Java is a widely used programming language that provides developers with many tools to create powerful, scalable, and robust applications. One of these essential tools is Java packages, which organize and manage classes, interfaces, and other related resources. Before we start discussing Packages in Java, why do we need this?
Our computers have folders or directories for the classification and accessibility of various files easily based on requirements or types. Similarly, in Java, we have packages that serve as a container for classes, interfaces, and other resources, providing a structured approach to code organization, improved code reusability, and modularization.
Post On: | Java Package API |
Post Type: | Java Tutorials |
Published On: | www.softwaretestingo.com |
Applicable For: | Freshers & Experience |
Get Updates: | Software Testingo Telegram Group |
Java packages are critical in making Java a popular language for developing enterprise-level applications. This article aims to provide an in-depth overview of Java packages, including their benefits, how to create them, and how they can be used to develop powerful Java applications.
If this sounds like something interesting to learn, then keep reading for more information because, in this post, we are going to discuss the following:
- Java Packages Definition
- Java: What Is A Package?
- Java Package Creation
- Types of packages in Java
- How do you access a package from another package?
- Java Packages Naming Convention
- Subpackage in Java
- Static Import in Java
- Java package class
- Advantages of Java Package
- Java Package Manager
Java Packages Definition
A Java package is a set of related classes, interfaces, and sub-packages with common characteristics, such as the same data types or performing similar operations on the data.
Classes in the same package can access each other’s package, private and protected members. This allows classes to share information and functionality while maintaining a high security level.
What is a package in Java?
Java packages are essential in managing the complexity of large-scale Java applications. Developers can easily navigate and manage their codebase by organizing related classes and resources into packages. Each Java package has a unique name, which consists of multiple segments separated by periods. The first segment of the package name typically represents the domain name of the organization that created the package, followed by a specific package name that describes the functionality of the contained classes and resources.
Java Package Creation
Java Package creation is an easy task in Java. If you want to create a package, add the package command as the first statement in the Java source file. The Java file may contain classes, enumerations, interfaces, and annotation types you want to include in the package.
With some examples, let’s understand how we can create packages in Java, compile Java programs inside the packages, and execute them.
For Creating Java packages, we need to write the statement below:
package myPackage;
Here Package is the keyword, and myPackage is the package’s name.
Types of packages in Java
In Java, we have two types of packages and which are:
- Java API packages or built-in packages
- User-defined package
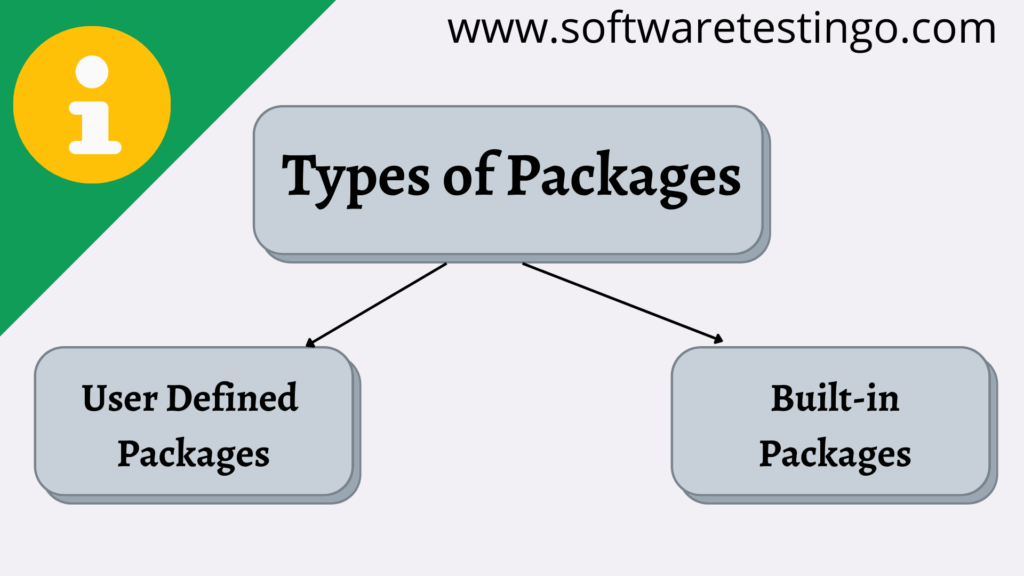
Java API packages or Built-in Packages
Built-in packages are included in the Java Development Kit (JDK) and contain classes and interfaces that provide essential functionality to Java applications. These packages are also referred to as standard or core packages. Some examples of built-in packages include java.lang, java.util, java.io, and java.net.
