Polymorphism In Java: Welcome to another brand new post on the Core Java tutorial Series; in our last post, we discussed the importance and how to use the method overriding & method hiding in Java. In this post, we will discuss another important feature of the Java OOPS concept: polymorphism.
Polymorphism allows us to perform a single action but in different ways. Polymorphism comes from a Greek language and means “Many Forms.” In simple words, we can say that this is the ability of Java programming language by which “we can create functions or reference variables which behave differently in a different programming context.”
What Is Polymorphism?
The word Polymorphism comes from two Greek words – poly and morphs. “Poly” means many, and “Morphs” means shapes or forms. So, polymorphism means having many forms. In Object-Oriented Programming, polymorphism is an important feature that allows objects to take on different forms or behaviors.
In Java, polymorphism means an object can act differently or have different forms depending on its use. This is made possible by using two techniques: inheritance and interfaces.
Inheritance allows a subclass to inherit the properties and methods of its superclass while also allowing the subclass to override or extend the behavior of those methods. This allows the subclass to behave differently from its superclass while still being treated as an instance of the superclass.
Interfaces, however, allow multiple classes to implement a common set of methods, which can be used interchangeably. This allows for greater flexibility and reusability in code design, as any class that implements an interface can be used instead of any other class that implements the same interface.
I hope you have got an idea about these important topics. Now, let’s move further and understand the different types of polymorphism in Java with examples.
Types of Polymorphism in Java
Java programming language supports two types of polymorphism, and that is:
- Static Polymorphism (Compile-time)
- Dynamic Polymorphism (Runtime)
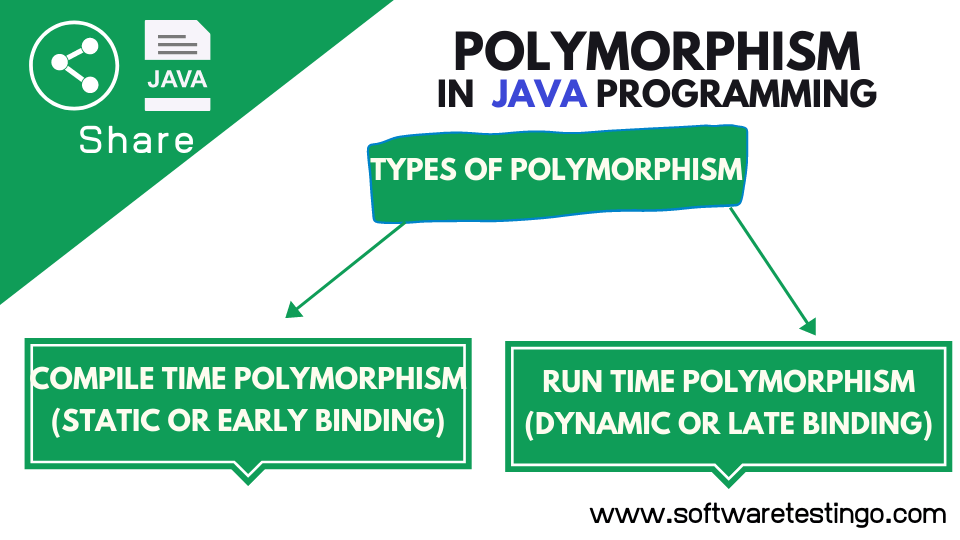
Compile Time Or Static Polymorphism
In this type of polymorphism, the method call is resolved at compile-time based on the number, types, and order of the arguments passed to the method. Method overloading is an example of compile-time polymorphism.
Method Overloading: This allows us to have multiple methods with the same name but different parameter lists where the sequence and data types of parameters are different. For more information, refer to our Method Overloading post.
Static Polymorphism Example: Method overloading is one of the ways Java supports static polymorphism.
package com.SoftwareTestingo.JavaBasics; class PolymorphismEx { public void displayString(String str) { System.out.println("Single Parameterized Display Method Executed"); } public void displayString(String str, String str1) { System.out.println("Double Parameterized Display Method Got Executed"); } } public class Static_Polymorphism { public static void main(String[] args) { ParentClassEx obj=new ParentClassEx(); obj.displayString("Welcome"); // Will Call Single Parameterized Method obj.displayString("WELCOME", "USER");// Will Call Double Parameterized Method } }
Output:
Single Parameterized Display Method Executed Double Parameterized Display Method Got Executed
Dynamic or Runtime Polymorphism
In this type of polymorphism, the method call is resolved at runtime based on the actual type of the object on which the method is called. An example of runtime polymorphism is method overriding, where a subclass can implement a method defined in its superclass. We have a full post where we discuss method overriding in Java.
package com.SoftwareTestingo.JavaBasics; class Vehicle { public void speed() { System.out.println("Speed Method of Vehicle Class Executed"); } } class Bike extends Vehicle { public void speed() { System.out.println("Speed Method Of Bike Class Executed"); } } public class MethodDispatch extends Vehicle { public static void main(String[] args) { Vehicle obj=new Vehicle(); Vehicle obj1=new Bike(); obj.speed(); obj1.speed(); } }
Output:
Speed Method of Vehicle Class Executed Speed Method Of Bike Class Executed
When a user wants to call an overridden method by using a parent class reference, then the object type determines which method will execute determined at run time. Since both classes have the same method at that time, JVM determines which method will be called at runtime.
Ref – article