Object In Java: Java is one of the most popular programming languages in the world. It mainly consists of Classes and Objects. We have already discussed Classes in our previous blog post. If you have not checked that, you can check this Class In Java link, but now we will try to understand the object in Java.
In this Java object blog post, we will discuss What an object is and how to store Java objects. We will also learn different ways to create objects in Java.
What Is An Object In Java Or Object In Java Definition?
Objects are the heart and soul of the Java programming language. In Java, everything is treated as an object, and this fundamental concept lies at the core of the language’s design. So, what exactly is an object in Java?
An object is a self-contained unit representing a real-world entity, concept, or data structure. It encapsulates both data (known as attributes or fields) and behavior (defined by methods). Objects are instances or member of classes that serve as blueprints for their creation. Each object has a unique identity, state, and the ability to perform actions (behavior).
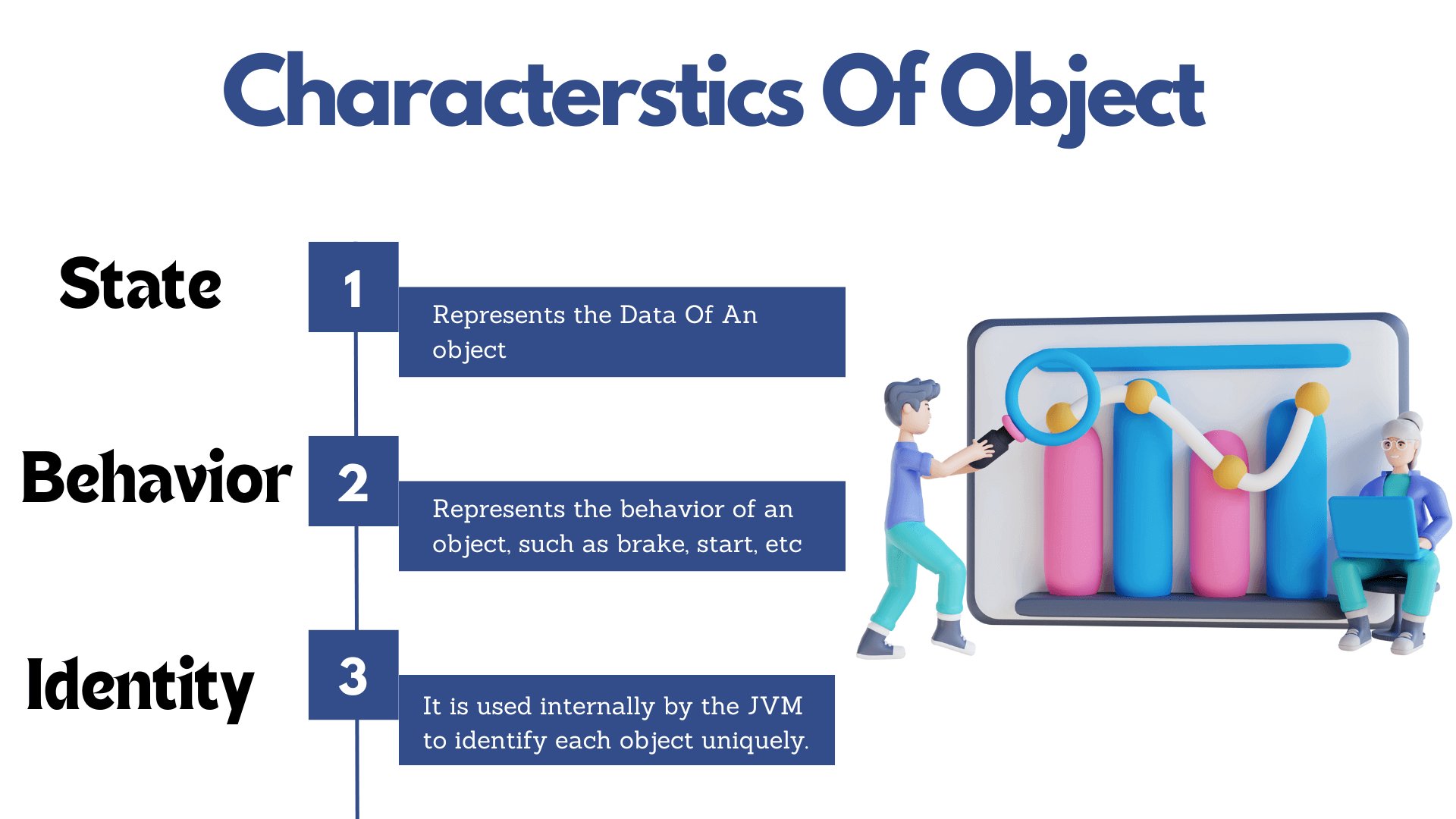
Characteristics Of Objects
The three fundamental characteristics of objects in Java are state, behavior, and identity. These characteristics are central to understanding how objects work in Java.
