Inheritance in Java Programming: In Our Previous Post, we discussed different Java access modifiers, and in this post, we will discuss one of the main OOPS concepts of Java called Inheritance.
To be a Good Java developer, you must know Java OOPS concepts like Inheritance, Abstraction, Encapsulation, and Polymorphism. So, in this post, we will discuss one of the important concepts of the OOPS concept, which is Inheritance, and we will also learn how we can achieve inheritance in the Java programming language.
In this post. We are going to discuss the following:
- Introduction of Inheritance
- Types Of Inheritance
- Important Fact about Inheritance
Introduction Of Inheritance in Java
The process by which one class acquires the data member (Variables) and member functions (methods) of another class that process is called inheritance. The main benefit of Inheritance is it provides code reusability so that a class can extend the properties and behaviors of an existing class. After that, we need to write only the unique new features.
Important Terminology Of Inheritance
- Parent Class: A Class whose properties and member functions are inherited by another class is known as a parent class, superclass, or base class.
- Child Class: A Class that extends the properties and behaviors of another class is called a child class, subclass, or derived class.
Inheritance Syntax in Java
For Inherit the data member and member functions, we are using the keyword “extends“. Suppose there are two classes (Class1 & Class2) present, where class2 inheriting the properties and methods of class1, Then we have to write like below
Class class2 extends class1 { //Body }
Types of Inheritance in Java
In Java, there are mainly five types of inheritance are there, that is:
Single Inheritance in Java
In this type of inheritance, there are two classes present: one is the parent class, and another one is the child class. Here, the child class inherits the properties of the parent class into the child class. We can reuse the existing code and add new features in the child class.
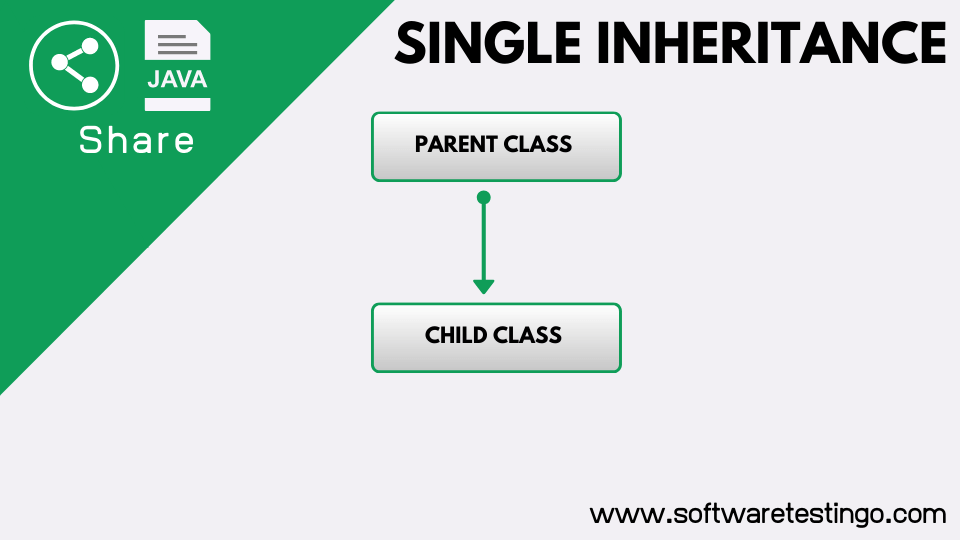
Single Inheritance Example:
package com.SoftwareTestingo.JavaBasics; class Single_ParentClass { public void parent_method() { System.out.println("Parent Class Method"); } } public class Single_Inheritance extends Single_ParentClass { public void child_method() { System.out.println("Child class Method"); } public static void main(String[] args) { Single_Inheritance obj=new Single_Inheritance(); obj.parent_method(); //Calling Parent Class Method obj.child_method(); //Calling Child Class Method } }
Output:
Parent Class Method Child class Method
Multi-Level Inheritance in Java
When a Class has more than one parent class, that type of inheritance is called multilevel inheritance. If you saw the pic, you can find that Class B inherits members and methods of Class A, and Class C extends Class B.
