Welcome to our tutorial on the Java SortedMap interface and its methods. In this tutorial, we will cover the SortedMap interface, how it works, and some of its most useful methods. By the end of this tutorial, you should understand how to work with the SortedMap interface in your own code. Let’s get started!
SortedMap is an interface in the collection framework providing a total order of its elements. The class that implements this interface is TreeMap, which allows you to traverse the elements in a sorted order of keys.
Post On: | SortedMap In Java |
Post Type: | Java Tutorials |
Published On: | www.softwaretestingo.com |
Applicable For: | Freshers & Experience |
Get Updates: | SoftwareTestingo Telegram Group |
SortedMap is great for ordering keys by natural ordering or specified comparator. If you want a map that satisfies the following criteria, consider using TreeMap:
- You can’t have a null key or value – it’s not allowed!
- The keys can be sorted by their natural order or a specified comparator. This makes it easy to find the right key for your needs.
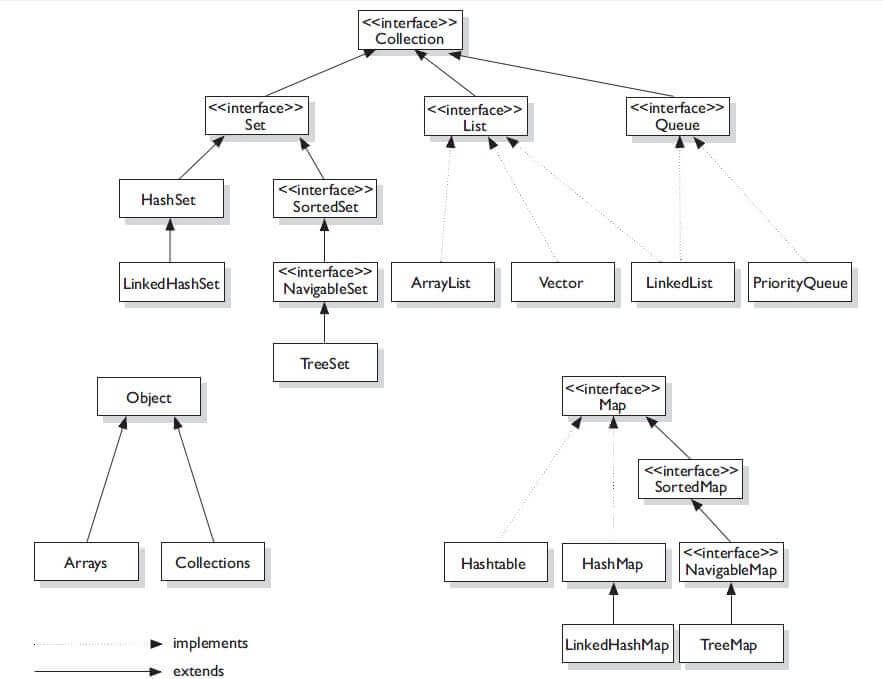
How to use SortedMap?
Since SortedMap is an interface, we always need a class that extends this interface in order to create an object. However, with the introduction of Generics in Java 1.5, it is now possible to restrict the type of object that can be stored in a SortedMap.
To use the SortedMap, we must first import the java.util.SortedMap package. Once we have imported the package, we can create a sorted map by following these steps:
// SortedMap implementation by TreeMap class SortedMap<Key, Value> numbers = new TreeMap<>();
Different Operations Of SortedMap
Since SortedMap is an interface, it can only be used with a class that implements this interface. TreeMap is the class that implements the SortedMap interface. Now, let’s look at how to perform some of the most frequently used operations on TreeMap.
Adding Elements:
To add an element to the SortedMap, we can use the put() method. However, insertion order is not retained in TreeMap since keys are compared and sorted internally in ascending order.
package com.SoftwareTestingO.collections; import java.util.SortedMap; import java.util.TreeMap; public class SortedMapAddEx { public static void main(String[] args) { // Default Initialization SortedMap stm1 = new TreeMap(); // Initialization using Generics SortedMap<Integer, String> stm2=new TreeMap<Integer, String>(); // Inserting the Elements stm1.put(1, "Software"); stm1.put(2, "Testingo"); stm1.put(3, "Blog"); stm2.put(new Integer(1), "Java"); stm2.put(new Integer(2), "Linux"); stm2.put(new Integer(3), "Tutorials"); // Displaying the SortedMap System.out.println(stm1); System.out.println(stm2); } }
Changing Elements:
If we want to change an element after adding it, we can use the put() method. Since the elements in a SortedMap are indexed according to their keys, we can insert an updated value for the key of the element we want to change.
package com.SoftwareTestingO.collections; import java.util.SortedMap; import java.util.TreeMap; public class SortedMapChangingEx { public static void main(String[] args) { // Default Initialization SortedMap stm1 = new TreeMap(); // Initialization using Generics SortedMap<Integer, String> stm2=new TreeMap<Integer, String>(); // Inserting the Elements stm1.put(1, "Software"); stm1.put(2, "Testingo"); stm1.put(3, "Blog"); // Before Changing The element System.out.println("Before Changing The element: "+stm1); // Changing the elements stm1.put(3, "Learn"); // After Changing The element System.out.println("After Changing The element: "+stm1); } }
Removing Element:
The remove() method is great for deleting an element from the SortedMap. All you need is the key value; this method will erase any trace of the mapping for that key from the map.
package com.SoftwareTestingO.collections; import java.util.SortedMap; import java.util.TreeMap; public class SortedMapRemoveEx { public static void main(String[] args) { // Default Initialization SortedMap stm1 = new TreeMap(); // Initialization using Generics SortedMap<Integer, String> stm2=new TreeMap<Integer, String>(); // Inserting the Elements stm1.put(1, "Software"); stm1.put(2, "Testingo"); stm1.put(3, "Blog"); // Before Changing The element System.out.println("Before Remove The element: "+stm1); // Remove the elements stm1.remove(3); // After Remove The element System.out.println("After Changing The element: "+stm1); } }
Iterating through the SortedMap:
There are several ways to iterate through a Map. The most popular way is to use an enhanced for loop and get the keys. You can find the value of the key by using the getValue() method.
package com.SoftwareTestingO.collections; import java.util.Map; import java.util.SortedMap; import java.util.TreeMap; public class SortedMapIteratingEx { public static void main(String[] args) { // Generic Initialization SortedMap<Integer, String> stm1 = new TreeMap<Integer, String>(); // Initialization using Generics SortedMap<Integer, String> stm2=new TreeMap<Integer, String>(); // Inserting the Elements stm1.put(1, "Software"); stm1.put(2, "Testingo"); stm1.put(3, "Blog"); for(Map.Entry element : stm1.entrySet()) { int key=(int) element.getKey(); String value=(String) element.getValue(); System.out.println(key +" -->" + value); } } }
Conclusion:
I hope you found this tutorial on the SortedMap interface in Java helpful and that you now have a good understanding of the topic.