Class In Java: Java Is one of the popular languages, and to start with Java language, one Java learner should have knowledge of Class in Java. So let us Start with:
What Is Class In Java?
A class in Java is a blueprint or a template for creating objects. It defines the structure and behavior of objects created from the class. Think of it as a blueprint for a house: it specifies the layout, design, and features of the house, but it’s not the actual house itself.
In the same way, a Java class defines the attributes (called fields or variables) and methods (functions) that objects of that class will possess. These objects can be considered instances of the class and inherit the properties and behaviors defined by the class.
This means classes are the fundamental concepts in Java’s object-oriented programming paradigm, which allows for code organization, reuse, and modularity.
So, let us start our discussion without delay. In this blog post, we will try to cover Java classes and their importance for developers. We will also learn the syntax, structure of a class, requirements, and behavior of Java classes by the end of this. After reading this, you’ll better understand Java classes and how to use them in your software development.
Class Structure In Java
In Java, a class consists of several essential elements that define the structure and behavior of a Java class. These elements include:
Class Name
A class is identified by its name, which should be a valid Java identifier. It follows the class keyword in the class declaration.
public class MyClass { // Class members and methods go here }
Fields (Variables)
Fields represent the state or properties of objects created from the class. They can be of various data types and may have different access modifiers (public, private, protected, package-private).
// Example of a private field private int myField;
Methods (Functions)
Methods define the behaviors or actions that objects of the class can perform. Methods have a return type (or void for methods that don’t return a value) and can also specify parameters.
We have discussed Variables in Java in detail in a separate post, and you can follow to learn more about variables.
public void myMethod() { // Method code here }
Constructors
Constructors are special methods used to initialize objects when they are created. They have the same name as the class and may have parameters to pass initial values to the object’s fields.
Constructor In Java is a very interesting and important concept for any developer. So, we have tried to cover this in detail in a separate blog post about Constructor in Java.
public MyClass(int initialValue) { // Constructor code here }
Access Modifiers
Access modifiers (e.g., public, private, protected) control the visibility of class members (fields and methods) from outside the class. They enforce encapsulation and access control. For a detailed understanding of Access Modifiers, you can check our detailed post about Access Modifiers by following the mentioned link.
// Example of a private field private int myField;
Inheritance
Java supports class inheritance, allowing one class (subclass or child class) to inherit the properties and methods of another class (superclass or parent class). This promotes code reuse and the creation of class hierarchies.
When you are going to develop a real-time application, inheritance plays an important role, so we try to cover it in a detailed manner in a separate blog. You can check that by clicking on the Inheritance In Java link.
public class ChildClass extends ParentClass { // Additional members and methods specific to ChildClass }
Modifiers
Other modifiers, such as static, final, and abstract, can be applied to fields and methods to modify their behavior and characteristics.
// Example of a static final field public static final double PI = 3.14159;
These elements collectively define the structure and behavior of a class in Java, enabling the creation of objects with consistent properties and behaviors.
Now, let us see the complete structure of a class in Java.
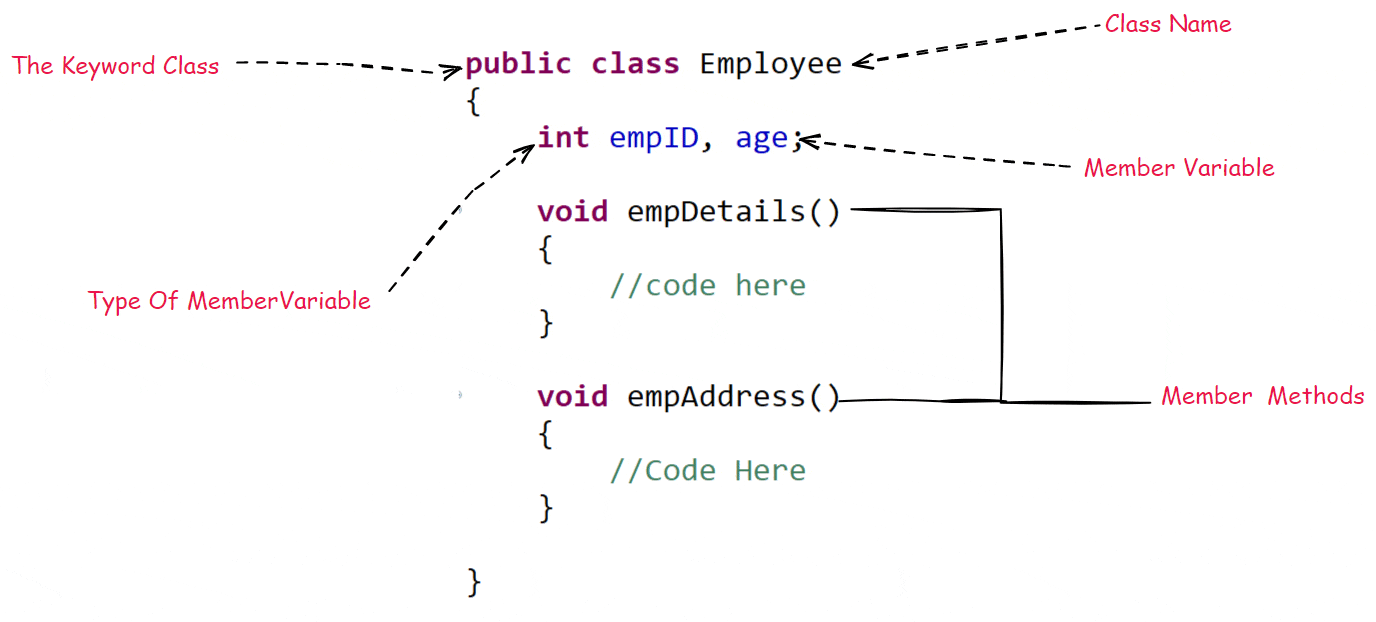
How do you define a class in Java syntax?
