Nested interfaces in Java are a core feature of the Java programming language. You can find some organizations that are particularly interested in maintaining the code base so that the representation of code is clear and well structured; in such cases, the inner interface helps us. code is necessary.
A nested interface in Java is an interface that is defined within another interface or class. This concept allows you to logically group related interfaces and provide a clear hierarchical structure to your code. Nested interfaces are similar to regular interfaces but have some unique characteristics and use cases.
This blog post will teach us how to write our code with Class and Interface, Syntax, and use cases with inner interface in Java example.
Nested Interface Key Points
When working with inner interfaces in Java, there are several important points to remember to ensure proper usage and maintain code clarity. Here are the key points to keep in mind:
Nested Interface Syntax
Nested interfaces are declared within the scope of another interface or class using the interface keyword. We can mention a nested interface within a class or interface. So, let’s see the nested interface syntax for both class and interface.
Nested Interface Syntax For Class
You can define an inner interface like the one below:
interface OuterInterface { // Other members of the outer interface interface NestedInterface { // Members of the inner interface } } class MyClass implements OuterInterface.NestedInterface { // Implement methods of the nested interface }
In this example, Nested Interface is defined within OuterInterface. The class MyClass implements OuterInterface.NestedInterface and provides implementations for its methods.
Nested Interface Syntax For Interface
Similarly, when you want to declare an interface inside a class, then you can follow the below the syntax:
class OuterClass { // Other members of the outer class interface NestedInterface { // Members of the inner interface } } class AnotherClass implements OuterClass.NestedInterface { // Implement methods of the nested interface }
Here, NestedInterface is declared within OuterClass. The class AnotherClass implements OuterClass.NestedInterface and provides implementations for its methods.
Nested Interfaces are Static by Default
When you declare an inner interface in Java, it’s considered static by default, regardless of whether you explicitly use the static keyword.
This means you don’t need an instance of the outer class or interface to access the nested interface. It’s like having a separate, standalone interface that doesn’t rely on anything else.
Accessing Nested Interfaces
To use an inner interface, you refer to it using the name of the outer interface or class it’s nested inside. It’s as if you’re saying, “I want to access this inner part of the outer thing.” This allows you to organize your code and access related interface definitions easily.
Example:
OuterInterface.NestedInterface
OuterClass.NestedInterface
Access Modifiers for Nested Interfaces
When you declare a nested interface inside a class, you can give it any access modifier you like, such as public, private, or protected. However, if the inner interface is inside another interface, it’s public by default. In other words, it’s visible to everyone. Think of it as declaring whether the inner part should be public or kept private within the outer thing.
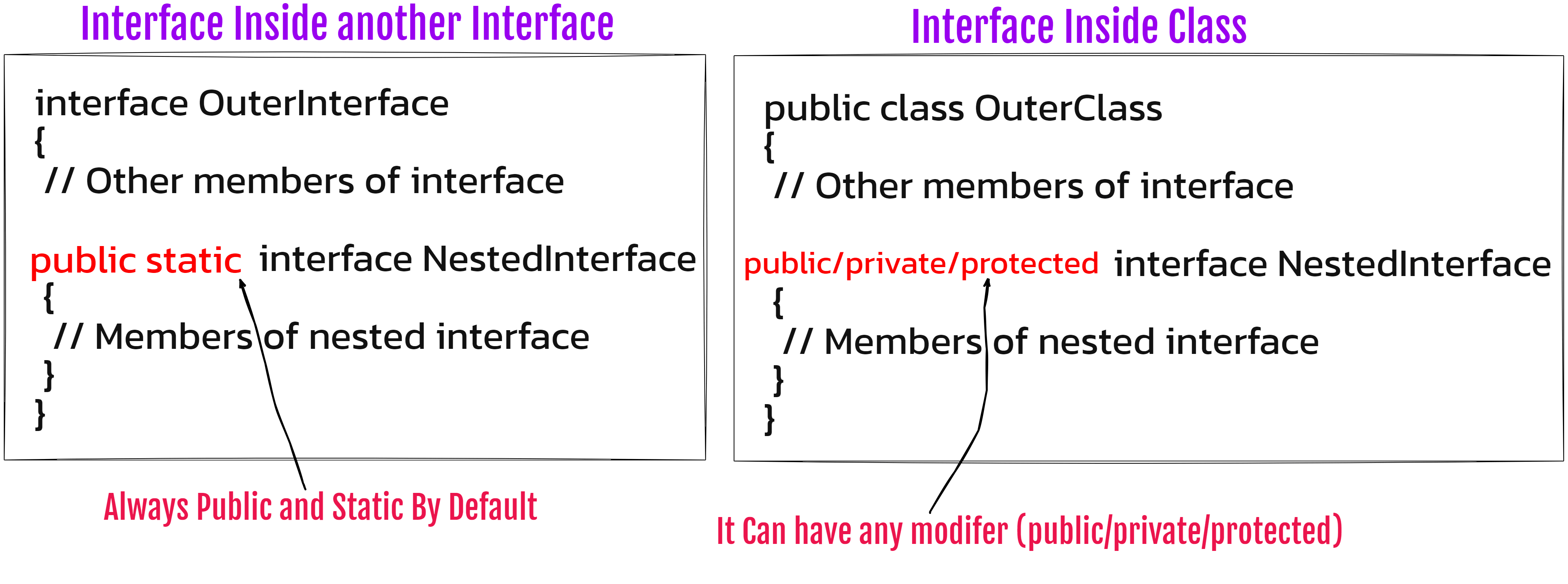
Implementing Inner Interfaces
If a class implements an inner interface (one nested inside an outer interface), it only needs to implement the methods declared in that inner interface. It doesn’t have to worry about implementing methods from the outer interface. This approach keeps things focused, like saying, “I’ll only handle the specific tasks required by this inner part.”
