Generics in Java have revolutionized how we write code because they allow for type parameterization, enabling the creation of classes, interfaces, and methods that operate on different data types in a type-safe and reusable manner.
Java Generics programming was introduced in J2SE 5, and Generics revolutionized the way Java code is written by providing compile-time type checking and eliminating the need for explicit type casting. This overview will explore the key concepts and benefits of Generics in Java.
Why We Use Generics In Java?
As we all know, an object is the superclass of all classes in the Java programming language, which is one of the main reasons object reference can refer to any object. Let’s take a simple scenario to understand in a better way why we need Generics in Java:
Suppose there is a requirement where you need to create a list and store integer values in it. So to this, you may write the following piece of Java program code:
List list = new LinkedList(); list.add(new Integer(1)); Integer i = list.iterator().next();
When the compiler executes the last line, the compiler doesn’t know what data type will return, and if we store that in an Integer variable, we will get the following error.
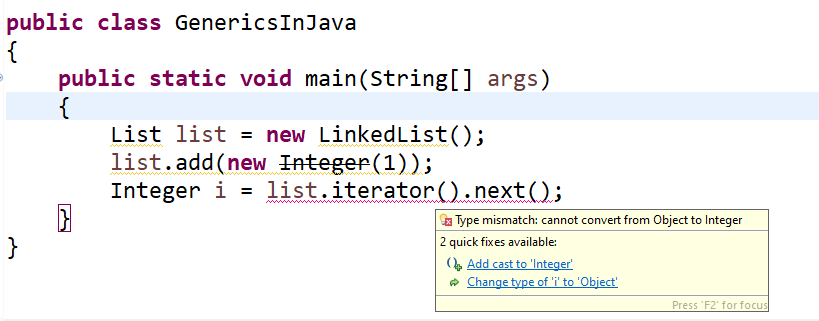
So, to fix this error, we need to do an explicit casting, like the below:
Integer i = (Integer) list.iterator.next();
So, in short, before Generics, Java programmers often used raw types (e.g., ArrayList without specifying a type) to work with collections and data structures, which could lead to runtime type errors and reduced code safety.
Generics were introduced to address these issues and provide a more structured approach to handling data of various types. For our above requirement, if Generics were used properly, you would declare the list with a specific type, like this:
List<Integer> list = new LinkedList<>();
With this declaration, the list would only allow Integer objects to be added, and you wouldn’t need to cast the result when retrieving elements. It would provide compile-time type safety, preventing you from adding elements of the wrong type and avoiding the need for explicit casting.
Advantages Of Generics In Java
Generics in Java are used for several essential reasons, which contribute to code safety, reusability, and maintainability:
- Type Safety: Generics provide compile-time type checking, ensuring you can only use compatible data types with generic classes, methods, and interfaces. This prevents type-related errors at runtime, making your code more robust and reliable.
- Code Reusability: Generics allow you to write classes, methods, and data structures with different data types. This promotes code reusability, as you can create generic algorithms and containers that can be used with various types without duplicating code.
- Cleaner Code: Using Generics often leads to cleaner and more concise code. You don’t need to write multiple versions of the same code for different data types. Instead, you can use a single generic implementation that adapts to various types.
- Compile-Time Errors: With Generics, errors related to incompatible data types are caught at compile time, which is an early stage of development. This means you can identify and fix issues before executing the code, reducing the likelihood of runtime exceptions.
- Improved Readability: Generics make code more readable and self-explanatory. When you see a generic type like List, it’s clear that this list contains strings. This improves code documentation and makes it easier for developers to understand the purpose of the code.
- Enhanced Code Quality: Generics encourage best practices in Java programming. They discourage using raw types and explicit type casting, which can lead to bugs and reduce code quality. Instead, Generics promote the use of strongly typed, safer code.
- Collections Framework: Java’s Collections Framework extensively uses Generics. Most standard data structures like ArrayList, HashMap, and LinkedList are generic classes. Using these generic collections allows you to store and manipulate data type-safely.
- API Design: Generics enables you to create more flexible and reusable components when designing APIs. For example, you can define generic interfaces or classes that work with a wide range of data types, making your API versatile and adaptable.
