Method Overloading In Java: Method overloading is a feature in Java that allows a class to have multiple methods with the same name but different parameters. In other words, method overloading allows multiple methods in a class to share the same name as long as their parameters differ in type, number, or order.
This feature is a part of polymorphism, which is one of the fundamental concepts in object-oriented programming. With the help of method overloading, we can write more concise and flexible code, making it more readable and easier to maintain. It is widely used in Java libraries, frameworks, and applications to create methods that perform similar tasks but with different data types or numbers of arguments.
This concept enables programmers to reuse code and reduces the effort required to implement new features, leading to more efficient and scalable code. This article will explore the basics of method overloading in Java, how it works, and its benefits.
Method Overloading in Java
If two or more methods have the same name but different parameters or arguments, then such methods are called overloaded methods, and that feature is called Method Overloading. It is similar to constructor overloading because a class has multiple constructors, but all constructor parameters differ.
Method overloading is a compile-time polymorphism. This means that the compiler determines which method to call based on the method name, number, and type of parameters. When a method is called, the compiler checks for a method with the same name in the class and then checks if the arguments match the parameter types of any of the methods with that name.
If there is an exact match, the corresponding method is called. If there is no exact match, the compiler tries to find a method that can be called by performing automatic type conversions or promotions. If multiple methods that match the arguments are found, the compiler selects the one with the most specific type.
Example Of Method Overloading
void test() {..} int test(int a) {..} float test(float b) {..} void test(int a, float b) {..}
All the method names are the same in the above code, but the parameters differ. So, we can say that the test() method is overloaded.
Note: If you have noticed the above methods, then you can find that all the method’s return type is not the same; that means in overloaded methods, the method name is the same, but they may be different in parameter and return type.
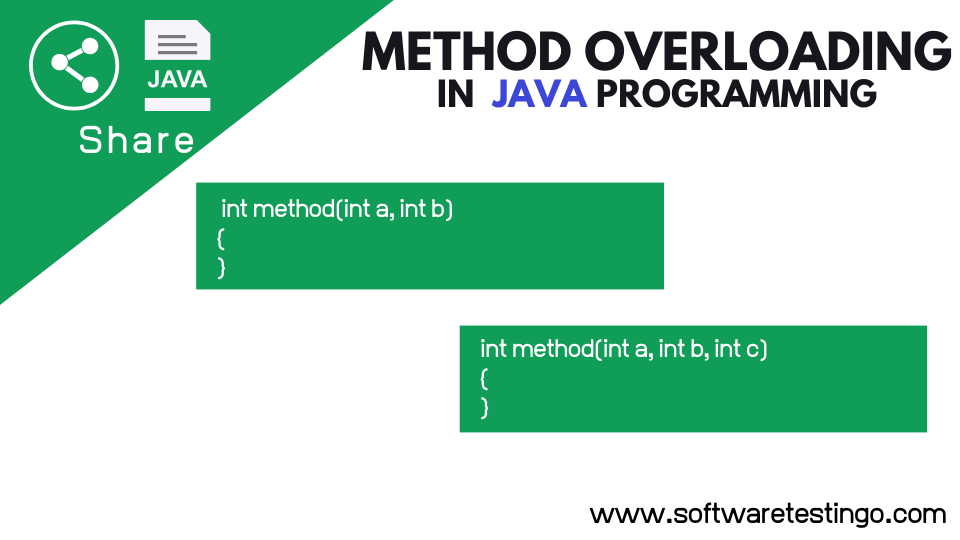
Why Method Overloading?
Let’s take a simple example to understand the requirement of method overloading. As you know, to operate a TV, we require a remote, but for the different company TVs, there is a chance that the remote may look different, but they are all used for the same purpose: to operate the TV.
If you think about the same thing in a programming language, then the operation performed by a Remote is the same, but the Remote looks different. If, for each company remote, you will create a separate class and methods, then their duplicate methods may be created for the same operation.
In the above case, we can accomplish the same thing better by overloading methods, and depending on the argument, the specific overloaded methods are called. This also helps increase the program’s readability and re-usability of code.
Different Ways to overloaded a Method
We Can Overload the methods in the following ways:
- The number of parameter differences: If two methods have the same name but different parameters, then that’s a valid case of method overloading.
void test(int); void test(int, int);
- Parameter Data Type Should be Different: If the number of parameters is the same, but the data type is not the same, then it’s also a valid example of Method overloading.
void test(int,int); void test(float, int);
- Sequent of Data type is different: If Two methods have the same name and number of the argument, but the argument’s data type order is different, that’s also a valid case of method overloading.
void test(int,float); void test(float, int);
- InValid Case of Method Overloading: When Two or More Methods have the same name and number of the argument but different method return types, that’s not a valid example of method overloading; in this case, you will get a compile-time error.
int test(int, int); float test(int, int);
Note: Method overloading is an example of Static polymorphism—more details of polymorphism are discussed here.
package com.SoftwareTestingo.JavaBasics; class ParentClassEx { public void displayString(String str) { System.out.println("Single Parameterized Display Method Executed"); } public void displayString(String str, String str1) { System.out.println("Double Parameterized Display Method Got Executed"); } } public class MethodOverloadingEx1 { public static void main(String[] args) { PolymorphismEx obj=new PolymorphismEx(); obj.displayString("Welcome"); // Will Call Single Parameterized Method obj.displayString("WELCOME", "USER");// Will Call Double Parameterized Method } }
Output:
Single Parameterized Display Method Executed Double Parameterized Display Method Got Executed
Static Polymorphism or Method Overloading is also called compile-time binding or early binding because the binding of methods happens at compile time.
Write a program to find the main method of overloading Java—an example program.
package com.java.Softwaretestingblog; public class MainMethodOverloading { public static void main(int a) { System.out.println("Main Method Overloading Done"); } @SuppressWarnings("static-access") public static void main(String[] args) { System.out.println("Main method "); MainMethodOverloading obj=new MainMethodOverloading(); obj.main(20); } }
Output:
Enter A Year 2000 it is leap year
Conclusion:
method overloading is a powerful and flexible feature in Java that allows a class to have multiple methods with the same name but different parameters. It provides a way to write more concise, flexible, and readable code. It is widely used in Java libraries, frameworks, and applications to create methods that perform similar tasks but with different data types or numbers of arguments. Using method overloading, programmers can reuse code and reduce the effort required to implement new features, leading to more efficient and scalable code.
After going through this article, I hope you can understand the method overloading and how we can use this in our script. If you still have any doubts, you can ask us by commenting in the comment section.
Ref: article