Comments In Java Programming: Comments in Java serve as a critical element of code documentation and clarity within the source code for human readers. They are textual annotations within the source code that are not executed as part of the program but provide valuable insights for developers and maintainers.
In this Java tutorial post, we will try to understand the significance of comments in Java, their types, best practices, and why they are indispensable in software development.
Why Do We Use Comments in Code?
Comments in codes are used to serve several important purposes, and that’s the reason for making them a basic element of software development. Here are a few key reasons why we use comments in code:
- Documentation: Comments provide a means to document the functionality of code. They describe what the code does, its purpose, and how it achieves its objectives. This documentation is invaluable for programmers who read and maintain the code in the future.
- Clarity: Comments enhance the readability of code. They break down complex logic or algorithms into understandable steps and explain the code’s components. This clarity makes it easier for developers to understand, modify, and debug the code.
- Communication: Comments facilitate communication among team members. When multiple developers work on a project, comments can serve as a form of communication to explain decisions, changes, or special considerations in the code.
- Debugging: Comments can assist debugging by providing insights into the programmer’s intent. They can help identify potential issues or suggest areas for modifications.
- Learning and Teaching: For beginners or those new to a codebase, comments act as a learning tool. They provide insights into how different parts of the code work, helping individuals understand programming concepts and best practices.
- Compliance: In certain industries or projects with strict coding standards or regulatory requirements, comments may be necessary to demonstrate compliance with guidelines and regulations.
Types of Comments In Java
In Java, there are three main types of comments:
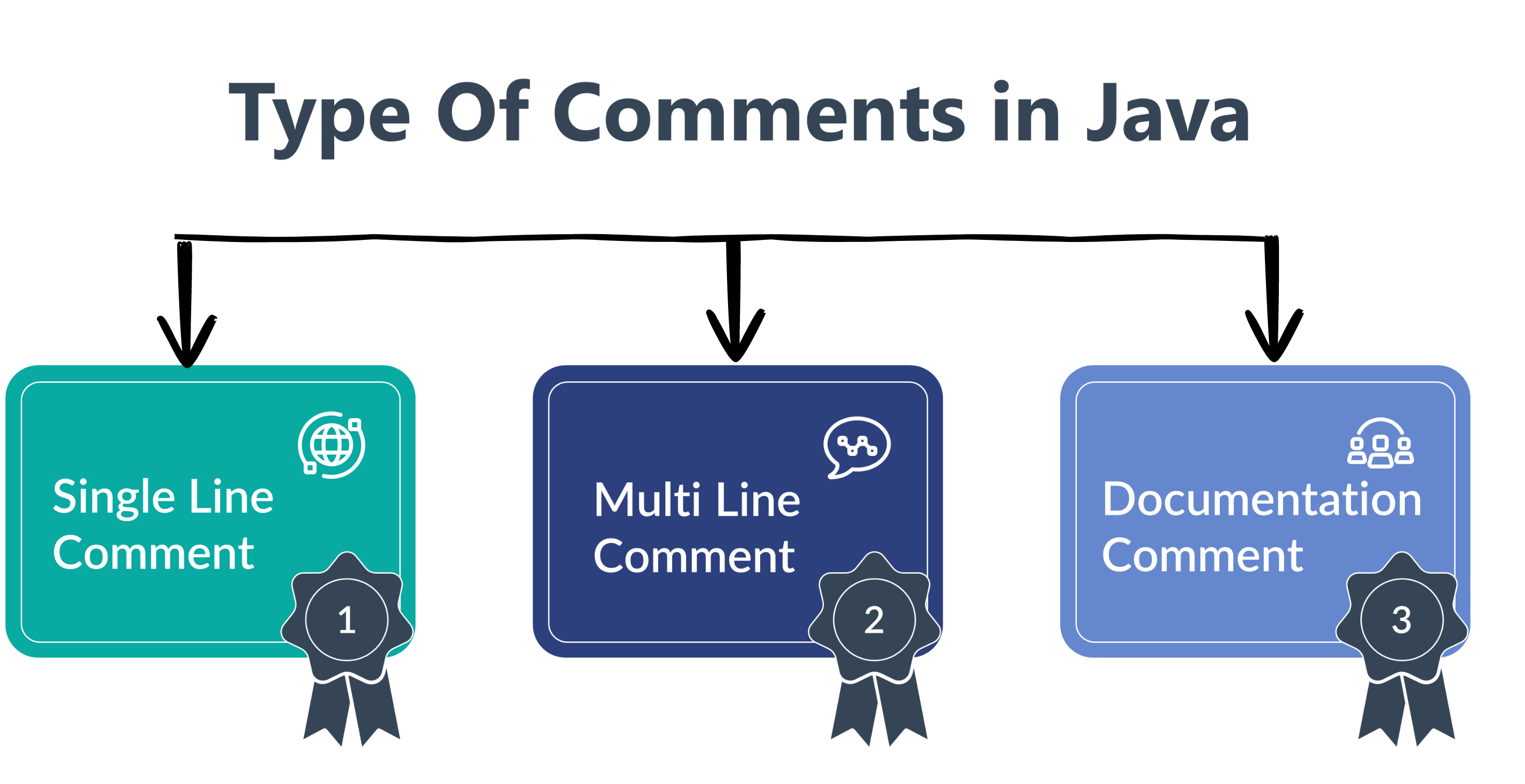
Let’s discuss each of these comments type in detail one by one:
Single Line Comment
Single-line comments are used to insert comments on a single line of code. These comments are ignored by the Java compiler and are meant to provide brief explanations or notes about a specific line of code.
To create a single-line comment, use two forward slashes // followed by the comment text. Anything written after // on the same line is treated as a comment.
- Syntax: Single-line comments are denoted by //.
- Purpose: Single-line comments are used for adding brief comments or explanations on a single line of code. They are primarily meant for providing short, context-specific notes.
- Usage: Single-line comments are handy for annotating a single statement, variable, or method call with a short comment.
