Hey there! We’ve often talked about Strings in Java in our articles and used them in several Java programs. A String is just a collection of characters. In Java, Strings are important because they can be manipulated in many ways. As a Java developer, learning how to manipulate Strings to create effective and efficient code is essential.
In this article, we will introduce you to String manipulation in Java with the StringBuffer class, which can help you manipulate Strings in many ways. We’ll also discuss various methods and constructors of the Java StringBuffer class.
Post On: | StringBuffer Class In Java |
Post Type: | Java Tutorials |
Published On: | www.softwaretestingo.com |
Applicable For: | Freshers & Experience |
Get Updates: | SoftwareTestingo Telegram Group |
What is StringBuffer in Java?
In Java, strings are immutable. This means that its value cannot be changed once you create a string object. If we want to alter or assign a new value to the string, it is not possible. It creates a string object and then assigns the value. Here, the StringBuffer class comes into play. With the help of StringBuffer, we can modify objects within a string buffer, allowing us to change our initial string.
Let us take some examples so we can easily understand the use case of StringBuffer. Consider the scenario of having a string “SoftwareTestingo” stored in a String variable and the intention of adding multiple characters to it. However, we face a limitation in this case. Whenever characters are added to the String, a new memory allocation is created for the modified String without modifying the original String itself.
This results in significant memory wastage. On the other hand, when the String is stored in a StringBuffer class, no new memory allocation occurs. StringBuffer objects are similar to String objects but can be modified, enabling manipulation operations on the StringBuffer. This characteristic of the StringBuffer in Java is called “mutable.”
Due to its advantageous characteristics, the StringBuffer class is commonly used for various String operations. One notable advantage is that StringBuffer objects are thread-safe, meaning multiple threads cannot access them simultaneously in Java. In simpler terms, it implies that we cannot perform multiple operations simultaneously on a StringBuffer object.
Syntax of StringBuffer class
So, the Object class is considered a parent of all classes. The StringBuffer class inherits two interfaces from the Object class – CharSequence and Serializable. This makes it a powerful and versatile tool for developers.
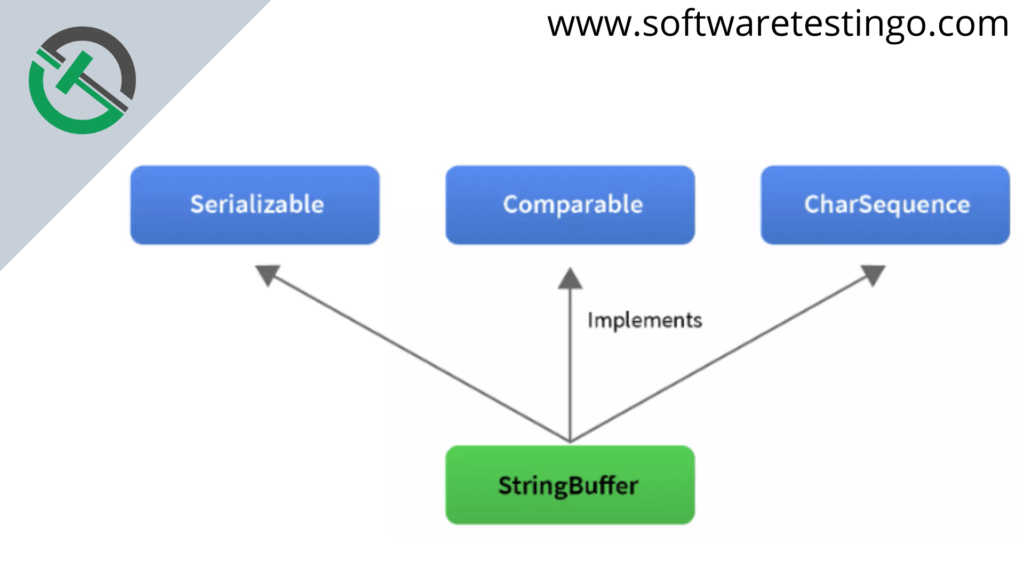
public final class StringBuffer extends Object implements Serializable, CharSequence
StringBuffer Class Declaration
StringBuffer is declared using the new keyword, just like all other classes in Java. However, StringBuffer is not a primitive class, so you cannot directly assign a value. For example, this statement is invalid: StringBuffer str = value.
StringBuffer<object_name> = new StringBuffer();
With the object_name reference, we can access various in-built methods of the StringBuffer class.
In Java, we have mainly 2 attributes are there. That is:
- Constructor
- Method
Constructors in the StringBuffer Class
Different types of constructors in StringBuffer can help you convert an ordinary string or character into a StringBuffer and configure properties such as size. Let us try to discuss the constructors of StringBuffer in Java:
StringBuffer():
This constructor creates a StringBuffer object with an initial capacity of 16 bytes. Capacity refers to the maximum number of elements that the StringBuffer can store. This constructor doesn’t require any parameters.
StringBuffer ob = new StringBuffer();
StringBuffer(int capacity):
This constructor is used to initialize the initial size of the StringBuffer. The capacity parameter indicates how many characters the StringBuffer can store before resizing itself. This constructor takes one parameter, i.e., the capacity of the StringBuffer.
//Initialising the StringBuffer capacity to the 10 bytes StringBuffer ob = new StringBuffer(10);
StringBuffer(String):
This constructor can convert a String object into a StringBuffer object. The default capacity of the new StringBuffer will be 16 bytes.
