0;i<arr.length;i++) { String str=arr[i]+arr[i]; AL.add(str); } //System.out.println(arr); System.out.println(AL); } }
Output:
[AA, BB, CC, DD]
Input: 1234
Output:{1234, 11223344, 111222333444, 1111222233334444}
Increasing Number of Repetitions of Input Number’s Characters: The goal of this program is to generate a specific pattern based on a given input number and store the generated patterns in a list.
The input for this program is the number “1234”. The desired output is a series of patterns where each pattern contains an increasing number of repetitions of the input number’s characters.
For example, when the input number is “1234”, the program generates the following patterns: “1234”, “11223344”, “111222333444”, and “1111222233334444”.
Increasing Number of Repetitions of Input Number’s Characters
To achieve this, the program uses nested loops. The outer loop iterates over the characters of the input number. The middle loop repeats the current character a specific number of times based on the outer loop index. The innermost loop concatenates the repeated character to the finals variable. After each iteration, the generated pattern is added to the list, and the finals variable is reset for the next iteration.
Finally, the program prints the generated patterns stored in the list using the System.out.println() statement.
package com.softwaretestingo.interviewprograms; import java.util.ArrayList; import java.util.List; public class InterviewPrograms9 { /* * Input: 1234 * Output:{1234, 11223344, 111222333444, 1111222233334444} */ public static void main(String[] args) { String num = "1234"; String finals = ""; char arr[] = num.toCharArray(); List<String> list = new ArrayList<String>(); for (int i = 0; i < arr.length; i++) { for (int j = 0; j < arr.length; j++) { for (int k = 0; k < i + 1; k++) { finals = finals + arr[j]; } } list.add(finals); finals = ""; } System.out.println(list); } }
Output:
[1234, 11223344, 111222333444, 1111222233334444]
Input: s = “egg”, t = “add”
Output: true
Input: s = “foo”, t = “bar”
Output: false
Input: s = “paper”, t = “title”
Output: true
Check Character Sequence Is in Same Order Or Not: The program InterviewPrograms54 aims to determine if two strings, s and t, are isomorphic. Two strings are considered isomorphic if the characters in one string can be replaced with characters in the other string while preserving the order of characters.
Each character in the first string should map to a unique character in the second string, and no two characters can map to the same character. However, a character may map to itself.
Check Character Sequence Is in Same Order Or Not
package com.softwaretestingo.interviewprograms; import java.util.ArrayList; import java.util.LinkedHashMap; import java.util.List; import java.util.Map; public class InterviewPrograms54 { /* * Given two strings s and t, determine if they are isomorphic. Two strings s * and t are isomorphic if the characters in s can be replaced to get t. * * All occurrences of a character must be replaced with another character while * preserving the order of characters. No two characters may map to the same * character, but a character may map to itself. * * Example 1: * * Input: s = "egg", t = "add" * Output: true Example 2: * * Input: s = "foo", t = "bar" * Output: false Example 3: * * Input: s = "paper", t = "title" * Output: true */ public static void main(String[] args) { System.out.println(areIsomorphic("egg","add")); } static boolean areIsomorphic(String str1, String str2) { List<Integer> str1ChrSequence=getCharSequence(str1); List<Integer> str2ChrSequence=getCharSequence(str2); return str1ChrSequence.equals(str2ChrSequence); } static List<Integer> getCharSequence(String str) { Map<Character,Integer> resultMap=new LinkedHashMap<>(); int count=1; for(char ch:str.toCharArray()) { if(resultMap.containsKey(ch)) { resultMap.put(ch,resultMap.get(ch)+1); } else { resultMap.put(ch,count); } } List<Integer> resultList=new ArrayList<>(); resultList.addAll(resultMap.values()); return resultList; } }
Output
true
Enter the String: softwaretestingo
Enter The K Value: 2
After Removing The Characters Having More then 2 Times:- fwaring
Remove Characters That Appear More Than K Times: This Java program takes a string as input and an integer value ‘k’, and then it removes all characters from the string that appear more than ‘k’ times. The program uses a HashMap to count the frequency of each character in the input string and then constructs a new string without the characters that exceed the given frequency threshold ‘k’.
