Static Keyword In Java: In the previous article, we discussed the do-while loop, a continuation post of the core Java tutorial post; we will learn about the static keyword in Java and its uses. In Java programming language, static keywords are used regularly.
In Java programming, the static keyword defines a class-level variable, method, or block shared across all class instances. When a variable or method is declared static, it is associated with the class rather than a specific instance of the class. This means that the variable or method can be accessed without creating an object of the class, using the class name instead.
The static keyword can be used in various contexts within a Java program, but before that, we will discuss a few more uses of the Static keyword:
Static Keyword Uses
The Static keyword in Java is used for memory management. We can use the static keyword with class, variable, methods, and block if a class has static members that belong to the classes instead of specific instances. That means if you make a member static, we can invoke those members without creating the objects.
Before going deeper, let’s take a simple example to understand better how the static keyword works.
class SimpleStaticEx { // This is a static method static void myDemoMethod() { System.out.println("Method Executed"); } public static void main(String[] args) { /* We are calling a static method without creating an object */ myDemoMethod(); } }
We have seen in the above example the myDemoMethod is a static method, and we are calling that method without creating an object. That means when we are creating a static member, then that is created at the class level. If we remove the static keyword, then to access that member, we have to create an instance of that class; otherwise, we can’t access that.
Static Variables
When you declare a variable with the keyword static, a single copy of that variable is created and shared among all objects or instances of the class. When declaring some global variables, we mostly define those variables with the static keyword. So that all instances of those classes can share the same static variable.
Note:
- We can create a static variable at a class level only.
- The execution order of the Static variable and the static block is the same as they are present in the program.
Let’s take an example to understand how the static variable and static block are executed.
Static Variable Example
package java_Basics; public class StaticVariable_Example { static int count=0; public void increment() { count++; } @SuppressWarnings("static-access") public static void main(String[] args) { StaticVariable_Example obj1=new StaticVariable_Example(); StaticVariable_Example obj2=new StaticVariable_Example(); obj1.increment(); obj2.increment(); System.out.println("Obj1: count is="+obj1.count); System.out.println("Obj2: count is="+obj2.count); } }
Output:
Obj1: count is=2 Obj2: count is=2
Static Method
When we define a method with the static keyword, that method is called a static method. The Common static method we regularly use when writing in any Java program is the main() method. As discussed above, we can access any static member before creating any objects of that class and without reference to any object; that’s why main() is executed without creating an object of that class.
The static method has several restrictions:
- They can directly call other static methods and static members.
- It cannot refer to this or super variable or methods.
When do you need to use Static variables and methods?
Using static variables and methods can be useful when you need to share data or functionality across multiple instances of a class. However, it is important to use them judiciously, as they can lead to difficult code to maintain and test. Additionally, using static variables can lead to synchronization issues in multithreaded environments, as multiple threads may attempt to access or modify the same static variable simultaneously.
That’s why you should use the static variables for all properties that are common to all. For example, all employees share the same organization name in an organization. Similarly, we need static methods to change the static variable’s value.
package java_Basics; public class StaticMethod_Example { static int var = 10; static String str = "SoftwareTestingo.com"; //This is a static method public static void main(String[] args) { System.out.println("Variable Value: "+var); System.out.println("String Value: "+str); } }
Output:
Variable Value: 10 String Value: SoftwareTestingo.com
Static Blocks
A static block is a code block executed when a class is loaded into memory. It performs any static initialization required for the class, such as initializing static variables, performing checks, or registering drivers for databases.
Static blocks can be useful for a number of different purposes, such as initializing static variables, performing security checks, or loading drivers for databases. They are executed only once when the class is loaded into memory and cannot be manually called.
Here’s an example of a static block that initializes a static variable:
public class MyClass { static int x; static { x = 10; } }
Static blocks can also perform more complex initialization tasks, such as registering drivers for a database. Here’s an example:
public class MyDatabase { static { try { Class.forName("com.mysql.jdbc.Driver"); } catch (ClassNotFoundException e) { throw new RuntimeException("Unable to load driver class!", e); } } }
For a Better understanding of the concept, refer to the below program:
package java_Basics; public class StaticBlock_Example { static int num; static String mystr; static { num = 100; mystr = "Static String Varibale Initilized inside static block"; } public static void main(String[] args) { System.out.println("Value of num: "+num); System.out.println("Value of mystr: "+mystr); } }
Output:
Value of num: 100 Value of mystr: Static String Varibale Initilized inside static block
Static Nested Classes
We can not declare the top-level classes with static modifiers, but we can declare the inner classes with static modifiers. Such types of classes are called Nested static classes.