If the capacity of StringBuffer gets full after adding an extra String, the new capacity of StringBuffer will be: (previousCapacity+1)*2
If the initial capacity is 10, then after full, the updated capacity becomes (10+1)*2=22
Methods of StringBuffer Class in Java:
If you’re looking to perform different operations on a StringBuffer in Java, various built-in methods can help. Let’s take a look at each method one by one.
StringBuffer: append() method
This method can be used to append a new sequence of characters to an existing character sequence in the StringBuffer class.
Program: Write a Java Program to append a string into another String.
package com.softwaretestingo.stringbuffer; public class StringBufferAppendEx { public static void main(String[] args) { StringBuffer str = new StringBuffer("Software"); // appends a string in the previously defined string. str.append("Testingo"); System.out.println("After Append the Value: "+str); } }
Output:
After Append the Value: SoftwareTestingo
Adding a value to an empty StringBuffer will not cause any errors.
StringBuffer: length() method
A StringBuffer sequence’s length can be found using the method .length(). Length is defined as the number of elements in the StringBuffer sequence.
Program: Write a Java Program to print the length of the StringBuffer object.
package com.softwaretestingo.stringbuffer; public class StringBufferLengthEx { public static void main(String[] args) { StringBuffer str = new StringBuffer("SoftwareTestingo"); int len=str.length(); System.out.println("The Length Of StringBuffer Object: "+len); } }
Output:
The Length Of StringBuffer Object: 16
StringBuffer: capacity() method
This method is used to find the initial capacity of the StringBuffer object. The default capacity of the StringBuffer class is 16 bytes, but it can be increased as needed.
Program: Write a Java Program to Find the Capacity of StringBuffer Object.
package com.softwaretestingo.stringbuffer; public class StringBufferCapacityEx { public static void main(String[] args) { StringBuffer str = new StringBuffer("SoftwareTestingo"); int len=str.capacity(); System.out.println("The Capacity Of String Buffer Object: "+len); } }
Output:
The Capacity Of String Buffer Object: 32
StringBuffer: insert() method
Use the insert method to add a string at a particular indexed position. This method takes two arguments: the index where you want to insert the new string and the string itself.
Program: Write a Java Program to insert a string into another String.
package com.softwaretestingo.stringbuffer; public class StringBufferInsertEx { public static void main(String[] args) { StringBuffer str = new StringBuffer("Welcome"); System.out.println(str); str.insert(7, " to "); System.out.println(str); str.insert(10, " SoftwareTestingo "); System.out.println(str); } }
Output:
Welcome Welcome to Welcome to SoftwareTestingo
StringBuffer: reverse() method
The reverse() method of the StringBuffer class reverses all the characters in the object and returns a reversed String object.
Program: Write a Java Program to reverse a string.
package com.softwaretestingo.stringbuffer; public class StringBufferReverseEx { public static void main(String[] args) { StringBuffer str = new StringBuffer("SoftwareTestingo"); System.out.println("Original String: " + str); str.reverse(); System.out.println("Reversed String: " + str); } }
Output:
Original String: SoftwareTestingo Reversed String: ognitseTerawtfoS
StringBuffer: delete() method
The delete() method of the StringBuffer class deletes a sequence of characters from the StringBuffer object, taking two arguments: the starting index of the string to delete and the last index up to which to delete.
Program: Write a Java Program to delete the string.
package com.softwaretestingo.stringbuffer; public class StringBufferDeleteEx { public static void main(String[] args) { StringBuffer str = new StringBuffer("Welcome To SoftwareTestingo"); System.out.println("Original String: " + str); str.delete(8,10); System.out.println("After Delete String: " + str); } }
Output:
Original String: Welcome To SoftwareTestingo After Delete String: Welcome SoftwareTestingo
StringBuffer deleteCharAt() Method
This method deletes the character at a given index from a StringBuffer sequence.
Program: Write a Java Program to delete specific characters from a string.
package com.softwaretestingo.stringbuffer; public class StringBufferDeleteEx2 { public static void main(String[] args) { StringBuffer str = new StringBuffer("Welcome To SoftwareTestingo"); System.out.println("Original String: " + str); str.deleteCharAt(8); System.out.println("After Delete Character at 8 position: " + str); } }
Output:
Original String: Welcome To SoftwareTestingo After Delete Character at 8 position: Welcome o SoftwareTestingo
Key Points:
- Java StringBuffer class is used to manipulate string objects.
- java.lang.StringBuffer class extends the Object class.
- The StringBuffer class is implemented interfaces: Serializable, Appendable, and CharSequence.
- The StringBuffer class inherits certain methods from the Object class, including clone, equals, finalize, getClass, hashCode, notify, and notifyAll.
- StringBuffer is a thread-safe mutable sequence of characters.
- Due to synchronization, it is recommended to use StringBuffer for string manipulation in a multithreaded environment.
- Common operations provided by StringBuffer include append, insert, reverse, and delete.
- Java 1.5 introduced the StringBuilder class, which is similar to StringBuffer but lacks thread safety. StringBuilder is suitable for string manipulation in a single-threaded environment.
Conclusion
The Java StringBuffer class is useful for performing various operations on strings, such as concatenation, insertion, deletion, and reversal. This allows for greater control over how strings are manipulated to achieve the desired results.
This article provides an overview of the StringBuffer class and how it can be used. If you still have any questions, let us know in the comment section, and we will be happy to help you.