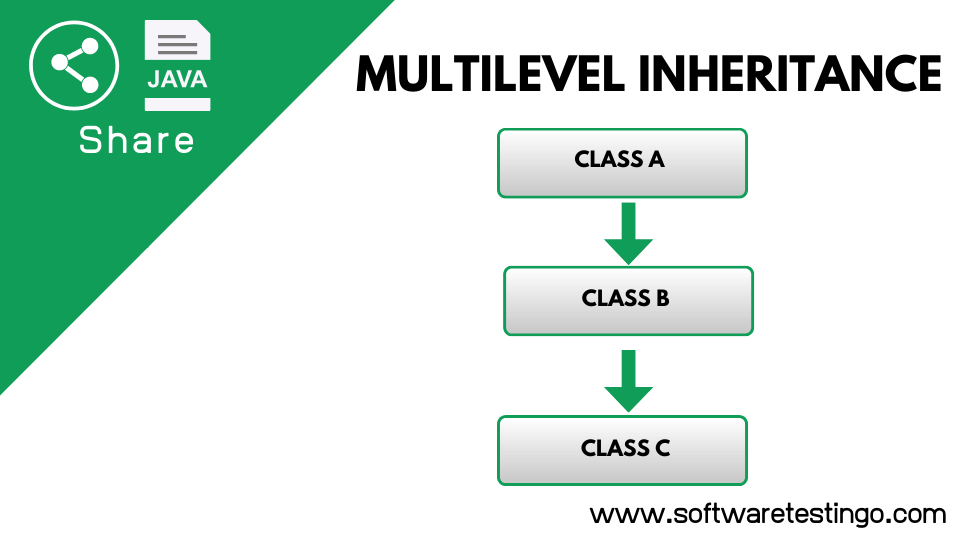
Multi-Level Inheritance:
package com.SoftwareTestingo.JavaBasics; class MultiLevel_ParentClass { public void parent_method() { System.out.println("Parent Class Method"); } } class Middle_Class extends MultiLevel_ParentClass { public void middleClass_method() { System.out.println("Middle Class Method"); } } public class MultiLevel_Inheritance extends Middle_Class { public void child_method() { System.out.println("Child Class Method"); } public static void main(String[] args) { MultiLevel_Inheritance obj=new MultiLevel_Inheritance(); obj.parent_method(); //Calling Grand Parent Method obj.middleClass_method(); // Calling Parent Method obj.child_method(); //Calling Child Method } }
Output:
Parent Class Method Middle Class Method Child Class Method
Hierarchical Inheritance in Java
In this type of inheritance, one class serves as a base class, and multiple subclasses extend the features and methods of that base class. As you saw in the diagram, Class A Is the base class, and Class B, Class C, And Class D are the subclasses extending Class A.
