StringBuilder Class In Java is used to create a changeable sequence of characters. Unlike the String class, which creates an unchangeable sequence of characters, StringBuilder allows us to modify the characters in the sequence.
It is similar to the StringBuffer class, as both provide an alternative to the String class by allowing changes to the character sequence. However, the main difference is that StringBuilder does not guarantee synchronization, while StringBuffer does.
StringBuilder is faster and more suitable for use in a single-threaded environment. If synchronization is needed for multiple threads, it is recommended to use StringBuffer. StringBuilder is not thread-safe but performs well compared to StringBuffer.
Post On: | StringBuilder Class In Java |
Post Type: | Java Tutorials |
Published On: | www.softwaretestingo.com |
Applicable For: | Freshers & Experience |
Get Updates: | SoftwareTestingo Telegram Group |
In this tutorial, we will use examples to learn about the StringBuilder class and its methods like append, reverse, delete, and toString.
What is StringBuilder in Java?
If you have the word “SoftwareTestingo” in a particular memory location, storing it in the StringBuilder class instead of the String class is better. No new memory is allocated when new characters are added as if you stored them in the String class. The StringBuilder class is mutable, meaning updating can be done within the sequence.
If we want to perform character manipulation, it’s better to use the StringBuilder class instead of the String class. Let’s take a closer look at some of the features of the StringBuilder class.
Syntax:
The StringBuilder class in Java allows you to create mutable strings. This means that you can change the value of a string after it has been created. This class extends the Object class, the parent class of all predefined and user-defined classes in Java.
The Class hierarchy is like this:
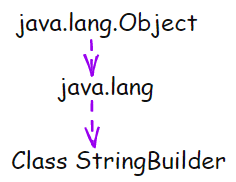
The StringBuilder class contains methods like hashCode() and equals(), and implements the two interfaces, Serializable and CharSequence. These features allow for different types of character sequences to be implemented.
The Syntax Of the StringBuilder Class is:
public final class StringBuilder extends Object implements Serializable, CharSequence
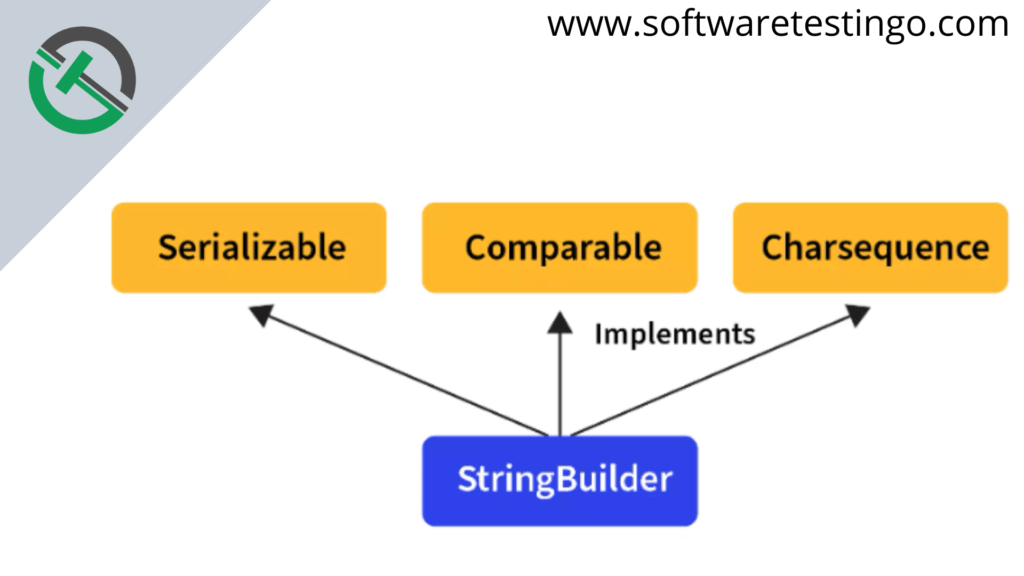
How does StringBuilder Work Internally?
What will happen when the Buffer gets full? A new Buffer double the size of the older buffer is automatically created. No new memory is allocated for every update. Also, StringBuilder is not synchronized, meaning multiple threads can access the StringBuilder object simultaneously. Therefore, StringBuilder is not a thread-safe class.
StringBuilder Class Constructors
The StringBuilder class provides different types of constructors that help convert an ordinary sequence of characters to a StringBuilder format and configure properties such as size. Let’s discuss the different constructors one by one.
