This tutorial will explain how to concat strings in Java, including different methods and examples of concatenating strings. String concatenation refers to combining two or more strings into a single string. The term “append” denotes adding an extra string to an existing string variable.
There are various ways for concat string, so we will try to learn them individually with examples. This tutorial contains all the useful information you need to start working with strings and concatenating them.
Post On: | Java String concat() in Java |
Post Type: | Java Tutorials |
Published On: | www.softwaretestingo.com |
Applicable For: | Freshers & Experience |
Get Updates: | SoftwareTestingo Telegram Group |
Java String Concatenation meaning
A Java String is an object that contains a sequence of characters. Our previous blog post taught us that Strings are immutable, which means they can’t be changed after creation. However, you can append strings together by using Java concatenation methods.
Before deepening it, you must have a basic knowledge of strings to understand the concatenation process. You can learn more about Java strings from this article. This tutorial will cover multiple ways to concatenate strings using Java.
Different Ways to Concatenate String in Java
There are a few different ways you can concatenate strings in Java. The most common methods are listed below:
Using + Operator
The string that results from concatenation using the + operator will be a new String pointing to a fresh memory location in the Java heap. If there’s already a string object in that pool, it’ll return that same string object; otherwise, it creates a new one.
There are some important things to keep in mind when using the + operator for joining strings:
- The + operator should be avoided when concatenating strings in a loop, as it will create a lot of garbage.
- You should always store references to objects that are returned after concatenating strings using the + operator.
- You can convert an integer to a string in Java by using the + operator.
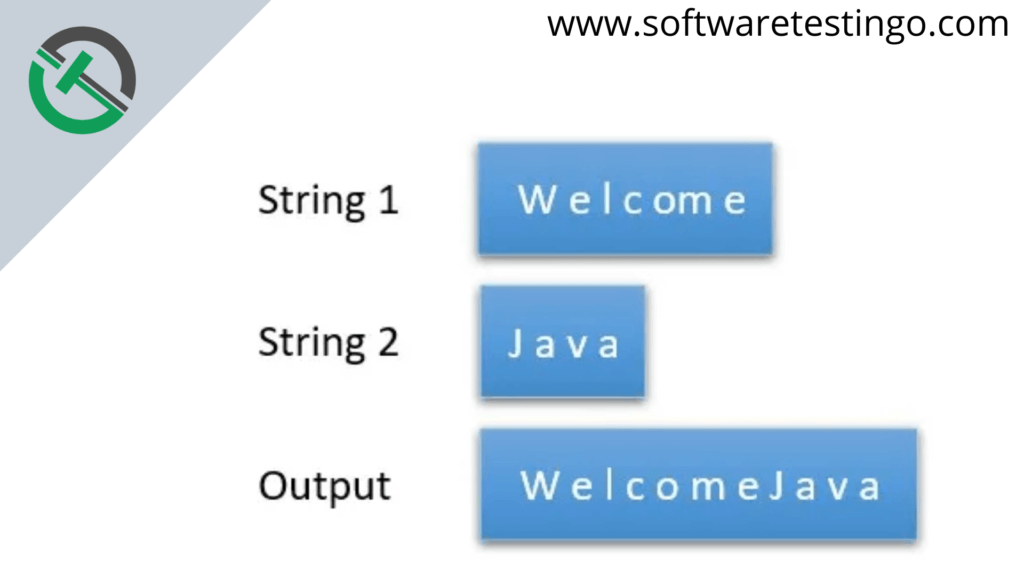
Program: Write a Program to Concat two strings using the + operator.
package com.SoftwareTestingO.Strings; public class StringConcatUsingoperator { public static void main(String[] args) { String s1 = "Welcome"; String s2 = "Java"; String s3 = s1 + s2; System.out.println("String 1: " + s1); System.out.println("String 2: " + s2); System.out.println("Concatenated string: " + s3); } }
Output:
String 1: Welcome String 2: Java Concatenated string: WelcomeJava
Using StringBuffer
This is a more efficient way to concatenate Java strings. Rather than using the + operator, which creates a new object of the resulting string, we can save memory space using StringBuffer.
When concatenating strings using a StringBuffer, initialize the string buffer object with a capacity equal to the number of characters in the final concatenated string. This will result in more efficient memory usage as well as saving time spent resizing characters.
