Access Modifiers in Java: In Java, access modifiers are keywords used to specify the accessibility of classes, methods, and variables in a program. They are an essential part of object-oriented programming and play a crucial role in enforcing encapsulation, one of the fundamental principles of object-oriented design.
Access modifiers allow developers to control the level of access to a class member, preventing unwanted modification or access to implementation details. Java provides four access modifiers: public, private, protected, and default. Each modifier provides a different level of access to a class member, making it easier to develop, maintain, and debug Java programs.
In this article, we will explore the different access modifiers in Java and their use cases, providing examples to help you better understand their application in Java programming.
What Are Access Modifiers In Java?
Access Modifiers are the keywords in Java that set the visibility of a class, interface, variables, data member, method, and constructor. Access modifiers enforce encapsulation, which is the concept of hiding the implementation details of a class from the outside world. By using access modifiers, we can control the level of access that other classes have to the members of our classes. This helps maintain the integrity of our code and makes it easier to maintain and modify in the future.
Types of Access Modifiers
In Java Programming Language, we have four types of access modifiers, that are:
- Default – Accessible Within the Package
- Private – Accessible within the class
- Public – Accessible anywhere
- Protected – Accessible within the package and all subclasses
Let’s discuss one by one briefly:
Default Access Modifier
When we are writing the Program, if you do not mention any modifiers for a class, interface, variables, data member, method, or constructor, then Java, by default, assigns all those with the default access modifiers. And the scope of default access modifiers is within the package only, which means you can access the default access modifiers declared variables, classes, methods, and constructors within the package alone.
Let’s check a program for a better understanding:
Program 1:
Here we have a class DisplayEx and display() of myPackage with a default access modifier, and we will try to access these classes and methods from another package.
package myPackage; //This class has default access modifer as no modifer is mentioned public class DisplayEx { void display() { System.out.println("Display Method Called of MyPackage Package"); } static public void main(String args[]) { DisplayEx obj=new DisplayEx(); obj.display(); } }
package com.softwaretestingo.AccessModifier; import myPackage.*; public class DefaultAccessModifierEx { public static void main(String[] args) { //Try to access other package default class and method DisplayEx obj=new DisplayEx(); obj.display(); } }
We will get the compile time error message when running the program. If you hover over the errors in the program, you can notice the JVM suggests changing the access like below:
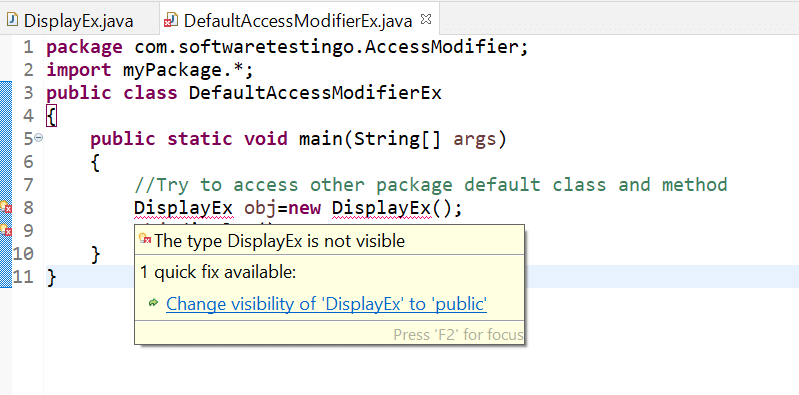
Program 2:
Suppose in myPackage, we have a class DisplayEx which has a display() [default] method, and we try to call the display() method of DisplayEx class from DefaultAccessModifierEx class of the same package.
DisplayEx class of myPackage
package myPackage; //This class has default access modifer as no modifer is mentioned public class DisplayEx { void display() { System.out.println("Display Method Called of MyPackage Package"); } static public void main(String args[]) { DisplayEx obj=new DisplayEx(); obj.display(); } }
DefaultAccessModifierEx class of myPackage
package myPackage; public class DefaultAccessModifierEx { public static void main(String[] args) { DisplayEx obj=new DisplayEx(); obj.display(); } }
As the display method is present in the same package, then with an instance when we try to access the display() method. We will get the following output.
Display Method Called of MyPackage Package
Private Access Modifier
You Can define any data member or method private using the private access modifiers; when we declare a class member with a private access modifier, that can only be accessed within the same class. It has the narrowest scope and is used to hide the implementation details of a class from outside access. Private members cannot be accessed outside the class, even from subclasses of the same package.