State
- Definition: The state of an object represents the data or attributes that define the object’s properties or characteristics. It’s the collection of values stored in the object’s instance variables.
- Significance: The state of an object makes it unique and distinguishes it from other objects of the same class. It defines the object’s current condition or configuration.
- Example: If you have an object representing a “Car,” the state of that object may include attributes like “color,” “make,” “model,” and “speed.”
Behavior
- Definition: Behavior refers to the actions or operations that an object can perform. In Java, behavior is defined by the methods associated with the object. Methods are functions that define how the object can interact with and manipulate its state.
- Significance: Behavior allows objects to perform meaningful actions. It encapsulates an object’s functionality, enabling it to respond to requests and perform specific tasks.
- Example: Continuing with the “Car” object, behavior can include methods like “start,” “accelerate,” “brake,” and “turn.” These methods determine how the car can be operated.
Identity
- Definition: Identity represents the unique identity or individuality of an object. In Java, every object has a distinct identity that differentiates it from all other objects, even if they have the same state.
- Significance: Identity ensures that each object is treated as a separate entity. It’s crucial for distinguishing between different objects, especially when dealing with collections of objects.
- Example: If you have two cars with the same make, model, and color, they are still distinct objects with different identities.
The state of an object is maintained through its instance variables, which are like containers for data. These variables store the specific characteristics or properties of the object. Meanwhile, the behavior of an object is defined by methods, which are functions associated with the object. These methods allow objects to perform actions or operations.
How Do You Create An Object In Java?
There are multiple ways to create an object in Java, but some are common and easy ways to create an object. We can create objects in the following ways:
We will discuss these methods for creating objects in our dedicated post. If we discuss this here, then this post becomes very long. So follow the hyperlink to learn more about how to create objects.
Let us take a simple example to understand how to create an object in Java.
class employee { int empID=1001; String empName="SoftwareTestingo"; } public class CreateObjectEx { public static void main(String[] args) { employee obj=new employee(); System.out.println("Employee ID: "+obj.empID); System.out.println("Employee Name: "+obj.empName); } }
Output:
Employee ID: 1001 Employee Name: SoftwareTestingo
Different ways to Ways to Initialize Object
Above, we have learned how to create an object, but after creating the object, we need to initialize (Storing values or data into) the object; there are several ways to initialize objects. Here are some of the most common methods:
Let us try to understand the above methods one by one.
Initialization By Reference Variable
This way, we will initialize the object using the reference variable.
class employee { int empID; String empName; } public class CreateObjectEx { public static void main(String[] args) { employee obj=new employee(); obj.empID=1001; obj.empName="SoftwareTestingo"; //Printing the values System.out.println("empID"+" "+"empName"); System.out.println("----------------------------"); System.out.println(obj.empID+ " "+obj.empName); } }
Output:
empID empName -------------------------- 1001 SoftwareTestingo
Initialization By Method
In the example below, we will understand how we can initialize the object’s value by calling a method.
class employee { int empID; String empName; // initializing The Values void updateValues(int id, String name) { empID=id; empName=name; } // Displaying the values void displayValues() { System.out.println("empID"+" "+"empName"); System.out.println("--------------"); System.out.println(empID+" "+empName); } } public class CreateObjectEx { public static void main(String[] args) { employee obj=new employee(); obj.updateValues(101, "Ramesh"); obj.displayValues(); } }
Output:
empID empName -------------- 101 Ramesh
When you create an object, we inform you that the objects are created in the heap memory, and the reference variable will be created in the stack memory area.
