Encapsulation In Java: Like in every post on our SoftwareTestingo blog, in this post, we are also going to learn some important concepts of Java programming language. As you know, for a better programmer, you clearly know about different Java OOPS concepts, such as inheritance, abstraction, encapsulation, and polymorphism. So, in this post, we will discuss Encapsulation In Java.
In this article, we will share one of the most important concepts of OOPS, Encapsulation in Java, and learn how to achieve that. Binding data members and methods in a single unit is called encapsulation in Java. During this post, we are going to discuss the following:
- What is Encapsulation
- Why do we need Encapsulation in Java?
- Benefits of Encapsulation
- Encapsulation Example
What is Encapsulation in Java?
Encapsulation refers to binding the data member and member function (methods) in a single unit. In other words, we can say that encapsulation is made of a protective shield that prevents the data from reaching the outer side of the shield.
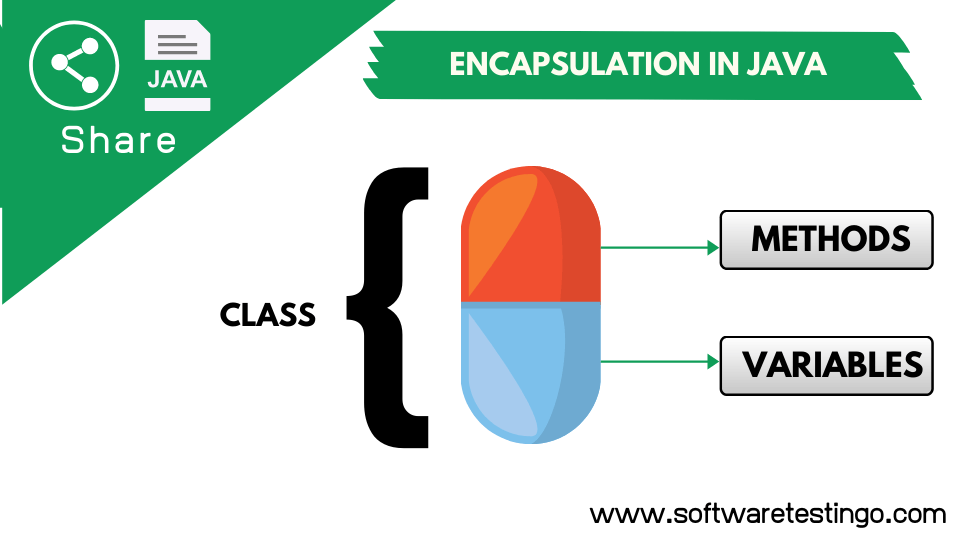
Example Program 1:
Suppose we have a class person, and in that, we have 2 data members and 2 methods like the below:
package com.softwaretestingo.oops; public class EncapsulationParent { String name; int age; void userinfo() {}; void address() {}; }
The EncapsulationParent class exemplifies encapsulation, as its variables (name and age) and methods (userinfo and address) are packaged together within a single unit. Encapsulation binds the implementation details of the EncapsulationParent class and hides them from other classes. To access these details, other classes must create an object of the EncapsulationParent class to access its data and behaviors, as shown below.
package com.softwaretestingo.oops; public class EncapsulationChild { public static void main(String args[]) { EncapsulationParent obj=new EncapsulationParent(); obj.name="SoftwareTestingo"; System.out.println("The Name: "+obj.name); } }
Data Hiding
Now, we will see another form of encapsulation: Data Hiding. In this Encapsulation type, a class’s variables and data members (methods) are hidden from other classes. They can be accessed only through the member function of the same class where they are declared.
This encapsulation type aims to protect the data from misuse by the outside world. This is also called Data hiding or Data encapsulation. And for that, we have to declare the variables with private access modifiers.
After declaring private variables, if you try to access them in a different class, then you will get this type of error:
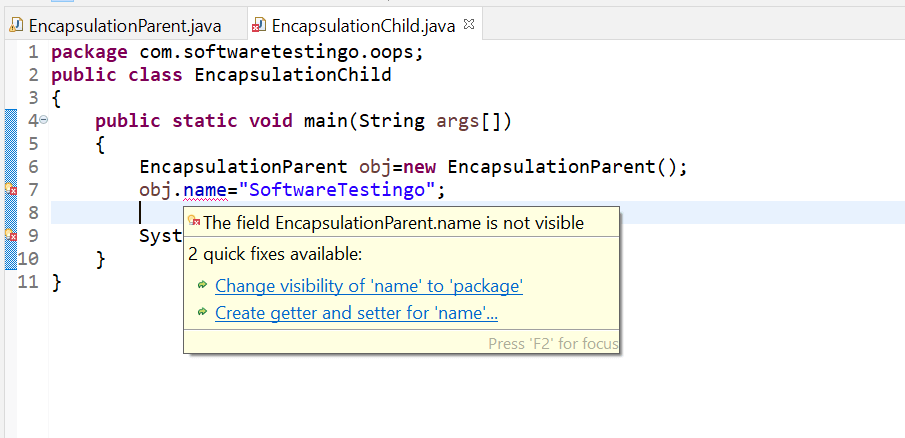
The question now is, what if other classes need to read and write this data? If other classes cannot access the data, then the usefulness of this class may be limited. To enable external access to the data, we can create getters (getXXX()) and setters (setXXX()) methods for these variables, as shown below.
package com.softwaretestingo.oops; public class EncapsulationEx { private String name; private int age; public String getName() { return name; } public void setName(String name) { this.name = name; } public int getAge() { return age; } public void setAge(int age) { this.age = age; } public static void main(String[] args) { EncapsulationEx obj=new EncapsulationEx(); //Set the Value obj.setName("Ramesh"); obj.setAge(20); //Print The Value System.out.println("The User Name is: "+obj.getName()); System.out.println("The User Age is: "+obj.getAge()); } }
Output:
The User Name is: Ramesh The User Age is: 20
Later, we can also enhance the setter method to avoid unwanted values. For Example, if you don’t want to set null values to the name variable, then we can use the following:
public void setName(String name) { if(name == null || name.equals("")) { throw new IllegalArgumentException("Name can not be null or empty"); } this.name = name; }
Output:
The User Name is: Ramesh The User Age is: 20
Why Do We Use Data Hiding?
The primary purpose of data hiding is to enhance security. By using private modifiers with our variables, we can store sensitive information in those variables that will only be visible within the class and cannot be directly accessed by anyone else. Additionally, by applying conditions in setter methods as needed, we can further increase security to prevent the setting of any illegal data that may be misused.
How To Achieve Encapsulation In Java?
While encapsulation is already achieved through the creation of classes, interfaces, objects, and so on, if you desire to create a completely encapsulated class in Java, the following steps should be followed:
- Declare all member variables with the private access modifier.
- Create public getter and setter methods for those variables to allow access outside the class.
Advantages of Encapsulation
Encapsulation offers several benefits to your code, including:
- Security: Encapsulation helps to secure your code by preventing other units (classes, interfaces, etc.) from accessing data directly.
- Flexibility: Encapsulation makes your code more flexible, enabling programmers to change or update the code easily.
- Control: Encapsulation provides control over the data stored in member variables. For instance, you can control the values set in the “age” variable of a “Person” class without breaking the code.
- Reusability: Encapsulated code or units can be reused anywhere within or across multiple applications. For example, if you have a “Person” or any other class in your application, you can reuse that class wherever needed.
- Maintainability: Encapsulated units are easy to maintain since you can easily locate or modify them.
Ref: article