Common Exception Handling In Selenium Webdriver: Selenium is one of the popular automation testing frameworks that interacts with web browsers to perform user actions and validate functionality. However, during our test automation, we may encounter various exceptions that can break test execution flows. That is why, for a good programmer, it’s important to learn how to properly handle exceptions while developing robust test automation frameworks in Selenium.
The “WebDriverException in Selenium” is a base class for various exceptions in the Selenium WebDriver hierarchy. Understanding the specific WebDriverException subclass can aid in targeted exception handling, improving script reliability.
If you don’t have prior knowledge of exceptions, then you can refer to our blog posts where we have discussed in detail Exception Handling.
Different Exceptions In Selenium Webdriver
Here is the list of Selenium exceptions:
Common Webdriver Exception Handling In Selenium
Selenium WebDriver has robust built-in exception classes to handle various error conditions or exceptions during our test automation. All the runtime exceptions thrown by Selenium WebDriver are part of an exception hierarchy, with the base superclass being WebDriverException.
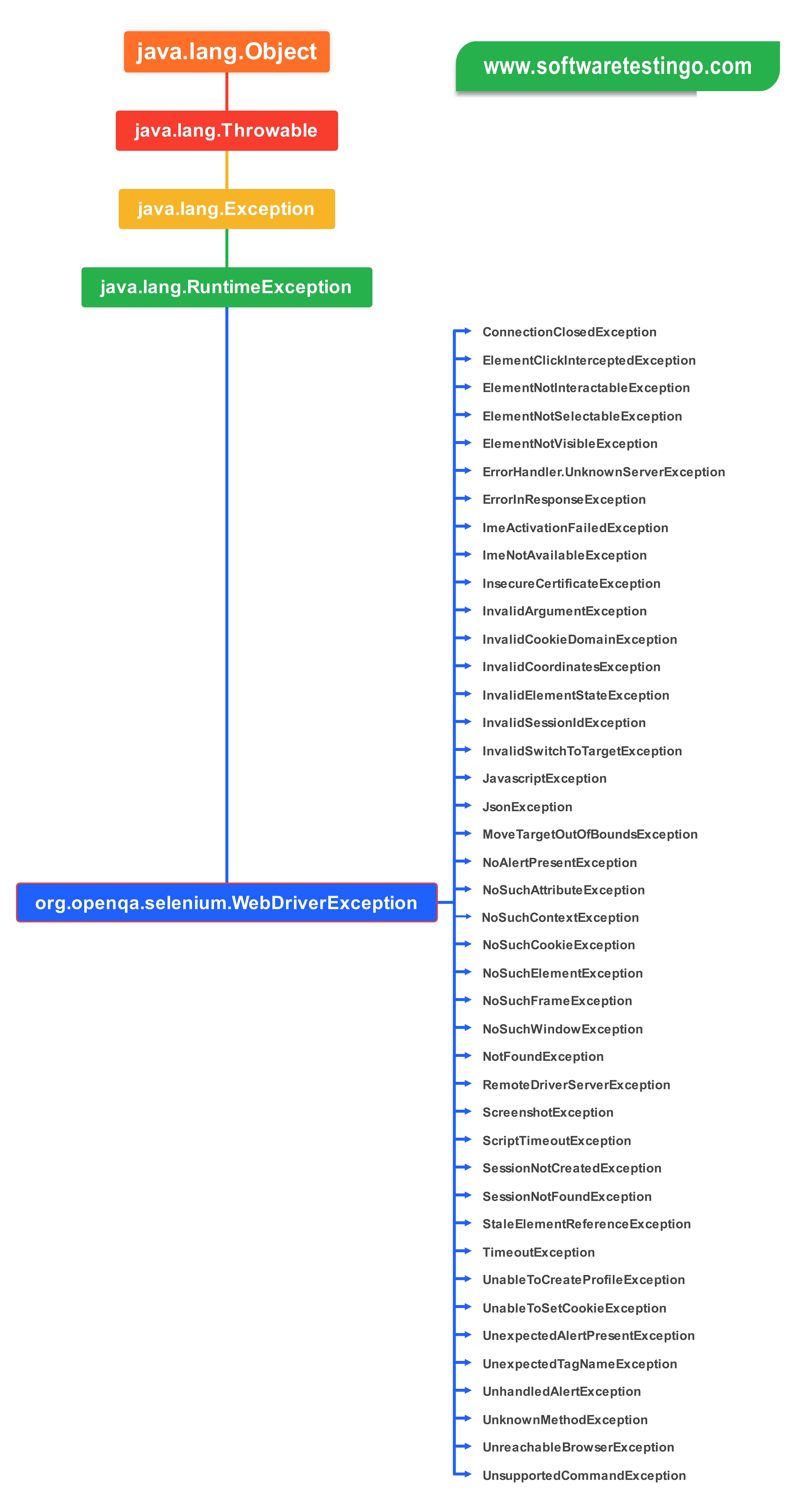
When exceptions occur in WebDriver scripts, they instantiate and throw subclasses of WebDriverException to categorize the issue. For example, ElementNotVisibleException would indicate an issue with an element not being visible, while NoSuchFrameException signifies trying to switch to a non-existent frame.