StringBuilder(): Constructs a string builder with no characters and an initial capacity of 16 characters. The maximum number of characters that a StringBuilder object can store is known as the capacity of the StringBuilder.
Syntax:
StringBuilder obj=new StringBuilder();
Example:
package com.softwaretestingo.stringbuilder; public class StringBuilderEx { public static void main(String[] args) { StringBuilder obj = new StringBuilder("Software Blog"); obj.insert(8, "Testingo"); System.out.println(obj); } }
Output:
SoftwareTestingo Blog
StringBuilder(int capacity): This constructor initializes a new instance of the StringBuilder class with no characters and the specified capacity. The following is the syntax for this constructor.
Syntax:
StringBuilder obj=new StringBuilder(10);
package com.softwaretestingo.stringbuilder; public class StringBuilderEx2 { public static void main(String[] args) { StringBuilder obj = new StringBuilder(10); // print string System.out.println("Object capacity = "+ obj.capacity()); } }
Output:
Object capacity = 10
StringBuilder(CharSequence seq): This constructor creates a StringBuilder of the char sequence. The char sequence is the sequence of characters in a string.
StringBuilder obj=new StringBuilder(CharSequence);
package com.softwaretestingo.stringbuilder; public class StringBuilderEx4 { public static void main(String[] args) { StringBuilder obj = new StringBuilder("AAAABBBCCCC"); // print string System.out.println("String1 = "+ obj.toString()); } }
Output:
String1 = AAAABBBCCCC
StringBuilder(String str): Constructs a string builder initialized to the contents of the specified string.
Syntax:
StringBuilder ob=new StringBuffer(String);
package com.softwaretestingo.stringbuilder; public class StringBuilderEx5 { public static void main(String[] args) { StringBuilder obj = new StringBuilder("AAAABBBCCCC"); // using StringBuilder(String) constructor StringBuilder obj1= new StringBuilder(obj.toString()); // print string System.out.println("Object 1 = "+ obj1.toString()); } }
Output:
Object 1 = AAAABBBCCCC
Methods of StringBuilder Class
The StringBuilder class provides a variety of inbuilt methods that can be used to perform different operations on Strings. Let’s discuss each method one by one.
append(): This method attaches the passed string at the end of the existing string.
This method takes one parameter. This method adds a specified value to the end of a StringBuilder sequence. The value can be any data type.
package com.softwaretestingo.stringbuilder; public class StringBuilderEx6 { public static void main(String[] args) { StringBuilder first = new StringBuilder("Software"); first.append("Testingo"); System.out.println("After Append: "+first); first.append(111); System.out.println("After Adding Different Datatype: "+first); } }
Output:
After Append: SoftwareTestingo After Adding Different Datatype: SoftwareTestingo111
Insert(): The capacity of a StringBuilder object can be found using this method. The default capacity of the StringBuilder class is 16 bytes. If the capacity is full, the new capacity will be current (capacity+1)*2.
package com.SoftwareTestingO.Strings; public class StringBuilderEx3 { public static void main(String[] args) { StringBuilder first = new StringBuilder(); System.out.println("Default Capacity: "+first.capacity()); //Capacity increase by (16(default capacity)+1)*2=34 first.append("aaaaaaaaaaaaaaaaaa"); System.out.println("After Full, New Capacity: "+first.capacity()); } }
substring() method:
Converting The StringBuilder Object to a String
Never fear if you need to change your StringBuilder object into a regular old string! The StringBuilder class provides a method, StringBuilder.toString(), which does just that.
package com.SoftwareTestingO.Strings; public class StringBuilderEx4 { public static void main(String[] args) { StringBuilder first = new StringBuilder(); first.append("SoftwareTestingo"); //Convert Stringbuilder to String System.out.println(first.toString()); } }
What is the use of String Builder?
The StringBuilder class is useful for storing mutable elements. This saves memory because no extra memory is used after updating the StringBuilder sequence.
Why do we use String Builder in Java?
One of the main reasons is that StringBuilder is not thread-safe. When multiple threads can access a StringBuilder object, it increases performance because no thread has to wait for its turn for a particular operation.
Is String Builder efficient?:
Yes, StringBuilder is efficient due to its mutable and non-thread-safe properties.
Which is better: StringBuilder or String?:
StringBuilder is better because it is 6000 times faster than the String class.