This tutorial will teach us about the Java Stack class and its methods. We will go through some examples to help illustrate how these methods work.
A stack is a collection of objects inserted and removed in order. The name “stack” comes from the fact that it resembles a stack of plates. So, when we need an object, we take it from the top of the stack (pop), and when we want to add an object, we put it at the top (push). We cannot add or remove an object from the middle of the stack.
Post On: | Stack in Java |
Post Type: | Java Tutorials |
Published On: | www.softwaretestingo.com |
Applicable For: | Freshers & Experience |
Get Updates: | We are Available Telegram |
Stack is a fundamental data structure, and it is used in many applications. One such application is the undo mechanism in text editors. All changes are stored in a stack; the most recent action pops up when you undo something. When you make changes, it pushes those changes onto the stack.
Introduction to Stack in Java
The Java Collection framework provides a Stack class that models and implements a Stack data structure. The class is based on the basic principle of last-in-first-out. In addition to the basic push and pop operations, the class provides three more functions: empty, search, and peek.
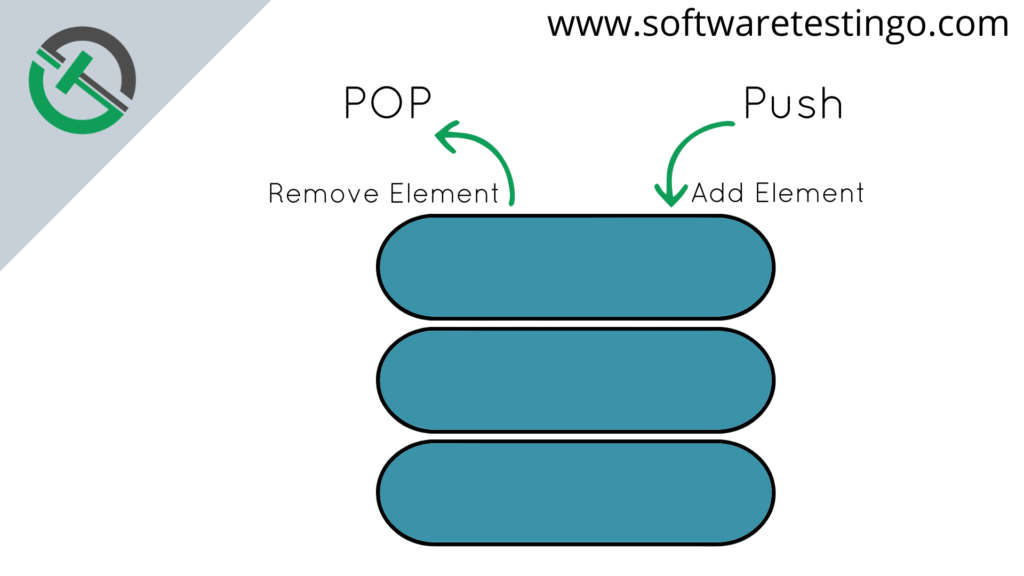
The Stack class is a subclass of Vector and can be considered a stack with the five mentioned functions. The below diagram shows the hierarchy of the Stack class:
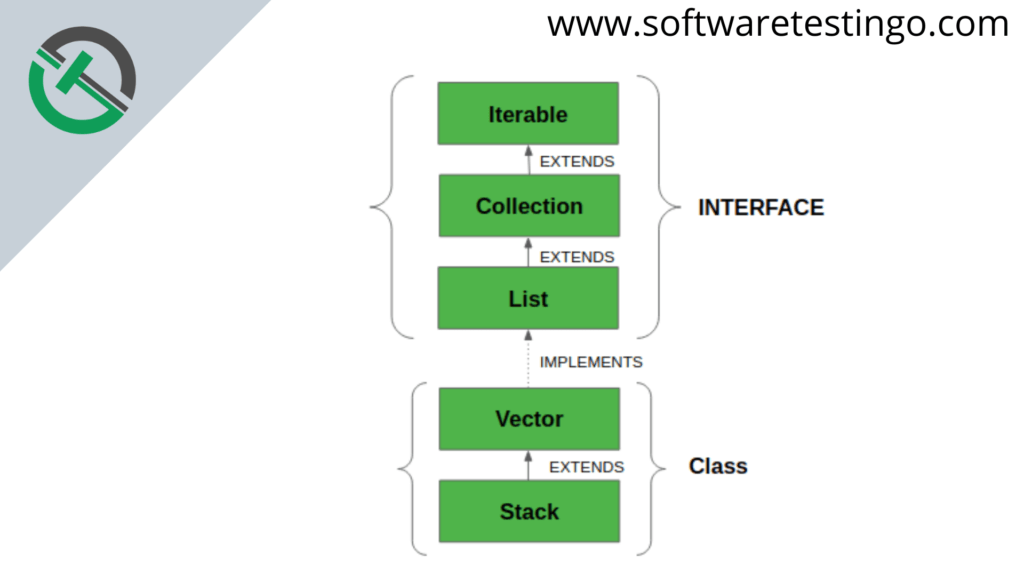
Creating a Stack
To create a stack in Java, we must first import the java.util.Stack package. Once we have imported the package, we can create a stack by following these steps:
Stack<Type> stacks = new Stack<>(); // Create Integer type stack Stack<Integer> stacks = new Stack<>(); // Create String type stack Stack<String> stacks = new Stack<>();
Methods of Java Stack
The Java Stack Methods are essential for creating the stack class, as they involve combining all five common operations.
