Checked Exception VS Unchecked Exception In Java: Exceptions are crucial in handling errors and unexpected situations in Java programming. Understanding the distinction between checked and unchecked exceptions is important for writing robust and maintainable code.
In this blog post, we will try to discuss Checked Exception and Unchecked Exceptions in detail; along with different types of Checked Exception and Unchecked Exception, we will explore the differences, use cases, and implications of checked and unchecked exceptions in Java.
What is Exception?
An exception in Java is a condition or event that disrupts the normal flow of a program’s execution. It represents an error or unexpected situation that can occur during runtime. Exceptions can be caused by various factors, such as invalid input, file not found, or division by zero.
Checked Exception VS Unchecked Exception In Java
Based on Behavior, the exception is divided into two types: Checked Exception and unchecked Exception. If you want to know more about both exceptions, you can check our Types of Exceptions in the Java blog post, where we have discussed them in detail.
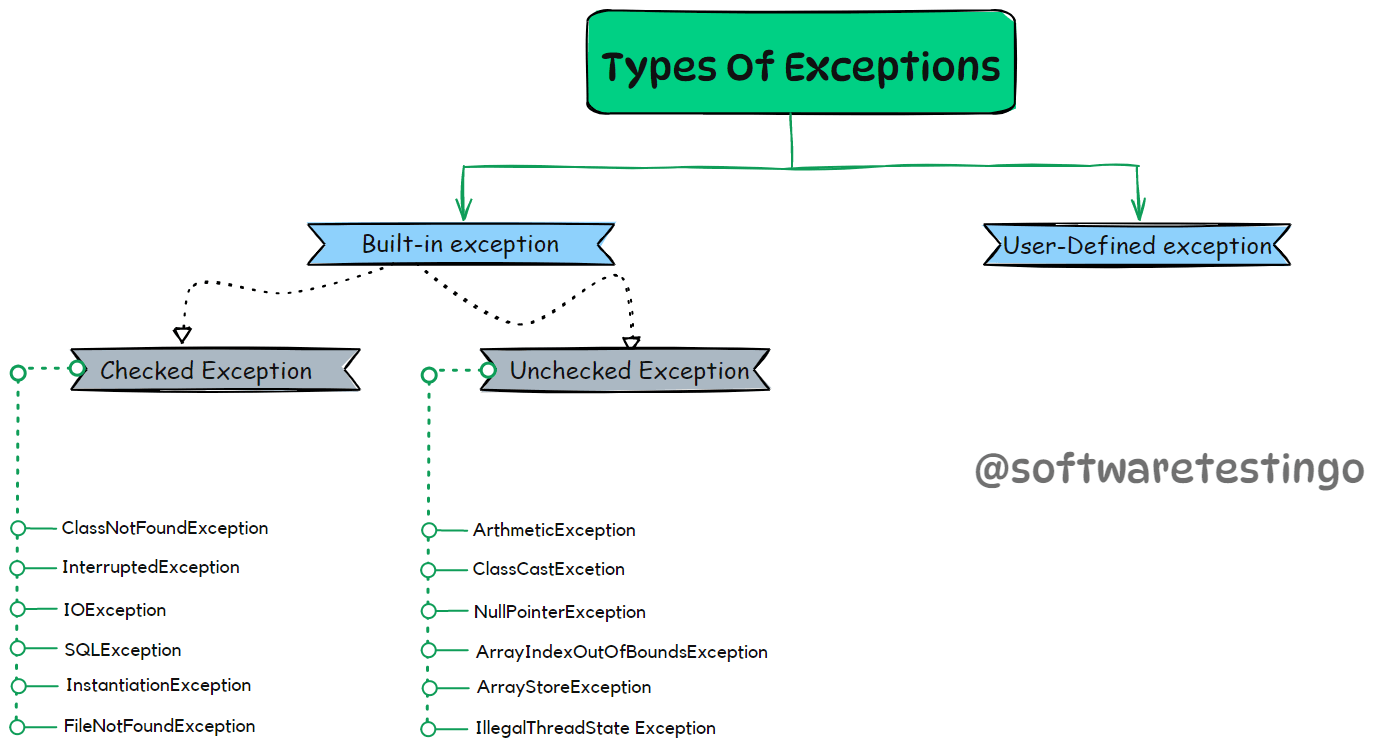
Checked Exception
- An exception that is checked at compile-time during compilation is known as Checked Exception.
- Alternate definition: any line of code that could throw an exception, and if it is raised to handle during compilation, is said to be checked exception.
- For example, accessing a file from a remote location could throw a file not found an exception.
- The programmer is responsible for handling the checked exception for successful compilation.