Remove Characters That Appear More Than K Times
package com.softwaretestingo.interviewprograms; import java.io.BufferedReader; import java.io.IOException; import java.io.InputStreamReader; import java.util.HashMap; import java.util.Map; public class InterviewPrograms85 { public static String removeChars(String str, int k) { // Using HashMap to count frequencies Map<Character, Integer> freq = new HashMap<>(); for (int i = 0; i < str.length(); i++) { char c = str.charAt(i); freq.put(c, freq.getOrDefault(c, 0) + 1); } // create a new empty string StringBuilder res = new StringBuilder(); for (int i = 0; i < str.length(); i++) { char c = str.charAt(i); // Append the characters which // appears less than equal to k times if (freq.get(c) < k) { res.append(c); } } return res.toString(); } public static void main(String[] args) throws IOException { BufferedReader br = new BufferedReader(new InputStreamReader(System.in)); System.out.println("Enter the String: "); String str=br.readLine(); System.out.println("Enter The K Value: "); int k = Integer.parseInt(br.readLine()); System.out.println("After Removing The Characters Having More then "+k+ " Times:- "+ removeChars(str, k)); } }
Output
Enter the String: softwaretestingo Enter The K Value: 2 After Removing The Characters Having More then 2 Times:- fwaring
Alternative Way 1:
This Java program takes a string as input and an integer value ‘k’, and then it removes all characters from the string that appear more than ‘k’ times. The program uses an array-based approach with a hash table to count the frequency of each character in the input string. It then modifies the input character array in-place to remove characters exceeding the given frequency threshold ‘k’.
package com.softwaretestingo.interviewprograms; import java.io.BufferedReader; import java.io.IOException; import java.io.InputStreamReader; public class InterviewPrograms85_1 { static final int MAX_CHAR = 26; public static void removeChars(char[] charArray, int k) { // Hash table initialised to 0 int hash[]=new int[MAX_CHAR]; for (int i = 0; i <MAX_CHAR; ++i) hash[i]=0; // Increment the frequency of the character int n = (charArray).length; for (int i = 0; i < n; ++i) hash[charArray[i] - 'a']++; // Next index in reduced string int index = 0; for (int i = 0; i < n; ++i) { // Append the characters which // appears less than k times if (hash[charArray[i] - 'a'] < k) { charArray[index++] = charArray[i]; } } for (int i = index; i < n; ++i) charArray[i] = ' '; } public static void main(String[] args) throws IOException { BufferedReader br = new BufferedReader(new InputStreamReader(System.in)); System.out.println("Enter the String: "); String str=br.readLine(); System.out.println("Enter The K Value: "); int k = Integer.parseInt(br.readLine()); char[] charArray=str.toCharArray(); removeChars(charArray,k); System.out.println("After Removing The Characters Having More then "+k+ " Times:- "+ String.valueOf(charArray)); } }
Output
Enter the String: softwaretestingo Enter The K Value: 2 After Removing The Characters Having More then 2 Times:- fwaring
Input: “abcdcbe”
Longest palindrome substring is: bcdcb
Length is: 5
Longest Palindrome Substring: This Java program finds the longest palindrome substring (LPS) from a given input string and prints it along with its length. A palindrome is a string that reads the same forwards and backward. The program employs a nested loop to check all possible substrings and uses a simple technique to verify whether each substring is a palindrome.
