In this tutorial, we’ll be discussing Java’s Queue interface. This interface allows you to create a queue of objects in your Java program. We’ll review how to use the Queue interface and some of its most important methods.
Post On: | Queue In Java |
Post Type: | Java Tutorials |
Published On: | www.softwaretestingo.com |
Applicable For: | Freshers & Experience |
Get Updates: | SoftwareTestingo Telegram Group |
The Java Queue interface is available in java.util package and extends the java.util.Collection interface. A queue is a data structure that stores elements in a sequence. The first element added to the queue (enqueued) will be the first to be removed (dequeued). This principle is called the FIFO method (First In, First Out).
Let’s Take a Real-world example: If you’re waiting in line for a concert, a new person will join the end of the line. The person at the front of the line would leave and be able to enter the venue.
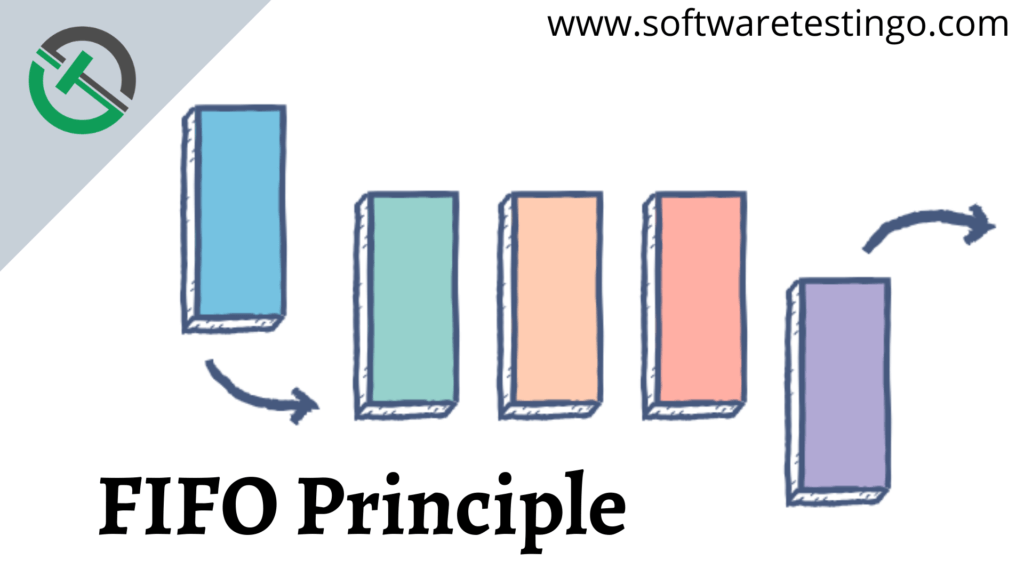
Java Queue Class Diagram
Classes that Implement Queue
Since the Queue is an interface, we cannot provide a direct implementation of it. To use the functionalities of Queue, we need to use classes that implement it.
- ArrayDeque
- LinkedList
- PriorityQueue
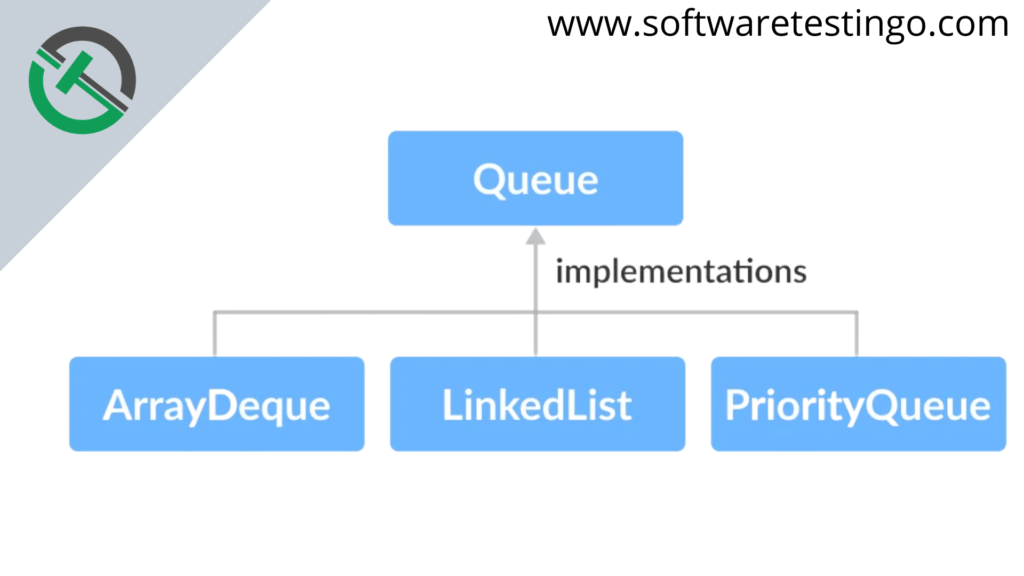
Interfaces that extend Queue
The Queue interface can be extended by various subinterfaces, which can be helpful in different situations. For example, the Deque interface provides additional methods for double-ended queues.