// Initialize a counter variable int count = 0;
Example Of Single Line Comment:
// Java Program For Single Line Comment public class SingleLineComment { public static void main(String[] args) { // Single Line Comment Of Print Statement System.out.println("Hello SoftwareTestingO"); } }
Multi-Line Comment
Multi-line comments are enclosed within /* and */. They are used to provide more extensive explanations or temporarily exclude a block of code from execution. These comments are often used to comment on large code sections or write longer explanations.
- Syntax: Multi-line comments are enclosed between /* and */.
- Purpose: Multi-line comments are used when you need to provide more extensive comments that span multiple lines or paragraphs. They are suitable for adding detailed explanations, commenting out code blocks, or creating headers for classes or methods.
- Usage: Multi-line comments are beneficial when adding comprehensive documentation or explanations to code blocks.
// Java Program For Multi Line Comment public class MultiLineComment { public static void main(String[] args) { /*This is a multi-line comment. It can span multiple lines and is typically used for providing detailed explanations about code sections.*/ System.out.println("Hello SoftwareTestingO"); } }
Java Documentation Comments
Documentation comments are represented by “/** and */” and are a special type of comment in Java. They are used for generating documentation using tools like JavaDoc. Documentation comments provide detailed descriptions of classes, methods, variables, and their usage. They are particularly important for creating comprehensive and well-documented APIs.
These comments typically appear before a class, method, or field declaration and can include special tags for documentation generation.
- Syntax: Documentation comments are a special form of multi-line comments that start with /** and end with */.
- Purpose: Documentation comments are used to generate API documentation automatically. They are often used to describe classes, methods, fields, and their purpose, parameters, and return values in a standardized way.
- Usage: Documentation comments are essential for generating well-structured API documentation using tools like Javadoc. They follow specific conventions and tags (e.g., @param, @return) to document code elements comprehensively.
/** * This is a Javadoc comment for a method. * @param x An integer parameter. * @return The result of some operation. */ public int myMethod(int x) { // Code here }
Different Tags Used in Javadoc Comments
- Inside the Java Documentation Comments, every line begins with @, interpreted as the special instruction for the documentation generator, which gives information about the source code.
- Inside the Java Documentation Comments, you can use various Javadoc annotations, and below, we have mentioned some of the frequently used Javadoc annotations.
