The SortedSet interface is part of the java.util package and extends the Set interface from the collection framework. The SortedSet interface in the Java Collections framework stores elements in a set according to some order.
Post On: | SortedSet In Java |
Post Type: | Java Tutorials |
Published On: | www.softwaretestingo.com |
Applicable For: | Freshers & Experience |
Get Updates: | SoftwareTestingo Telegram Group |
A set is used to provide a particular ordering of its elements. The elements are ordered either by using a natural ordering or a Comparator. All the elements inserted into a sorted set must implement the Comparable interface for them to be properly sorted.
The set’s iterator will go through the set in ascending order. Several other operations can be used to make the most of the ordering. All elements must be able to be compared with each other.
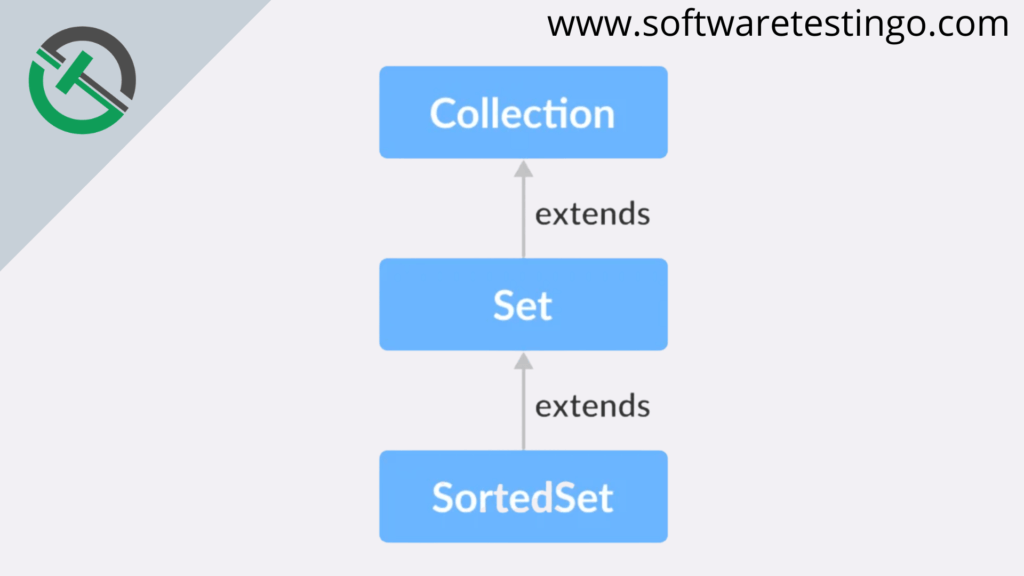
The class that implements SortedSet
To use the functionalities of the SortedSet interface, we need to use the TreeSet class that implements it.
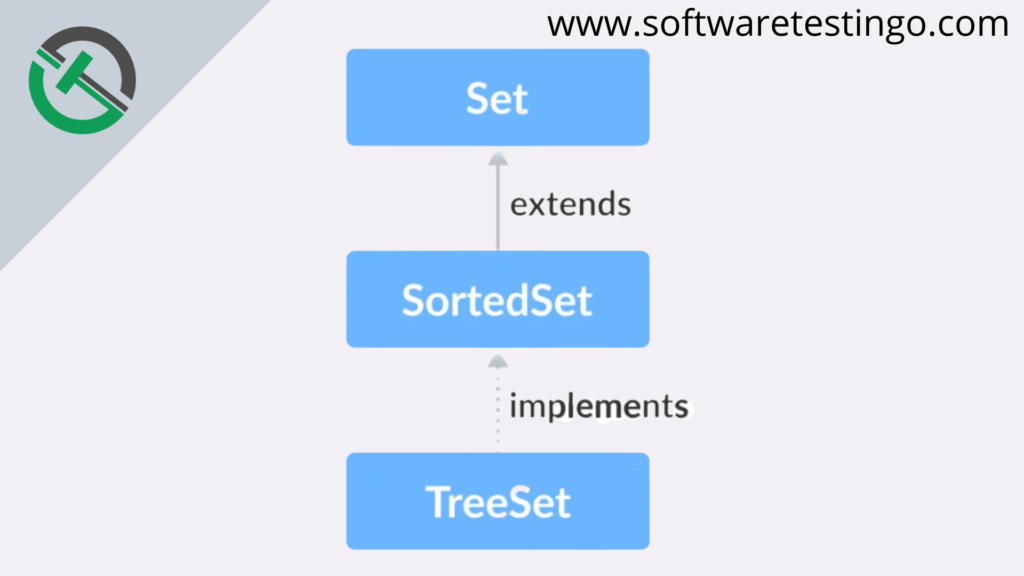
How to use SortedSet?
To use SortedSet, we must first import the java.util.SortedSet package.
