Static Binding and Dynamic Binding in Java: To help the Java community, Java developers, and testers who are working with Java programming language, we have prepared a core Java tutorial post. We have shared the complete post on polymorphism in Java and the different types.
After discussing that, in this post, we will discuss another important concept of Java, which every Java programmer uses. That’s why you should be aware of static and dynamic binding in Java. It is directly related to the execution of Java program code.
What Is Binding?
As we have seen in Method overloading and Method Overriding, it’s always tricky to select or find which one will be used in run time if there is more than one method of the same name or two variables having the same.
We can resolve this issue by using static and dynamic binding, but for those Java learners who do not know what binding is? Put, “Binding is a process of linking which method or variable is going to be called as per the code [ Linking between a method call and method definition].”
Some bindings are resolved during compile time, and some are dependent upon the object and resolved during runtime when the object is available.
Types Of Binding
There are two types of Binding in Java:
- Static Binding Or Early Binding
- Dynamic Binding Or Late Binding
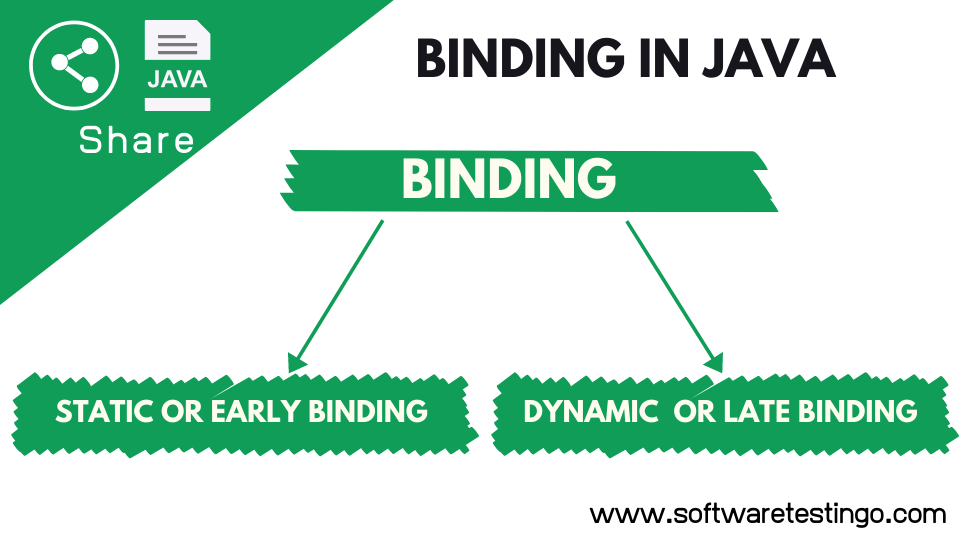
Static Binding Or Early Binding
The Binding, resolved at compile time by the JVM compiler, is called Static Binding Or Early Binding. The Binding for static, private, and final methods is completed at the compiled time because static, private, and final methods can not be overridden, and the class of those methods is determined at compile time.
Let’s take a look at a simple program to understand this concept more clearly:
package com.SoftwareTestingo.JavaBasics; class basevehicle { public static void speed() { System.out.println("The Speed Of Vehicle Class"); } } public class Static_Binding extends basevehicle { public static void speed() { System.out.println("The Speed of Static Binding Class"); } public static void main(String[] args) { //Obj is Reference & object of Base vehicle class and basevehicle obj=new basevehicle(); //obj1 is reference of base vehicle class and Object is of Static_Binding basevehicle obj1=new Static_Binding(); obj.speed(); obj.speed(); } }
Output:
The Speed Of Vehicle Class The Speed Of Vehicle Class
Dynamic Binding Or Late Binding
Sometimes, the binding process is not completed at the compile-time and waits for the object creation. After the creation of the object, binding is completed during the run time; such a type of binding process is called Dynamic Binding Or Late Binding.
One of the perfect examples of Dynamic Binding is method overriding because both a parent class and child class have the same method name in overriding. Still, the object type is determined by which method will execute at run time. So, it’s an example of dynamic binding.
package com.SoftwareTestingo.JavaBasics; class basevehicleclass { public void speed() { System.out.println("The Speed Of Vehicle Class"); } } public class Dynamic_Binding extends basevehicleclass { public void speed() { System.out.println("The Speed of Dynamic Binding Class"); } public static void main(String[] args) { //Reference & Object of Base Vehicle Class basevehicleclass obj=new basevehicleclass(); //Reference is of Base Vehicle Class & Object is of Dynamic Binding Class basevehicleclass obj1=new Dynamic_Binding(); obj.speed(); obj1.speed(); } }
Output:
The Speed Of Vehicle Class The Speed of Dynamic Binding Class
Ref: article