Longest Palindrome Substring
package com.softwaretestingo.interviewprograms; public class InterviewPrograms87 { static int findLongSubstr(String str) { // Get length of input String int n = str.length(); // All subStrings of length 1 are palindromes int maxLength = 1, start = 0; // Nested loop to mark start and end index for (int i = 0; i < str.length(); i++) { for (int j = i; j < str.length(); j++) { int flag = 1; // Check palindrome for (int k = 0; k < (j - i + 1) / 2; k++) if (str.charAt(i + k)!= str.charAt(j-k)) flag = 0; // Palindrome if (flag != 0 && (j - i + 1) > maxLength) { start = i; maxLength = j - i + 1; } } } System.out.print("Longest palindrome substring is: "); printSubStr(str, start, start + maxLength - 1); // Return length of LPS return maxLength; } // Function to print a subString str[low..high] static void printSubStr(String str, int low, int high) { for (int i = low; i <= high; ++i) System.out.print(str.charAt(i)); } public static void main(String[] args) { String str = "abcdcbe"; System.out.print("\nLength is: "+ findLongSubstr(str)); } }
Output
Longest palindrome substring is: bcdcb Length is: 5
Alternative Way 1:
This Java program finds the longest palindrome substring (LPS) from a given input string using a different approach compared to the previous programs. It uses two nested loops to explore all possible substrings and checks whether each substring is a palindrome by comparing characters from both ends of the substring.
package com.softwaretestingo.interviewprograms; public class InterviewPrograms87_1 { static int findLongSubstr(String str) { // Stores Longest Palindrome Substring String longest = ""; int n = str.length(); int j; // To store substring which we think can be a palindrome String subs = ""; // To store reverse of substring we think can be palindrome String subsrev = ""; for (int i = 0; i < n; i++) { j = n - 1; while (i < j) { // Checking whether the character at i and j // are same. // If they are same then that substring can be LPS if (str.charAt(i) == str.charAt(j) && longest.length() < (j - i + 1)) { subs = str.substring(i, j + 1); subsrev = new StringBuilder(subs).reverse().toString(); if (subs.equals(subsrev)) { longest = subs; } } j--; } } // If no longest substring then we will return first // character if (longest.length() == 0) { longest = str.substring(0, 1); } System.out.println( "Longest palindrome substring is: " + longest); // Return length of LPS return longest.length(); } public static void main(String[] args) { String str = "abcdcbe"; System.out.print("\nLength is: "+ findLongSubstr(str)); } }
Output
Longest palindrome substring is: bcdcb Length is: 5
Print Pascal’s triangle: We can able to print Pascal’s triangle in various ways. Below we have mentioned various ways:
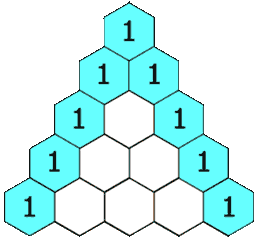
Print a Pascal’s Triangle Without Using an Array
This Java program prints Pascal’s Triangle based on the number of rows input by the user. Pascal’s Triangle is a triangular array of binomial coefficients, named after the French mathematician Blaise Pascal. The program uses nested loops and basic mathematical calculations to generate and print the triangle.
package com.softwaretestingo.interviewprograms; import java.util.Scanner; public class InterviewPrograms88 { public static void main(String[] args) { int row, i, j, space, num; Scanner in = new Scanner(System.in); System.out.print("Enter value for rows: "); row = in.nextInt(); for(i=0; i<row; i++) { for(space=row; space>i; space--) { System.out.print(" "); } num=1; for(j=0; j<=i; j++) { System.out.print(num+ " "); num = num*(i-j)/(j+1); } System.out.print("\n"); } } }
Output
Enter value for rows: 10 1 1 1 1 2 1 1 3 3 1 1 4 6 4 1 1 5 10 10 5 1 1 6 15 20 15 6 1 1 7 21 35 35 21 7 1 1 8 28 56 70 56 28 8 1 1 9 36 84 126 126 84 36 9 1
Print a Pascal’s Triangle With Using Array
This Java program prints Pascal’s Triangle using the concept of the binomial coefficient. Pascal’s Triangle is a triangular array of binomial coefficients, named after the French mathematician Blaise Pascal. The program calculates the binomial coefficients for each position in the triangle and then prints the triangle.
