This tutorial will teach us about the Java NavigableSet interface and its methods. We will use an example to help illustrate how these concepts work.NavigableSet in Java with Examples.
Post On: | NavigableSet in Java |
Post Type: | Java Tutorials |
Published On: | www.softwaretestingo.com |
Applicable For: | Freshers & Experience |
Get Updates: | SoftwareTestingo Telegram Group |
The NavigableSet interface represents a navigable set in the Java Collection Framework. It inherits from the SortedSet interface and provides navigation methods in addition to the sorting mechanisms of the SortedSet.
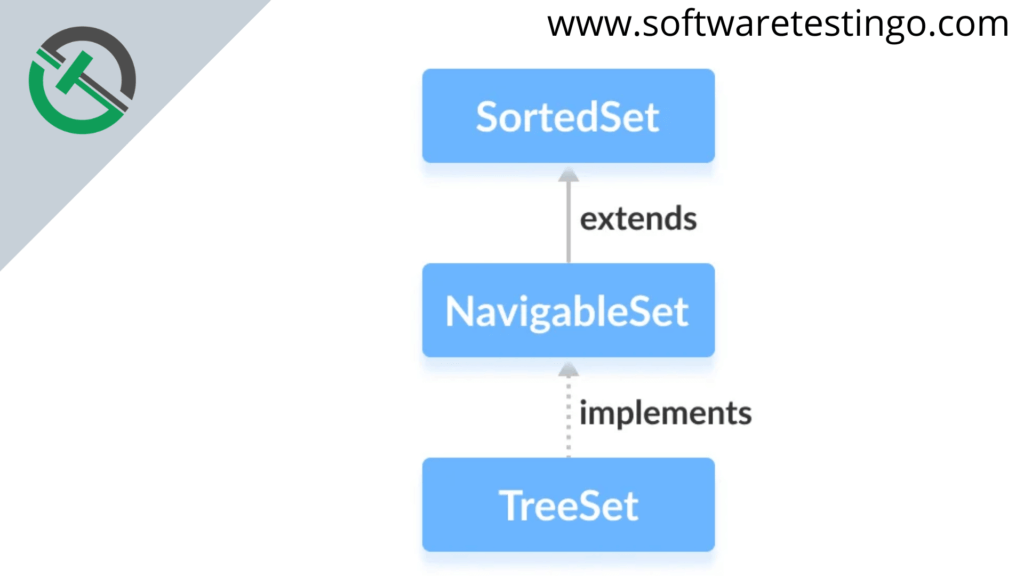
Since NavigableSet is an interface, we always need a class that extends this interface in order to create an object. You can use Generics to restrict the type of object that can be stored in a NavigableSet. This way, you can create a type-safe set.
// SortedSet implementation by TreeSet class NavigableSet<String> numbers = new TreeSet<>();
Methods of NavigableSet
NavigableSet is an interface that can be used with a class that implements it. TreeSet is one such class. Let’s look at how to perform some common operations on TreeSet.
Adding Elements:
You can use the add() method to add an element to a NavigableSet. However, remember that the insertion order will not be retained if you’re using a TreeSet because it internally sorts values in ascending order by comparing them.
It’s important to keep in mind that duplicate elements are not allowed in a NavigableSet. All duplicate elements will be ignored. Additionally, Null values are not accepted by a NavigableSet.
package com.SoftwareTestingO.collections; import java.util.NavigableSet; import java.util.TreeSet; public class NavigableSetAdd { public static void main(String[] args) { NavigableSet<String> sto = new TreeSet<String>(); // Elements are added using add() method sto.add("Java"); sto.add("C"); sto.add("Linux"); sto.add("Java"); System.out.println(sto); } }
Accessing the Elements:
After adding elements to a data structure, we can use inbuilt methods like contains(), first(), last(), etc., to access the elements.
package com.SoftwareTestingO.collections; import java.util.NavigableSet; import java.util.TreeSet; public class NavigableSetAccessing { public static void main(String[] args) { NavigableSet<String> sto = new TreeSet<String>(); // Elements are added using add() method sto.add("Java"); sto.add("C"); sto.add("Linux"); sto.add("Java"); // Printing All the Elements System.out.println(sto); String check = "Linux"; // Check if the above string exists System.out.println("Contains: " + check + " - " + sto.contains(check)); // Print the first element System.out.println("First Value is: " + sto.first()); // Print the last element System.out.println("Last Value is: " + sto.last()); } }
Removing the Elements:
You can remove values from the NavigableSet using the remove(), pollFirst(), or pollLast() methods.
package com.SoftwareTestingO.collections; import java.util.NavigableSet; import java.util.TreeSet; public class NavigableSetRemove { public static void main(String[] args) { NavigableSet<String> sto = new TreeSet<String>(); // Elements are added using add() method sto.add("Java"); sto.add("C"); sto.add("Linux"); sto.add("Java"); sto.add("Windows"); sto.add("Mac"); sto.add("Dos"); //Print All the elements System.out.println(sto); // Removing the element sto.remove("Dos"); System.out.println("After removing element " + sto); // Remove the First element sto.pollFirst(); System.out.println("After the removal of First Element " + sto); // Remove the Last element sto.pollLast(); System.out.println("After the removal of Last Element " + sto); } }
Iterating through the NavigableSet:
There are various ways to iterate through the NavigableSet. The most common way is to use the enhanced for loop.
package com.SoftwareTestingO.collections; import java.util.NavigableSet; import java.util.TreeSet; public class NavigableSetIterate { public static void main(String[] args) { NavigableSet<String> sto = new TreeSet<String>(); // Elements are added using add() method sto.add("Java"); sto.add("C"); sto.add("Linux"); sto.add("Java"); sto.add("Windows"); sto.add("Mac"); sto.add("Dos"); // Iterating for (String value : sto) System.out.print(value + ", "); System.out.println(); } }