- Deque
- BlockingQueue
- BlockingDeque
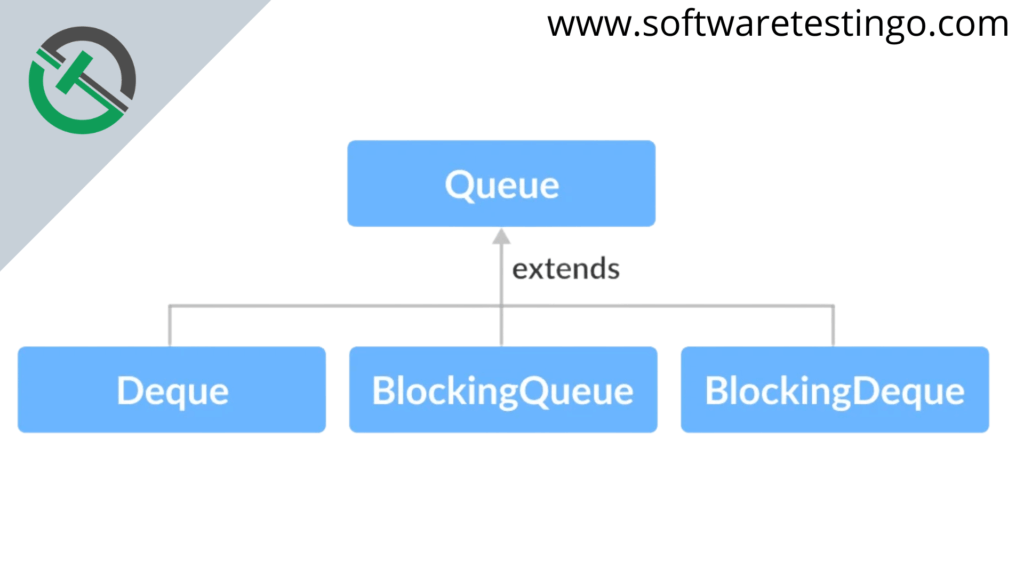
How Is Queue Working?
In queues, elements are stored and accessed first in, First Out. This means elements are added from the back and removed from the front.
Adding an element to the back of a queue is called Enqueue, and removing an element from the front of a queue is called Dequeue.
How to use Queue?
To use Queue in Java, we must import the java.util.Queue package.
// LinkedList implementation of Queue Queue<String> obj1= new LinkedList<>(); // Array implementation of Queue Queue<String> obj2= new ArrayDeque<>(); // Priority Queue implementation of Queue Queue<String> obj3 = new PriorityQueue<>();
Methods Of Queue
The Queue interface includes all the methods of the Collection interface because it is a sub-interface.
Adding Elements:
You can use the add() method to add an element to a queue. The PriorityQueue does not retain insertion order – instead, elements are stored based on priority order (ascending by default).
package com.SoftwareTestingO.collections; import java.util.PriorityQueue; import java.util.Queue; public class AddQueueEx { public static void main(String[] args) { Queue<String> obj = new PriorityQueue<>(); obj.add("Java"); obj.add("Linux"); obj.add("Script"); // Print All the Elements System.out.println(obj); } }
Removing Elements:
If you want to remove an element from a queue, you can use the remove() method. This will remove the first occurrence of the object. If there are multiple objects that you want to remove, then you can use the poll() method. This will remove the head and return it.
