This blog post will discuss the Java String compareTo() method. This is a useful and important function for any Java Developer. We will review all the different scenarios in which it can be used, such as sorting arrays or checking if strings are equal.
We will be talking about the String compareTo method with you today. We’ll show you how it’s used and what the different return values mean for your code. This topic is important, so we hope this post will help clear up any confusion!
Post On: | compareTo() String Method in Java |
Post Type: | Java Tutorials |
Published On: | www.softwaretestingo.com |
Applicable For: | Freshers & Experience |
Get Updates: | SoftwareTestingo Telegram Group |
Introduction
The Java String compareTo() method can compare two strings lexicographically (means comparing strings character by character based on their Unicode values from left to right). This involves converting each character of both strings into a Unicode value and comparing them. If the two strings are equal, this method will return 0. Otherwise, it will return either a positive or negative value. The result is positive if the first string is lexicographically greater than the second string; otherwise, the result will be negative.
compareTo() Return Value
In short, Suppose there are two strings, s1 and s2, then
if s1 > s2, it returns positive number
if s1 < s2, it returns negative number
if s1 == s2, it returns 0
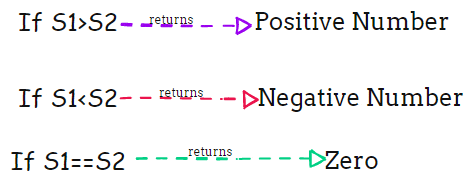
Syntax of compareTo()
The compareTo() method in Java compares two objects of the same type. It returns a positive integer, negative integer, or zero. The implementation of this method varies depending on the object’s class.
public int compareTo(String anotherString)
Program: Write a Java Program to Compare Two Strings.
package com.SoftwareTestingo.JavaBasics; public class CompareToExample1 { public static void main(String[] args) { String s1="Software"; String s2="Software"; String s3="Visit"; String s4="Testingo"; String s5="Blog"; //Returns 0 because both are equal System.out.println(s1.compareTo(s2)); //Returns -3 because "S" letter is 3 times lower than "V" System.out.println(s1.compareTo(s3)); //Return -1 because "S" is 1 times lower than "T" System.out.println(s1.compareTo(s4)); //Return 17 because "S" is 17 times greater than "S" System.out.println(s1.compareTo(s5)); } }
Output:
0 -3 -1 17
compareTo() in Java takes only a single Object as a parameter to be compared with. After comparing the compareTo() method, return an integer value. And sometimes, we are getting Exceptions such as ClassCastException & NullPointerException.
Variants of the compareTo() Method in Java
Before discussing the exception, let’s try to understand different compareTo() method variants. There are three variants of the compareTo() method. We will discuss those variants.
- int compareTo(Object obj)
- int compareTo(String anotherString)
- int compareToIgnoreCase(String str)
int compareTo(Object obj)
This method compares a string with an object, which will return values based on that. And syntax of this:
int compareTo(Object obj)
This method returns 0 if the argument is a string that is lexicographically equal to this string. If the argument is a string that is lexicographically greater than this string, the method returns a value less than 0. Finally, if the argument is a string that is lexicographically less than this string, the method returns a value greater than 0.
Java int compareTo(Object obj) Example
package com.softwaretestingo.string; public class CompareToExample2 { public static void main(String[] args) { // Initializing Strings String str1 = "Software"; String str2 = new String("Software"); String str3 = new String("Testingo"); // Checking str1 string with str2 object System.out.println("Difference is : "+ str1.compareTo(str2)); // Checking str1 string with str3 object System.out.print("Difference is : "+str1.compareTo(str3)); } }
Output:
Difference is : 0 Difference is : -1
int compareTo(String anotherString)
This method compares two string objects lexicographically.
