Do you want to know the term “String pool in Java?” Well, if you are not aware of this, then you have come to the right place. String Pool in Java is a group of strings stored in Java’s heap memory. Let us explore this concept of the Java String pool a little further and understand it more clearly.
Introduction
In Java Heap memory, there is an area called String Pool or String Constant Pool where the JVM stores the string literals. If the String literal exists in the pool, a reference to the pooled instance is returned. If the String does not exist in the pool, a new string instance is created and placed into the pool.
Post On: | String Pool in Java |
Post Type: | Java Tutorials |
Published On: | www.softwaretestingo.com |
Applicable For: | Freshers & Experience |
Get Updates: | SoftwareTestingo Telegram Group |
What is String Pool in Java?
A string is a set of characters always enclosed in double quotes. A string can also be defined alternatively as an array of characters. In addition, strings in Java are treated as objects. This means they have certain properties and methods that can be invoked.
As you probably know, strings are immutable in nature. That means strings have a constant value, and even if they are altered, a new object is created instead of reflecting the alterations in the original string. This immutability is achieved through the String Pool.
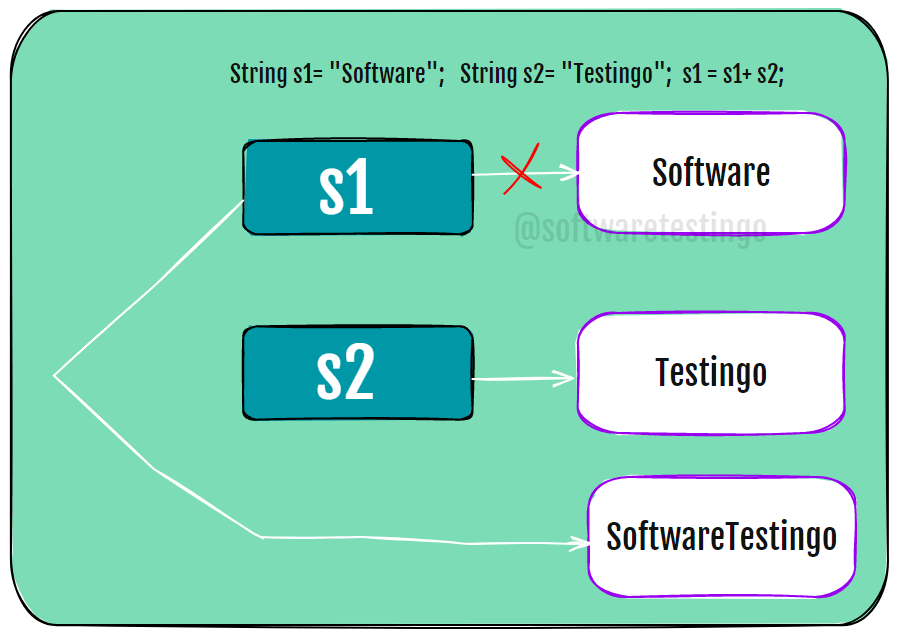
Java Strings are created and stored in the String Pool, a special storage space in Java heap memory. Other names for the String Pool include the String Constant Pool or even the String Intern Pool.
You may notice we are mentioning strings are created in the heap memory, so one question that may come into your mind is, “Why are strings stored in heap memory?” This is a great question; we will help clear up this confusion.
When we talk about memory in programming, there are two types:
- Stack memory: In stack memory, the only data types stored are primitive ones like int, char, byte, short, boolean, long, float, and double.
- Heap memory: In heap memory, data types that are not primitive, like strings, are stored. The stack memory holds a reference to this location.
The figure provides an example of stack and heap memory and how primitive and non-primitive data types are stored.
The Java String class uses a string pool to store strings. This is similar to the way objects are allocated. The string pool is empty by default but uses some space in the heap when we create a string. If we create many strings, this can use a lot of memory and be costly. However, JVM optimizes how the strings are stored using the string constant pool to improve performance and reduce memory usage.
