One-Dimensional Array In Java: In Java, when you want to store the collection of values of the same data type in a contiguous memory location, you should go with the one-dimensional array or single-dimensional array in Java. These arrays provide a convenient way to work with a series of elements, such as integers, floating-point numbers, or objects.
Some other terms are also used for the one-dimensional array, like a single or 1-dimensional array in Java. So, if you come across such a name, there is no need to panic about this.
Before we jump to the detailed one-dimensional array, if you are unaware of what an array is, we suggest you follow the hyperlink where we have discussed the array in detail.
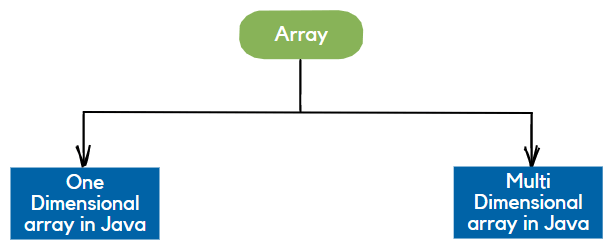
What is a Single or One Dimensional Array?
A One-Dimensional Array in Java is a linear data structure that stores a collection of elements of the same data type in a single row or line. It can be thought of as a sequence of values, all stored under a single variable name.
Single-dimensional arrays in Java have a fixed size, which means you need to specify the number of elements it can hold when you declare it. Once the size is defined, it cannot be changed during the program’s execution.
If you want to access an array element, you can access it by its position or index, starting from 0 for the first element, 1 for the second, and so on.
These arrays are particularly useful when you need to work with a list of items of the same type, such as storing a list of integers, characters, or objects.
One Dimensional Array In Java Syntax
The syntax for declaring and initializing a one-dimensional array in Java is as follows:
data_type[] array_name = new data_type[size];
Let’s break down the components of this syntax:
- data_type: This represents the data type of the elements you want to store in the array. It can be any valid data type in Java, such as int, double, String, or a custom class type.
- array_name: This is the name you give to your array. It follows the usual Java naming conventions for variable names.
- New data_type[size]: This syntax part creates a new array object and specifies its size. You use the new keyword followed by the data type and square brackets to create the array. Inside the square brackets, you specify the number of elements the array can hold, known as its size. For example, if you want to create an array that can hold 5 integers, you would write a new int[5].
Here’s an example of declaring and initializing a one-dimensional array of integers:
int[] myArray = new int[5];
In this example, myArray is the name of the integer array, capable of holding 5 integer values. You can then access and manipulate the elements in myArray using their indices, ranging from 0 to 4 in this case, since arrays in Java are 0-based.
One Dimensional Array In Java Example Program
Here’s a simple example program in Java that demonstrates the creation and usage of a 1D array to store and manipulate a list of integers:
Example 1:
public class OneDimensionalArrayEx { public static void main(String[] args) { // Declare and initialize an array of integers int[] myArray = new int[5]; // Populate the array with values myArray[0] = 10; myArray[1] = 20; myArray[2] = 30; myArray[3] = 40; myArray[4] = 50; // Access and print the elements of the array System.out.println("Elements of the array:"); for (int i = 0; i < myArray.length; i++) { System.out.println("Element at index " + i + ": " + myArray[i]); } } }
In this program:
- We declare and initialize an integer array, myArray, with a size of 5. This array can store 5 integer values.
- We populate the array with values using the array indices, starting from 0 and going up to 4.
- We then use a for loop to iterate through the elements of the array and print their values along with their indices.
When you run this program, it will output:
Elements of the array: Element at index 0: 10 Element at index 1: 20 Element at index 2: 30 Element at index 3: 40 Element at index 4: 50
This demonstrates the basic usage of a one-dimensional array in Java for storing and accessing elements.
Advantages Of Single Dimensional Array
Here are some key benefits of using a 1D array:
- Structured Data Organization: One-dimensional arrays provide an organized structure for storing and managing elements of the same data type. This structured approach simplifies data access and manipulation.
- Memory Efficiency: They allocate memory efficiently by requiring contiguous storage for elements of uniform data types, reducing memory overhead.
- Random Access: One-dimensional arrays enable direct access to elements through their indices, ensuring quick and predictable retrieval.
Challenges and Considerations While Using Single-Dimensional Array
- Fixed Size: One-dimensional arrays have a predetermined size set during declaration, making them less flexible when dealing with dynamic data.
- Initialization Complexity: Initializing arrays, especially with many elements, can be complex and error-prone.
- Data Type Uniformity: All elements within a one-dimensional array must be the same data type, which may not suit all programming scenarios.
Key Points
- In Java, a single-dimensional array is a linear data structure for storing values of the same data type.
- It stores elements sequentially in memory.
- It can hold simple data types like integers booleans or complex data types like custom classes.
- Elements are stored in a linear list in memory.
- Indexing starts at 0 and goes up to size-1.
- Initialization can be done with values separated by commas or specifying a static size.
- Single-dimensional arrays are a useful tool for efficient data organization.
- They provide easy access to data through loop structures.
Conclusion
Understanding one-dimensional arrays in Java is fundamental to harnessing the full power of the language. These arrays provide a structured way to store and manipulate collections of elements, making data management more efficient and organized. With the ability to store elements of the same data type in a contiguous memory location and easy access through indices, one-dimensional arrays are a crucial concept for Java developers.
As we continue to explore the world of Java programming, there is always room for improvement and learning. We encourage our readers to actively engage with this topic and offer their valuable suggestions to enhance our content. Your input can help us provide even more comprehensive and informative resources for the Java community.
Furthermore, we encourage you to share this article with others who may benefit from it. Collectively, we contribute to a more informed and skilled community of Java programmers by spreading knowledge and education. Together, we can advance our skills and understanding of Java’s capabilities, making us more proficient developers.