Tag | Description | Applies to |
---|---|---|
@see | Notes API version when the item was added | Class, method, or variable |
@code | Source code content | Class, method, or variable |
@link | Associated URL | Class, method, or variable |
@author | Author name | Class |
@version | Version number | Class |
@param | Parameter name and description | Method |
@return | Return type and description | Method |
@exception | Exception name and description | Method |
@deprecated | Declares an item to be obsolete | Class, method, or variable |
@since | Notes API version when the item was added | Variable |
Examples:
/** * Divides two numbers. * @param dividend The number to be divided. * @param divisor The number to divide by. * @return The result of the division. * @throws ArithmeticException If the divisor is zero. */ public double divide(double dividend, double divisor) throws ArithmeticException { if (divisor == 0) { throw new ArithmeticException("Division by zero is not allowed."); } return dividend / divisor; }
How to Add Comments In Eclipse?
To add comments in Eclipse IDE, you need to enter “/** [Enter]” before the public method or class, and then it will automatically add the necessary Javadoc annotation based on the method.
Example 1:
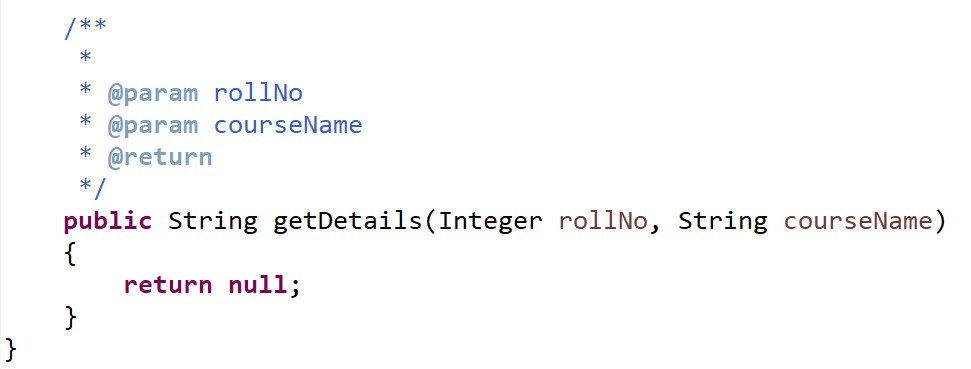
Example 2:
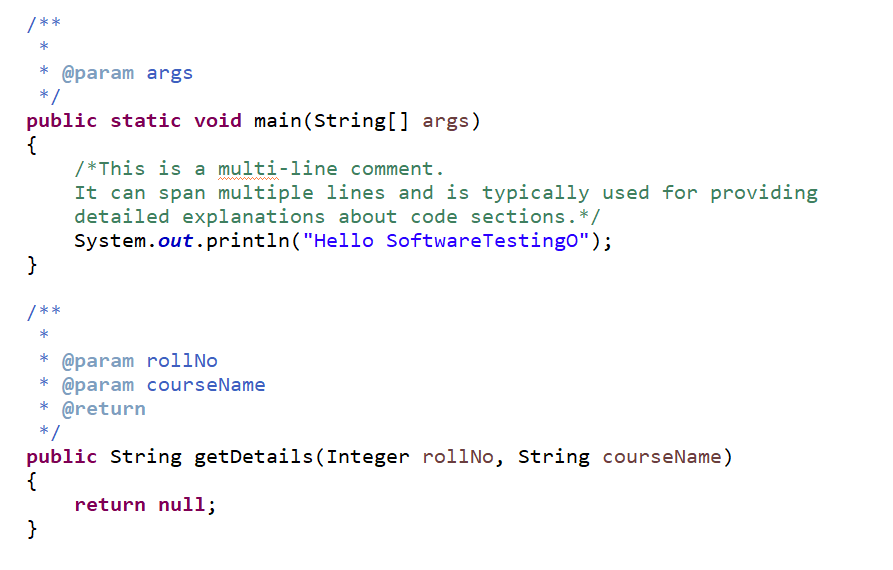
Conclusion:
Comments in Java are indispensable for enhancing code clarity, understanding, and maintainability. They come in various forms, each serving specific purposes. By following best practices in comment writing, developers can make their code more accessible and collaborative.
If you have any doubts about using comments in Java or suggestions to improve this article, please feel free to comment below. Your feedback is valuable in creating better programming practices and resources.