- Compile-Time Performance: Since Generics perform type checking at compile time, there’s no overhead at runtime for type checking or casting. This can improve performance than non-generic code requiring runtime type checking and casting.
- Maintainability: Code that uses Generics is often easier to maintain because it’s more structured and less error-prone. You can do so in a single generic implementation rather than modifying multiple specialized versions when you need to make changes or enhancements.
Java Generic Type Parameter
In Java, a generic type parameter is a placeholder for a specific data type that can be used in generic classes, interfaces, and methods. It allows you to create components with different data types while providing type safety.
Generic type parameters are enclosed in angle brackets (‘<>’) and typically represented by a single uppercase letter, such as ‘T’, ‘E’, ‘K’, or ‘V’.
Character | Data Type |
---|---|
T | Type |
E | Element (Used in Collection Framework Ex: ArrayList, Set etc) |
K | Key (Used In Map to Store Key) |
N | Number |
V | Value (Used In Map to Store Value) |
S,U,V etc. | 2nd, 3rd, 4th types |
Types Of Generics In Java
There are two primary categories of generics in Java: Generic Classes and Interfaces and Generic Methods. Each of these types serves distinct purposes in enhancing the flexibility and robustness of your Java codebase.
In this section on the generics types in Java, we will try to understand the concepts and practical applications of generic classes/interfaces and methods. Understanding these generics types is fundamental for Java developers aiming to write efficient, error-free, and adaptable code. Let’s start to understand the advantages of generics in Java programming.
Generic Classes In Java With Example
Generic classes allow you to create classes that work with different data types. These classes are parameterized with a type parameter enclosed in angle brackets (‘<>’). Examples include ArrayList, LinkedList, and custom classes like Box.
class Box<T> { private T value; public void setValue(T value) { this.value = value; } public T getValue() { return value; } } Box<Integer> integerBox = new Box<>(); Box<String> stringBox = new Box<>();
In Parameter type, we can not use primitives like ‘int,’’ char’, or ‘double.’
Example:
public class GenericsClassInJava <T> { private T content; public GenericsClassInJava(T content) { this.content = content; } public T getContent() { return content; } public static void main(String[] args) { // Create a GenericsClassInJava for Integer GenericsClassInJava<Integer> integerBox = new GenericsClassInJava<>(42); int intValue = integerBox.getContent(); System.out.println("Integer Value: " + intValue); // Create a GenericsClassInJava for String GenericsClassInJava<String> stringBox = new GenericsClassInJava<>("Hello, SoftwareTestingo User"); String stringValue = stringBox.getContent(); System.out.println("String Value: " + stringValue); // Create a GenericsClassInJava for Double GenericsClassInJava<Double> doubleBox = new GenericsClassInJava<>(3.14); double doubleValue = doubleBox.getContent(); System.out.println("Double Value: " + doubleValue); } }
Output:
Integer Value: 42 String Value: Hello, SoftwareTestingo User Double Value: 3.14
Generic Interfaces In Java With Example
Unlike generic classes, generic interfaces allow you to define interfaces with type parameters. Implementing classes specify the actual types when implementing the interface. For instance, the List interface is generic, and classes like ArrayList and LinkedList implement it.
Example:
//Define a generic interface interface Pair<T, U> { T getFirst(); U getSecond(); } //Create a class that implements the generic interface class OrderedPair<T, U> implements Pair<T, U> { private T first; private U second; public OrderedPair(T first, U second) { this.first = first; this.second = second; } @Override public T getFirst() { return first; } @Override public U getSecond() { return second; } } public class GenericsInterfaceEx <T> { public static void main(String[] args) { // Create an instance of OrderedPair with Integer and String Pair<Integer, String> pair = new OrderedPair<>(1, "One"); // Access the elements through the interface methods Integer first = pair.getFirst(); String second = pair.getSecond(); // Print the values System.out.println("First: " + first); System.out.println("Second: " + second); } }
Output:
First: 1 Second: One
Generic Methods In Java With Example
You can also create generic methods within non-generic classes. These methods have their own type parameters, independent of the class’s. This allows you to write methods that can work with various data types.
