Java Hello World Program Example With Java Code: In the last post, we get to know how we can successfully install Java installation on Windows 10 machines, and after that, in this post, we are going to learn how to write a simple Java program. As you all know, whenever we try to learn any new language, the first program we try to write is simple: a Hello World program. Now our environment is ready, so let’s write a simple Java program to print Hello World.
Java Hello World Program in Java
It is a basic program, so let’s learn how to write a simple Java Hello World program in Notepad and execute it using the command prompt. So, for that, open the notepad and write the sample codes.
package softwaretestingo.java.basics; public class HelloWorldProgram { public static void main(String[] args) { System.out.println("Hello World"); } }
After writing the code, save this file as “HelloWorldProgram.java” in any directory.
Compile and Run the Java Hello World Program
To compile and run the Hello World Program, go to the directory where you have stored the Java program and try to build and run the Java program. For compilation, the program uses this code:
javac helloWorldProgram.java
As you can see from the above image, when I compiled the program, I got an error stating that ‘javac‘ is not recognized as an internal or external command, operable program, or batch file. We got this error because we have not set the JAVA_HOME environment variable.
So when we try to compile the java program using the javac command, the compiler cannot find the javac, and because of that, we are getting this error. To resolve this issue, we have written an article, “How to set java_home in Windows. ” You can check that.
For Run the Program:
java helloWorldProgram
Java Hello World Program Explanation
Now, we know how to write a Java program and run a Java program by using the command prompt. But let’s go deeper and learn the meaning of whatever code is written.
public class helloWorldProgram {
It is the first statement of our Java program. Let me tell you that every Java program or application must have one class definition, which consists of a class keyword followed by a class name.
The above statement has keywords (public and class) and a class name (helloWorldProgram). When we listen to keywords in Java, that means keywords are predefined in Java with a specific purpose and utilization, and most importantly, you can’t change that; you have to use that keyword as it is. The other one is the class name, which you can give.
Now, let me tell you why I have mentioned public and class keywords in the statement. For now, I can say that I have mentioned it as public because I want others to access my program/class, so I made this class with a public access modifier. But I will share more details about the access modifier in a different post.
A Java file can have N numbers of classes, but it can have only one public class, and the Java file name should be the same as the public class name.
The next statement is:
public static void main(String args[]){
Let’s break down the above statement so that we can easily understand it:
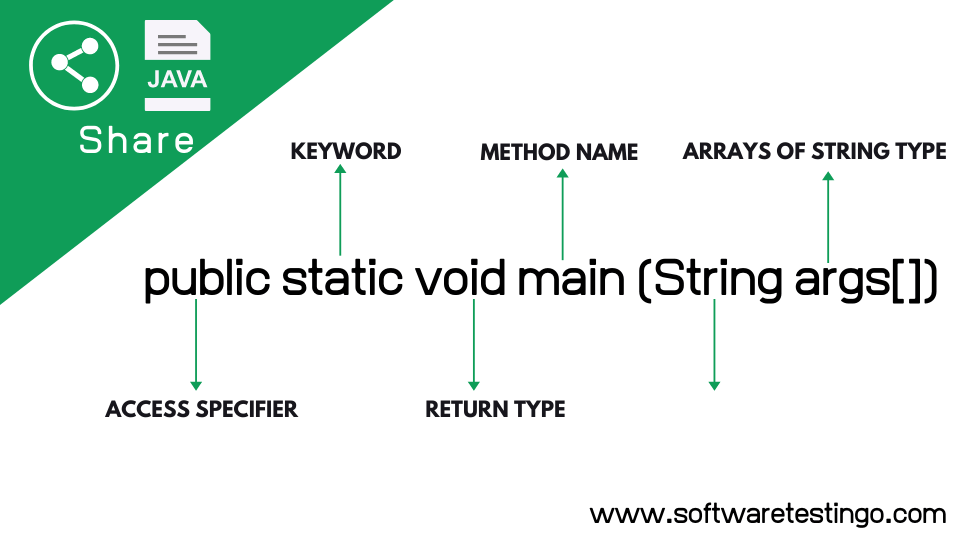
- Public: Public is an access modifier, and by declaring public to the Main method, we can call the Main method from outside of the class.
- Static: By declaring the Main method as static, we can call or run the Main method without creating an object because the static methods are run or executed while creating an object.
- Void: The main method returns nothing, so we declare it void.
- main: it is the method’s name and the starting point from which JVM starts to run your program.
- (String[] args): This is used for receiving the arguments in the command line as strings.
Note: The Keyword Public and Static position can be changed, but the Void & Main should be declared like void main. If you have mentioned the main void, then you will get errors.
The Final statement is:
System.out.println("Hello World");
So let’s break this statement also for a better understanding
- System: It is a class name.
- Out: It is a static member of the system class.
- println: This method prints the message in the output destinations like the console or file.
We hope we have given the Java Hello World Program example explanation in a detailed manner with a step-by-step explanation. Still, if you have any questions, you can drop them in the comment box, and we will be happy to resolve them.
Why is Hello World the first program?
A very simple program was used first to test things out. According to Wikipedia, the tradition of using the phrase Hello, the world as the test message was influenced by an example program in the book The C Programming Language by Brian Kernighan and Dennis Ritchie.
How do I write my first Java program?
The basic steps to create the Hello World program are to write the program in Java, compile the source code, and run the program.
Very nyc explanation about the very basic things of Java
Nice explanation , Great job for new learners as well as experienced peoples also