How does Static Block work in Java with Example Updated?
package com.java.Softwaretestingblog; class Staticsimple1 { static { System.out.println("Staticsimple1 Static Block"); } Staticsimple1() { System.out.println("Staticsimple1 constructor Called"); } } class Staticsimple2 extends Staticsimple1 { static { System.out.println("Staticsimple2 Static Block"); } Staticsimple2() { super(); System.out.println("Staticsimple2 Constructor Called"); } } public class StaticBlockExample { static { System.out.println("Main Method Static Block"); } public static void main(String[] args) { // TODO Auto-generated method stub @SuppressWarnings("unused") StaticBlockExample obj=new StaticBlockExample(); @SuppressWarnings("unused") Staticsimple2 obj1=new Staticsimple2(); @SuppressWarnings("unused") Staticsimple1 obj2=new Staticsimple1(); } }
Output:
Main Method Static Block Staticsimple1 Static Block Staticsimple2 Static Block Staticsimple1 constructor Called Staticsimple2 Constructor Called Staticsimple1 constructor Called
How does the static method work in Java? With example?
package com.java.Softwaretestingblog; class StaticComponents { static int staticVariable; static { System.out.println("StaticComponents SIB"); staticVariable = 10; } static void staticMethod() { System.out.println("From StaticMethod"); System.out.println(staticVariable); } } public class MainClassExample { //First MainClass Static Method Executed Later Above Class Static Method Executed static { System.out.println("MainClass SIB"); } public static void main(String[] args) { //Static Members directly accessed with Class Name StaticComponents.staticVariable = 20; StaticComponents.staticMethod(); } }
Output:
StaticComponents SIB From StaticMethod 20
Method Hiding In Java
Method hiding in Java is a concept that allows a subclass to define a method with the same name and signature as a method in its superclass, thereby hiding the superclass method. Method hiding occurs when a static method in a subclass has the same signature as a static method in its superclass. This mechanism is called method hiding in Java or function hiding.
Both parent and child class methods must be static.
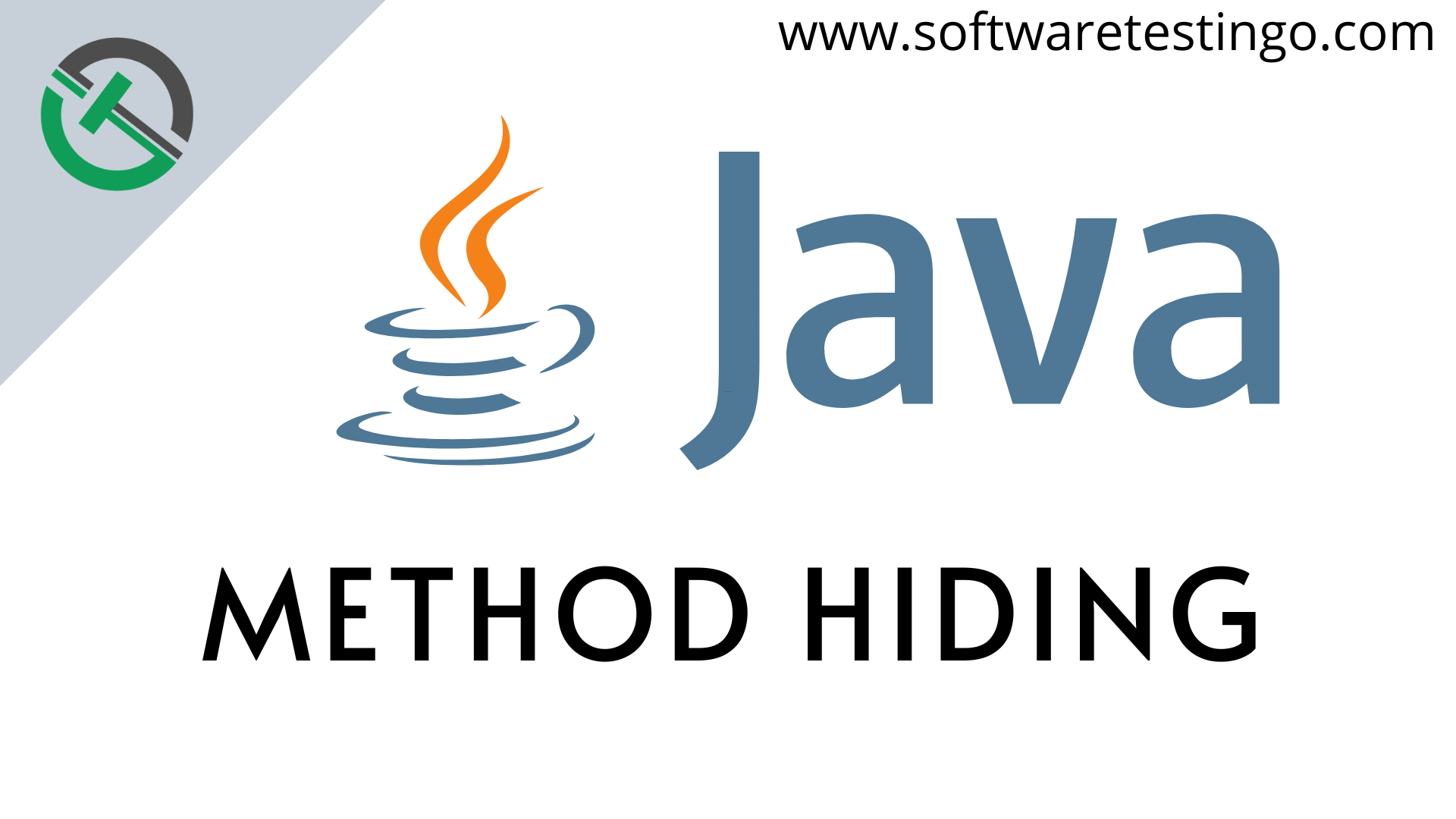
Features of Method Hiding in Java
- Method hiding, which involves hiding a method in a subclass with the same name and signature as a method in its superclass, is sometimes called compile-time polymorphism because it is the compiler’s responsibility to resolve method resolution based on the reference type.
