Welcome to this tutorial on the PriorityQueue class of the Java collections framework. In this lesson, we’ll learn how to use this powerful tool through examples. By the end of this tutorial, you’ll be an expert on using PriorityQueues in your Java programming projects. So let’s get started!
If you need to process objects based on priority rather than FIFO, then a PriorityQueue is what you’re looking for. It’s implemented in Java using the Queue interface and uses a Binary Heap internally.
Post On: | PriorityQueue In Java |
Post Type: | Java Tutorials |
Published On: | www.softwaretestingo.com |
Applicable For: | Freshers & Experience |
Get Updates: | SoftwareTestingo Telegram Group |
Priority Queue in Java
A PriorityQueue is perfect when you need to process objects based on priority. As we all know, a Queue follows the First-In-First-Out algorithm, but In PriorityQueue, items are retrieved according to their priorities. By default, the priority is determined by objects’ natural ordering, but this can be overridden by a Comparator provided at queue construction time.
A priority queue is a data structure that allows users to insert elements in any order but retrieve them in a sorted manner. Priority queues use a data structure called a binary heap to store elements. A binary heap is a self-organizing binary tree that adjusts itself each time elements are added or removed from it. In the case of min-heap, the smallest element (1) is always kept at the very top, regardless of the order in which it was inserted.
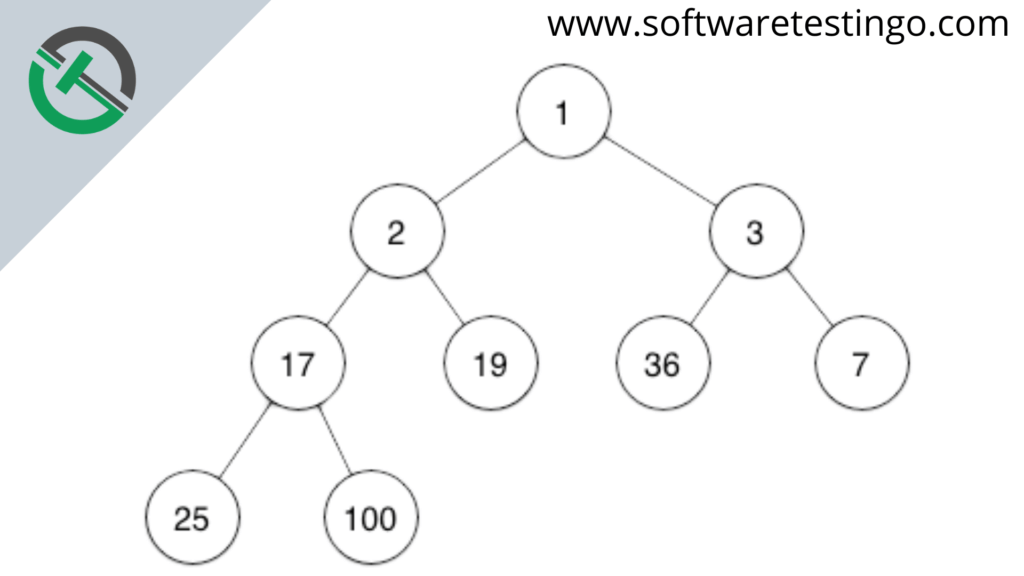
It’s important to note that priority queues (binary heaps) don’t necessarily store elements in absolute sorted order. This is for efficiency in terms of speed of insertion and retrieval. I’ll show this with an example by iterating through a priority queue.
Creating PriorityQueue
To create a priority queue in Java, we must import the java.util.PriorityQueue package. Once we have imported the package, we can create a priority queue by following these steps:
PriorityQueue<Integer> numbers = new PriorityQueue<>();
Methods Of PriorityQueue:
The Priority Queue class provides a way to manage data so that the most important items are always processed first. Let’s look at how to perform some of the most common operations on this type of data structure.
Adding Elements:
To add an element to a priority queue, we can use the add() method. The elements in the queue are stored according to their priority, with lower priorities being stored first by default.
package com.SoftwareTestingO.collections; import java.util.PriorityQueue; public class PriorityQueueAdd { public static void main(String[] args) { PriorityQueue<String> pqueue = new PriorityQueue<>(); pqueue.add("Software"); pqueue.add("Testingo"); pqueue.add("Blog"); System.out.println(pqueue); } }
Removing Elements:
You can remove an element from a priority queue using the remove() method. If there are multiple objects you want to remove, then the first occurrence of the object will be removed. You can also use the poll() method to remove and return the head.
package com.SoftwareTestingO.collections; import java.util.PriorityQueue; public class PriorityQueueRemove { public static void main(String[] args) { PriorityQueue<String> pqueue = new PriorityQueue<>(); pqueue.add("Software"); pqueue.add("Testingo"); pqueue.add("Blog"); System.out.println("Initial PriorityQueue "+pqueue); //Remove Element pqueue.remove("Blog"); System.out.println("After Remove - " + pqueue); System.out.println("Poll Method - " + pqueue.poll()); System.out.println("Final PriorityQueue - " + pqueue); } }
The sequence of items in the priority queue is not always sorted, but when we retrieved the items, they were always retrieved in sorted order.
Accessing the elements:
Since a queue follows the First In First Out principle, we can only access the head of the queue. We can use the peek() method to access elements from a priority queue.
package com.SoftwareTestingO.collections; import java.util.PriorityQueue; public class PriorityQueueAccess { public static void main(String[] args) { PriorityQueue<String> pqueue = new PriorityQueue<>(); pqueue.add("Software"); pqueue.add("Testingo"); pqueue.add("Blog"); System.out.println(pqueue); //Accessing The elements String element=pqueue.peek(); System.out.println("Accessed Element: " + element); } }
Iterating the PriorityQueue:
There are multiple ways to traverse through the PriorityQueue. The most famous way is converting the queue to an array and using a for loop. However, there is also an inbuilt iterator that can be used.
package com.SoftwareTestingO.collections; import java.util.Iterator; import java.util.PriorityQueue; public class PriorityQueueIterating { public static void main(String[] args) { PriorityQueue<String> pqueue = new PriorityQueue<>(); pqueue.add("Software"); pqueue.add("Testingo"); pqueue.add("Blog"); System.out.println(pqueue); //Iterating The elements Iterator iterator = pqueue.iterator(); while (iterator.hasNext()) { System.out.print(iterator.next() + " "); } } }
Conclusion:
In this Java queue tutorial, we learned how to use the PriorityQueue class, which can store elements either by default in a natural ordering or you can specify a custom ordering using a comparator.