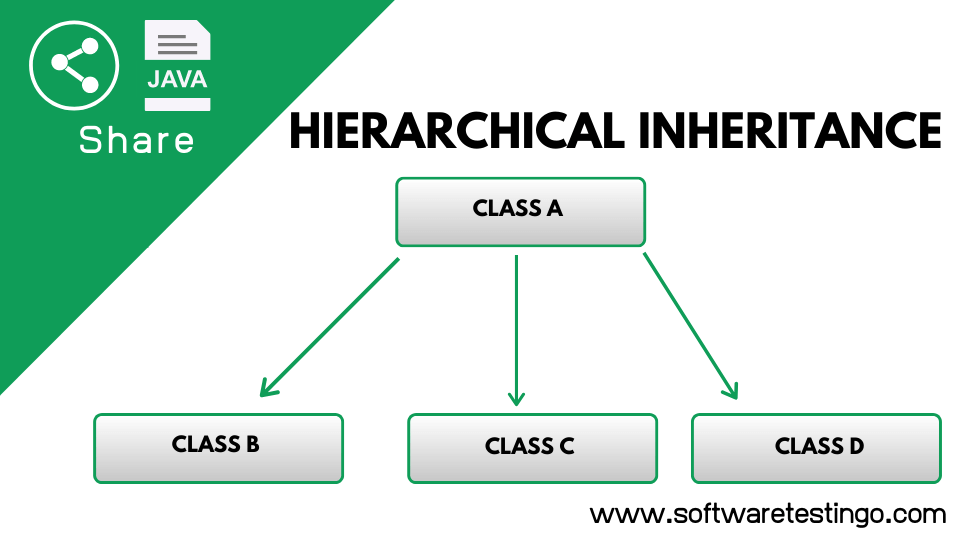
Hierarchical Inheritance Example:
package com.SoftwareTestingo.JavaBasics; class parent_class { public void parent_method() { System.out.println("Parent Class Method"); } } class ClassA extends parent_class { public void classAMethod() { System.out.println("Class A Method"); } } class ClassB extends parent_class { public void classBMethod() { System.err.println("Class B Method"); } } class ClassC extends parent_class { public void ClassCMethod() { System.out.println("Class C Method"); } } public class Hierarchical_Inheritance { public static void main(String[] args) { ClassA obj1=new ClassA(); ClassB obj2=new ClassB(); ClassC obj3=new ClassC(); // Call All Classes Method by Using above objects obj1.classAMethod(); obj2.classBMethod(); obj3.ClassCMethod(); } }
Output:
Class A Method Class B Method Class C Method
Multiple Inheritance in Java
In Multiple inheritances, one class has more than one base class and inherits features from all its parent classes. Java doesn’t support multiple inheritances with classes, But we can achieve multiple inheritances by implementing Interface.
