Literals in Java: Java is a versatile and widely-used programming language known for its portability, robustness, and readability. A fundamental concept in Java, which forms the building blocks of any program, is the concept of literals.
In this article, we will discuss what Java literals are, how they work, and explore the various types of literals in Java. We’ll demonstrate their usage through illustrative examples, helping you comprehensively understand this crucial aspect of Java programming.
What is a Literal in Java Or Definition Literal?
In Java, a literal is a constant value explicitly defined in a program’s source code. These values remain unchanged during the program’s execution. Literals represent data that does not need further computation or processing and are often assigned to variables for various operations.
Literals are vital in Java programming, providing unambiguous data representation. They are used to initialize variables, define constants, specify values for method arguments, and much more.
How Literals Work in Java
Literals in Java are recognized by the compiler and are assigned the appropriate data type based on their format and value. Java supports various types of literals; here is the list below:
Let’s explore each of these in detail.
Different Types of Literals in Java
Literals of Java are divided into the following types:
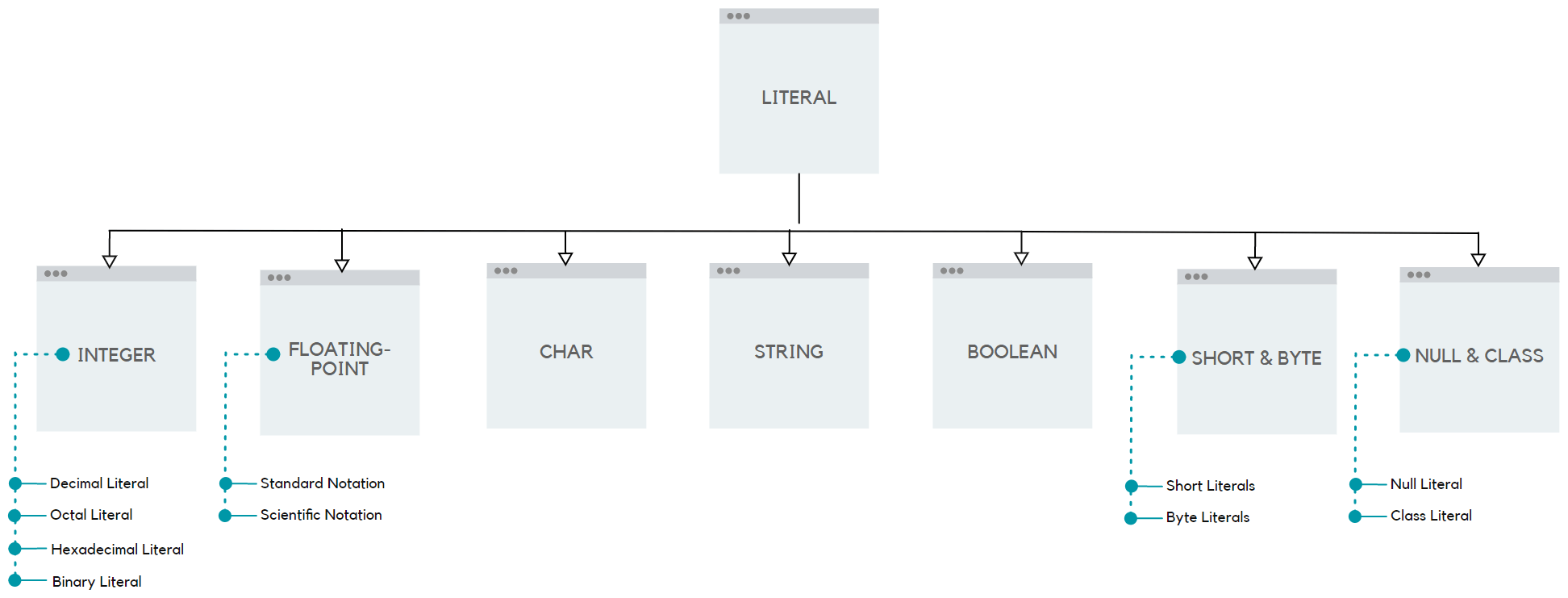
Integer Literals
Integer literals represent whole numbers and can be further categorized into decimal, octal, hexadecimal, and binary literals. Integral literals assign fixed integer values to variables or as operands in mathematical operations. In Java, you can declare integral literals with a suffix to specify their data type (e.g., int or long).