// SortedSet implementation by TreeSet class SortedSet<String> testing= new TreeSet<>();
Performing Various Operations on SortedSet
SortedSet is an interface, meaning it can only be used with a class that implements it. TreeSet is a class that implements SortedSet. Here’s how to do some common operations on TreeSet.
- Adding Elements
- Accessing the Elements
- Removing the Values
- Iterating through the SortedSet
Adding Elements
To add an element to the SortedSet, we can use the add() method. However, please remember that the insertion order is not retained in the TreeSet. This is because, internally, the values are compared and sorted in ascending order for every element.
It’s important to keep in mind that duplicate elements are not allowed and will be ignored. Additionally, SortedSet does not accept null values.
package com.SoftwareTestingO.collections; import java.util.SortedSet; import java.util.TreeSet; public class AddingElementInSortedSet { public static void main(String[] args) { SortedSet<String> obj= new TreeSet<String>(); // Elements are added using add() method obj.add("Learn"); obj.add("Software"); obj.add("Testing"); obj.add("Learn"); // Printing All the Elements of SortedSet System.out.println(obj); } }
Accessing the Elements:
After adding elements to a data structure, we can use inbuilt methods like contains(), first(), last(), etc., to access the elements.
package com.SoftwareTestingO.collections; import java.util.SortedSet; import java.util.TreeSet; public class AccessingElementInSortedSet { public static void main(String[] args) { SortedSet<String> obj= new TreeSet<String>(); // Elements are added using add() method obj.add("Learn"); obj.add("Software"); obj.add("Testing"); obj.add("Learn"); // Printing All the Elements of SortedSet System.out.println(obj); String check = "Blog"; // Check if the above string exists in the SortedSet System.out.println("Contains: " + check + " - " + obj.contains(check)); // Print the first element in the SortedSet System.out.println("First Value is: " + obj.first()); // Print the last element in the SortedSet System.out.println("Last Value is: " + obj.last()); } }
Removing the Values:
You can remove values from the SortedSet using the remove() method.
package com.SoftwareTestingO.collections; import java.util.SortedSet; import java.util.TreeSet; public class RemoveElementInSortedSet { public static void main(String[] args) { SortedSet<String> obj= new TreeSet<String>(); // Elements are added using add() method obj.add("Learn"); obj.add("Software"); obj.add("Testing"); obj.add("www.SoftwareTestingo.com"); System.out.println("Before Removing Element: " + obj); // Removing the element Testing obj.remove("Testing"); System.out.println("Before Removing Element: " + obj); } }
Iterating through the SortedSet:
There are various ways to iterate through the SortedSet. The most common way is to use the enhanced for loop.
package com.SoftwareTestingO.collections; import java.util.SortedSet; import java.util.TreeSet; public class IteratingElementsinSortedSet { public static void main(String[] args) { SortedSet<String> obj= new TreeSet<String>(); // Elements are added using add() method obj.add("Unit Testing"); obj.add("UAT Testing"); obj.add("Testing"); obj.add("Automation"); // Iterating though the SortedSet for (String value : obj) System.out.print(value+ ", "); System.out.println(); } }
Methods of SortedSet
The SortedSet interface includes all the methods of the Set interface. This is because Set is a super interface of SortedSet. In addition to methods included in the Set interface, the SortedSet interface also includes these methods:
Method | Description |
---|---|
Comparator comparator( ) | Returns the invoking sorted set’s comparator. If the natural ordering is used for this set, null is returned. |
Object first( ) | Returns the first element in the invoking sorted set. |
SortedSet headSet(Object end) | Returns a SortedSet containing those elements less than the end in the invoking sorted set. The invoking sorted set also references Elements in the returned sorted set. |
Object last( ) | Returns the last element in the invoking sorted set. |
SortedSet subSet(Object start, Object end) | Returns a SortedSet that includes those elements between the start and end.1. The invoking object also references Elements in the returned collection. |
SortedSet tailSet(Object start) | Returns a SortedSet containing elements greater than or equal to the start contained in the sorted set. The invoking object also references elements in the returned set. |
package com.SoftwareTestingO.collections; import java.util.Iterator; import java.util.SortedSet; import java.util.TreeSet; public class SortedSetExample { public static void main(String[] args) { SortedSet<String> obj= new TreeSet<String>(); // using add() method add elements into the TreeSet obj.add("Testing"); obj.add("Manual Testing"); obj.add("Automation Testing"); // Displaying the TreeSet Before Adding the Duplicate element System.out.println("Before Adding the Duplicate element" +obj); // Adding the Duplicate Element obj.add("Testing"); // Displaying the TreeSet After Adding the Duplicate element System.out.println("After Adding the Duplicate element"+obj); // Removing items from TreeSet obj.remove("Manual Testing"); System.out.println("Set after removing "+ "Manual Testing:" + obj); // Iterating over Tree set items System.out.print("Iterating over set:"); Iterator<String> i = obj.iterator(); while (i.hasNext()) System.out.print(i.next()+" "); } }