Super Keyword in Java: The Keyword Super refers to the immediate parent class object. But we should know inheritance before we learn about a super keyword and how to use it in Java programming language. If you don’t know about inheritance, follow our Inheritance Java tutorial guide here.
The “super” keyword is used to refer to the superclass of a class. It is commonly used to access methods, fields, and constructors of the parent class from the child class. The “super” keyword avoids naming conflicts between the parent and child class and enables code reuse.
Use of Super Keyword
- You Can access the data member of the parent class using the super keyword when the same data member is present in both parent and child classes.
- You Can call the parent class no-arg constructor or parametrized constructor using the Super keyword in the child class.
- When you have to override a parent class method in the child class but want to call the parent class method, you need to use the super keyword to access the parent class method.
Let’s discuss using super keywords with variables, methods & constructors with simple examples to understand them easily.
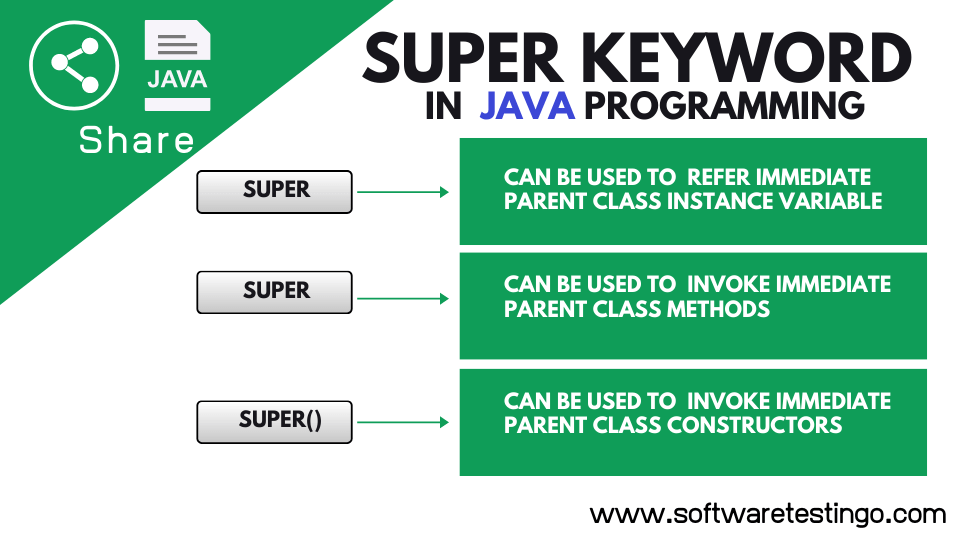
How to Use Super Keyword With Variables
Both parent and child classes have the same variable or data member in your program. In this case, there is a chance of ambiguity for JVM. So, to access the parent class variable from the child class, we can use the super keyword. Let’s go through a program so that you can understand how the super keyword works with the variables.
package com.SoftwareTestingo.JavaBasics; class Parent { //Super Class Variable int var=111; } public class Super_Keyword_With_Variable extends Parent { //Child Class Variable int var=222; public void printSuperVar() { System.out.println("Parent Class Var Value: "+super.var); } public static void main(String[] args) { Super_Keyword_With_Variable obj=new Super_Keyword_With_Variable(); System.out.println("The Value Of Local Variable: " + obj.var); obj.printSuperVar(); } }
Output:
The Value Of Local Variable: 222 Parent Class Var Value: 111
How to Use Super Keywords with Method
When you want to call the parent class method from the child class, where the parent and child class have the same method name, this type of situation is called method overriding. But there is a chance of ambiguity for the JVM because, in the child class, it has both parent and child class methods with the same name, so to resolve the ambiguity situation, we can use the super keyword.