- If an exception is raised during execution, the respective handling code will be executed.
- Note: if it isn’t handled, then the program will throw a compile-time error
- Example: IOException, FileNotFoundException, InterruptedException, SQLException, etc
- Except for Runtime exception & its child classes and error & its child classes, all other exceptions fall under the category of Checked Exception.
CheckedException.java
import java.io.BufferedReader; import java.io.FileReader; public class CheckedException { public static void main(String[] args) { FileReader fileReader = new FileReader("F:\\BenchRes.txt"); BufferedReader bufferedReader = new BufferedReader(fileReader); // logic for reading } }
Output:
Compile-time error: Unhandled exception type FileNotFoundException
Checked exception screen-capture from Eclipse IDE:
Unchecked Exception
- An exception that is NOT checked at compile-time is known as Unchecked Exception.
- Alternate definition: any line of code that could throw an exception at runtime is said to be an unchecked exception
- An unchecked exception is because of a programming error
- For example, accessing out of index position to assign some value during execution could throw an exception at runtime
- So, the programmer is responsible for handling the unchecked exception by providing an alternate solution in the exception handling code.
- Note: if it isn’t handled properly, then the program will terminate abnormally at runtime
- Example: Runtime exception & its child classes and error & its child classes are examples of Unchecked Exception
- Like ArithmeticException, NullPointerException, NumberFormatException, ArrayIndexOutOfBoundsException, StatckOverflowError, etc
UncheckedException.java
public class UncheckedException { public static void main(String[] args) { char[] ch = new char[4]; ch[7] = 'B'; System.out.println(ch); } }
Exception in thread "main" java.lang. ArrayIndexOutOfBoundsException: 7 at in.bench.resources.exception.handling. UncheckedException.main(UncheckedException.java:8)
Misconception On Checked Exception VS Unchecked Exception In Java
- Sometimes, a checked exception is called a compile-time exception, and unchecked exceptions are called runtime exceptions.
- But this is misleading because every exception (whether it is checked or unchecked) occurs/raised only at the runtime, i.e., during program execution-only
- Reason: during compilation, the checked exception is caught and raises a compile-time error, due to which the programmer has to handle the exception by providing either try-catch blocks or using the throws keyword
- Whereas unchecked exception isn’t caught during compilation, it raises an exception during execution because of a programming error.
Checked And Unchecked Exception Handling In Java
Now, we will discuss the difference between Checked And Unchecked Exception Handling In Java.
Aspect | Checked Exceptions | Unchecked Exceptions |
---|---|---|
Handling Requirement | It must be explicitly handled using a try-catch block or declared in a throws clause. | It is not required to be explicitly handled; it can be caught but often left unhandled. |
Subclass of Exception | Subclasses of java.lang.Exception excluding java.lang.RuntimeException. | Subclasses of java.lang.RuntimeException. |
Checked at Compile-Time | Yes, checked exceptions are detected by the compiler during code compilation. | No, unchecked exceptions are not checked by the compiler. |
Examples | IOException, SQLException, ClassNotFoundException. | NullPointerException, ArrayIndexOutOfBoundsException, IllegalArgumentException. |
Use Cases | Typically used for recoverable scenarios, such as file I/O or database connections. | Used for programming errors and other unrecoverable issues. |
Forced Exception Handling | Enforces explicit error handling, which can make code more robust but also more verbose. | It does not enforce explicit handling, which can simplify code but may lead to unexpected runtime failures. |
Documentation Requirement | It should be documented to indicate potential issues and help other developers understand error-handling requirements. | It should also be documented for clarity and understanding, even though explicit handling is not enforced. |
Examples of When to Use | Handling database connection errors, reading from a file, and network communication issues. | Detecting null references, out-of-bounds array access, and programming logic errors. |
Impact on Code Readability | The code may be more verbose due to required try-catch blocks or throw declarations. | It can simplify code by not requiring explicit handling, but care is needed to prevent unexpected failures. |
Conclusion:
Understanding the difference between Checked Exceptions and Unchecked Exceptions in Java is highly required for writing robust and reliable code. Checked exceptions, enforced by the compiler, are ideal for handling anticipated and recoverable errors, promoting robust error management.
On the other hand, unchecked exceptions, which are not enforced by the compiler, are typically used for unexpected runtime conditions and programming errors. While they offer flexibility, they require careful coding to prevent unexpected crashes.
Please comment below if you have any doubts or need further clarification on Java exceptions or other programming topics. Additionally, if you found this information helpful, don’t hesitate to share it with your friends and colleagues to help them enhance their Java programming skills. Your feedback and sharing can contribute to a more informed and skilled developer community.