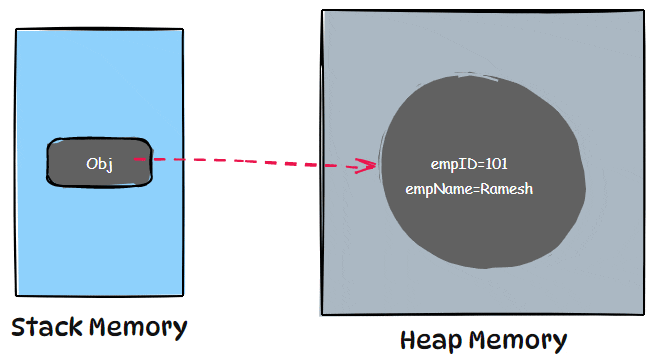
Initialization Through By Constructor
In this section, we will try to learn how to initialize objects using the constructors.
class employee { int empID; String empName; // initializing The Values employee(int id, String name) { empID=id; empName=name; } public int getEmpID() { return empID; } public String getEmpName() { return empName; } } public class CreateObjectEx { public static void main(String[] args) { employee obj=new employee(101, "Suresh"); System.out.println("Employee ID: "+obj.getEmpID()); System.out.println("Employee Name: "+obj.getEmpName()); } }
Output:
Employee ID: 101 Employee Name: Suresh
Anonymous Object
An anonymous object in Java refers to an instance of a class that does not have a name. These objects are typically created for a single-use scenario and are not stored in a reference variable. Instead, they are created and used on the fly.
Here’s an example in Java code to illustrate the concept of an anonymous object:
public class CreateObjectEx { void display(String msg) { System.out.println("Message: "+msg); } public static void main(String[] args) { new CreateObjectEx().display("Welcome to SoftwareTestingO"); } }
In this example, we have anonymous objects of the CreateObjectEx class created within the main method. These objects call the display method directly without assigning them to any variables. This is useful when performing a one-time operation without reusing the object.
Anonymous objects are typically used for short-lived operations and are not accessible once the execution of the method or block in which they are created is completed.
How to Multiple Objects of the Same Type?
We have already seen that in a single statement or time, we are creating multiple variables of the same primitive type like below:
int age=23, rollno=21;
By Following the same process, we can Initialization objects or reference variables like below:
// Creating Two Objects Of Same Type Employee emp1=new Employee(), emp2=new Employee();
Let’s See an example:
public class Employee { void display(String msg) { System.out.println("Message: "+msg); } public static void main(String[] args) { // Creating Two Objects Of Same Type Employee emp1=new Employee(), emp2=new Employee(); //Calling the Display method emp1.display("Hi Ramesh"); emp2.display("Hi Suresh"); } }
Output:
Message: Hi Ramesh Message: Hi Suresh
Conclusion:
If you want to build your career as a Java developer, then a thorough understanding of objects in Java is essential. We’ve explored the core concepts of objects, including state, behavior, and identity, and how these characteristics define the essence of objects in Java.
As you explore Java more, remember that objects are your program’s building blocks, allowing you to model real-world entities and create organized, reusable, and efficient code. The principles of encapsulation, inheritance, and polymorphism further enhance the power and flexibility of objects in Java.
If you have any doubts or questions regarding “Object In Java,” we encourage you to reach out and ask. Your understanding is our priority, and we’re here to assist you in clarifying any concepts or addressing any queries you may have.
Moreover, your feedback is invaluable to us. If you have suggestions on how we can improve this article or topics you’d like to see covered in more detail, please provide your insights in the comment section below. Your input helps us tailor our content to meet your needs better as you continue your Java programming journey.