In Java programming language, if you want to create a class in Java, you can follow the class below in Java syntax.
[access modifier] class ClassName { // Fields (variables) [access modifier] [static] [final] [data type] fieldName [= initialValue]; // Constructors [access modifier] ClassName([parameters]) { // Constructor code } // Methods [access modifier] [static] [final] [return type] methodName([parameters]) { // Method code [return statement]; // (if the method returns a value) } }
Let’s break down each part of the syntax:
- [access modifier]: This is an optional part of the syntax that specifies the visibility or access level of the class, fields, constructors, or methods. Common access modifiers include public, private, protected, and package-private (no modifier).
- class: The class keyword is used to declare that you are defining a class.
- ClassName: This is the name of the class, which should be a valid Java identifier and follow Java naming conventions. It is the name by which you’ll refer to the class when creating objects.
- Fields (Variables): These are optional and represent the attributes or data members of the class. You can define multiple fields, each with an optional access modifier (public, private, etc.), optional modifiers (static for class-level fields, final for constants), a data type (e.g., int, String), and an optional initial value.
- Constructors: Constructors are used to initialize objects when they are created. They have the same name as the class and can accept parameters for initialization. Constructor code goes inside the curly braces {}.
- Methods: Methods define the behaviors or actions that objects of the class can perform. They include an access modifier, optional modifiers (static for class-level methods, final for unmodifiable methods), a return type (or void if the method doesn’t return a value), a name, and optional parameters. Method code goes inside the curly braces {}.
- [return statement]: If the method returns a value, you can include a return statement to specify the value returned.
Here’s a simple example:
public class MyClass { // Field private int myField; // Constructor public MyClass(int initialValue) { myField = initialValue; } // Method public void printField() { System.out.println("myField: " + myField); } }
Class In Java Example Program
Let us take a complete Class In Java Example Program to understand the class in Java better.
package com.java.self.practice; public class CalculationEx { // instance variables int a; int b; // constructor Of CalculationEx Class to instantiate the variables public CalculationEx (int x, int y) { this.a = x; this.b = y; } // method to add numbers public int add () { int result = a + b; return result; } // method to subtract numbers public int subtract () { int result = a - b; return result; } // method to multiply numbers public int multiply () { int result = a * b; return result; } // method to divide numbers public int divide () { int result = a / b; return result; } public static void main(String[] args) { // creating object of Class CalculationEx c1 = new CalculationEx(20, 10); // Calling All the Methods Of CalculationEx Class System.out.println("Addition is :" + c1.add()); System.out.println("Subtraction is :" + c1.subtract()); System.out.println("Multiplication is :" + c1.multiply()); System.out.println("Division is :" + c1.divide()); } }
Output:
Addition is :30 Subtraction is :10 Multiplication is :200 Division is :2
Conclusion:
Understanding classes in Java is an important concept for anyone looking to develop software in Java language. Classes act as the blueprints for creating objects, defining their properties and behaviors, and enabling key concepts like encapsulation, inheritance, and polymorphism.
If you have any doubts or questions regarding “Class In Java,” please don’t hesitate to ask. Your comments and questions are valuable; we are here to provide clarity and assistance.
Additionally, if you have any suggestions on improving this article or topics you’d like to see covered in more detail, we welcome your feedback in the comment section. Your input will help us create even more informative and helpful content in the future. Thank you for reading!