Abstraction is one of the fundamental principles of object-oriented programming (OOP) within the Java programming language. It is essential for building structured and maintainable software. It allows developers to simplify complex systems by focusing on the essential features while hiding the complex implementation details.
What is Abstraction in Java?
Abstraction in Java is a concept that simplifies complex systems by focusing on what things do rather than how they do it. It’s like using a TV remote control without knowing all the inner electronics.
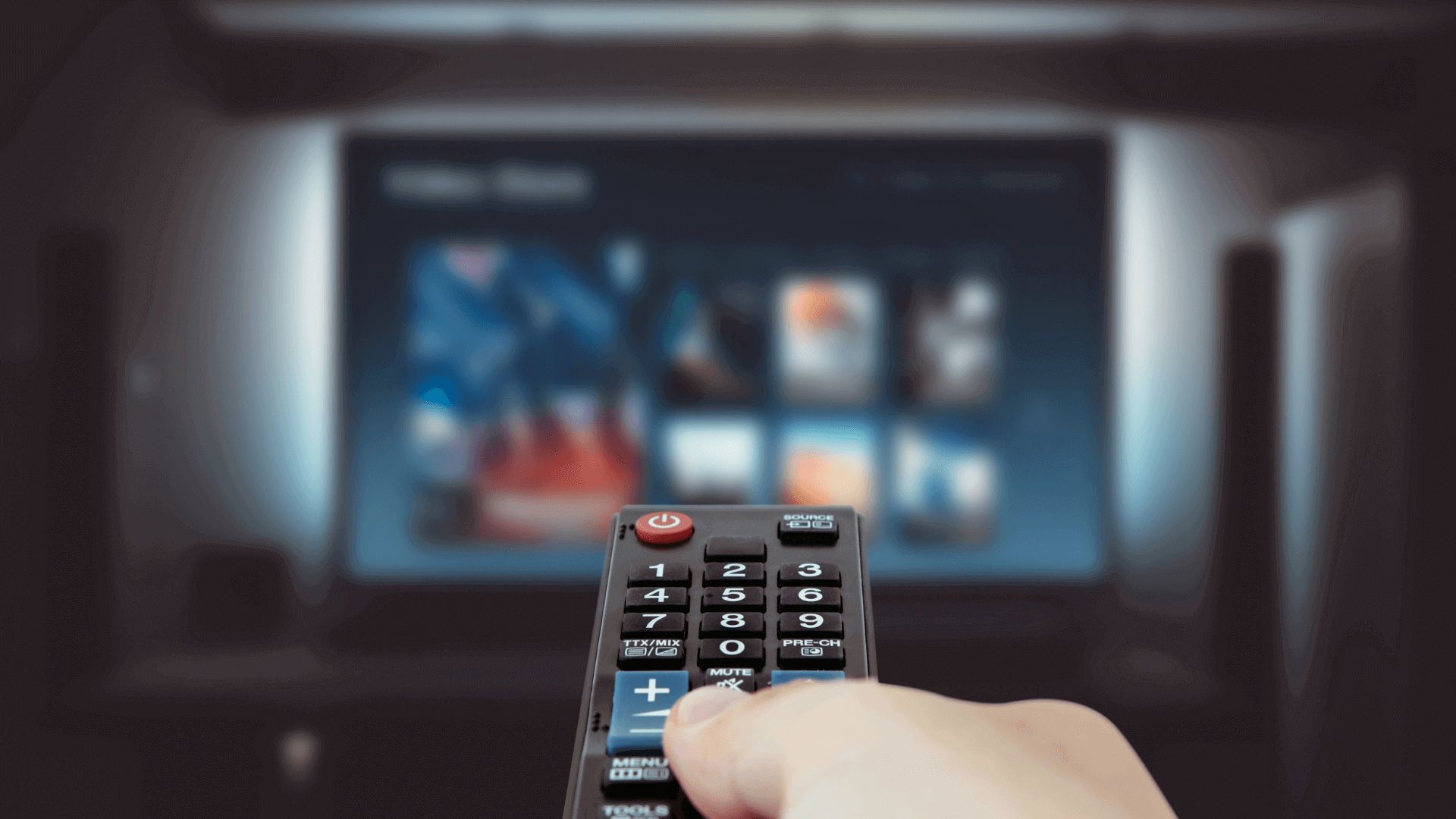
In Java, we create abstract classes and interfaces that define what methods an object should have, but we don’t specify how those methods work. This allows us to build organized and reusable code, making it easier to manage and understand. Abstraction is a key principle in Java and helps build flexible and maintainable software.
In Java, we can achieve abstraction through abstract classes, interfaces, and the practice of creating class hierarchies.
The primary goal of abstraction is to allow developers to work with higher-level, generalized concepts rather than getting bogged down by complicated implementation details.
By focusing on “what” an object does rather than “how” it does it, abstraction simplifies software design, promotes code reusability, and enhances the maintainability of Java applications.
Key Aspects of Abstraction
Here, we have noted down all the key points about Abstraction In Java:
Abstract Classes
An abstract class is a class that cannot be instantiated on its own. Instead, it serves as a blueprint for other classes. Abstract classes often include abstract methods declared without an implementation.
The subclasses of an abstract class are responsible for providing concrete implementations for the abstract methods. We can declare an abstract class and method like the one below:
abstract class Animal { abstract void sound(); }
We have discussed abstract class and abstract method in a separate post; check that for better knowledge of abstract class.
Interfaces
Interfaces in Java define a contract that concrete classes must adhere to. All methods declared in an interface are abstract and must be implemented by the interface’s classes.
This promotes a high level of abstraction and ensures that classes adhere to a specific set of behaviors. You can declare an interface by following the below syntax:
interface Drawable { void draw(); }
We have developed a detailed article discussing one of the important concepts of Java programming language. Follow these Interface in Java links for more details.
Hiding Complexity
Abstraction allows you to hide the internal complexity of a class or system. It exposes only the necessary functionality while keeping the implementation details hidden. This simplifies the usage of classes and makes the code more manageable.
Encapsulation
Abstraction is closely related to encapsulation, another fundamental Java concept. Encapsulation involves binding data members and methods that operate on that data into a single unit (class) and controlling access to the data.
Abstraction helps encapsulation by exposing only the essential methods and properties while keeping the rest private. We have already discussed in detail about Encapsulation, and you can check that by clicking on Encapsulation In Java Link.
Real-world Analogy
Think of abstraction like driving a car. You don’t need to know how the engine, transmission, and brakes work in intricate detail to drive the car effectively. You interact with the car through a simple interface: the steering wheel, pedals, and gear shift. This abstraction allows you to drive without worrying about the complex internal mechanisms.
Abstraction in Java is a powerful tool for managing complexity and building modular, extensible, and maintainable code. By creating abstract classes and interfaces, you define a clear structure for your software, promote code reuse, and facilitate collaboration among developers. It encourages a high-level view of your program, making it easier to understand and adapt as your project evolves.
Conclusion:
Abstraction in Java is a foundational concept that simplifies the development of complex software systems by focusing on what objects should do rather than how they do it. It’s like providing instructions without diving into every detail of the process.
If you have any doubts or questions about Abstraction in Java, please feel free to comment below. I’m here to provide further clarification and answer any queries.
Additionally, if you have any suggestions on improving this article or topics you’d like to see covered in more detail, your feedback is greatly appreciated. Your insights can help make this resource even more valuable to the Java programming community. So, don’t hesitate to share your thoughts in the comment section below. Thank you for your engagement!