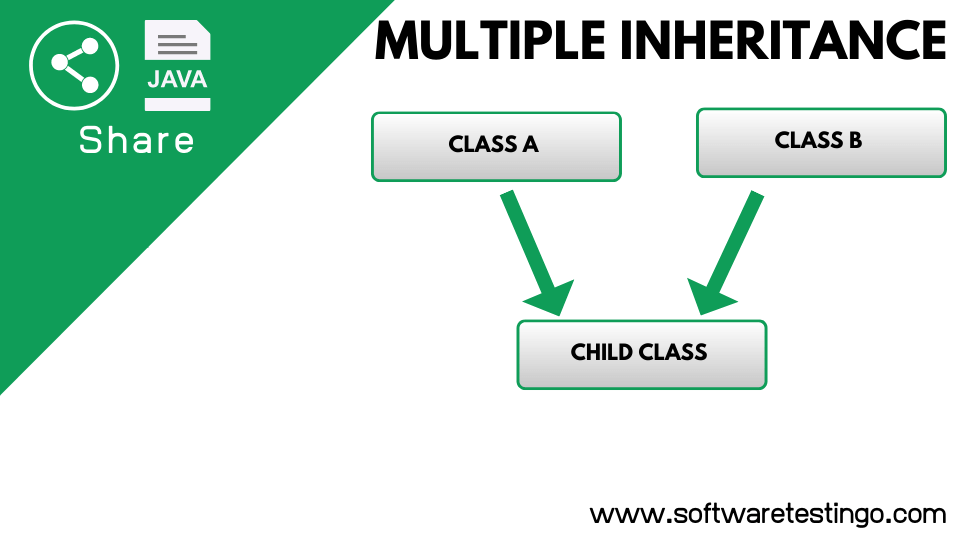
Multiple Inheritance Example:
package com.SoftwareTestingo.JavaBasics; class ParentClass1 { public void parentMethod1() { System.out.println("Parent Method Called"); } } class ParentClass2 { public void parentMethod2() { System.out.println("Parent Method Called"); } } //Java Does Not Support Multiple Inheritance //public class Multiple_Inheritance extends ParentClass1, ParentClass2 //{ // public static void main(String[] args) // { // Multiple_Inheritance obj=new Multiple_Inheritance(); // obj.parentMethod1(); // obj.parentMethod2(); // } //}
You Will get the following errors:
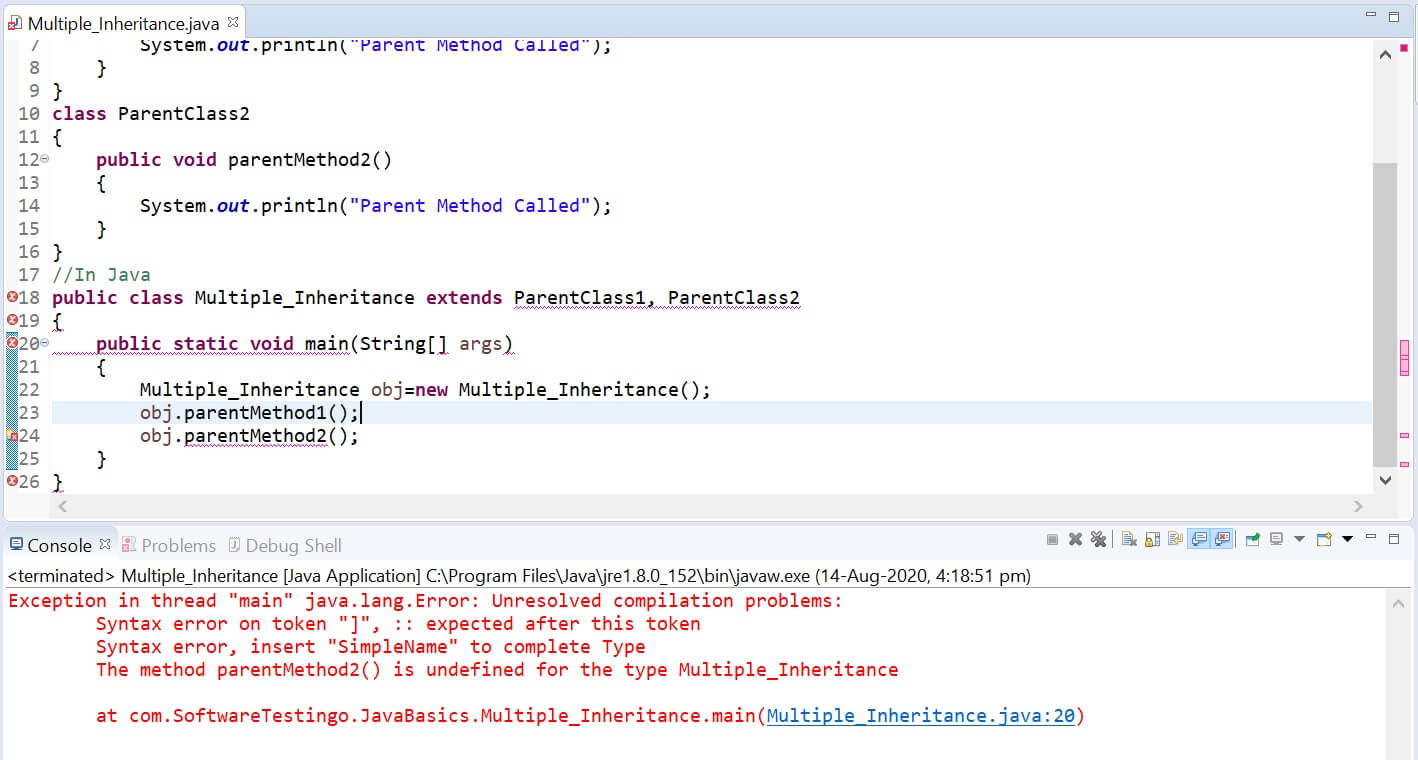
Hybrid Inheritance in Java
The combination of one or more types of inheritance is called Hybrid inheritance.
Points to Remember
- Multiple Inheritance is Not allowed in Java: Multiple inheritances mean a class has more than one parent class. Because of these features, there is a chance of ambiguous situations. That’s why multiple inheritances are not permitted in Java.
