Different Types of Exceptions Handling In Java: Java is a programming language that follows the object-oriented approach. One of its useful features is exception handling, which helps developers manage and control runtime issues related to exceptions.
In Java, an exception is an unexpected event that occurs while a program runs, causing the program to deviate from its normal flow. These exceptions are errors or bugs that we want to avoid as they disrupt the smooth functioning of our application.
In this article, we will discuss the following things:
What is Java Exception?
Exceptions are problems or unexpected events that can disrupt the normal running of a program or even make it crash. To deal with these issues, programming languages often have a useful feature called exception handling. It allows developers to handle and manage errors caused by exceptions during runtime, preventing the program from crashing.
Types of Exceptions in Java
Java provides a wide range of features, which can lead to developers making various types of mistakes and encountering different exceptions in Java. To make things easier to handle, we will categorize and classify Java’s different types of exceptions.
Exceptions can be divided into two main types:
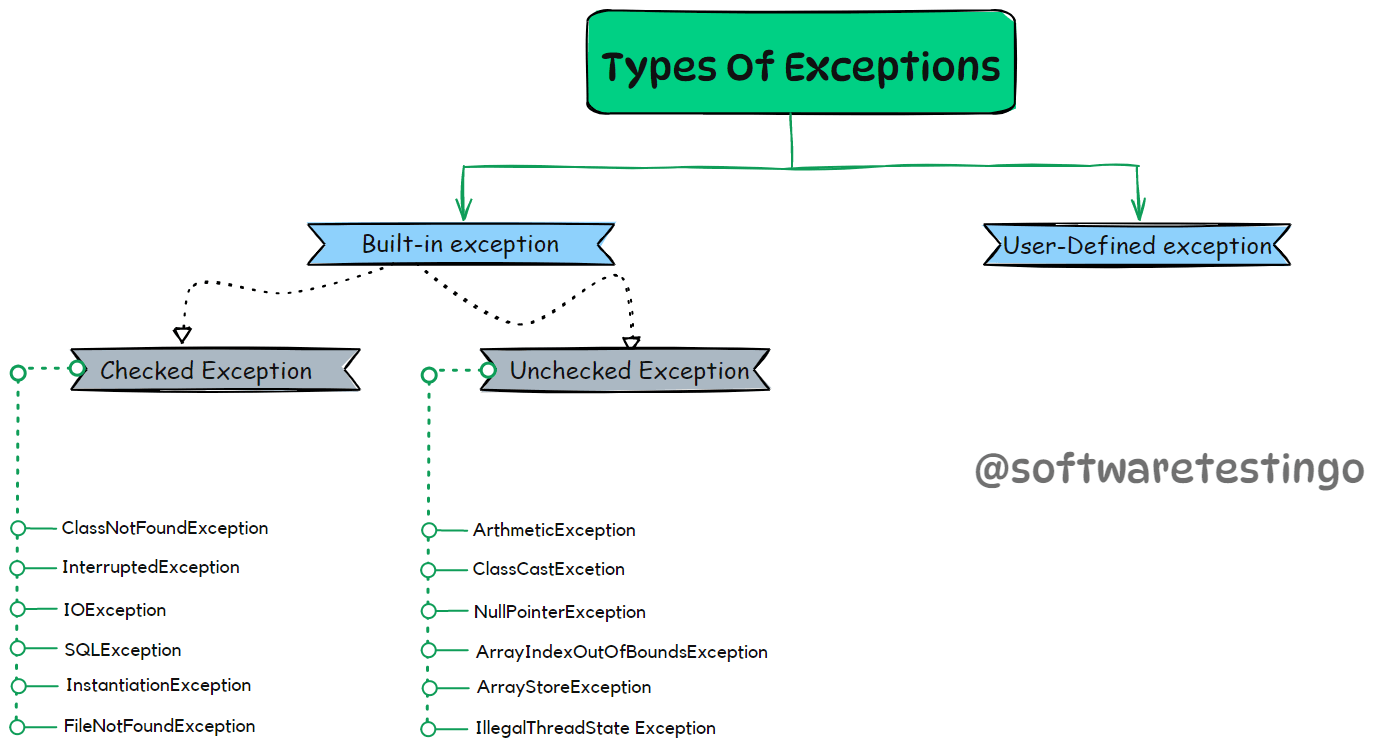
Checked Exceptions
Checked exceptions are exceptions that the Java compiler checks during the compilation time, and these exceptions do not come under the runtime exception class. If a method throws a checked exception in a program, it must either handle the exception within itself or pass it to the calling method.
We can handle the checked exceptions using the try-and-catch block or specify a throws clause in the method declaration. A compile-time error will occur if we do not handle the checked exceptions properly.
There is a common misconception that checked exceptions occur during compile-time, but that is incorrect. All exceptions happen during runtime, but the compiler detects some exceptions during compilation.
In Java, checked exceptions are those the Java compiler examines during compilation. Programmers must be prepared for checked exceptions and ensure their programs can handle them effectively. Apart from RuntimeException, Error and their subclasses fall under the checked exception.
Examples of Checked Exception
Unchecked Exceptions (Runtime Exceptions) in Java
In Java, the Java compiler does not check unchecked exceptions, but They are checked by the Java Virtual Machine (JVM). Unchecked Exceptions occur during the runtime of a program. Exceptions that fall under the runtime exception class are referred to as unchecked exceptions or runtime exceptions. If you are unsure which exception falls under the runtime exception class, you can check our Exception Hierarchy blog post, where we have discussed this in detail.
When we write and compile a Java program, we cannot see the impact of unchecked exceptions and errors until we run the program. The Java compiler allows us to write a Java program without explicitly handling unchecked exceptions and errors.
Unlike checked exceptions, the Java compiler does not verify whether the programmer has handled runtime exceptions at compile time. If a runtime exception occurs within a method and the programmer has not handled it, the JVM terminates the program without executing the remaining code.
Examples Of Unchecked Exceptions (Runtime Exceptions)
Conclusion:
Exceptions in Java play a crucial role as they help identify and handle errors that can lead to abnormal program termination. Dealing with exceptions can be time-consuming for programmers, impacting the program’s execution. Hence, it is important to minimize the occurrence of such critical exceptions during production or implementation.
After going through our blog post on the Types of Exceptions in Java, we encourage you to share your thoughts, comments, and suggestions in the comment box below. We value your feedback and would love to hear your insights or any additional information you may have on this topic. Your comments will help us improve our content and provide more valuable information to our readers. So please take a moment to leave a comment and let us know your thoughts.