One downside to this approach is that the append methods by StringBuffer are synchronized. However, it otherwise works similarly to the StringBuilder method. Additionally, it provides various overloaded append methods to concatenate characters, integers, shorts, etc.
The following is the simple syntax of the StringBuffer class.
StringBuffer NewStringBuffer = new StringBuffer(); NewStringBuffer.append(stringName);
Program: Write a Java Program to combine two strings using StringBuffer
package com.softwaretestingo.string; public class StringConcatUsingStringBuffer { public static void main(String[] args) { String s1 = "Welcome"; String s2 = "Java"; // create StringBuffer Instance StringBuffer newstring=new StringBuffer(); // using append method to concatenate strings StringBuffer concatenate=newstring.append(s1).append(s2); // printing System.out.println("The String s1 Value:"+s1); System.out.println("The String s1 Value:"+s2); System.out.println("After Concate: "+concatenate); } }
Output:
The String s1 Value:Welcome The String s1 Value:Java After Concate: WelcomeJava
With the append() method, we can use two different data types like the below:
package com.softwaretestingo.string; public class StringConcatUsingStringBuffer2 { public static void main(String[] args) { String s1 = "Welcome to SoftwareTestingo on: "; Integer s2 = 2023; // create StringBuffer Instance StringBuffer newstring=new StringBuffer(); // using append method to concatenate strings StringBuffer concatenate=newstring.append(s1).append(s2); // printing System.out.println("The String s1 Value:"+s1); System.out.println("The String s1 Value:"+s2); System.out.println("After Concate: "+concatenate); } }
Output:
The String s1 Value:Welcome to SoftwareTestingo on: The String s1 Value:2023 After Concate: Welcome to SoftwareTestingo on: 2023
Using StringBuilder
One of the best methods to concatenate strings in Java is using the StringBuilder class. This method is easy and efficient when you want to combine multiple strings. However, this method is not commonly used when you only need to concatenate two strings.
Program: Write a Java Program to Combine two strings using the StringBuilder
package com.softwaretestingo.string; public class StringConcatUsingStringBuilder { public static void main(String[] args) { String s1 = "Welcome to SoftwareTestingo on: "; Integer s2 = 2023; // create StringBuffer Instance StringBuilder newstring=new StringBuilder(); // using append method to concatenate strings StringBuilder concatenate=newstring.append(s1).append(s2); // printing System.out.println("The String s1 Value:"+s1); System.out.println("The String s1 Value:"+s2); System.out.println("After Concate: "+concatenate); } }
Output:
The String s1 Value:Welcome to SoftwareTestingo on: The String s1 Value:2023 After Concate: Welcome to SoftwareTestingo on: 2023
Using String.concat() Method
This method appends some specific Strings to the end of the string. It creates a char array whose length equals the combined lengths of both joined strings. This method copies the String data into a new string using the private String constructor. The private String constructor does not copy the input char[].
Program: Write a Java program to combine two strings using the concat() method.
package com.softwaretestingo.string; public class StringConcatUsingConcatEx { public static void main(String[] args) { String s1 = "Welcome to : "; String s2 = "SoftwareTestingo"; // java string concatenation String s3 = s1.concat(s2); System.out.println(s3); } }
Output:
Welcome to : SoftwareTestingo
Performance:
It’s important to be mindful of how you concatenate strings in Java, as it can impact performance. Let’s see which method is best for string concatenation in terms of performance.
It is always best to use StringBuilder to concatenate strings in Java. This will provide the best performance as it outperforms all other methods. We can easily test that it is several hundred times faster than using the + operator and concat() method in Java. Additionally, StringBuilder performs better than StringBuffer due to the overhead caused by StringBuffer’s thread safety.
It is important to note that we should prefer StringBuilder over StringBuffer as a buffer for Strings. This is because StringBuffer takes extra time to synchronize. However, in a multithreaded environment, it is advisable to use StringBuffer.
Conclusion
The ‘string concatenation operation’ refers to joining two strings together. In this tutorial, we learned about how to do this in Java. Four different methods can be used for string concatenation in Java,: using the StringBuffer class, using the Plus operator, using the concat() method, and using the StringBuilder class.
We value your feedback! We would love to hear from you if you have any questions or suggestions to improve this blog post. Please feel free to leave your comments in the comment section below. Your insights and ideas are important to us, and we appreciate your participation in making this blog post even better. Thank you for being so supportive!