JVM will check the string pool first if a new string is created. If it finds the same string, it will return the existing instance of that string to the variable rather than creating a new one. If no matching string is found, only a new literal will be created and memory allocated.
The process of string interning in Java helps improve the performance of your program by searching for identical strings in the string pool and sharing memory with other strings with the same value.
Different Ways to Create Strings
There are three different ways to create a string:
- Using String literal
- Using new keyword
- Using String.intern() method
Using String literal
The simplest way to declare a string is by using double quotes.
String <variable name> = “<value of string>”;
Example:
String str = "Software"; String str1 = "Software"; String str2 = "Testingo";
Here, the first string gets created in the string pool. When the compiler executes the second line, it checks to see if a string already matches str1 in value in the string pool. If the JVM finds any matching value, then instead of creating a new object, it simply returns a reference to that existing object.
But when the compiler executes the 3rd statement again, it checks for the string in the string pool as it will not be able to find any string with that value so it will create that string in the string pool.
Using New Keyword
This is another great way to create strings using the ‘new’ keyword and a constructor. The ‘new’ keyword guarantees that a new instance will always be created, even if the same value was used previously. These strings are stored in heap memory rather than the string constant pool.
The following method is used when we want to create an identical string outside of the string constant pool. In other words, when we want to create another object with the same value as an existing string.
String <variable name> = new String(“<value of the string>”);
String str = "Software"; String str1 = new String("Software");
Using String.intern() method
Wouldn’t it be great if we could have all of our strings with the same value placed inside the string constant pool? We can make this happen using the String.intern() method!
This String.intern() method places a string in the pool regardless of whether or not that exact string is already present.
String <variable name> = new String(<"string value">).intern(); OR <String name>.intern();
Example:
String str = "Software"; String str1 = new String("Software"); str.intern(); String str2 = new String("Testingo").intern();
Even though the first two strings have the same values, they get created in the string pool because of the String.intern() method!
Program: Basic string Program
package com.SoftwareTestingo.String; public class StringConstantPool { public static void main(String[] args) { //it will get stored in the string pool String str1 = "Software"; String str2 = "Testingo"; //str3 will not be created in the string pool String str3 = "Software"; //stored in Java heap memory String str4 = new String ("Software"); //stored in Java heap memory String str5 = new String ("Testingo"); //it will be stored in string pool String str6 = new String ("Software").intern(); //returns false because both object reference is different System.out.println(str1 == str4); //returns false //str2 occupies space in string pool and str5 occupies space in Java heap System.out.println(str2 == str5); //it returns true //address of both strings are the same System.out.println(str1 == str3); } }
Advantages of String Pool in Java
- Java’s String Pool is an amazing way to cache strings and improve performance! Storing data in a cache can reduce memory usage and keep things running smoothly.
- It’s not just about saving time – although that’s important, too. When you’re reusing strings, you’re being efficient in more ways than one. Not only are you avoiding the hassle of creating brand new strings, but you’re also freeing up memory for other things your program might need to use.
Disadvantages of Using String Objects
Strings always have the same value, even if they’ve changed. A new object is created instead of reflecting changes in the original string. If you keep updating the value of the string, this causes many objects to be created in the heap and wastes a lot of memory.
To take advantage of the benefits provided by the String class, Java also provides the StringBuffer and StringBuilder classes. These two classes allow for mutable string objects, which can be incredibly useful in some situations.
What is meant by String Pool in Java?
String Pool in Java is a special area in Java Heap memory where string literals are stored.
Where is the String Pool stored?
The string Pool is stored in the Heap Memory.
Why do we need a String Pool in Java?
It is created to decrease the number of string objects in the memory. Whenever a new string is created, JVM first checks the string pool. If it encounters the same string, it returns the same instance of the found string instead of creating a new string to the variable.
Why is String immutable in Java?
An immutable object is an object whose internal state remains constant after it has been created. Strings are immutable as references can be treated as normal variables, and we can pass them around in the code, between methods, without worrying about whether the actual String object to which it is pointing will change.
Does string pool make Java more memory efficient?
Yes, a string pool helps to save memory by preserving immutable strings in a pool so that the instances can be reused.