The “if” statement is one of the most fundamental control flow statements in the Java programming language. An “if statement” evaluates a condition, typically a boolean expression, to determine whether a specific block of code should be executed.
If the condition is true, the code within the “if” block is executed, allowing for one set of actions. If the condition is false, the code within the “if” block is bypassed, and optionally, an “else” block of code can be executed to handle an alternative set of actions.
The If statement empowers the developers to make decisions within their code, allowing different execution branches based on specified conditions. In this detailed blog post on the If statement guide, we will explore the “if” statement in Java, its syntax, and usage, and provide numerous examples to illustrate its functionality.
If Statement in Java
In the world of programming, decisions are important. You must often instruct your program to perform different actions based on certain conditions. This is where the If Statement And Java comes into play. The “if” statement is a fundamental control flow structure that allows you to create conditional branches in your code.
If Statement Syntax
The basic syntax of an “if” statement in Java is as follows:
if (condition) { // Code to execute if the condition is true }
Here’s a breakdown of the components:
- if: This is the keyword that initiates the “if statement.”
- Condition: Inside the parentheses, you provide an expression resulting in a boolean value (true or false). This condition is evaluated.
- {}: After the condition, you use curly braces to enclose a code block. This code block is executed if the condition is true.
If Statement Flowcharts
Statement flowcharts are a visual representation of the logical flow of a Java program or process. Here’s a simplified flowchart for an “if statement” in Java:
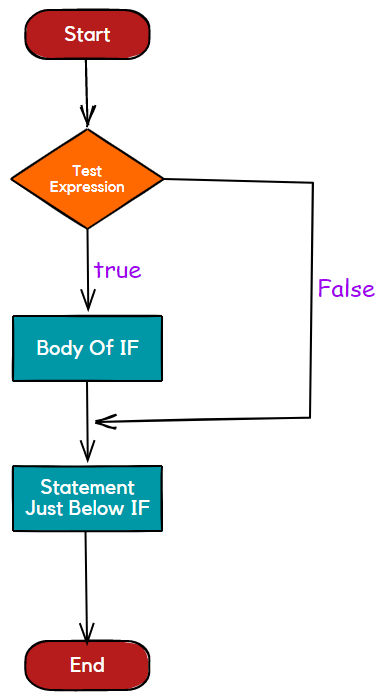
In this If Statement Flowcharts:
- The process starts at the “Start” symbol.
- It then evaluates the condition, which is represented as a diamond-shaped decision point. The condition can be any expression that results in a boolean value (true or false).
- If the condition is true, the flow executes the code block associated with the “if” statement.
- If the condition is false and an “else” block is present, the flow executes the code in the “else” block.
- Finally, the process ends with the “End” symbol.
If Statement Example In Java
Let’s consider a simple example where we want to check if a person is eligible to vote based on their age:
int age = 20; if (age >= 18) { System.out.println("You are eligible to vote."); }
In this example:
- We define an integer variable age with a value of 20.
- The “if” statement checks if the age is greater than or equal to 18.
- Since the condition is true (age is greater than 18), the code block within the “if” statement is executed.
- As a result, the message “You are eligible to vote.” is printed on the console.
if statement and java Example Handling Multiple Conditions
You can also use logical operators like && (AND) and || (OR) to handle more complex conditions in “if” statements. For example, if you want to check both age and citizenship for voting eligibility:
int age = 16; boolean isCitizen = true; if (age >= 18 && isCitizen) { System.out.println("You are eligible to vote."); } else { System.out.println("You are not eligible to vote."); }
In this case, both the age condition and citizenship condition must be true for the person to be eligible to vote.
Nested If Statement In Java Example
if statements can also be nested within each other to create more intricate decision trees. This allows you to handle multiple conditions and outcomes in a structured manner.
int age = 20; boolean hasID = true; if (age >= 18) { if (hasID) { System.out.println("You are eligible to vote."); } else { System.out.println("You need to provide ID to vote."); } } else { System.out.println("You are not eligible to vote."); }
In this nested “if” statement, we first check the age, and if the age condition is met, we further check if the person has an ID to vote.
Conclusion
The “if” statement is a foundational building block of Java programming that allows you to introduce conditional logic into your code. It empowers you to create flexible programs to make decisions and adapt to different scenarios.
Whether building a simple voting eligibility checker or a complex decision-making algorithm, mastering the “if” statement is essential in your journey as a Java developer.
Suppose you have doubts regarding the “if” statement in Java or would like to share suggestions for improving this article. Your feedback is valuable in enhancing our understanding of this core aspect of Java programming.