Java Tokens: In Java, a token is the smallest unit of a Java program’s source code, which is meaningful to the Java compiler. Java tokens represent various program elements, such as keywords, identifiers, literals, operators, and punctuation.
This article will discuss the Java tokens, explore their types, and learn how to work with them.
What is a Java Token?
In programming language, a token refers to the smallest indivisible unit or element of a program’s source code that holds a meaningful purpose to the programming language or compiler.
In other words, tokens are fundamental building blocks that help the compiler or interpreter understand and process the code correctly.
Java Tokens Types
In Java, several types of tokens are available, each serving a unique purpose in the code. Let’s explore these token types and provide examples for better understanding:
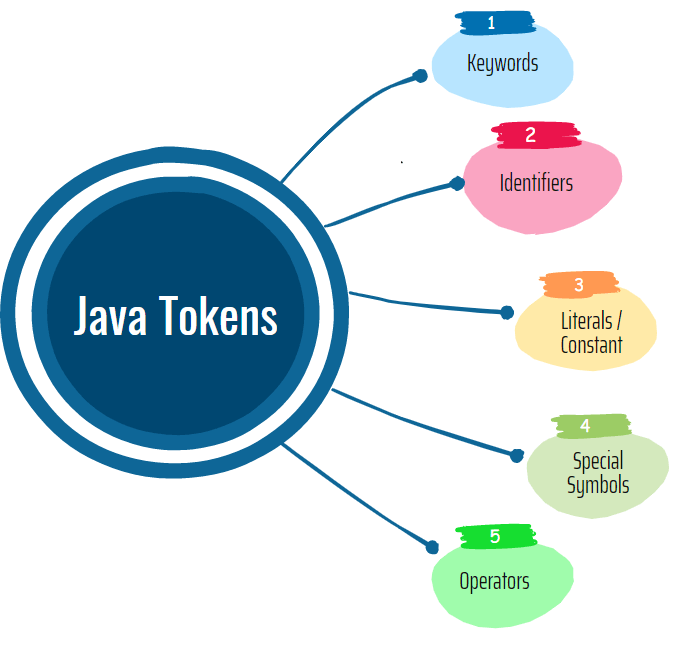
Keywords
In Java, a keyword is a specific token representing a reserved word with a predefined and special meaning within the Java programming language. Keywords cannot be used as identifiers (variable, method, or class names) because they are already reserved for specific language features and functionality.
Examples of Keywords are:
Java keywords are case-sensitive, meaning that writing them with different letter cases (e.g., “public” vs. “Public”) will result in different meanings.
Identifiers
Identifier tokens give names to various program elements such as variables, methods, classes, packages, and interfaces. These Identifiers are user-defined, meaning that programmers create them to represent specific entities within their Java programs.
Here are some key rules and characteristics of Java identifiers:
Naming Rules:
- After the initial character, identifiers can include letters, digits (0-9), underscores, and dollar signs.
- Identifiers must start with a letter (a-z or A-Z), an underscore (_), or a dollar sign ($).
- Java is case-sensitive, so uppercase and lowercase letters are considered distinct. For example, “myVariable” and “myvariable” are different identifiers.
Examples of Identifiers:
- Variable names: count, totalAmount, myVar.
- Method names: calculateTotal, getAge.
- Class names: Person, Car, MyClass.
- Package names: com.example.myapp, org.utils.tools.
Reserved Words:
- Identifiers cannot be Java-reserved words or keywords, as they have predefined meanings in the language. For example, you cannot use “class” or “public” as identifiers.
CamelCase Convention:
- It’s a common convention in Java to use camelCase for naming identifiers, especially for variables and methods. CamelCase involves starting each word with a capital letter (except the first word) without spaces or underscores. For example, myVariableName is in camelCase.
Package Names:
- Package names are a special form of identifier used to organize and namespace classes. They follow a reverse domain convention, like com.example.myapp.
In summary, identifiers in Java are user-defined names used to identify and distinguish program elements. Adhering to naming rules and conventions for identifiers is essential for writing readable and maintainable Java code.
Constants
In Java, literals or constants are tokens representing fixed values or data that do not change during the execution of a program. They are used to initialize variables or provide specific values directly in code. Java supports various types of literals, each designed to represent a different type of value:
Integer Literals:
- Integer literals represent whole numbers.
- Examples: 42, 0xFF (hexadecimal), 1234567890.