- java.lang package: This package provides classes fundamental to the Java programming language, such as Objects, Strings, and Integers.
- java.util package: This package provides classes for data manipulation, such as ArrayList, LinkedList, and HashMap.
- java.io package: This package provides classes for input and output operations, such as FileReader, FileWriter, and InputStream.
- java.net package: This package provides classes for networking operations, such as Socket, URL, and HttpURLConnection.
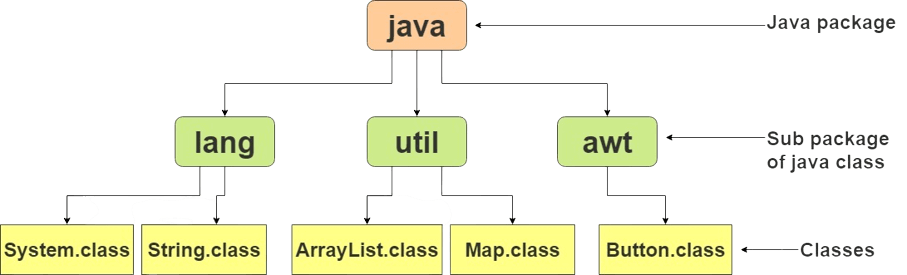
User Defined Packages
As the name suggests, the packages created by the user are called user-defined packages. Most user-defined packages are created by developers to group related classes and resources together. These packages are usually created to organize project-specific classes and resources. By creating user-defined packages, developers can create modular, reusable code that can be easily shared across different projects.
To create a user-defined package, developers must follow a specific naming convention. The package name should be in lowercase and follow the organization’s domain name, the project name, and the package name. For example, if the organization’s domain name is “example.com,” the project name is “project,” and the package name is “utils,” the complete package name should be “com.example.project.utils.”
Creating a user-defined package involves creating a directory structure that matches the package name and placing related classes and resources in that directory. Once the package directory structure is in place, developers can create classes and interfaces within the package by specifying the package name at the top of the source file.
User-Defined Packages Example:
In the below example, we will create a package called “myPackage.” And inside that, we will create two classes. But it would help if you kept in mind that the package statement should be written as the first statement Of your program, and the class is part of your package.
We are creating a directory whose name should be the same as the package name for creating a package. Then, inside the directory, we can create classes.
Note: A class can have only one package declaration. To understand this, you can follow the below program.
Add Class
package myPackage; public class Add { public int add(int a, int b) { return a+b; } public static void main(String args[]) { Add obj = new Add(); System.out.println(obj.add(10, 20)); } }
Multi-Java Class
package myPackage; public class Multi { public int multiplication(int a, int b) { return a*b; } public static void main(String args[]) { Multi obj = new Multi(); System.out.println(obj.multiplication(5, 10)); } }
After Creating the package, it will look like the below image:

How do you access a package from another package?
We can use the class of a package in another package in three different ways.
- Import package.class name;
- Import package.*;
- Fully Qualified Name.
