Break Statement in Java: The break statement is a control statement that allows you to terminate the execution of a loop or switch statement prematurely. When encountered inside a loop or switch, the break statement immediately exits the loop or switch, causing the program to continue executing from the next statement after the loop or switch. The break statement is a powerful tool for controlling the flow of your program and can help you write cleaner and more efficient code.
In this article, we will explore the syntax and usage of the break statement in Java and provide some examples to demonstrate its functionality. Whether you are a beginner or an experienced Java developer, understanding the break statement can help you write more effective code and easily solve complex programming problems.
Break Statement Java Syntax
The Java break statement has a simple syntax that can be used within loops and switch statements. The syntax is as follows:
for(...) { //loop statements break; }
When the break statement is encountered, it immediately terminates the innermost loop or switch statement and causes program control to transfer to the next statement after the loop or switch.
Types of Break Statements
As mentioned above, a break statement is used to come out from the loop or block. There are two forms of break are there, that is:
Let’s see examples to understand the difference between unlabeled Vs. Labeled break statements easily.
Unlabeled Break Statement
Those break statements used without any labels are called unlabeled break statements. Those are written as a simple “break;”. An Example of an unlabeled break statement is:
int i = 1; while (true) { // Cannot exit the loop from here if (i <= 10) { System.out.println(i); i++; } else { break; // Exit the loop } }
We Can use the same in a switch statement, like the below
switch (switch-expression) { case label1: statements; break; case label2: statements; break; default: statements; }
Labeled Break Statement
If we Mention a label name after the break statement, that’s called a labeled break statement. You can refer to the below program for the labeled break statement:
package com.softwaretestingo.ConditionalStatements; public class LabeledBreakStatementEx1 { public static void main(String[] args) { blockLabel: { int i = 10; for(i=0;i<=10;i++) { if (i == 5) { System.out.println("Break Statement Is going to Execute"); break blockLabel; // Exits the block } if (i == 10) { System.out.println("i is not five"); } } } } }
Output:
Break Statement Is going to Execute
Note: The Break Statement terminates the flow of the execution at the labeled break statement, but it doesn’t transfer the flow of execution to that mentioned block name.
One crucial point you must remember when using the labeled break statement is that ” The Label name used with the break statement should be the same block name where the labeled break statement is present; otherwise, you will get an undefined label error message.”
package com.softwaretestingo.ConditionalStatements; public class LabeledBreakStatementEx2 { public static void main(String[] args) { lab1: { int i = 10; if (i == 10) break lab1; // Ok. lab1 can be used here } lab2: { int i = 10; if (i == 10) { // A compile-time error. lab1 cannot be used here because this block is not // associated with the lab1 label. We can use the only lab2 in this block //break lab1; } } } }
You will get the errors below while compiling the program. It cannot locate the label lab1 because it is not in the same block.
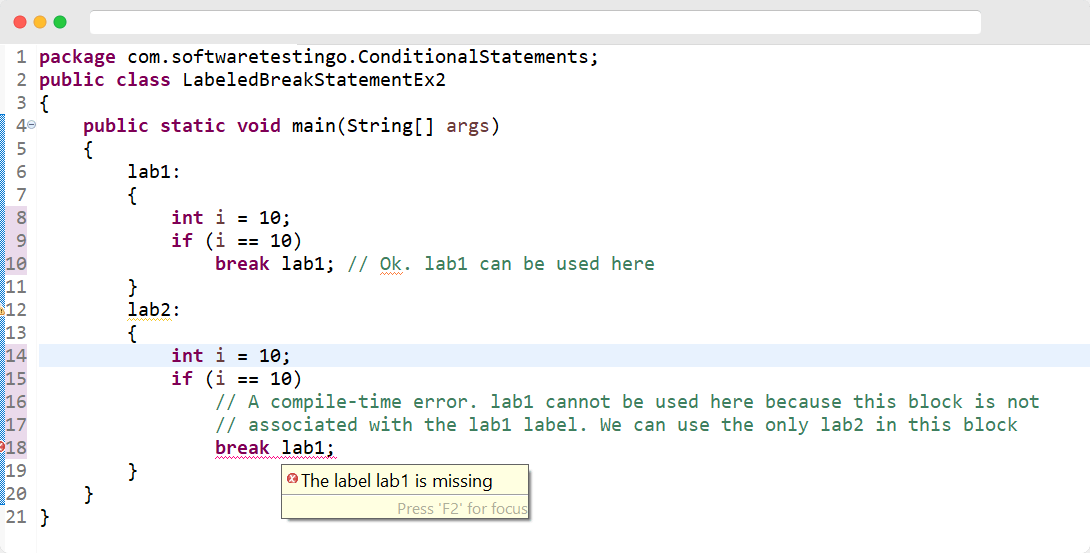
Break Statement With Example
Let’s look at examples of how the break statement can be used in Java.
Using Break in a For Loop
Suppose we have an array of integers and want to find the first element divisible by 3. We can use a for loop and the break statement to accomplish this as follows:
Program: How do you use the break statement in Java?
package com.softwaretestingo.ConditionalStatements; public class BreakEx1 { public static void main(String[] args) { int[] numbers = { 2, 4, 6, 7, 9, 10, 11 }; for (int i = 0; i < numbers.length; i++) { if (numbers[i] % 3 == 0) { System.out.println("Found a number divisible by 3: " + numbers[i]); break; } } } }
Found a number divisible by 3: 6
In this example, we initialize a for loop that iterates over each array element. Inside the loop, we check whether the current element is divisible by 3 using the modulus operator (%
). If the element is divisible by 3, we immediately print a message to the console and then use the break statement to terminate the loop. This ensures that we only find the first element divisible by 3 and do not continue iterating unnecessarily.
Using Break in a While Loop
The break statement can also be used in a while loop. For example, suppose we have a list of names and want to print each name until we encounter the name “John”, at which point we want to stop printing names. We can accomplish this as follows:
Program: Write a Program on How to use the break statement in a while loop.
package com.softwaretestingo.ConditionalStatements; import java.util.Arrays; import java.util.Iterator; import java.util.List; public class BreakEx2 { public static void main(String[] args) { List<String> names = Arrays.asList("Alice", "Bob", "Charlie", "John", "Dave"); Iterator<String> iterator = names.iterator(); while (iterator.hasNext()) { String name = iterator.next(); System.out.println(name); if (name.equals("John")) { System.out.println("Encountered John, stopping."); break; } } } }
Output:
Alice Bob Charlie John Encountered John, stopping.
Overall, the continue statement can be a useful tool for controlling the flow of your Java programs and skipping over certain iterations of loops based on certain conditions. It can help you write more efficient code and solve complex programming problems easily.
Ref: article