Go through the example program below to understand clearly without any confusion.
package com.SoftwareTestingo.JavaBasics; class PClass { void show() { System.out.println("Parent Class Show Method Called"); } } public class Super_Keyword_With_Method extends PClass { void show() { System.out.println("Child Class Show Method Called"); } void callShowMethod() { show(); super.show(); } public static void main(String[] args) { Super_Keyword_With_Method obj=new Super_Keyword_With_Method(); obj.callShowMethod(); } }
Output:
Child Class Show Method Called Parent Class Show Method Called
Note: When the child class does not override the parent class methods, there is no need to use the super keyword to access the parent class methods.
How to Use Super Keywords with Constructor
When creating the child class object using the new keyword, it calls the child class default constructor. The child class constructor indirectly calls the parent class constructor. So, The order of execution of the construct is from parent class to child class.
It all happens because the JVM compiler automatically adds the super() statement at the beginning of the child class construct. So, the super() statement calls the no-args constructor of the parent class. In this way, the constructor execution comes from the parent class constructor to the child class constructor.
Let’s go through with an example to understand how the child class constructor calls the parent class constructor.
package com.SoftwareTestingo.JavaBasics; class ParentClass { public ParentClass() { System.out.println("Parent Class No Arg Consructor Executed"); } public ParentClass(String abc) { System.out.println("Parent Class With Argument Consructor Executed"); } } public class Super_Keyword_With_Constructor extends ParentClass { public Super_Keyword_With_Constructor() { //super(); super("Welcome"); System.out.println("Child Class Constructor Got Executed"); } public static void main(String[] args) { Super_Keyword_With_Constructor obj=new Super_Keyword_With_Constructor(); System.out.println("Main Method executed"); } }
Output:
Parent Class With Argument Consructor Executed Child Class Constructor Got Executed Main Method executed
How to Call the Parent Class parametrized Constructor from Child Class.
But If our requirement is called the parent class parameterized constructor, we can also achieve that by using the parametrized super() statement inside the child class constructor.
Go through with the below Example program:
package com.SoftwareTestingo.JavaBasics; class Super_Parent_Class { Super_Parent_Class() { System.out.println("Parent Class Default Constructor"); } Super_Parent_Class(String str) { System.out.println(str +": This is Parametrized Constructor Of Parent Class"); } } public class Super_Keyword_With_Param_Constructor extends Super_Parent_Class { Super_Keyword_With_Param_Constructor() { super("User"); System.out.println("Child Class Default Constructor"); } public void display() { System.out.println("Simply Printing Hello"); } public static void main(String[] args) { Super_Keyword_With_Param_Constructor obj=new Super_Keyword_With_Param_Constructor(); obj.display(); } }
Output:
User: This is Parametrized Constructor Of Parent Class Child Class Default Constructor Simply Printing Hello
Note: The Super() should be the first statement inside the method where you want to call the parent constructor.
Important Point About Super() In Constructor
- When using a super() statement in the child class constructor, the super() statement should be the first statement of the child class. Otherwise, you will get the compilation error: “Constructor call must be the first statement in a constructor”.
- If you have not added any super() statement inside the child class constructor at that time, the Java JVM compiler automatically adds a super() statement at the beginning of the child constructor; if the parent class doesn’t have any no-argument constructor at that time, you will get a compile-time error.
- When you mention the super() statement inside the child class constructor, the JVM compiler doesn’t call the parent class no-args constructor.
If you find any mistake or want to add more information to this post, comment in the comment section.
What are super keywords in Java?
The “super” keyword is used to refer to the superclass of a class. It is used to access methods, fields, and constructors of the parent class from the child class and avoid naming conflicts between the parent and child. It is commonly used to call the constructor of the parent class, access methods and fields of the parent class, and call a constructor or method of the parent class from within the constructor or method of the child class.
What is the difference between this() and super() in Java?
we use “This()” to invoke a constructor within the same class, but “super()” is used to invoke a constructor in the superclass. “This” refers to the current instance of the class, while “super” refers to the parent class.
What is the use of the super keyword in the Java interface?
The “super” keyword is impossible in interfaces because interfaces do not have parent classes. Nonetheless, when an interface extends another interface, the “super” keyword can be used to access the methods of the parent interface.
What are Super keyword significance? 🤔