Using import package.class name;
Note: The import keyword is used to import the classes or interfaces of other packages into current packages and make them accessible.
package com.SoftwareTestingo.PackageExamples; import myPackage.Add; public class Use_UDPForAdd { public static void main(String[] args) { Add obj = new Add(); System.out.println(obj.add(100, 200)); } }
In the above program, We want to implement the Add method of the Add Java class of myPackage java packages. So, for that, we have to use the import statement.
import myPackage.Add;
But if a package has more classes and you want to consume them in your program, we can import them using a single statement. We must use * with the import statement to import all the package classes.
import myPackage.*;
Using import package name.*;
When we want to import all the classes of a specific package at that time, we can use the package name.*. Then, it will import all the classes and interfaces of that package, but it will not import any classes or interfaces of the sub-packages.
Example of package that imports the package name.*
package com.SoftwareTestingo.PackageExamples; import myPackage.*; public class Use_UDPForMulti { public static void main(String[] args) { // Accessing Add method of Add Class Add obj = new Add(); System.out.println("Sum Of Two Numbers: "+obj.add(100, 200)); // Accessing Multiplication method of Multi Class Multi obj1=new Multi(); System.out.println("Multiplication Of Two Numbers: "+obj1.multiplication(20, 30)); } }
We have to use the import statement with *in the above program. So it imports all the classes of myPackage, and that’s why we can access the Add & Multi classes methods inside the PackageExamples package.
Using a Fully Qualified Name
Suppose you don’t want to use the import statement in your Java program but want to use other package classes. Then, in that case, we can use the fully qualified name. Then, only the mentioned class of another package will be accessible.
It is generally used when two packages have the same class name, e.g., java.util and java.sql packages contain the Date class.
Here’s an example to help you understand the concept. I declared a package earlier in the blog called myPackage. I’m going to use that same package here as an example.
Note: The program sequence must be the package name then the import statement after that class begins.
package com.SoftwareTestingo.PackageExamples; public class Use_FullyqualifiedName { public static void main(String[] args) { // using fully qualified name myPackage.Add obj=new myPackage.Add(); System.out.println("Sum Of Two numbers: "+ obj.add(11, 22)); } }
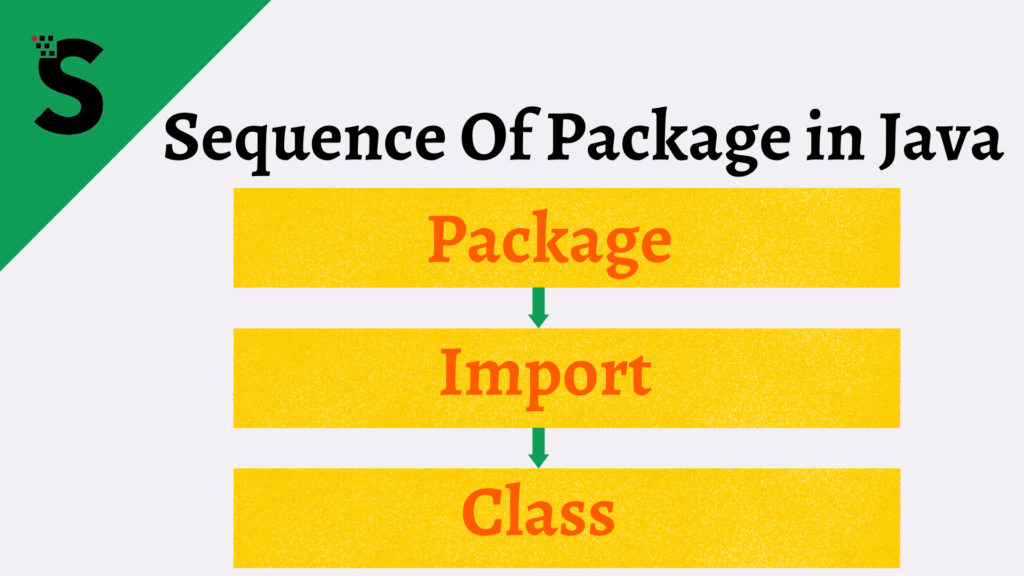
Java Package Naming Convention
There is a possibility that multiple packages can have the same name. So, to avoid this, we are following some naming conventions:
- We define our package names in all lowercase.