- Method hiding is also known as static polymorphism or early binding.
- In method hiding, the method call is always resolved by the Java compiler based on the reference type, and there is no involvement of runtime polymorphism in this process.
- It is important to ensure that static in a method declaration is consistent between the superclass and subclass when implementing method hiding in Java.
package com.softwaretestingo.AccessModifier; class parent { public static void foo() { System.out.println("Inside foo method in parent class"); } public void bar() { System.out.println("Inside bar method in parent class"); } } class Child extends parent { // Hiding public static void foo() { System.out.println("Inside foo method in child class"); } // Overriding public void bar() { System.out.println("Inside bar method in child class"); } } public class MethodHidingEx { public static void main(String[] args) { parent p = new parent(); //Here c is the reference variable of parent class but points to Child class parent c = new Child(); System.out.println("****************Method Hiding*******************"); p.foo(); // This will call method in parent class c.foo(); // This will call method in parent class System.out.println("****************Method overriding*******************"); p.bar(); // This will call method in parent class c.bar(); // This will call method in child class } }
Output:
****************Method Hiding******************* Inside foo method in parent class Inside foo method in parent class ****************Method overriding******************* Inside bar method in parent class Inside bar method in child class
Conclusion:
The static keyword is an essential concept in Java programming language used for memory management, data sharing, and global initialization. With static variables, methods, and blocks, you can access class-level resources without creating an instance of the class. By using static methods and variables, you can easily share data and functionality across multiple instances of a class.
However, static variables and methods should be judiciously applied, considering the potential synchronization issues in multithreaded environments. Understanding the concept of the static keyword and its uses is crucial for developers to write efficient and maintainable Java code.
Your feedback is incredibly valuable to us. If you have any suggestions for how we can improve our blog or if you noticed anything we missed, please leave a comment below.