Method Overriding In Java: Method Overriding is an important concept in Java, allowing a subclass to implement a method already defined in its parent class. This means that when an object of a subclass is created, its method will be executed instead of the method defined in the parent class.
Method Overriding is a key feature of object-oriented programming, which allows for polymorphism, where objects of different classes can be treated as objects of the same class. In this way, Method Overriding provides flexibility and extensibility to Java programs, allowing for more customized and specialized behaviors in different parts of the program.
What is Method Overriding?
Declaring a similar method in the subclass, already present in the parent class, is known as method overriding. We are using method overriding because it allows a subclass or child class to provide a specific implementation of a method already provided by one of its super-classes or parent classes. That’s why the parent class method is called the overridden method, and the child class method is called the overriding method.
To override a method in Java, the subclass must use the same method signature as the method it wants to override. The method signature consists of the method’s name, number, and types of its parameters. The return type of the overriding method can be the same or a subclass of the return type of the overridden method.
When a method is called on an object, the JVM determines which implementation of the method to use at runtime. If the method is overridden in the subclass, the JVM will use the implementation provided by the subclass. If the method is not overridden in the subclass, the JVM will use the implementation provided by the parent class.
Let’s take an example to understand the method overriding in Java easily. Suppose there is a parent class called an animal, and the animal class has some methods like running, walking, eating, and sleeping with the implementation. There is a subclass called human, which extends the parent animal class, but the way humans eat, walk, and run are all different from the animal. So, in the human class, you want to give the implementation for human methods like running, walking, eating, and sleeping.
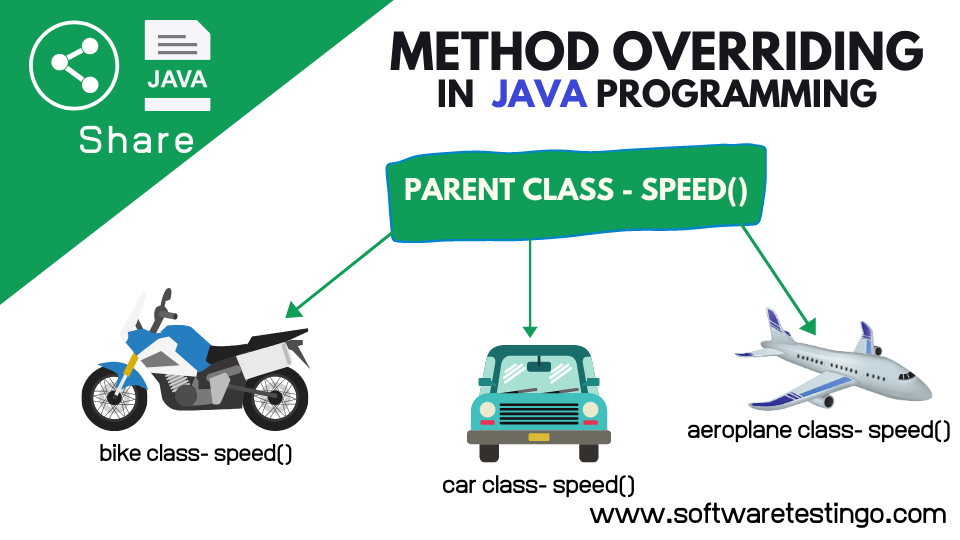
So, the user wants to do method overriding because he wants to give his implementation in the child class. So that when the user calls the child class methods, it operates like a human.
package com.SoftwareTestingo.JavaBasics; class MethodOverridingEx1 { public void speed() { System.out.println("Parent Speed method Executed"); } } public class MethodOverriding extends MethodOverloadingEx1 { //This Method Becomes Override the Parent Method public void speed() { System.out.println("Child Speed Method executed"); } public static void main(String[] args) { MethodOverriding obj=new MethodOverriding(); obj.speed(); } }
Output:
Child Speed Method executed
Advantages of Method overriding
One of the significant benefits of method overriding is that when a user wants to give his specific implementation to the inherited method without changing the parent class methods.
Method overriding is helpful when there are several child classes, and one parent class is there, and all extend the parent class and use the parent class methods. So those classes that want to use the parent class methods as it is they can use those, and those that want different implementations as per their requirement can implement them in the child class so that others using the same method are not affected by these changes.
Method overriding is an example of runtime polymorphism when a parent class object references the child class object. If you want to call, an overridden method is determined during runtime. Because when you call a method, which method (parent or child class method) is going to call is determined according to the type of the object. So, the process in which a call to an overridden method is resolved, and the process is called dynamic method dispatch.
package com.SoftwareTestingo.JavaBasics; class Vehicle { public void speed() { System.out.println("Speed Method of Vehicle Class Executed"); } } class Bike extends Vehicle { public void speed() { System.out.println("Speed Method Of Bike Class Executed"); } } public class MethodDispatch extends Vehicle { public static void main(String[] args) { Vehicle obj=new Vehicle(); Vehicle obj1=new Bike(); obj.speed(); obj1.speed(); } }
Output:
Speed Method of Vehicle Class Executed Speed Method Of Bike Class Executed
Note: When the parent class reference refers to the child class object, the child class method is executed, called dynamic method dispatch or runtime polymorphism.
Rules Of Method Overriding
- Argument list: The data type of arguments and parent and child classes sequence should match exactly.