int compareTo(String anotherString);
package com.softwaretestingo.string; public class CompareToExample3 { public static void main(String[] args) { // Initializing Strings String str1 = "Software"; String str2 = "Software"; String str3 = "Testingo"; // Checking str1 string with str2 string System.out.println("Difference is : "+ str1.compareTo(str2)); // Checking str1 string with str3 string System.out.print("Difference is : "+str1.compareTo(str3)); } }
Output:
Difference is : 0 Difference is : -1
The value 0 if the argument is a string that is equal to this string in terms of alphabetical order; a value less than 0 if the argument is a string that comes after this string in alphabetical order; and a value greater than 0 if the argument is a string that comes before this string in alphabetical order.
int compareToIgnoreCase(String str)
This method compares two strings lexicographically, ignoring case differences.
int compareToIgnoreCase(String str);
package com.softwaretestingo.string; public class CompareToExample4 { public static void main(String[] args) { // Initializing Strings String str1 = "software"; String str2 = "SoFTwaRe"; // Checking str1 string with str2 string System.out.println("Using compareTo Difference is : "+ str1.compareTo(str2)); // Checking str1 string with str3 string using System.out.print("Using compareToIgnoreCase Difference is :"+str1.compareToIgnoreCase(str2)); } }
Output:
Using compareTo Difference is : 32 Using compareToIgnoreCase Difference is : 0
Like other compareTo() methods, this method returns negative, positive, and zero. Based on the comparison, it will return 0 if both the strings are equal. It returns a positive or negative value. The result is positive if the first string is lexicographically greater than the second string. Otherwise the result would be negative.
Java String compareTo() Exceptions
The compareTo method in Java can throw two exceptions:
- NullPointerException: If you try to compare a null object with any other object, NullPointerException will be thrown.
- ClassCastException: If you try to compare objects of two different data types, ClassCastException will be thrown.
Java String compareTo(): NullPointerException
The NullPointerException is thrown when a null object invokes the compareTo() method. For example, if you try to compare an object that doesn’t exist, this exception will be thrown.
package com.SoftwareTestingo.JavaBasics; public class CompareToExample5 { public static void main(String[] args) { // Initializing Strings String str1 = null; System.out.println(str1.compareTo("Software")); } }
Output:
Exception in thread "main" java.lang.NullPointerException: Cannot invoke "String.compareTo(String)" because "str1" is null at com.softwaretestingo.string.CompareToExample5.main(CompareToExample5.java:9)
Java String compareTo(): ClassCastException
The ClassCastException is thrown when objects of incompatible types are compared. In the following example, we compare an object of the ArrayList (testing) with a string literal (“Softwaretestingo”).
package com.SoftwareTestingo.JavaBasics; import java.util.ArrayList; import java.util.Collections; class testing { private String name; public testing(String str) { name=str; } } public class CompareToExample6 { public static void main(String[] args) { // Initializing Strings testing obj1=new testing("Manual Testing"); testing obj2=new testing("Automation Testing"); testing obj3=new testing("Java"); ArrayList<testing> al=new ArrayList<testing>(); al.add(obj1); al.add(obj2); al.add(obj3); // performing binary search on the list al Collections.binarySearch(al, "Sehwag", null); } }
Output:
Exception in thread "main" java.lang.ClassCastException: class com.softwaretestingo.string.testing cannot be cast to class java.lang.Comparable (com.softwaretestingo.string.testing is in unnamed module of loader 'app'; java.lang.Comparable is in module java.base of loader 'bootstrap') at java.base/java.util.Collections.indexedBinarySearch(Collections.java:229) at java.base/java.util.Collections.binarySearch(Collections.java:217) at java.base/java.util.Collections.binarySearch(Collections.java:321) at com.softwaretestingo.string.CompareToExample6.main(CompareToExample6.java:29)
Conclusion:
This blog post taught us about the Java String compareTo() method. The compareTo method, like a dictionary, helps compare strings in a specific order. We explored different scenarios using examples and saw how the compareTo method behaves. We also looked at case-sensitive comparisons and how to ignore case sensitivity. Additionally, we discussed exceptions that can occur when using the compareTo() method in Java and how to handle them.