- package names are period-delimited
- Names are also determined by the company or organization that creates them
To find the package name for a particular organization, we usually start by reversing the company’s URL. After that, the company’s naming convention may include the names of divisions and projects.
For example,
com.softwaretestingo;
We can then further define sub-packages of this, like:
com.softwaretestingo.packageexamples;
Subpackage in Java
If a package is inside another package, the inner package is called a subpackage. It is created to categorize packages further. For example, if we make a tutorial package inside the Softwaretestingo package, then the tutorial package will be called a subpackage.
package softwaretestingo.tutorials;
Here softwaretestingo is a package, whereas tutorials is a subpackage. The standard for defining the package is domain.company.package. For Example, com.SoftwareTestingo.PackageExamples.
Static Import in Java
This Static Import in Java feature was first introduced in Java 5 and above. The static import allows the method & variables of a class to be directly used inside other classes without mentioning the class name in which these public static fields or methods are defined.
Note: If you overuse this static import in your program, then your program will be unreadable and unmaintainable.
package com.SoftwareTestingo.PackageExamples; import static java.lang.System.*; public class StaticImportExample { public static void main(String[] args) { // We don't need to use 'System.out' // Bcoz we have added imported the package by using static. out.print("SoftwareTestingo: This Is Example of Static Import"); } }
Java package class
The Java package class provides methods that help get information about a package’s specification and implementation. In the Java package class, we have different methods like getName(), getImplementationTitle(), getImplementationVendor(), getImplementationVersion(), etc.
package com.SoftwareTestingo.PackageExamples; public class PackageInfo { public static void main(String[] args) { Package p=Package.getPackage("java.lang"); System.out.println("package name: "+p.getName()); System.out.println("Specification Title: "+p.getSpecificationTitle()); System.out.println("Specification Vendor: "+p.getSpecificationVendor()); System.out.println("Specification Version: "+p.getSpecificationVersion()); System.out.println("Implementaion Title: "+p.getImplementationTitle()); System.out.println("Implementation Vendor: "+p.getImplementationVendor()); System.out.println("Implementation Version: "+p.getImplementationVersion()); System.out.println("Is sealed: "+p.isSealed()); } }
Output:
package name: java.lang Specification Title: Java Platform API Specification Specification Vendor: Oracle Corporation Specification Version: 1.8 Implementaion Title: Java Runtime Environment Implementation Vendor: Oracle Corporation Implementation Version: 1.8.0_152 Is sealed: false
Advantages of Java Package
People often ask what the benefit of using Package is, so we thought we would club all the advantages of using Java in a single place.
- Java packages enable better modularity and code reuse.
- Java packages organize classes with the same general functionalities, such as GUI or validation, etc., but they don’t need to be in any particular order.
- Java package groups related classes into one modular unit, which can be used for better code organization.
- Java packages enable better modularity and code reuse with the help of Java imports, which make it possible to use different Java packages in a single Java file without needing to import them directly.
- Java package provides an easy mechanism for organizing Java classes that have been logically grouped together based on their similar functionalities. But they don’t need to be located at the same level as they would if they were children or siblings of some common parent class from which all inherited functionality came.
- Java packages are also helpful when working on multiple projects because you can group your related projects under specific folders within your IDE.
Java Package Manager
A package manager keeps track of what software is installed on your computer and allows you to install new software easily, upgrade the software to more recent versions, or remove previously installed software.
We have different package managers for different languages available for Python and pypi.org. For ruby, we have rubygems.org. And for Csharp, we have nuget.org. But when we think about Java Package Manager, there is no such specific Java Manager.
Final Words:
At the end of our tutorial, we learned about the importance of packages for better management and access to code & also discussed the two types of Java Packages. We also covered how to access packages in other packages in Java with the help of syntax and coding examples. This will surely be helpful for your further study of the Java language.
Thanks for reading our article on Packages in Java. We hope you enjoyed reading our post! If you have any additional thoughts or comments, please share them in the comment section below. We’d love to hear from you.