These literals play a fundamental role in many programming tasks, from simple arithmetic calculations to controlling program flow and defining constants. They provide a concise and explicit way to work with whole numbers in code.
Here are examples of each:
int decimalLiteral = 42; // Decimal Literal int octalLiteral = 052; // Octal Literal (starts with 0) int hexadecimalLiteral = 0x2A; // Hexadecimal Literal (starts with 0x) int binaryLiteral = 0b101010; // Binary Literal (starts with 0b)
Explain Integral Literals with Example Programs
Now, let’s explore integral literals in more depth with example programs.
Decimal Literal Example
int decimalLiteral = 42; System.out.println(decimalLiteral); // Output: 42
Octal Literal Example
int octalLiteral = 052; System.out.println(octalLiteral); // Output: 42 (Decimal equivalent of octal 052)
Hexadecimal Literal Example
int hexadecimalLiteral = 0x2A; System.out.println(hexadecimalLiteral); // Output: 42 (Decimal equivalent of hexadecimal 0x2A)
Binary Literal Example
int binaryLiteral = 0b101010; System.out.println(binaryLiteral); // Output: 42 (Decimal equivalent of binary 0b101010)
Floating-Point Literals
Floating-point literals are a means to represent real numbers with a fractional part. These literals are used to define constants or assign values to variables when working with non-integer numerical data, such as decimal numbers.
Floating-point literals can be written in standard notation (e.g., 3.14159) or scientific notation (e.g., 2.5e-3 to represent 0.0025). The precision of floating-point literals depends on the data type used (typically float or double in Java).
They are essential for mathematical calculations, scientific applications, and any scenario where decimal values must be accurately represented in code. However, due to the nature of floating-point representation, developers should be aware of potential precision and rounding issues when working with these literals.
double standardNotation = 3.14159; double scientificNotation = 2.5e-3; // 2.5 x 10^-3
Explain Floating-Point Literals with Example Programs
Let’s now explore floating-point literals with example programs.
Standard Notation Example
double standardNotation = 3.14159; System.out.println(standardNotation); // Output: 3.14159
Scientific Notation Example
double scientificNotation = 2.5e-3; System.out.println(scientificNotation); // Output: 0.0025
Char Literals
These literals are enclosed in single quotes (e.g., ‘A’) and can assign character values to variables or directly as operands in various operations. Character literals are essential for tasks involving individual characters, such as working with strings, defining characters for user input, and specifying characters for output or display.
They provide a clear and concise means to represent and manipulate characters in your program, making it more readable and intuitive. Like many programming languages, Java allows you to work with Unicode characters, enabling the handling of a wide range of characters from different writing systems and symbol sets.
char characterLiteral = 'A';
Explain Char Literals with Example Programs
Character literals are represented using single quotes. Here’s an example:
char characterLiteral = 'A'; System.out.println(characterLiteral); // Output: A
String Literals
These literals are enclosed in double quotes (e.g., “SoftwareTestingo”) and are commonly used to assign string values to variables or directly as operands in various string-related operations. String literals are fundamental for working with text and handling textual data in your program.
They are widely used for tasks such as storing and manipulating user input, displaying messages, and processing textual information from files and databases. String literals enhance code readability by providing a clear and convenient means to represent and manipulate strings of characters, making them an essential element of most software applications.
String greeting = "Java Tutorials";
Explain String Literals with Example Programs
String greeting = "Learn Java From SoftwareTestingo"; System.out.println(greeting); // Output: Learn Java From SoftwareTestingo
Boolean Literals
These literals are used to denote logical states or conditions within your code. Boolean literals play a fundamental role in decision-making and control flow, enabling you to create conditional statements and control structures like loops and branching.
They are used to express logical conditions, comparisons, and tests, making it possible to control the flow of a program based on whether a condition is true or false. Boolean literals are indispensable for implementing logic and enabling your program to make choices, making them a core element in programming for tasks like validation, decision-making, and algorithm design.
boolean isJavaFun = true;
Explain Boolean Literals with Example Programs
boolean isJavaFun = true; System.out.println(isJavaFun); // Output: true
Short and Byte Literals
These literals are typically assigned to variables or used directly in operations requiring short or byte data types.