Let’s go through with a program for a better understanding
package java_Basics; class ABC { private double num = 100; private int square(int a) { return a*a; } } public class PrivateAccessModifiers_Example { public static void main(String[] args) { ABC obj = new ABC(); System.out.println(obj.num); System.out.println(obj.square(10)); } }
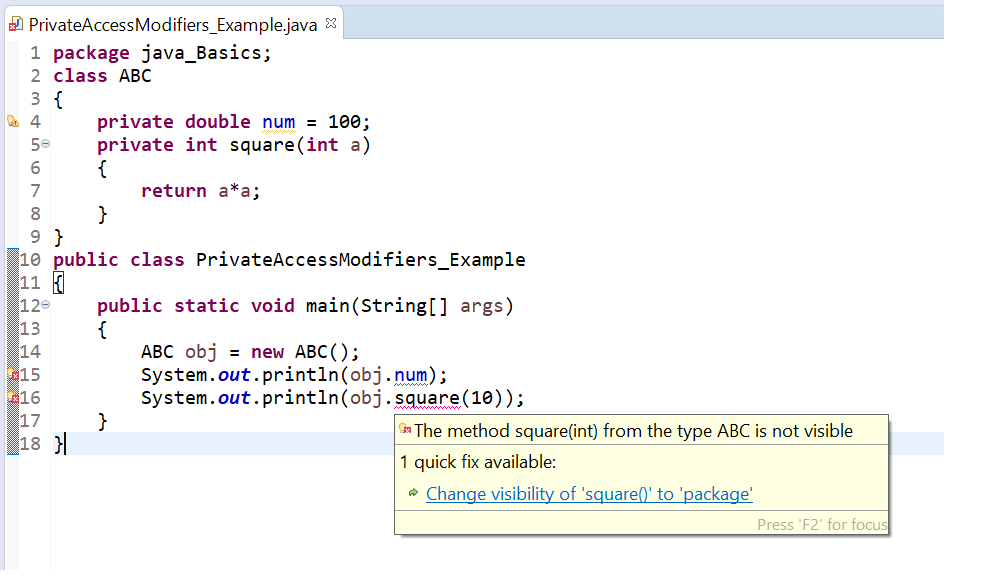
Output:
When you compile the program, you will get a compile-time error
Exception in thread "main" java.lang.Error: Unresolved compilation problems: The field ABC.num is not visible The method square(int) from the type ABC is not visible at java_Basics.PrivateAccessModifiers_Example.main(PrivateAccessModifiers_Example.java:15)
Public Access Modifier
The public access modifier is used to declare a class member that can be accessed anywhere in the program. It has the widest scope and is not restricted to any particular package or class. The public members of a class can be accessed by any other class, regardless of whether they are in the same or different package.
Let’s go through with a program for better understanding.
package myPackage; //This class has default access modifer as no modifer is mentioned public class DisplayEx { public void display() { System.out.println("Display Method Called of MyPackage Package"); } }
package com.softwaretestingo.AccessModifier; import myPackage.*; public class DefaultAccessModifierEx { public static void main(String[] args) { DisplayEx obj=new DisplayEx(); obj.display(); } }
Output:
Display Method Called of MyPackage Package
Important Points:
- If any other programmer uses your class, giving particular data members the most restrictive access level is better.
- Avoid Public access modifiers in the public field except for constants.
Protected Access Modifier
Protected modifiers are specified by the keyword protected. Suppose you assign a data member or method with a protected access modifier. In that case, that data member or method is accessible by the classes of the same package and the subclasses of the different packages but through inheritance only.
The protected and default access modifiers are almost the same, with only one exception: its visibility in subclasses of a separate package and more accessibility than the default access modifier.
We can access the protected access modifier with the data member method and constructor, but we cannot apply it to a class and interface. In the below example, we are trying to explain how the protected access modifier or specifier works. So for this, we have created two packages: myPackage and com.softwaretestingo.AccessModifier.
In myPackage, we have created one class, Class_A, with a public access modifier to be accessed outside of the package; inside that class, we have declared one method display() with a protected access modifier.
package myPackage; public class Class_A { protected void display() { System.out.println("Protected - Class A Display Method Of myPackage Package"); } }
package com.softwaretestingo.AccessModifier; import myPackage.Class_A; public class ProtectedAccessModifierEx extends Class_A { public static void main(String[] args) { ProtectedAccessModifierEx obj=new ProtectedAccessModifierEx(); obj.display(); } }
After running the above program, we will get the below result:
Protected - Class A Display Method Of myPackage Package
Protected Data members and methods are accessible within the same package and different packages through inheritance.