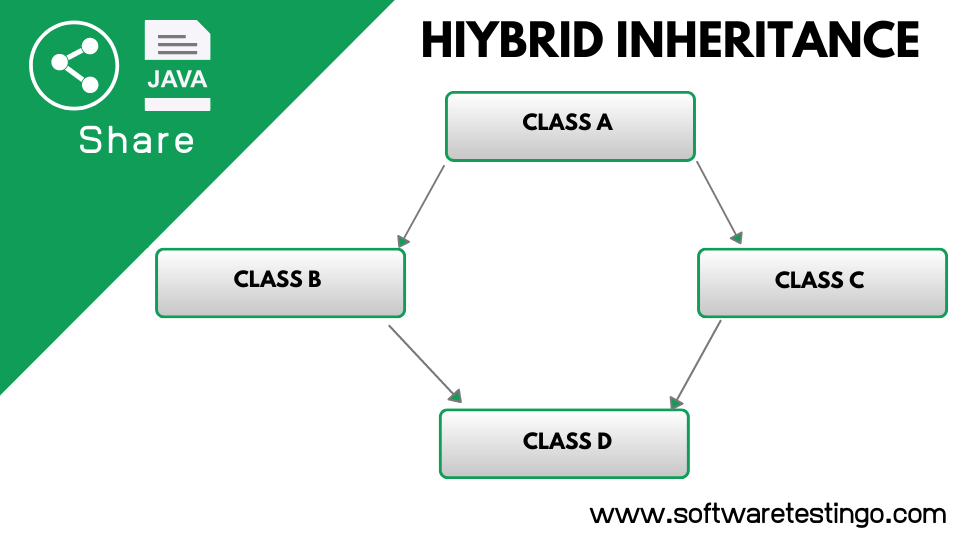
Note: Multiple inheritances are not permitted in Java, but you can achieve that by using Interface.
- Cyclic Inheritance Is not allowed: It is a type of inheritance where a class extends itself or by some loop. Such inheritance is not allowed in Java because this leads to ambiguity.
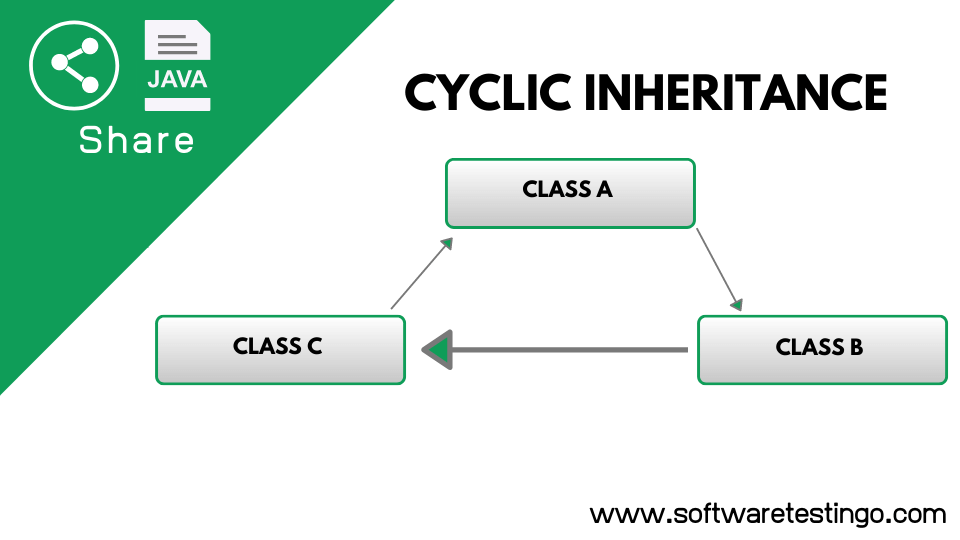
- Private Members Are Not Inherited
- Constructors Can Not be Inherited In Java
Constructors can not be inherited in Java as the subclass has a different name. But when we are creating the subclass object, that time default constructor of the parent class is called by default. It is all happening because when the child class constructor is called, the first statement of a child class constructor is a super keyword. That’s why when we create a child class object, the parent class-default constructor is also called automatically.
Because of the super keyword, we can call the parent class constructor. Other than this, we can not call the parent class constructor directly.
Default | Private | Protected | Public | |
---|---|---|---|---|
Same Class | Yes | Yes | Yes | Yes |
Same Package Subclass | Yes | No | Yes | Yes |
Same Package Non-Subclass | Yes | No | Yes | Yes |
Different Package Subclass | No | No | Yes | Yes |
Different Package Non-Subclass | No | No | No | Yes |
Ref: article