By having all exceptions inherit from WebDriverException, scripts can broadly catch and handle exceptions from the WebDriver domain as a group if desired. The subclasses refine the exceptions by type to support handling or reporting on specific issues. So, the hierarchy structure of WebDriverException allows for both generalized and targeted exception processing in automated tests.
The available Selenium exception types help testers better understand common automation errors. And the exception parenting inherits the taxonomy of exception categories found in WebDriver implementations.
WebDriverException
WebDriverException is the central exception class in Selenium WebDriver, with all Selenium automation-related runtime exceptions subclassing from it either directly or indirectly. It extends RuntimeException to function as an unchecked exception. When any error happens in browser automation code, Selenium will throw a specialized subclass, e.g. NoSuchElementException or ElementClickInterceptedException, that ultimately inherits from WebDriverException.
This enables broadly catching and handling exceptions from the WebDriver domain in try-catch blocks by catching WebDriverException. Individual subclasses offer precise exceptions for specific issue types. WebDriverException also contains a detailed error message on the cause through its message attribute. So, it serves as the parent exception class in Selenium, binding all WebDriver exceptions for generalized and targeted error handling.
StaleElementReferenceException
This happens when the referenced element no longer exists in the DOM. This often occurs because the page was refreshed, the user navigated to a different page, or the element was dynamically removed. The solution is to re-locate the element after any page changes before attempting to interact with it again. Minimize page reloads and avoid operating on elements across page changes where possible.
NoSuchElementException
This error occurs when the element locator used does not match any elements in the DOM. Reasons include typing errors in the locator, the element being dynamically removed from the page, or the page changing after the element was located. Solutions ensure the locator syntax is correct, wait for the element to load, re-locate the element after navigation, or add waits and retries to handle dynamic content.
NoAlertPresentException
This exception indicates an attempt was made to switch to or interact with a browser alert popup when one was not present. The fix is to add proper waits or synchronizations to ensure an alert is displayed before attempting alert-based operations.
NoSuchFrameException
This exception signifies attempting to switch to a frame that does not exist on the current page. Reasons can include typos in frame ID, naming changes, or the frame being dynamically removed. The solution is to check that double-specified frame IDs match the names or IDs of existing frames before switching.
NoSuchWindowException
This exception indicates trying to switch to a browser window that no longer exists or is closed. Potential fixes include re-verifying open window handles after navigation or window close events before switching windows.
NoSuchAttributeException
This exception indicates trying to access an element attribute that does not exist, often due to spelling errors or dynamic changes. The solution is to verify attribute names are correct and account for dynamically added or removed attributes in test logic.
ElementNotVisibleException
This exception indicates that a command could not be completed because the element is not visible on the page. Common reasons include the element not existing in the DOM, having display: none style, being hidden by other elements, or being outside the current viewport. To resolve, first verify the element locator is correct and that the element exists. Then, scroll the element into view and/or wait for it to become visible before interacting with it.
ElementNotSelectableException
The ElementNotSelectableException in Selenium signifies trying to select an element that is not selectable, like calling the select() command on a text field. It usually occurs when the locator identifies an element that is not an inherent selectable component, like a dropdown or list box. To fix this, the locator must be corrected to point only to proper selectable elements for selection operations.
ElementNotInteractableException
This exception suggests trying to interact with an element not in an interactable state, like clicking on a hidden or disabled element. It occurs when an element is not displayed, not enabled, or obscured by other elements from interaction. Resolutions focus on only performing actions on displayed and enabled elements, scrolling elements into view first, or sometimes, using JavaScript instead of normal interaction.