package com.softwaretestingo.interviewprograms; import java.util.Scanner; public class InterviewPrograms88_1 { public static void main(String[] args) { int row, i, j,k,l, space; Scanner in = new Scanner(System.in); System.out.print("Enter value for rows: "); row = in.nextInt(); int ncr[][] = new int[row][row]; //First element is always 1 ncr[0][0] = 1; //start from 2nd row i.e from i=1 for(i=1; i<row; i++) { //First element of each row is always 1 ncr[i][0] = 1; for(j=1; j<=i; j++) { ncr[i][j] = ncr[i-1][j-1] + ncr[i-1][j]; } } //Output the array for(i=0; i<row; i++) { //Output the blank space for(k=0; k<row-i; k++) { System.out.print(" "); } for(j=0; j<=i; j++) { System.out.print(ncr[i][j]+" "); } System.out.println(); } } }
Output
Enter value for rows: 5 1 1 1 1 2 1 1 3 3 1 1 4 6 4 1
Enter the string: SoftwareTestingo
Enter the Character you want to search: e
e is present at index 7
Search a Letter In a String
package com.softwaretestingo.interviewprograms; import java.io.BufferedReader; import java.io.IOException; import java.io.InputStreamReader; public class InterviewPrograms94 { //Write a program for removing white spaces in a String. public static void main(String[] args) throws IOException { String str; char c; // create an object of Scanner BufferedReader br=new BufferedReader(new InputStreamReader(System.in)); System.out.println("Enter the string"); str=br.readLine(); System.out.println("Enter the Character you want to search"); c=(char)br.read(); int index = str.indexOf(c); System.out.printf(c +" is present at index %d", index); } }
Output
Enter the string SoftwareTestingo Enter the Character you want to search e e is present at index 7
Original String: Hello Interview Is Going On Now
After Converting: hello interview is going on now
Convert Unique Uppercase to Lowercase Letter: The “Uppercase to Lowercase Conversion” Java program addresses the task of converting uppercase letters within a given string into their corresponding lowercase forms. By processing the provided input string, the program produces an output string where all uppercase letters have been transformed to lowercase while preserving the positions and characters of the remaining text.
For instance, when presented with the input string “Hello Interview Is Going On Now,” the java programming language executes the conversion and yields the output string “hello interview is going on now.” This program is particularly useful in scenarios where uniform letter casing is required for consistency and ease of further processing.
Through this program, learners become acquainted with the essentials of string manipulation, character iteration, and conditional transformations in Java programming. This foundational exercise introduces beginners to fundamental concepts used in text processing and prepares them for more complex tasks involving string handling and data transformation.
Convert Unique Uppercase to Lowercase Letter Program
This Java program, InterviewPrograms_100, aims to convert uppercase letters within a given string to their corresponding lowercase forms. It does so by iteratively processing each character in the input string and transforming uppercase characters to lowercase while leaving the rest of the string unchanged.
package com.softwaretestingo.interviewprograms; public class InterviewPrograms_100 { /* * Given a string and return the string after replacing every uppercase letter * with the same lowercase letter. Input : s = "Hello Interview Is Going On Now" * Output : "hello interview is going on now" */ //This Questions Asked in Cigniti technologies public static void main(String[] args) { String s= "Hello Interview Is Going On Now"; char[]c = s.toCharArray(); for(int i=0;i<c.length;++i ) { if(c[i]>=65 && c[i]<=90) { c[i] = (char) (c[i] + 32); } } System.out.println("After Converting: "+String.valueOf(c)); } }
Output:
Original String: Hello Interview Is Going On Now After Converting: hello interview is going on now
Input: 01230
Output:03210
WAP to Convert 01230 To 03210: This Java program is designed to reverse a given integer represented as a string, add leading zeros if necessary, and print the reversed number. The program demonstrates how to format an integer with leading zeros using String.format, convert an integer to a string, iterate through a string in reverse order, and concatenate characters to form the reversed number.
