Escape Sequence in Java: Escape sequences in Java are fundamental to handling special characters within strings and characters. In this blog post about escape characters in Java, we will discuss the role of escape character, their types, and their importance in Java programming.
What Is Escape Sequence?
An escape sequence is a series of characters used in computer programming and text processing to represent special characters or control characters within a text string.
These special characters might be challenging to include directly in a string because they can be mistaken for regular text and have reserved meanings in the programming language.
Escape sequences typically start with a special escape character, which is often a backslash \, followed by one character that conveys a specific meaning. When the programming language or text processor encounters an escape character, it interprets it as the special character or action it represents rather than as literal text.
List Of Escape Characters in Java
In Java, we have the following escape characters available.
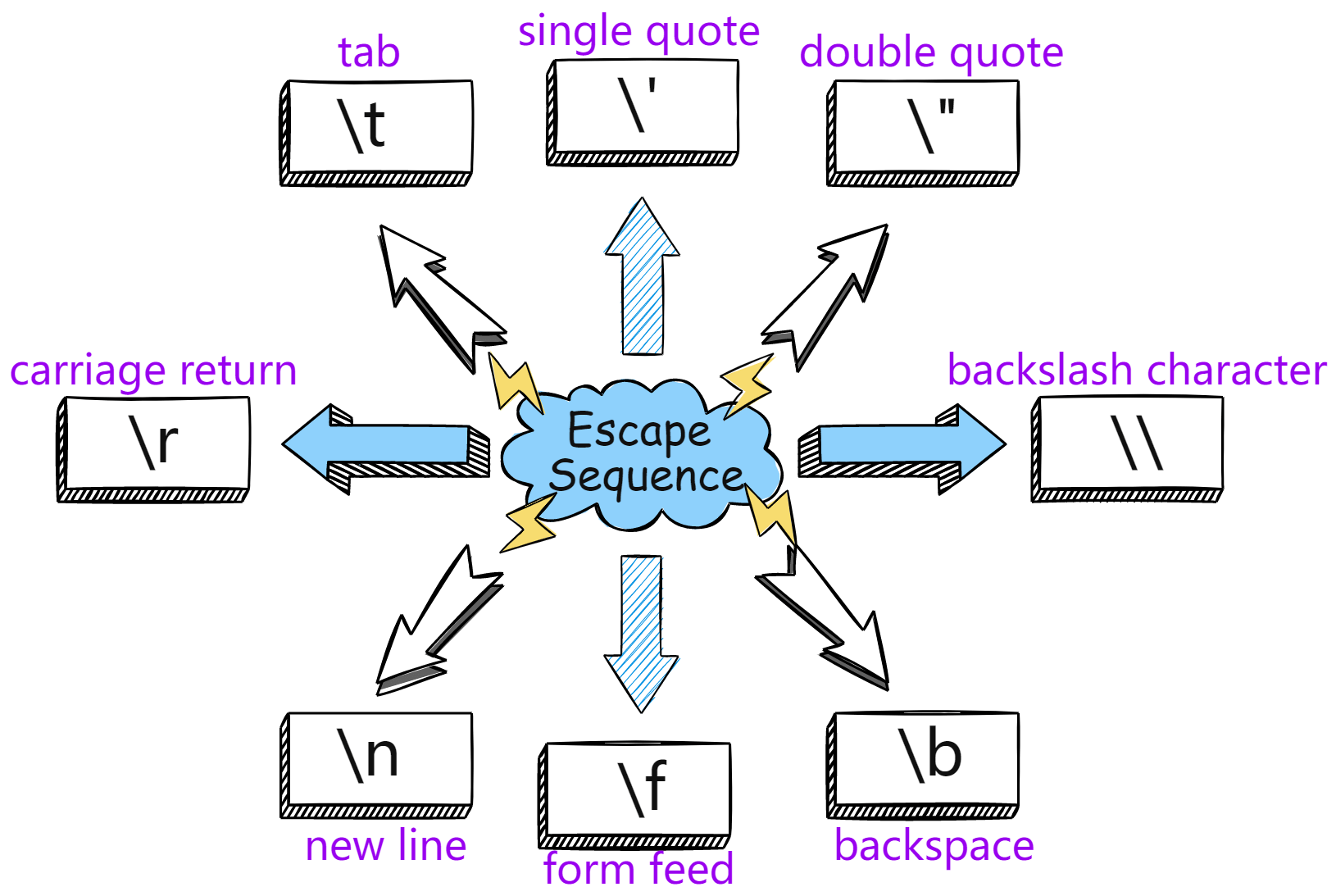
Escape Characters | Description |
---|---|
\t | It is used to insert a tab in the text. |
\’ | It is used to insert a single quote character in the text at this point. |
\” | It is used to insert a double quote character in the text |
\r | It is used to insert a carriage return in the text. |
\\ | It is used to insert a backslash character in the text. |
\n | It is used to insert a new line in the text. |
\f | It is used to insert a form feed in the text. |
\b | It is used to insert a backspace in the text. |
Why We Need Escape Sequences In Java?
Escape sequences are essential for tasks such as including double quotes within a string enclosed by double quotes (\”), inserting tabs (\t) to create horizontal spacing, or representing characters that are not easily typed, like special symbols or non-ASCII characters using Unicode escape sequences (e.g., \uXXXX).