- Short Literals: Short literals represent small integer values within the short data type. They are often defined without special notation and range from -32,768 to 32,767. For example, short temperature = 25; assigns the short literal 25 to the variable temperature.
- Byte Literals: Byte literals represent smaller integer values within the byte data type. They are typically defined without special notation and range from -128 to 127. For example, byte count = 10 assigns the byte literal 10 to the variable count.
Short and byte literals are particularly useful when memory efficiency is crucial or when working with data types that occupy less memory. They allow you to represent small integer values explicitly and efficiently within your code.
short shortLiteral = 1000; byte byteLiteral = 42;
Explain Short and Byte Literals with Example Programs
Short and byte literals represent values of their respective data types. Here are examples:
short shortLiteral = 1000; System.out.println(shortLiteral); // Output: 1000 byte byteLiteral = 42; System.out.println(byteLiteral); // Output: 42
Null and Class Literals
Null and class literals are special types of literals in Java used for specific purposes:
Null Literal:
- The null literal in Java represents the absence of a value or a null reference.
- It is often assigned to variables or used to check if an object or reference doesn’t point to any valid data.
- For example, String name = null assigns the null literal to the string variable name, indicating that it doesn’t currently reference any string object.
- Null literals are crucial for handling situations where an object may or may not exist, preventing potential runtime errors.
Class Literal:
- The class literal in Java refers to the class itself rather than an instance of that class.
- It is often used for tasks like reflection, to obtain information about a class, or to pass class references as arguments.
- Class literals are denoted using the .class notation. For example, Class<String> stringClass = String.class; assigns the class literal String.class to the stringClass variable.
- They play a significant role in dynamic code analysis and manipulation at runtime.
Both null and class literals have distinct purposes and are essential in various Java programming scenarios. Null literals handle the absence of data, while class literals facilitate interactions with class-level information and dynamic class loading.
The null literal is a special literal representing the absence of a value or a null reference. Class literals, on the other hand, represent the class itself and can be used with the .class notation:
String nullString = null; Class<String> stringClass = String.class;
Explain Null and Class Literals with Example Programs
The null literal represents the absence of a value or a null reference:
String nullString = null; System.out.println(nullString); // Output: null
Class literals represent the class and can be used for various purposes, such as reflection. Here’s an example:
Class<String> stringClass = String.class; System.out.println(stringClass.getName()); // Output: java.lang.String
Why Use Literals?
Literals in Java are used to represent fixed values directly in the source code. These values can be of various types, including integers, floating-point numbers, characters, and strings.
Here are some key reasons for using literals in Java programs:
- Readability: Literals make your code more human-readable. When you use literal values in your code, anyone reviewing your code can quickly understand the value’s meaning without referring to external documentation.
- Clarity: They provide clarity and eliminate ambiguity. Using literals clarifies what values you intend to use, reducing the chances of misunderstanding or errors.
- Consistency: Using literals ensures that the same constant value is consistently used throughout your program. If you need to change that value later, you only need to update it in one place.
- Ease of Maintenance: Maintenance becomes more manageable when constants are represented as literals. You can easily locate and update values that may change over time.
- Reduced Magic Numbers: Magic numbers (hard-coded numerical values without explanation) are discouraged in programming because they can be confusing. Replacing magic numbers with named literals provides context and makes the code more maintainable.
Here’s a simple example of how to use literals in Java:
public class LiteralExample { public static void main(String[] args) { int age = 25; double price = 19.99; char grade = 'A'; String message = "Welcome to Java!"; boolean isJavaFun = true; System.out.println("Age: " + age); System.out.println("Price: $" + price); System.out.println("Grade: " + grade); System.out.println("Message: " + message); System.out.println("Is Java fun? " + isJavaFun); } }
In this example, we’ve used literals to initialize variables of various data types. Using literals clarifies what values we intend to store in these variables.
Conclusion
Literals are the bedrock of programming, offering a straightforward way to represent constant values in your code. Understanding the various types of literals, from integral and floating-point to character, string, boolean, and class literals, is essential for effective Java programming. With this knowledge, you can confidently work with constants and constants-based operations in your Java applications, creating robust and reliable software.