UnexpectedAlertPresentException
This exception indicates an alert popup appeared when not anticipated or handled by the test script. Fixes include adding waits and synchronizations around operations that may spawn alert popups and ensuring the code properly handles switching to and dismissing alerts.
TimeoutException
This exception indicates a Selenium command failed to complete within the allotted timeout duration, defaulting to 30 seconds. Solutions include increasing timeouts, refactoring test logic to minimize waits, optimizing target page load times, and isolating slow operations.
InvalidElementStateException
This exception signifies trying to perform an invalid operation on an element in its current state. For example, clicking a hidden element or submitting a form more than once. The solution is first checking the state of elements before interacting, scrolling elements into view, or refreshing the state after certain events.
InvalidSelectorException
This indicates the selector used to find an element does not conform to the standards of supported locator strategies like CSS, XPath, or others. The resolution is to debug and correct selector syntax to specify elements uniquely and accurately.
SessionNotFoundException
This happens when trying to make a WebDriver call without an active session. Often from test code that is outside the context of the browser session. The fix is to ensure the driver setup is correct and the session is created properly and accessible where commands are attempted.
Handling Exceptions In Selenium WebDriver
Exception handling is an important concept in Selenium to create robust test scripts that can recover from unexpected errors during execution. Using try-catch blocks, Multiple catch blocks, Throw, Multiple Exceptions, Finally and various Selenium methods, we can catch exceptions and prevent scripts from failing and skipping subsequent steps.
The main way to handle exceptions in Selenium is with try-catch blocks. The try block surrounds the code that could throw an exception like a locator not being found or an element not being clickable. The catch block then catches any exceptions thrown from the try block:
try { driver.findElement(By.id("submit")).click(); } catch (NoSuchElementException e) { System.out.println("Element not found on page"); }
If the element with ID submit cannot be found, Selenium will throw a NoSuchElementException. But the catch block handles this gracefully by catching the exception and printing a message instead of allowing the script to fail.
Some best practices for exception handling in Selenium include:
- Catch specific exceptions instead of general Exception types for precise handling.
- Log the exception details like stack trace to help debug errors
- Throw exceptions again if you can’t handle recovery. The TestNG framework will automatically mark it as a failed
- Only use try-catch blocks around risky sections of code instead of whole tests.
Selenium also provides useful methods that can avoid exceptions in the first place:
- FindElement vs FindElements – returns an empty list instead of an exception if not found.
- isDisplayed() – checks if the element is visible before interacting
- WebDriverWait – waits for elements to become available before timing out
Proper exception handling and built-in Selenium methods make tests reliable and stable. Even when the application under test changes and breaks tests, scripts can automatically recover or fail gracefully without halting the entire test suite. This results in more robust automation with less maintenance when unexpected runtime errors occur.
Methods For Displaying Exception Messages
When exceptions occur in a Java program, displaying the exception message to the user or log files is crucial for diagnosing and fixing issues. There are several common methods to display exception messages effectively:
printStackTrace(): Calling this on an Exception object prints the exception type and detailed stack trace to the console output. It shows exactly where the exception occurred in the code:
try { // risky code } catch (Exception e) { e.printStackTrace(); }
getMessage(): This returns the exception message text without stack traces. Useful for displaying concise errors to end users:
catch (Exception e) { System.out.println("Error: " + e.getMessage()); }
Logger Classes: Using java.util.logging, Log4J, SLF4J or other logger classes allows flexible logging of exception details to external log files or monitoring tools:
private static final Logger LOG = LoggerFactory.getLogger(Main.class); LOG.error("Exception occurred", e); //pass in exception object
Conclusion:
Handling exceptions smoothly is crucial for creating reliable test automation frameworks with Selenium WebDriver. We can build resilience into our scripts by leveraging built-in Java exception-handling features like try-catch blocks combined with Selenium’s exception-aware methods. Techniques like finding elements safely, catching specific exception types, and recovering when elements are missing allow testing to continue uninterrupted despite changes in the application.
I hope this overview gives you ideas on best practices for handling exceptions in your own Selenium test automation projects. Please provide any comments or suggestions for improving this guide – I welcome feedback to enhance the material and help others on their test automation journey. Share this article if you found it useful, and happy exception handling!