package com.SoftwareTestingO.collections; import java.util.PriorityQueue; import java.util.Queue; public class RemoveQueueEx { public static void main(String[] args) { Queue<String> obj = new PriorityQueue<>(); obj.add("Java"); obj.add("Linux"); obj.add("Script"); // Print All the Elements System.out.println(obj); obj.remove("Linux"); System.out.println("After Remove " + obj); System.out.println("Poll Method " + obj.poll()); System.out.println("Final Queue " + obj); } }
Iterating the Queue:
There are a few different ways to iterate through a Queue. The most popular method is converting the queue into an array and using a for loop to traverse it. However, the Queue also has its built-in iterator, which can go through the elements in the Queue.
package com.SoftwareTestingO.collections; import java.util.Iterator; import java.util.PriorityQueue; import java.util.Queue; public class IteratingQueueEx { public static void main(String[] args) { Queue<String> obj = new PriorityQueue<>(); obj.add("Java"); obj.add("Linux"); obj.add("Script"); Iterator iterator = obj.iterator(); while (iterator.hasNext()) { System.out.print(iterator.next() + " "); } } }
Classes That Implement the Queue Interface
The Java Queue interface extends the collection of interfaces. In particular, it extends the iterable interface. Several classes implement queues, including:
There are various types of queues, such as LinkedList, PriotityQueue, ArrayBlockingQueue, DelayQueue, LinkedBlockingQueue, and PriotityBlockingQueue. The queue interface is designed to make it easier to implement queues.
PriorityQueue:
The PriorityQueue class in the collection framework provides a way to process objects based on priority. A queue typically follows the First-In-First-Out algorithm, but sometimes, elements in the queue need to be processed according to priority. That’s when PriorityQueue comes into play. Let’s see how to create a queue object using this class.
package com.SoftwareTestingO.collections; import java.util.PriorityQueue; import java.util.Queue; public class PriorityQueueEx { public static void main(String[] args) { Queue<String> obj = new PriorityQueue<>(); obj.add("Java"); obj.add("Linux"); obj.add("Script"); // Printing the top element and removing it System.out.println("Extracting Top Element & Show: "+obj.poll()); // Printing the top element again System.out.println("Top Element After Remove:" +obj.peek()); } }
LinkedList:
LinkedList class implements the collection framework, which implements the linked list data structure. It is a linear data structure where elements are not stored in contiguous locations, but each element is its object with a data and addresses part.
The elements in a LinkedList are linked together using pointers and addresses. Each element is known as a node. LinkedList is preferred over arrays or queues because they’re more dynamic and easy to insert and delete elements. Let’s see how to create a queue object using this class.
package com.SoftwareTestingO.collections; import java.util.LinkedList; import java.util.Queue; public class LinkedListQueueEx { public static void main(String[] args) { Queue<Integer> li= new LinkedList<Integer>(); li.add(111); li.add(222); li.add(333); // Printing the top element Before Remove System.out.println("Top Element Before Remove:" +li.peek()); // Printing the top element and removing it System.out.println("Extracting Top Element & Show: "+li.poll()); // Printing the top element again System.out.println("Top Element After Remove:" +li.peek()); } }
PriorityBlockingQueue:
Please note that neither the PriorityQueue nor LinkedList implementations are thread-safe. If you need a thread-safe implementation, consider using PriorityBlockingQueue.
The PriorityBlockingQueue class is an unbounded blocking queue that uses the same ordering rules as the PriorityQueue class. It provides blocking retrieval operations, meaning that if you try to retrieve an element from the queue and it’s empty, your thread will be blocked until an element becomes available. However, since it is unbounded, adding elements may sometimes fail due to resource exhaustion, resulting in OutOfMemoryError. Let’s look at how to create a queue object using this class.
package com.SoftwareTestingO.collections; import java.util.Queue; import java.util.concurrent.PriorityBlockingQueue; public class PriorityBlockingQueueQueueEx { public static void main(String[] args) { Queue<Integer> obj = new PriorityBlockingQueue<>(); obj.add(123); obj.add(456); obj.add(789); // Printing the top element Before Remove System.out.println("Top Element Before Remove:" +obj.peek()); // Printing the top element and removing it System.out.println("Extracting Top Element & Show: "+obj.poll()); // Printing the top element again System.out.println("Top Element After Remove:" +obj.peek()); } }
Conclusion
This article has covered everything there is to know about Java Queues. By the end of this article, you should understand how queues work and can implement them in your programs.