Else Statement Java: The else statement in Java complements the “if statement” by providing an alternative course of action when a specified condition is unmet. While the “if statement” allows us to execute a code block when a condition is considered true, the “else statement” defines what should happen when the condition is false.
This branching capability is fundamental for creating programs to make informed decisions and execute instructions based on varying circumstances. In Java, the “else statement” enhances the language’s versatility and adaptability, enabling developers to build more sophisticated and responsive applications.
If Else Statement Syntax
Certainly! The syntax of an “if-else statement” in Java is as follows:
if (condition) { // Code to execute if the condition is true } else { // Code to execute if the condition is false }
Here’s a breakdown of the components:
- if: This is the keyword that initiates the “if-else statement.”
- condition: Inside the parentheses, you provide an expression resulting in a boolean value (true or false ). This condition is evaluated.
- {}: After the “if” condition, you use curly braces to enclose a code block. This code block is executed if the condition is true.
- else: After the “if” block, you can include an “else” keyword to specify an alternative block of code executed if the condition is false.
- {}: Following the “else” keyword, you use another set of curly braces to enclose the code that should be executed when the condition is false
Here’s an example:
int age = 15; if (age >= 18) { System.out.println("You are an adult."); } else { System.out.println("You are not yet an adult."); }
In this example, if the age is under 18, the code inside the “else” block is executed, resulting in the message “You are not yet an adult” printed on the console. If the age is 18 or greater, the code inside the “if” block is executed, and the message “You are an adult.” is printed.
How does the else statement in Java work?
This works something similar to the if Statement. We have prepared an if-else statement flowchart to understand better how the else statement works.
Check the below if else statement flowchart:
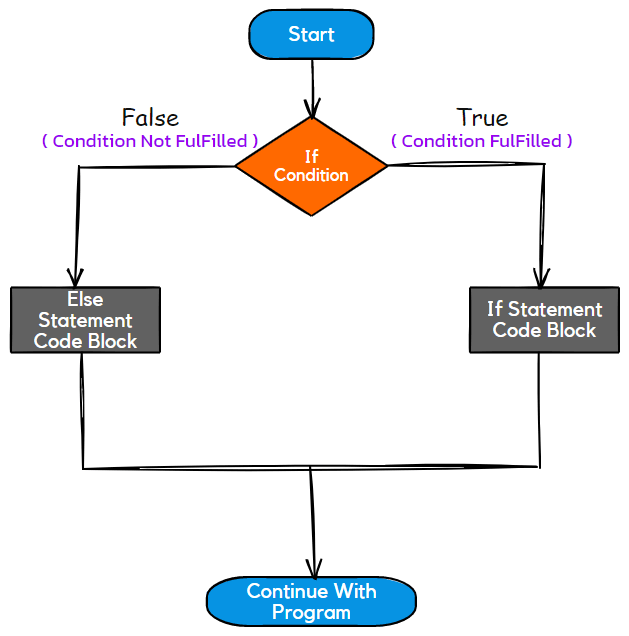
The if-else statement flowchart is a graphical representation used in programming to illustrate decision-making processes. It begins with a “Start” symbol and ends with a “Continue with program” symbol. In between, a diamond-shaped “Decision” symbol signifies a point where a condition is evaluated.
Two arrows extend from the decision symbol, typically labeled “True” and “False.” These arrows lead to rectangles representing specific actions or processes to be executed based on whether the condition is true or false. The “Yes” branch contains actions associated with the “if” block, while the “No” branch holds actions associated with the “else” block.
Flowcharts like these provide a visual and systematic way to understand, document, and troubleshoot complex decision logic in code, promoting clarity and error detection. They are invaluable tools for programmers and those analyzing the program’s functionality.
Common errors and mistakes when using the else statement in Java
Common mistakes in Java programming include:
- Forgetting to use an “else statement” when necessary can lead to a lack of code execution in certain program scenarios.
- Neglecting to specify what should happen in the “else” case when dealing with user input or decision points, potentially causing program flow issues.
- Incorrectly using a condition in the “else statement” when “else” should not have a condition. Using “else-if” for additional conditions is more appropriate in such cases.
- Accidentally adding a semi-colon after the “if statement” is not a common mistake but can occur. Most compilers will provide a warning for this error.
Conclusion:
the “else” statement in Java is essential for creating robust and flexible code. It enables precise decision-making and code branching with “if” statements.
We encourage you to comment if you have any doubts regarding the “else” statement in Java or would like to share suggestions for improving this article. Your feedback contributes to our understanding of this critical aspect of Java programming.