Implementing Outer Interfaces
Conversely, when a class implements an outer interface, it must implement only the methods defined in that outer interface. There’s no need to implement methods from any inner interfaces. This simplifies the class’s responsibilities, as it focuses on fulfilling the duties of the entire outer structure rather than delving into the details of inner interfaces.
Nested Interface Inside Interface In Java Example
In the example below, we have created a nested interface inside an interface and will see how this works.
package com.softwaretestingo.nestedinterface; //Outer interface interface Vehicle { void start(); // Nested-interface interface Engine { void ignite(); } } //Class implementing the inner-interface class Car implements Vehicle.Engine { @Override public void ignite() { System.out.println("Car engine ignited."); } } public class NestedInterfaceInsideInterfaceEx { public static void main(String[] args) { Car myCar = new Car(); // Calling the method from the nested interface myCar.ignite(); // Accessing the outer interface's method giving error //myCar.start(); } }
The output of the above program will be:
Car engine ignited.
The above example showcases how nested interfaces can organize related interface definitions and how classes can implement these inner interfaces to provide specific functionality.
Nested Interface Inside Interface In Java Example
Now, we will see another program where we will try to execute another scenario: a nested interface inside a class.
package com.softwaretestingo.nestedinterface; //Outer class class School { void attendClasses() { System.out.println("Students attend classes."); } // Nested-interface interface Teacher { void teach(); } } //Class implementing the nested-interface class MathTeacher implements School.Teacher { @Override public void teach() { System.out.println("Math teacher is teaching."); } } public class NestedInterfaceInsideClassEx { public static void main(String[] args) { School school = new School(); // Calling the method from the outer class school.attendClasses(); MathTeacher mathTeacher = new MathTeacher(); // Calling the method from the nested interface mathTeacher.teach(); } }
The output will be:
Students attend classes. Math teacher is teaching.
This example demonstrates how a nested interface can be declared inside a class, and classes can implement these nested interfaces to provide specific functionality. It helps organize related interface definitions within a class and maintains a clean code structure.
Nested Interface Usage Or Advantages
Nested interfaces in Java offer several uses and benefits in software development. They are a powerful tool for organizing code, enhancing encapsulation, and improving code readability. Here are some common uses of nested interfaces:
- Code Organization: One primary use of nested interfaces is organizing related interface components within a single logical unit. This helps in structuring code and making it more manageable.
- Reducing Name Conflicts: By nesting interfaces within a class or an outer interface, you can reduce the likelihood of name conflicts. This is especially valuable in larger codebases where naming clashes can be problematic.
- Enhanced Encapsulation: Nested interfaces can encapsulate definitions within a class or outer interface. This encapsulation hides the details of the inner interface from the outside world, promoting better encapsulation and reducing complexity.
- Maintaining a Clear Hierarchy: When you have a hierarchy of interface definitions, nested interfaces help you maintain a clear structure. Inner interfaces can represent more specific behaviors related to the outer interface, creating a natural hierarchy.
- Readability and Documentation: Inner interfaces improve code readability. They convey the relationship between interfaces and their intended use. Properly documented nested interfaces make understanding your code easier for other developers.
- Grouping Related Functionality: Nested interfaces are useful for grouping related functionality or capabilities within an outer class or interface. This grouping makes it easier to identify and work with specific methods.
- Reducing Scope: Inner interfaces can have different access modifiers (public, private, protected, or package-private). This allows you to control the visibility and scope of inner interfaces as needed, restricting access to only relevant parts of your code.
- Modularization: Nested interfaces can be used to modularize your code. You can define interfaces closely related to specific components or modules within your application, making managing and maintaining them easier.
- Library and Framework Design: When designing libraries or frameworks, nested interfaces can be valuable for defining contracts and extension points. They allow you to specify more granular requirements while maintaining the overall structure of your framework.
- Versioning and Compatibility: Nested interfaces can help with versioning and compatibility in evolving codebases. You can introduce new inner interfaces without affecting existing implementations of the outer interface, minimizing backward compatibility issues.
Conclusion:
In this blog post, we’ve explored various topics of nested interfaces, like the concept of “Nested Interface In Java” and how it can be implemented within both interfaces and classes.
Nested interfaces provide a structured and organized way to define related interface components within your code. We’ve seen examples of nested interfaces inside interfaces and classes, highlighting their versatility and usefulness.
If you have any doubts or questions regarding nested interfaces in Java, please don’t hesitate to ask in the comment section below. Your questions are valuable; I’m here to provide answers and clarifications.
Additionally, if you have any suggestions or ideas on improving this article or topics you’d like to see covered, please feel free to share your feedback in the comment section. Your input helps us create more informative and helpful content. Thank you for reading!