Floating-Point Literals:
- Floating-point literals represent decimal numbers with a fractional part.
- Examples: 3.14, 1.0e-5 (scientific notation), 0.0.
Character Literals:
- Character literals represent a single character enclosed in single quotes (”).
- Examples: ‘A’, ‘7’, ‘\n’ (newline).
String Literals:
- String literals represent sequences of characters enclosed in double quotes (“”).
- Examples: “Hello, Java!”, “12345”, “” (empty string).
Boolean Literals:
- Boolean literals represent the two possible boolean values: true and false.
Null Literal:
- The null literal is a special literal that references no object.
- Example: null.
Literals and constants play a crucial role in Java programs. They provide initial values for variables, allow you to hard-code specific data, and make code more self-explanatory.
Using the appropriate type of literal for the data you intend to represent is important, ensuring correctness and readability in your Java code.
Special Symbols
In Java, special symbols refer to characters or symbols used for various purposes in the Java Program or code, such as delimiters, operators, and separators. These symbols serve to structure and control the flow of a Java program.
Here are some common categories of special symbols in Java tokens:
Punctuation Symbols: These symbols are used for separation, grouping, and delimitation. Here are some examples of punctuation symbols.
Semicolon (;) | Terminates statements. |
Comma (,) | Separates items in a list. |
Period (.) | Accesses members of a class or object. |
Parentheses (( and )) | Encloses method parameters and expressions. |
Curly Braces ({ and }) | Define code blocks. |
Square Brackets ([ and ]) | Used for arrays. |
Operators: Operators are symbols that perform specific operations on operands.
Arithmetic Operators | +, -, *, / |
Relational Operators | <, >, <=, >=, ==, != |
Logical Operators | &&, ||, ! |
Assignment Operators | =, +=, -= |
Comment Symbols:
- While not technically tokens, comment symbols are essential for code documentation.
- Single-line comment: // precedes a single-line comment.
- Multi-line comment: /* and */ enclose a block of comments.
Other Symbols:
- Java includes other symbols and special characters for specific purposes, such as the @ symbol in annotations or the:: operator in method references.
Special symbols are an integral part of the Java programming language, helping to define code structure, logic, and functionality. Proper use and understanding of these symbols are essential for effectively writing and comprehending Java code.
Operators
The Java operators are symbols or characters used to perform specific operations on operands (values or variables). Operators allow you to manipulate data, make comparisons, perform calculations, and control the flow of your Java programs.
Java supports various operators, categorized into different types based on their functions. Here are some common types of operators in Java:
Arithmetic Operators:
- Arithmetic operators perform mathematical operations like addition, subtraction, multiplication, and division.
- Examples: + (addition), – (subtraction), * (multiplication), / (division), % (modulus).
Relational Operators:
- Relational operators compare values and return a boolean result indicating the relationship between the operands.
- Examples: < (less than), > (greater than), <= (less than or equal to), >= (greater than or equal to), == (equal to), != (not equal to).
Logical Operators:
- Logical operators perform logical operations on boolean values or expressions.
- Examples: && (logical AND), || (logical OR), ! (logical NOT).
Assignment Operators:
- Assignment operators are used to assign values to variables.
- Examples: = (assignment), += (addition assignment), -= (subtraction assignment), *= (multiplication assignment), /= (division assignment).
Increment and Decrement Operators:
- These operators are used to increase or decrease the value of a variable by 1.
- Examples: ++ (increment), — (decrement).
Bitwise Operators:
- Bitwise operators perform bit-level operations on integers.
- Examples: & (bitwise AND), | (bitwise OR), ^ (bitwise XOR), << (left shift), >> (right shift), >>> (unsigned right shift).
Conditional (Ternary) Operator:
- The conditional operator (? : ) is a shorthand way of writing an if-else statement.
- Example: result = (x > y) ? x: y; assigns the value of x to result if x is greater than y. Otherwise, it assigns the value of y.
Instanceof Operator:
- The instanceof operator tests if an object is an instance of a particular class or interface.
- Example: if (obj instanceof MyClass) { /* do something */ }.
Operators are fundamental to Java programming, enabling you to perform various tasks and operations in your code. Understanding operators’ use effectively is essential for writing functional and efficient Java programs.
Conclusion
Understanding Java tokens is essential for writing and debugging Java programs. They form the foundation upon which your code is built, and a firm grasp of them is crucial for every Java developer. Please comment below if you have any doubts about Java tokens or suggestions to improve this article.