For example, we must display as [ Welcome To “SoftwareTestingo” blog ]. You will get this error when you try to write inside the println statement to display the below statement.
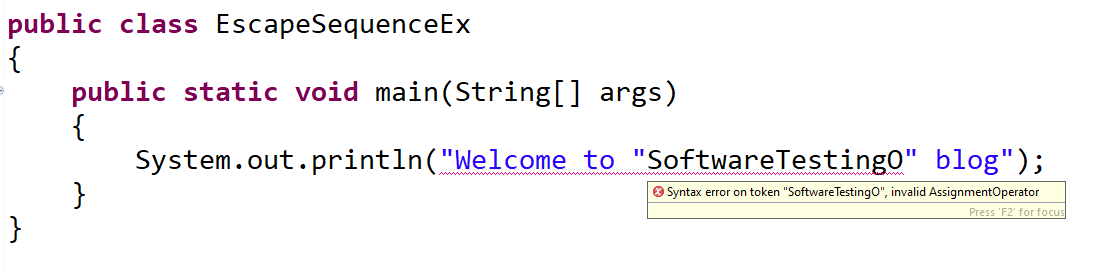
There are some other scenarios where we need Escape Characters to handle smoothly. Below, we have given examples of how to use these escape characters in your Java program.
Escape Sequence In Java With Example
Here’s an explanation of some commonly used Java escape characters, along with real-time examples:
\n – Newline Character:
public class EscapeSequenceEx { public static void main(String[] args) { System.out.println("Welcome to \nSoftwareTestingO blog"); } }
\t – Tab Character:
\t -> It gives a tab between two words.
public class EscapeSequenceEx { public static void main(String[] args) { System.out.println("Welcome to \tSoftwareTestingO blog"); } }
\’ – Single Quote Character:
This \’ escape sequence is for printing a single quotation mark on the text string.
public class EscapeSequenceEx { public static void main(String[] args) { System.out.println("Welcome to SoftwareTestingO\'s blog"); } }
\” – Double Quote Character:
This \” escape sequence is for printing a double quotation mark on the text string.
public class EscapeSequenceEx { public static void main(String[] args) { System.out.println("Welcome to \"SoftwareTestingO\" blog"); } }
\\ – Backslash Character:
This \ escape sequence is for printing a backslash on the text string
public class EscapeSequenceEx { public static void main(String[] args) { System.out.println("Welcome to SoftwareTestingO \\g blog"); } }
\b – Backspace Character:
The escape sequence \b is a backspace character; it moves the cursor one character back with or without deleting the character(depending upon the compiler)
public class EscapeSequenceEx { public static void main(String[] args) { System.out.println("Welcome to SoftwareTestingO \bg blog"); } }
\r – Carriage Return Character:
This \r escape sequence is a carriage return character. It moves the output point back to the beginning of the line without moving down a line (usually).
public class EscapeSequenceEx { public static void main(String[] args) { System.out.println("12345\rABCD"); } }
\f – Form Feed Character:
public class EscapeSequenceEx { public static void main(String[] args) { System.out.println("Hello\fWorld"); } }
These escape sequences are essential in Java to include special characters in strings or character literals. They allow you to represent characters that would otherwise be challenging to include directly.
Using Escape Characters in Java Program
Certainly! Here’s a Java program that uses all the escape sequence characters:
public class EscapeSequenceExample { public static void main(String[] args) { // Newline character (\n) System.out.println("Hello,\nWorld!"); // Tab character (\t) System.out.println("Name:\tJohn"); // Single Quote (\') char singleQuote = '\''; System.out.println("This is a single quote: " + singleQuote); // Double Quote (\") String message = "She said, \"Hello!\""; System.out.println(message); // Backslash character (\\) String filePath = "C:\\Users\\John\\Documents\\file.txt"; System.out.println("File path: " + filePath); // Backspace (\b) System.out.println("Hello\bWorld"); // Carriage Return (\r) System.out.println("12345\rABCD"); // Form Feed (\f) System.out.println("Hello\fWorld"); // Using all escape characters in a string String allEscapeChars = "Newline: \\n, Tab: \\t, Single Quote: \', Double Quote: \", Backslash: \\\\", + " Backspace: \\b, Carriage Return: \\r, Form Feed: \\f"; System.out.println(allEscapeChars); } }
This program demonstrates various escape sequence characters in Java, including newline, tab, single quote, double quote, backslash, backspace, carriage return, and form feed. It also includes a string that contains all these escape sequences for reference.
Output:
Hello, World! Name: John This is a single quote: ' She said, "Hello!" File path: C:\Users\John\Documents\file.txt HelloWorlABCD HelloWorld Newline: \n, Tab: \t, Single Quote: ', Double Quote: ", Backslash: \, Backspace: \b, Carriage Return: \r, Form Feed: \f
This output demonstrates how each escape sequence character affects the formatting and content of the displayed text.
Conclusion:
Escape sequences in Java are indispensable for handling special characters, formatting text, and working with Unicode. Understanding their types and applications is vital for writing robust and versatile Java code.
If you have any doubts about using escape sequences in Java or suggestions to improve this article, please don’t hesitate to comment below. Your feedback is essential in enhancing our resources.