If you try to access the display() method of the Class_A class in a different package without extending to other package classes, you will get the compile time error like the one below.
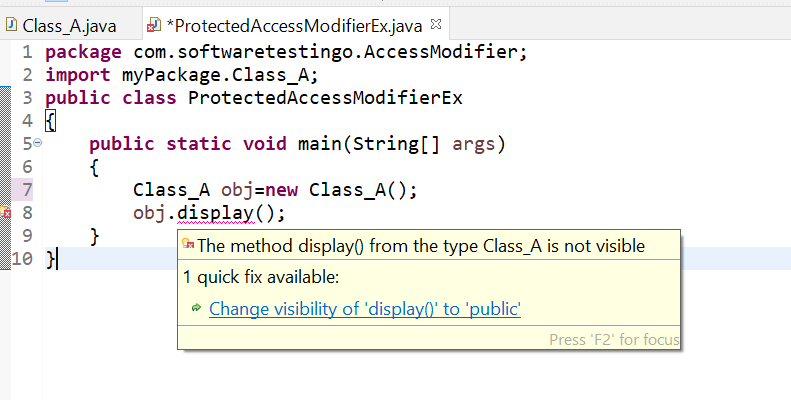
Java Acess Modifier During Method Overriding
If you want to override a method of a subclass, then the subclass method must not be restrictive, which means you can not decrease the scope of the child class method.
package com.SoftwareTestingO.Java.basics; class AccessModifier_Parentclass { protected void parentmethod() { System.out.println("Access Modifier Parent Method"); } } public class Acess_Modifier_During_Method_Overriding extends AccessModifier_Parentclass { private void parentmethod() { System.out.println("Access Modifier Child Method"); } public static void main(String[] args) { } }
As you have seen, Java suggests that you can not reduce the visibility of an inherited method and will also get a compile-time error.
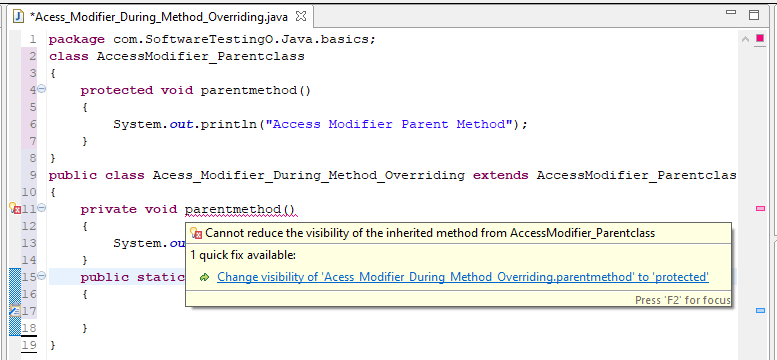
Default | Public | Private | Protected | |
---|---|---|---|---|
Same Class | Yes | Yes | Yes | Yes |
Same Package Subclass | Yes | Yes | No | Yes |
Same Package Non-subclass | Yes | Yes | No | Yes |
Different Package Subclass | No | Yes | No | Yes |
Different Package Non-subclass | No | Yes | No | No |
Conclusion:
Access modifiers in Java provide a crucial mechanism for controlling the visibility and accessibility of class members. Java developers or testers can use access modifiers such as public, private, protected, and default to ensure the code is secure, maintainable, and scalable. Choosing the appropriate access modifier for each member depends on the design and requirements of the class and its usage scenarios.
While public members provide unrestricted access, private members offer maximum encapsulation, and protected members provide limited access within the class hierarchy. Package-private members are useful for restricting access within the package scope. A good understanding of access modifiers is essential for Java developers to write efficient and secure code.
We’re always looking for ways to improve our content and make it more valuable for our readers. If you have any suggestions, please share them in the comments section below.
Ref: article
What are access modifiers in Java?
Access modifiers in Java are the keywords used to control the use of the methods, constructors, fields, and methods in a class.
What is void in Java?
Void in Java is used to specify no return value with the method.
What are the 12 modifiers in Java?
12 Modifiers in Java are public, private, protected, default, final, synchronized, abstract, native, strictfp, transient, and volatile.
Why are Access Specifiers important?
Access specifiers are used for encapsulation purposes and provide different types of restrictions according to the requirement so that we can protect our sensitive information from direct access.
Which Modifiers are not used for the class?
The private and protected modifiers cannot be used for the class because if they are used for the class, due to their restricted scope, JVM will not be able to execute the class because of the external calling system of JVM.