Convert 01230 To 03210
package com.softwaretestingo.interviewprograms; public class InterviewPrograms15 { /* * Input: 01230 * Output: 03210 */ public static void main(String[] args) { int n = 1230 ; String s = String.format ( "%05d",n); // to get 1230 to 01230 System.out.println ( s ) ; int len = s.length ( ) ; String rev = "" ; for ( int i = len - 1 ; i >= 0 ; i-- ) { rev = rev + s.charAt ( i ) ; } System.out.println ( rev ) ; } }
Output:
01230 03210
Alternative 1:
This Java program takes an input string “01230” and reverses its characters to produce the output “03210”. The Java program function demonstrates how to convert a string into a character array, iterate through the character array in reverse order, and then concatenate the characters to generate the reversed string.
package com.softwaretestingo.interviewprograms; public class InterviewPrograms15_1 { /* * Input: 01230 * Output: 03210 */ public static void main(String[] args) { String s= "01230"; System.out.println("Input: "+s); String newStr = " " ; char c [ ] =s.toCharArray ( ) ; for ( int i = c.length - 1 ; i >= 0 ; i-- ) { newStr= newStr + c [ i ] ; } System.out.println ("Output"+newStr); } }
Output:
01230 03210
Input: tomorrow
Output: ooorrtmw
Sort A String According To The Frequency Of Characters: The program InterviewPrograms51 aims to rearrange the characters of a given input string in descending order based on their frequency of occurrence.
Sort A String According To The Frequency Of Characters
To solve this program, we have taken the help of various Java topics like:
package com.softwaretestingo.interviewprograms; import java.util.ArrayList; import java.util.Comparator; import java.util.LinkedHashMap; import java.util.List; import java.util.Map; public class InterviewPrograms51 { /* * Input: tomorrow * Output: ooorrtmw */ public static void main(String[] args) { String input = "tomorrow"; Map<Character, Integer> map = StringManipulation(input); // sort and print List<Map.Entry<Character, Integer>> toSort = new ArrayList<>(map.entrySet()); toSort.sort(Map.Entry.comparingByValue(Comparator.reverseOrder())); for ( Map.Entry<Character, Integer> x : toSort ) { int count = x.getValue(); while ( count > 0 ) { System.out.print ( x.getKey ( ) ) ; count-- ; } } } private static Map<Character, Integer> StringManipulation(String input) { Map<Character, Integer> map=new LinkedHashMap<>(); for(char ch : input.toCharArray()) if(map.containsKey(ch)) map.put(ch, map.get(ch)+1); else map.put(ch, 1); return map; } }
Output
ooorrtmw
Input: 5450595638154862
After Masking Output: 545059******4862
Modified Output: 5450-59**-****-4862
Method 1:
package com.softwaretestingo.stringmanipulationpgms; public class STG01ReverseWordsInAGivenString { public static void main(String[] args) { String str="5450595638154862 "; System.out.println("Input: "+str); String FirstString=str.substring(0, 6); String lastSubString=str.substring((str.length()-5), str.length()); String middleStrting=str.substring(7,(str.length()-5)); String maskString= middleStrting.replaceAll("[0-9]", "*"); String finalString=FirstString+maskString+lastSubString; System.out.println("After Masking: "+finalString); char arr[]=finalString.toCharArray(); StringBuilder builder=new StringBuilder();; for(int i=0;i<str.length()-1;i++) { builder.append(arr[i]); if(i==3||i==7||i==11) { builder.append("-"); builder.append(arr[i]); } } System.out.println("After Adding -: "+builder.toString()); } }
Output:
Input: 5450595638154862 After Masking: 545059*****4862 After Adding -: 5450-059**-****4-4862