Adding Elements:
We can use the push() method to add an element to the stack. This will place the element at the top of the stack.
package com.SoftwareTestingO.collections; import java.util.Stack; public class StackAdd { public static void main(String[] args) { // Default initialization of Stack Stack stack1 = new Stack(); // Initialization of Stack // using Generics Stack<String> stack2 = new Stack<String>(); // pushing the elements stack1.push("WelCome"); stack1.push("To"); stack1.push("SoftwareTestingo"); stack2.push("Software"); stack2.push("Testingo"); stack2.push("Blog"); // Printing the Stack Elements System.out.println(stack1); System.out.println(stack2); } }
Accessing the Element:
The peek() method can be used to retrieve or fetch the first element of the Stack or the element present at the top of the Stack. The element retrieved does not get deleted or removed from the Stack.
package com.SoftwareTestingO.collections; import java.util.Stack; public class StackPeek { public static void main(String[] args) { // Creating an empty Stack Stack<String> stack = new Stack<String>(); // Use push() to add elements into the Stack stack.push("Welcome"); stack.push("To"); stack.push("SoftwareTestingo"); stack.push("Blog"); // Displaying the Stack System.out.println("Initial Stack: " + stack); // Fetching the element at the head of the Stack System.out.println("The element at the top of the"+" stack is: " + stack.peek()); // Displaying the Stack after the Operation System.out.println("Final Stack: " + stack); } }
Removing Elements:
If you want to remove an element from the stack, you can use the pop() method. This will remove the element from the top of the stack.
package com.SoftwareTestingO.collections; import java.util.Stack; public class StackPop { public static void main(String[] args) { // Creating an empty Stack Stack<String> stack = new Stack<String>(); // Use push() to add elements into the Stack stack.push("Welcome"); stack.push("To"); stack.push("SoftwareTestingo"); stack.push("Blog"); // Displaying the Stack System.out.println("Initial Stack: " + stack); // Removing the element System.out.println("Removed Element: " + stack.pop()); // Displaying the Stack after the Operation System.out.println("Final Stack: " + stack); } }
Checking Stack Empty or Not:
This method checks if a value is present on the top of the stack. If the value is not present, it will return true. If the value is present, it will return false.
package com.SoftwareTestingO.collections; import java.util.Stack; public class StackIsEmpty { public static void main(String[] args) { // Creating an empty Stack Stack<String> stack = new Stack<String>(); // Use push() to add elements into the Stack stack.push("Welcome"); // Checking the Stack System.out.println("Current Stack: " + stack); System.out.println("Is Stack Is Empty? " + stack.isEmpty()); // Removing the element System.out.println("Removed Element: " + stack.pop()); // Displaying the Stack after the Operation System.out.println("Is Stack Is Empty? " + stack.isEmpty()); } }
Search Element In Stack:
This method is used to search for an element in a stack and to check if that element is present. If the element being searched for matches the element at the top of the stack, it will return a value; otherwise, it will return -1.
package com.SoftwareTestingO.collections; import java.util.Stack; public class StackSearch { public static void main(String[] args) { // Creating an empty Stack Stack<String> stack = new Stack<String>(); // Use push() to add elements into the Stack stack.push("Welcome"); stack.push("To"); stack.push("SoftwareTestingo"); stack.push("Blog"); // Checking the Stack System.out.println("Current Stack: " + stack); //Checking For InValid Value System.out.println("Is Element Present " + stack.search("Testing")); // Checking For a Valid Value System.out.println("Is Element Present " + stack.search("SoftwareTestingo")); } }
Conclusion
The Java Stack Methods are extremely helpful for constructing the stack class. This gives the entire Java collection Framework an advantage by making it more flexible and versatile. The vector class subclass uses all the operations like pop, push, peek, empty, and search. By providing a prototype for the stack data structure, it helps immensely.