- Overriding and Access-Modifiers: The scope of the child class method (Overriding method) can be increased, but you can not decrease the existing scope of parent class methods (Overridden method). Suppose the scope of a method in the parent class is protected; then, in the subclass, you can change the scope to the public, but you can’t change the access modifiers to private. The Java JVM compiler generates a compile-time error if you change the access modifiers to private.
package com.SoftwareTestingo.JavaBasics; class ParentCL { public void display() { System.out.println("Parent Display Method Executed"); } } class ChildCL extends ParentCL { private void display() { System.out.println("Child Display Method Executed"); } } public class MethodOverriding_Scope { public static void main(String[] args) { // TODO Auto-generated method stub } }
In the above example, you can see in the child class that we are trying to decrease the method’s scope from public to private. That’s why we are getting the below error:
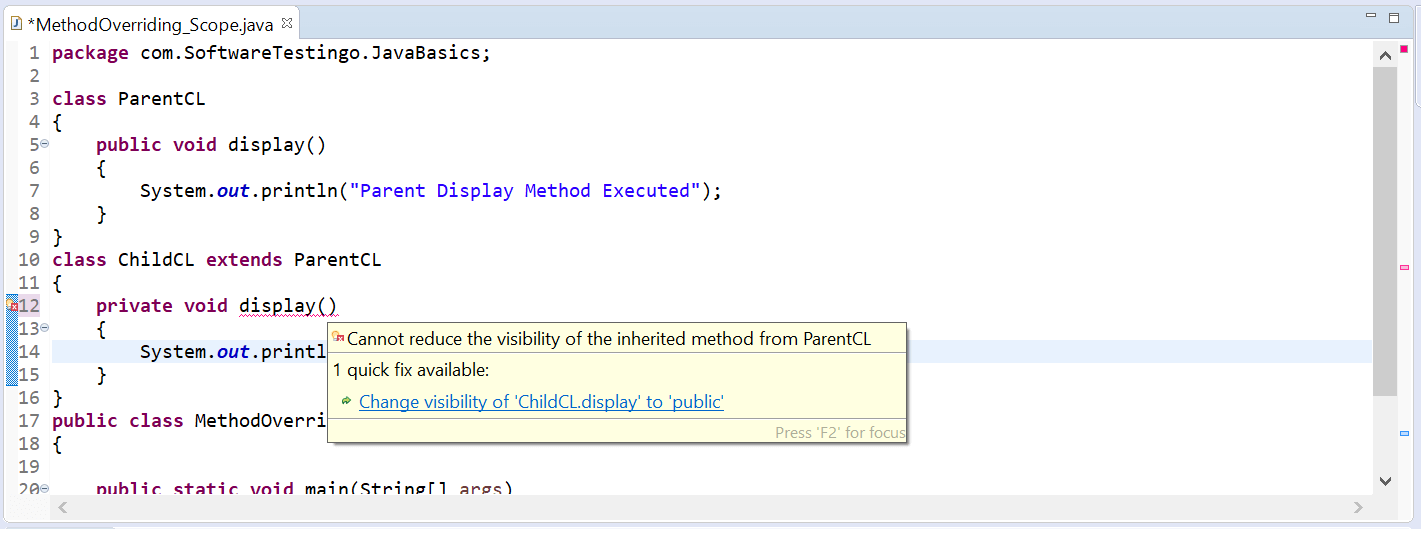
- Final Methods cannot be overridden: If you don’t want other classes to override your method, then you can declare the method with the final keyword so that no one can override your method. If anyone tries to override, then he will get an error.
- A static method can not be overridden (Method Hiding): When you define a static method in the base class and with the same method signature, if you declare another static method with the same as the base class signature in the child class, that is called method hiding in Java.
package com.SoftwareTestingo.JavaBasics; class bus { public static void driver() { System.out.println("Driver Method Of Bus Class Executed"); } public void conductor() { System.out.println("Conductor Method Of Bus Class Got Executed"); } } class Volvobus extends bus { //This Method Hides public static void driver() { System.out.println("Driver Method Of Volvobus Class Executed"); } public void conductor() { System.out.println("Conductor Method Of Volvobus Class Got Executed"); } } public class Method_Hiding { public static void main(String[] args) { bus pobj=new bus(); bus cobj=new Volvobus(); System.out.println("+++++++++ Method Hiding +++++++++"); System.out.println(); pobj.driver(); //This will call the parent method cobj.driver(); //This will call the parent method System.out.println(); System.out.println("+++++++++ Method Overriding +++++++++"); System.out.println(); pobj.conductor(); //This will call the parent method cobj.conductor(); //This will call the child method } }
Output:
+++++++++ Method Hiding +++++++++ Driver Method Of Bus Class Executed Driver Method Of Bus Class Executed +++++++++ Method Overriding +++++++++ Conductor Method Of Bus Class Got Executed Conductor Method Of Volvobus Class Got Executed
- Private methods can not be overridden: Private methods can not be overridden because the scope of the private methods is within the class, so outside of the class, they cannot extend.
- Invoking Parent Class method from child class: We Can call the parent class method from the child class using the super keyword.
- Overriding and constructor: We Can not override the constructor in the child class because the parent class constructor is never extended in the child class. Per the constructor rule, the constructor name should be the same as the class name. But from the child class, we can call the parent class constructor by using the super keyword.
- Abstract Method: If a class is extending an abstract class or implementing an interface, all methods should be implemented in the child class; otherwise, you will get a compile-time error.
Conclusion:
Method Overriding is a powerful feature of Java that allows subclasses to implement a method defined in the parent class. It is used to implement runtime polymorphism, where objects of different classes can be treated as objects of the same class. Method Overriding is an essential tool for designing flexible and extensible object-oriented software.
We believe discussion and debate are the best ways to learn and grow. If you have a different perspective or opinion than what we shared in our post, please share it in the comments below.