Example:
public class GenericMethodExample <T> { // Generic method to find the maximum of two values public static <T extends Comparable<T>> T findMax(T first, T second) { return first.compareTo(second) >= 0 ? first : second; } public static void main(String[] args) { // Test the generic method with integers int maxInt = findMax(5, 8); System.out.println("Maximum Integer: " + maxInt); // Test the generic method with doubles double maxDouble = findMax(3.14, 2.71); System.out.println("Maximum Double: " + maxDouble); // Test the generic method with strings String maxString = findMax("apple", "banana"); System.out.println("Maximum String: " + maxString); } }
Output:
Maximum Integer: 8 Maximum Double: 3.14 Maximum String: banana
Bounded Type Parameters In Java With Example
Bounded type parameters restrict the types used as arguments for a generic class or method. Using the extends and super keywords, you can specify upper and lower bounds for the type parameter.
Example:
// Define a generic class with a bounded type parameter class Box<T extends Number> { private T value; public Box(T value) { this.value = value; } public T getValue() { return value; } public void display() { System.out.println("Value: " + value); } } public class BoundedTypeParametersExample <T> { public static void main(String[] args) { // Create a Box of Integer Box<Integer> intBox = new Box<>(42); int intValue = intBox.getValue(); intBox.display(); System.out.println("Integer Value: " + intValue); // Create a Box of Double Box<Double> doubleBox = new Box<>(3.14); double doubleValue = doubleBox.getValue(); doubleBox.display(); System.out.println("Double Value: " + doubleValue); // Attempt to create a Box of String (which is not allowed due to the bound) // Box<String> stringBox = new Box<>("Hello"); // This line would cause a compilation error } }
Example:
Value: 42 Integer Value: 42 Value: 3.14 Double Value: 3.14
In this program:
- We define a generic class Box with a bounded type parameter T extends Number. This means that T must be a subclass of the Number class or implement the Number interface. This bound restricts the type of values stored in the Box to numeric types.
- Inside the main method, we create instances of Box with specific types (Integer and Double). These are valid because both Integer and Double extend the Number class.
- We also attempt to create a Box with a String value, which is not allowed due to the bounded type parameter. This line is commented out to avoid a compilation error.
Wildcard Types In Java With Example
Wildcard types allow you to work with unknown types in a generic context. The wildcard ‘?’ serves as a placeholder for an unknown type. Wildcards can be used with extends and super bounds to specify type constraints.
import java.util.ArrayList; import java.util.List; class Animal { public void speak() { System.out.println("Animal speaks."); } } class Dog extends Animal { @Override public void speak() { System.out.println("Dog barks."); } } class Cat extends Animal { @Override public void speak() { System.out.println("Cat meows."); } } public class WildcardTypesExample <T> { public static void main(String[] args) { List<Animal> animals = new ArrayList<>(); animals.add(new Animal()); animals.add(new Dog()); animals.add(new Cat()); // Wildcard type - accepts any subtype of Animal List<? extends Animal> wildcards = animals; // Loop through and call speak() on each element for (Animal animal : wildcards) { animal.speak(); } // Attempt to add an element (this will result in a compilation error) // wildcards.add(new Animal()); // Unbounded wildcard - can hold any type List<?> unbounded = new ArrayList<>(); unbounded.add(null); // Valid because we can add null to any type // Loop through and print elements (since it's unbounded, we can only treat them as Objects) for (Object obj : unbounded) { System.out.println(obj); } } }
Output:
Animal speaks. Dog barks. Cat meows. null
Conclusion:
By Using Generics in Java, we’ve learned how this feature enhances Java code’s type safety, reusability, and readability. Generics allow us to create classes, interfaces, and methods that work seamlessly with different data types, all while catching type-related errors at compile time. We’ve covered generic classes, generic methods, and the practical applications of generics, illustrating how they contribute to the robustness of Java programs.
Your feedback and questions are invaluable as the Java programming community continues to evolve. If you have any doubts or questions regarding Generics in Java, please don’t hesitate to comment below. Your inquiries can spark insightful discussions and help clarify any confusion.
Additionally, we welcome your suggestions for improving this article or any topics you’d like to see covered in more detail. Your input is vital in shaping our content for the Java community. Feel free to share your thoughts in the comment section. Thank you for being part of our learning community!