Final Keyword Variable, Class, & Method In Java: In this tutorial, we will learn about the Final keyword of Java programming language. In Java, final is a reserved keyword with variables, methods, classes, etc.
In Java, the final keyword declares a variable, method, or class as unchangeable or unextendable. When a variable is declared as final, its value cannot be modified once assigned, and when a method or class is declared as final, it cannot be overridden or extended by any subclass. The final keyword is essential in ensuring data integrity and program security, preventing unexpected modification or inheritance.
In this article, we will explore the different uses of the final keyword in Java programming and provide examples to illustrate its functionality.
Java Final Keyword Uses
In Java programming language, we use a final keyword to give restrictions to the users. We can use the Java keyword in many contexts:
Let’s discuss each of them one by one in detail:
Java Final Variable
When you declare a variable with the final keyword, that variable is called the final variable. You cannot change a final variable’s value once it is initialized. However, the final variable differs from the constant variable because it’s not compulsory to assign the value to a final variable at the time of declaration.
We can assign the variable in 3 different ways:
- By an assigned statement
- Inside Constructor Block
- Static Initializer Block
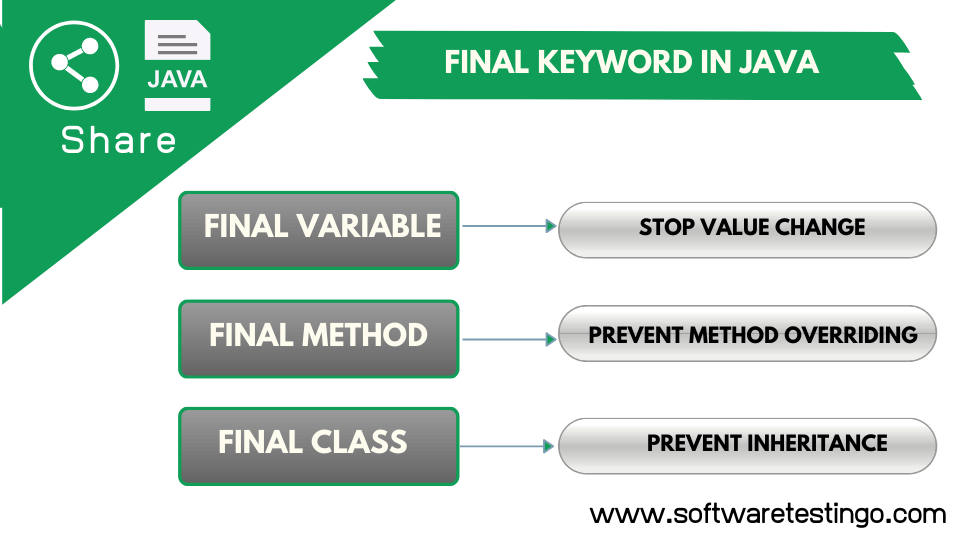
Initialized By Assign Statement
Using the assignment operator, we can initialize a final variable at the time of the declaration. You can check the below example for understanding:
class Point { public final int x = 1; // final variable public static void main (String[] args) { System.out.println("The Value of X is:-"+ x); } }
Inside Constructor Block ( Blank final variable )
Suppose a final variable is not initialized at the time of declaration. In that case, that variable type is called the final blank variable. Then, we can initialize that final variable inside the class constructor on which the final variable is mentioned. If you try to initialize a final blank variable in any other way, you will get a compilation error.
Take a look at the below example of how to initialize a final blank variable:
class Demo { //Blank final variable final int MAX_VALUE; Demo() { //It must be initialized in constructor MAX_VALUE=100; } void myMethod() { System.out.println(MAX_VALUE); } public static void main(String args[]) { Demo obj=new Demo(); obj.myMethod(); } }
Note: If you have more constructors in a class, you need to be initialized in all the constructors; otherwise, you will get a compile-time error.
Static Initializer Block ( Static Final Variable )
If a variable is declared with static and final keywords, then such a variable type is called the final static variable. We can initialize a final static variable at the time of declaration or inside the static block of the class in which it is declared. If a final static variable is not initialized at the time of declaration, then such variables are called blank static final variables.
You will get a compilation error if you try to initialize a final static variable outside a static block.
When to use a Final Variable
The difference between a normal and a final variable is that we can change or re-assign the value of a normal variable between the programs. Still, if we initialize a value for a final variable, you can’t change the final variable’s value. So, it’s better to use the final variable for the constant variables of the program.
Final Method In Java
Sometimes, we want to restrict other classes to override the method of the parent classes. To achieve this, we can define the methods with the final keyword, and such methods are called final methods.
The main reason behind the final method declaration is the content of the method should not be changed by outside.
class A { final void m1() { System.out.println("This is a final method."); } } class B extends A { void m1() { // COMPILE-ERROR! Can't override. System.out.println("Illegal!"); } }
Final Class In Java
If a class is defined with the keyword final, then that class is called the final class. If a class is declared final, it can not be extended (inherited), and final classes are completed in nature. We are using the final class for secure and efficient code. In Java programming language, several classes are declared final in the standard library—for example, Java.lang package and String class.
Final Keyword Important Points
- You Can not declare the constructor as final.
- If you want no other class, you can extend your class, and then you can declare your class as final.
- If you declare data members as final, that acts as constant. You can not change the values of those data members.
- All an interface’s variables or data members are static and final by default.
Ref: article