Method hiding in Java is a topic of significant importance within object-oriented programming. It involves using the static keyword in method declarations and presents an interesting case of compile-time binding and polymorphism.
This analytical discussion will discuss the nuances of method hiding, its relationship with method overriding, Variable Hiding in Java, Instance Variable Hiding in Java, Method Hiding Factors (MHF), and its implications in Java programming.
What Is Method Overriding?
Before we start our discussion about method hiding, you should be aware of method overriding. Because without knowledge about method overriding, you can not understand the concept of method hiding.
Method overriding means we declare a variable or method in a subclass with the same name and return type as the method or variable in the superclass. In this case, the method or variable of the superclass will be overridden or replaced by the subclass method and variable. This is called Method overriding.
We have written a detailed blog post on method overriding Java, and you can follow this link for more knowledge.
What is Method Hiding In Java?
Method hiding is a concept in Java related to method overriding and inheritance. When a subclass defines a static method with the same name and method signature as a static method in its superclass, the subclass method is said to hide the superclass method.
This differs from regular method overriding, where the subclass method provides a specific implementation for an instance of a superclass method.
Key points about method hiding:
- Static Methods: Method hiding applies only to static methods in Java. It doesn’t work with instance methods. If a subclass and superclass have a static method with the same signature, the subclass method hides the superclass method.
- Method Signature: For method hiding to occur, the method in the subclass must have the same name and method signature (including the number and types of parameters) as the method in the superclass.
- Access Modifiers: The access modifier of the subclass method cannot be more restrictive than the superclass method. If the superclass method is public, the subclass method must also be public or package-private (default). It cannot be made more private.
- Return Type: In Java 5 and later versions, method hiding allows the return type of the subclass method to be a subtype (subclass) of the return type of the superclass method. This is known as covariant return types.
- Inheritance: Method hiding is closely related to inheritance. It occurs when a subclass inherits a static method from its superclass and defines a static method with the same signature.
- Invoking the Method: The method that gets invoked depends on the reference type at compile time. If you have a reference of the superclass type, but the object is an instance of the subclass, the superclass’s static method will be called (due to method hiding).
These factors collectively determine whether method hiding takes place in a Java program. Method hiding can sometimes lead to unexpected behavior, especially when dealing with class hierarchies and static methods, so it’s essential to understand these factors to avoid potential issues.
To understand the above points and method hiding in a better way, let us go through some of the examples:
Examples 1:
package com.softwaretestingo.methodhiding; class Superclass { //Static Method static void display() { System.out.println("Static method in Superclass"); } } class Subclass extends Superclass { //Static Method static void display() { System.out.println("Static method in Subclass"); } } public class MethodHidingEx1 { public static void main(String[] args) { // Superclass Method Will Be Called Superclass supobj=new Superclass(); supobj.display(); // Subclass Method Will Be Called Subclass subobj=new Subclass(); subobj.display(); /* * In Method Hiding - Method Execution will be based on Reference type which * is Superclass, So super Class method will be called */ Superclass obj=new Subclass(); obj.display(); } }
The Output Will be:
Static method in Superclass Static method in Subclass Static method in Superclass
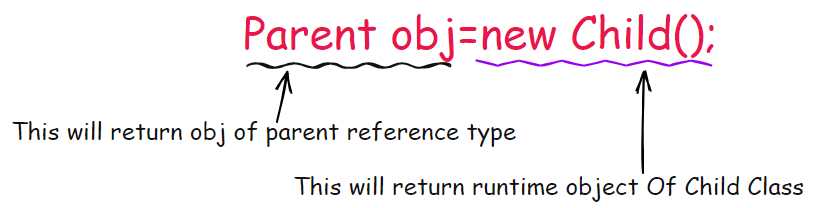
- You can not override a static method with a non-static method and vice versa. If you try this, you will get a compile-time error.
- But you can override a static method with another static method, and this concept is called the Method Hiding Concept. That means the overriding concept is only applicable to non-static methods.
- In method hiding, method execution is always taken care of by the compiler based on the reference type.
- In method overriding, method execution is always taken care of by JVM based on the runtime object.
As we are discussing something very close to method overriding, that’s why it’s better if we take an example of a nonstatic method and see the output. This way, after seeing the output, we can compare method hiding and method overriding easily and understand the method hiding concept more clearly.
package com.softwaretestingo.methodhiding; class Parent { //Static Method void display() { System.out.println("Parent Class Method Called"); } } class Child extends Parent { //Static Method void display() { System.out.println("Child Class Method Called"); } } public class MethodOverridingEx { public static void main(String[] args) { // Superclass Method Will Be Called Parent supobj=new Parent(); supobj.display(); // Subclass Method Will Be Called Child subobj=new Child(); subobj.display(); /* * In Method Overriding - Method Execution will be based on Runtime Object(new Child()) which is * Child Class, So Child Class method will be called */ Parent obj=new Child(); obj.display(); } }
Output:
Parent Class Method Called Child Class Method Called Child Class Method Called
Method Hiding vs. Method Overriding
Here are some of the basic differences:
Aspect | Method Hiding | Method Overriding |
---|---|---|
Type of Methods | Static methods | Instance methods |
Inheritance | It is typically associated with the inheritance of static methods in a subclass from a superclass. | Associated with the inheritance of instance methods in a subclass from a superclass. |
Method Signature | A Subclass defines a static method with the same name and method signature as the static method in the superclass. | A subclass provides a specific implementation for an instance method with the same name and method signature as the superclass method. |
Access Modifiers | The access modifier of the subclass method cannot be more restrictive than the superclass method. | The subclass method can have the same or more permissive access modifier (e.g., from protected to public) compared to the superclass method. |
Return Type | The return type of the subclass method can be a subtype (covariant return type) of the return type of the superclass method. | The return type must be the same or a subtype of the return type of the superclass method (covariant return type is allowed). |
Polymorphism | Static methods are not polymorphic. The method invoked is determined at compile time-based on the reference type. | Instance methods are polymorphic. The method invoked is determined at runtime based on the actual object type. |
Usage | Less common, primarily used with static methods. | More commonly, it provides specific implementations of instance methods in subclasses. |
Example | class Superclass { static void display() { … } } class Subclass extends Superclass { static void display() { … } } | class Animal { void makeSound() { … } } class Dog extends Animal { void makeSound() { … } } |
In short, if we describe method hiding as specific to static methods and related to the inheritance of static methods with the same name and signature in a subclass, Method overriding, on the other hand, applies to instance methods and allows a subclass to provide its implementation for a method inherited from a superclass.
Conclusion:
Understanding method hiding is important for Java developers, as it can impact the behavior of their programs. When a subclass hides a static method from its superclass, it may lead to unexpected results if the developer is not aware of this behavior. Therefore, developers should exercise caution when using static methods in class hierarchies and be mindful of the potential for method hiding.
If you have any doubts or questions about the method hiding in Java, please feel free to comment below. Additionally, if you have any suggestions to improve this article or topics you’d like to see covered, we encourage you to share your feedback in the comment section. Your input is valuable in helping us provide informative and engaging content.