In this post, we will discuss one more type of Java operator called the ternary operator. The Java ternary operator functions are very similar to the Java If Statement. This ternary operator validates a condition; after evaluating it, it returns true or false. Based on the evaluation result, it will return the second or third operand.
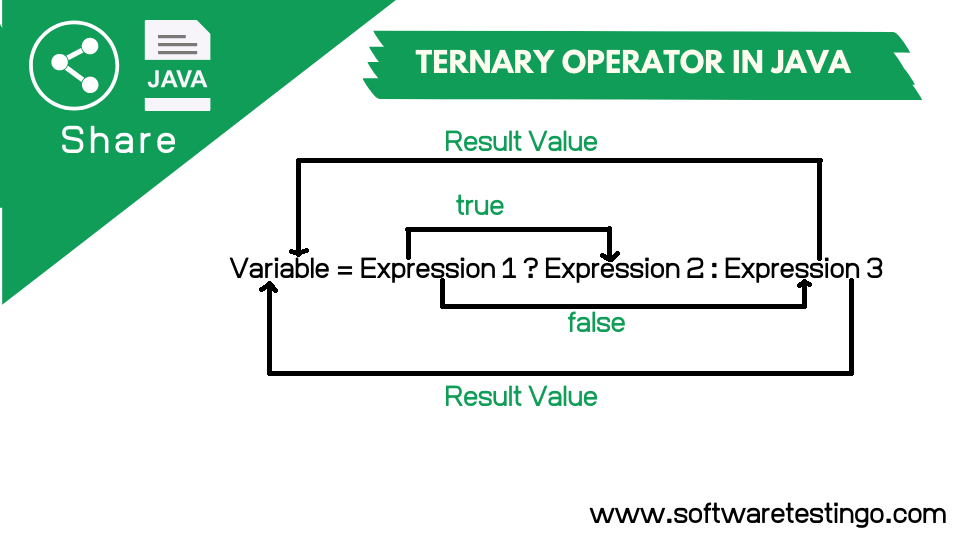
Ternary Operator In Java
Syntax of Java ternary operator is:
result = testStatement ? value1 : value2;
In the above syntax, if the test statement is true, the value1 is assigned to the result. Otherwise, the value2 is assigned to the result.
package com.softwaretestingo.operators; import java.util.Scanner; public class TernaryOperatorEx { public static void main(String[] args) { int num1, num2, num3, result, temp; /* Scanner is used for getting user input. * The nextInt() method of scanner reads the * integer entered by user. */ Scanner scanner = new Scanner(System.in); System.out.println("Enter First Number:"); num1 = scanner.nextInt(); System.out.println("Enter Second Number:"); num2 = scanner.nextInt(); System.out.println("Enter Third Number:"); num3 = scanner.nextInt(); scanner.close(); /* In first step we are comparing only num1 and * num2 and storing the largest number into the * temp variable and then comparing the temp and * num3 to get final result. */ temp = num1>num2 ? num1:num2; result = num3>temp ? num3:temp; System.out.println("Largest Number is:"+result); } }
Output:
Enter First Number: 10 Enter Second Number: 20 Enter Third Number: 30 Largest Number is:30